Using the Pipedrive API to Get Organization in PHP
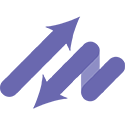
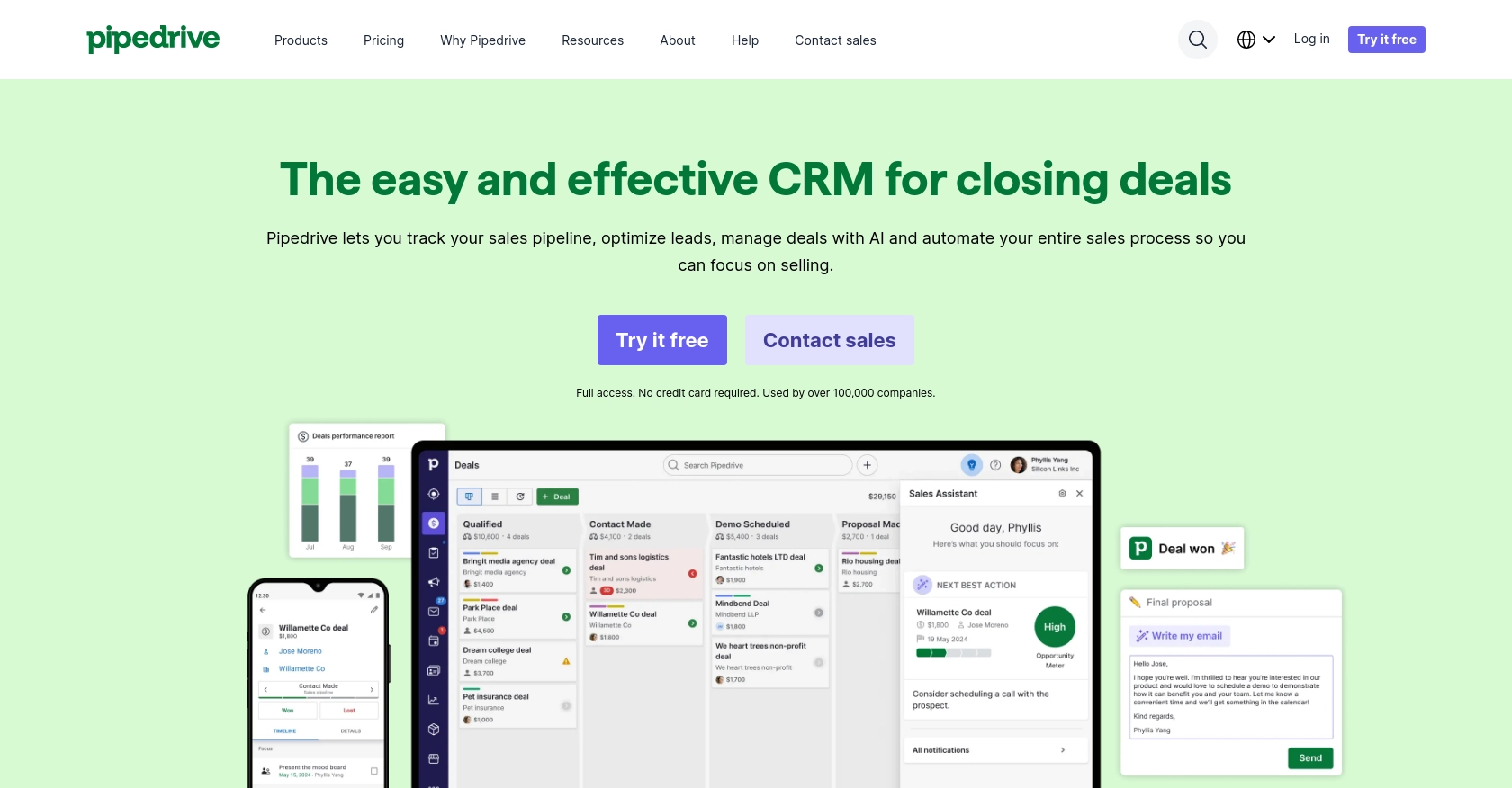
Introduction to Pipedrive CRM and API Integration
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage customer relationships, and close deals efficiently.
Developers often seek to integrate with Pipedrive's API to automate and enhance their sales workflows. By connecting to the Pipedrive API, developers can access and manipulate data such as organizations, deals, and contacts. For example, retrieving organization data using the Pipedrive API in PHP can streamline the process of syncing customer information with other business systems, ensuring that sales teams have up-to-date data at their fingertips.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can begin integrating with the Pipedrive API, it's essential to set up a developer sandbox account. This account provides a risk-free environment for testing and development, ensuring that your integration works seamlessly before going live.
Creating a Pipedrive Developer Sandbox Account
To get started, you'll need to create a developer sandbox account. This account functions like a regular Pipedrive company account but is specifically designed for development and testing purposes. Follow these steps to set up your sandbox account:
- Visit the Pipedrive Developer Sandbox Account page.
- Fill out the form to request access to your sandbox account.
- Once your request is approved, you'll receive access to the Developer Hub, where you can manage your apps and integrations.
Generating OAuth Credentials for Pipedrive API
The Pipedrive API uses OAuth 2.0 for authentication. To interact with the API, you'll need to create an app and obtain the necessary credentials:
- Log in to your Pipedrive Developer Hub.
- Navigate to the "Apps" section and click on "Create an App."
- Fill in the required details, such as the app name and description.
- Once the app is created, you'll receive a Client ID and Client Secret. Keep these credentials secure, as they are essential for authenticating API requests.
Configuring OAuth Scopes and Permissions
When setting up your app, it's crucial to configure the appropriate OAuth scopes and permissions to ensure your app has the necessary access to Pipedrive data:
- In the app settings, navigate to the "Scopes" section.
- Select the scopes that match your integration needs, such as accessing organization data.
- Ensure that your app requests only the permissions it requires to function correctly.
With your sandbox account and OAuth credentials set up, you're ready to start integrating with the Pipedrive API using PHP. This setup ensures a secure and efficient development process, allowing you to test your integration thoroughly before deploying it in a live environment.
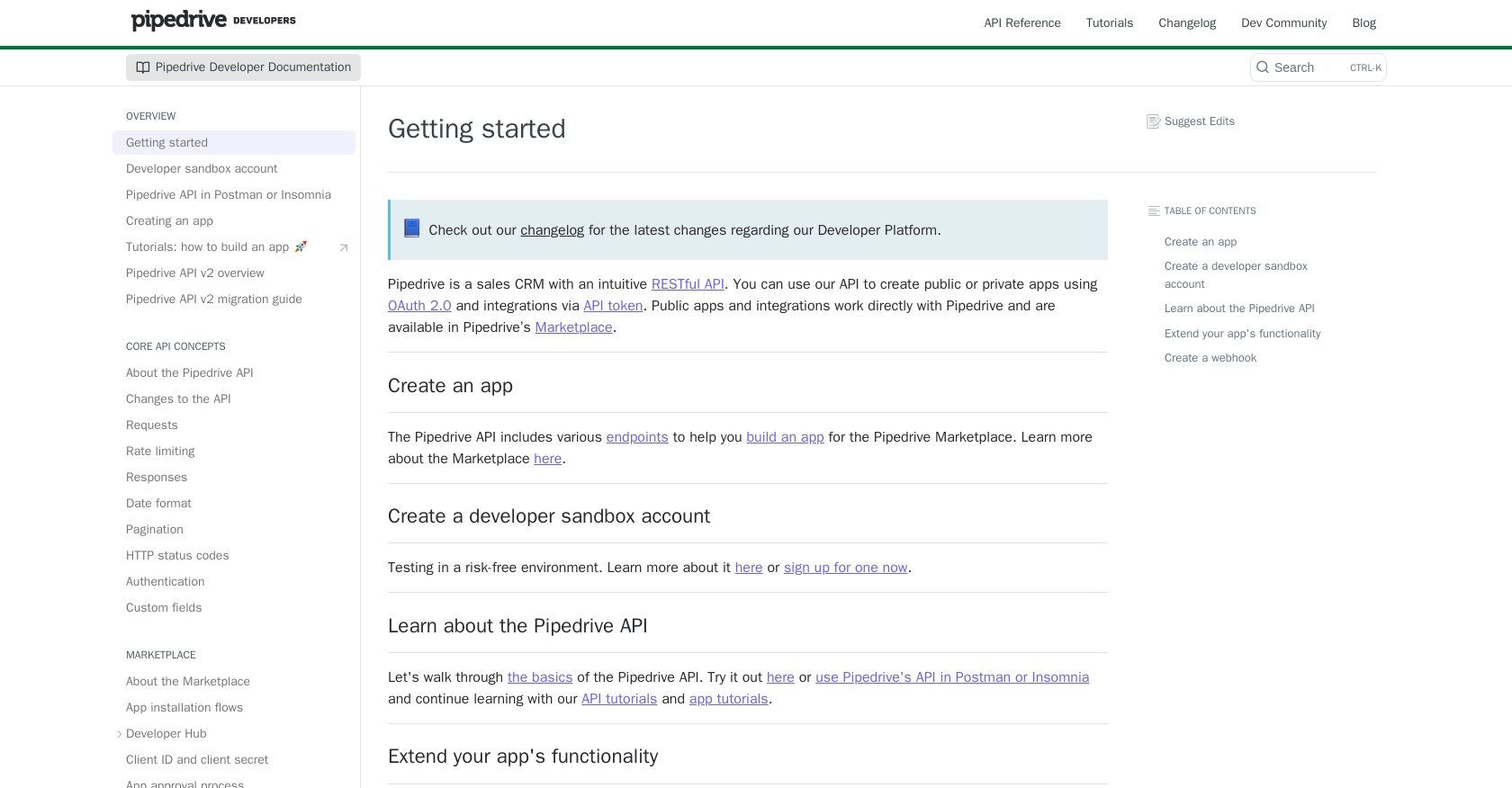
sbb-itb-96038d7
Making API Calls to Retrieve Pipedrive Organizations Using PHP
To interact with the Pipedrive API and retrieve organization data using PHP, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process of making API calls, handling responses, and managing errors effectively.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure that your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- cURL extension enabled
- Composer for dependency management
Install the required dependencies using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Organizations from Pipedrive
With your environment set up, you can now write the PHP code to make API calls to Pipedrive. The following example demonstrates how to retrieve a list of organizations:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$response = $client->request('GET', 'https://api.pipedrive.com/v1/organizations', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
]
]);
if ($response->getStatusCode() === 200) {
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $organization) {
echo 'Organization Name: ' . $organization['name'] . "\n";
}
} else {
echo 'Failed to retrieve organizations. Status Code: ' . $response->getStatusCode();
}
Replace Your_Access_Token
with the access token obtained from your OAuth setup. This code uses the Guzzle HTTP client to send a GET request to the Pipedrive API endpoint for organizations. It then processes the JSON response to display the organization names.
Handling API Responses and Errors
It's crucial to handle API responses and potential errors gracefully. The Pipedrive API returns various HTTP status codes to indicate the result of your request:
- 200 OK: Request was successful.
- 401 Unauthorized: Invalid or missing access token.
- 429 Too Many Requests: Rate limit exceeded.
For more detailed information on error codes, refer to the Pipedrive documentation.
Verifying API Call Success in Pipedrive Sandbox
After running your PHP script, verify the results by checking your Pipedrive sandbox account. Ensure that the organizations retrieved match the data in your sandbox environment. This step confirms that your integration is functioning correctly.
Managing Rate Limits and Best Practices
Pipedrive enforces rate limits on API requests. For OAuth apps, the limit is 80 requests per 2 seconds per access token. To avoid hitting these limits, consider implementing strategies such as:
- Batching requests
- Implementing exponential backoff for retries
- Using webhooks for real-time updates
For more details on rate limits, see the Pipedrive rate limiting documentation.
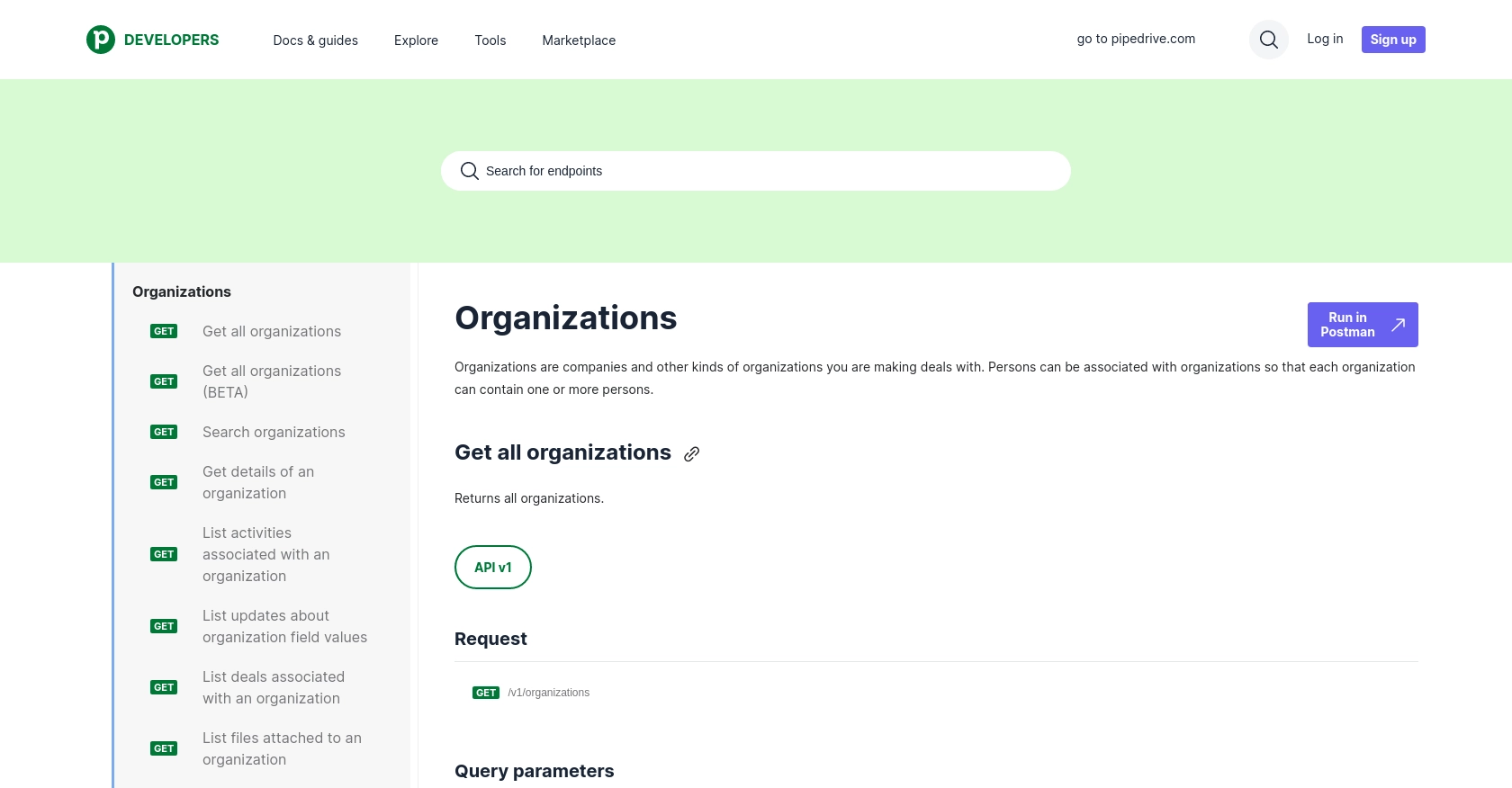
Conclusion and Best Practices for Pipedrive API Integration Using PHP
Integrating with the Pipedrive API using PHP provides a powerful way to automate and enhance your sales processes. By retrieving organization data, you can ensure that your sales team has access to the most current information, facilitating better decision-making and customer interactions.
Best Practices for Secure and Efficient Pipedrive API Integration
- Secure Storage of Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limits Gracefully: Implement strategies to manage API rate limits, such as batching requests or using exponential backoff for retries. This ensures your application remains responsive and avoids unnecessary errors.
- Data Standardization: Ensure that data retrieved from Pipedrive is standardized and transformed as needed to fit your business processes. This can include mapping custom fields or normalizing data formats.
Leveraging Endgrate for Streamlined Integration Management
While building custom integrations can be rewarding, it often requires significant time and resources. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Pipedrive. By using Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration processes and allow you to focus on your core product offerings. Visit Endgrate to learn more about how it can benefit your business.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Organizations
- https://developers.pipedrive.com/docs/api/v1/OrganizationFields
- https://developers.pipedrive.com/docs/api/v1/OrganizationRelationships
Ready to get started?