How to Create or Update Customers with the Commerce7 API in Javascript
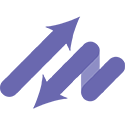
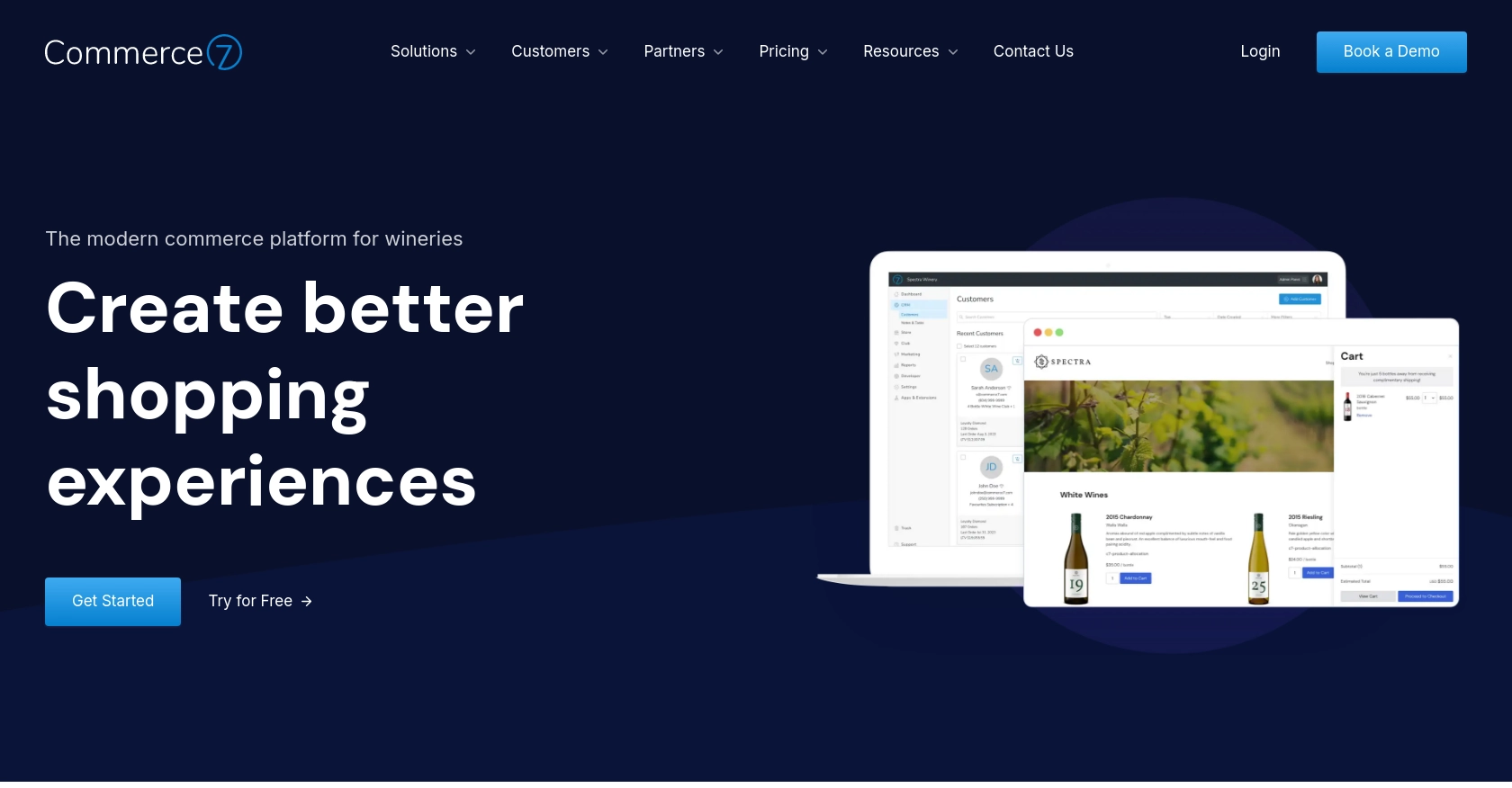
Introduction to Commerce7 API Integration
Commerce7 is a modern commerce platform designed specifically for the wine industry, offering a suite of tools to enhance customer experiences and streamline operations. With features like club memberships, reservations, and comprehensive customer management, Commerce7 empowers wineries to connect with their customers in meaningful ways.
Integrating with the Commerce7 API allows developers to manage customer data efficiently, enabling seamless updates and creation of customer records. For example, a developer might use the Commerce7 API to automatically update customer information from a CRM system, ensuring that all customer interactions are personalized and up-to-date.
Setting Up a Commerce7 Developer Account and App
Before you can start integrating with the Commerce7 API, you need to set up a developer account and create an app within the Commerce7 platform. This will allow you to access the necessary credentials for authentication and testing.
Creating a Commerce7 Developer Account
If you don't already have a Commerce7 account, follow these steps to create one:
- Visit the Commerce7 website and sign up for a developer account.
- Once registered, log in to your account and navigate to the Developers tab.
- Access the App Dev Center by going to dev-center.platform.commerce7.com.
Creating a Commerce7 App for API Access
To interact with the Commerce7 API, you'll need to create an app within the platform:
- In the App Dev Center, click the "Add App" button to start the app creation process.
- Fill in the required details for your app, such as the app name and description.
- Select the API endpoints you need access to, ensuring you include customer-related endpoints for managing customer data.
- Once your app is created, you'll receive an App ID and App Secret Key. Keep these credentials secure as they are essential for authenticating API requests.
Configuring Authentication for Commerce7 API
Commerce7 uses Basic Auth for API requests. Here's how to configure it:
- Use your App ID as the username and your App Secret Key as the password.
- Ensure that these credentials are stored securely and not exposed in your frontend code.
For more detailed information, refer to the Commerce7 App Creation Guide and Commerce7 API Documentation.
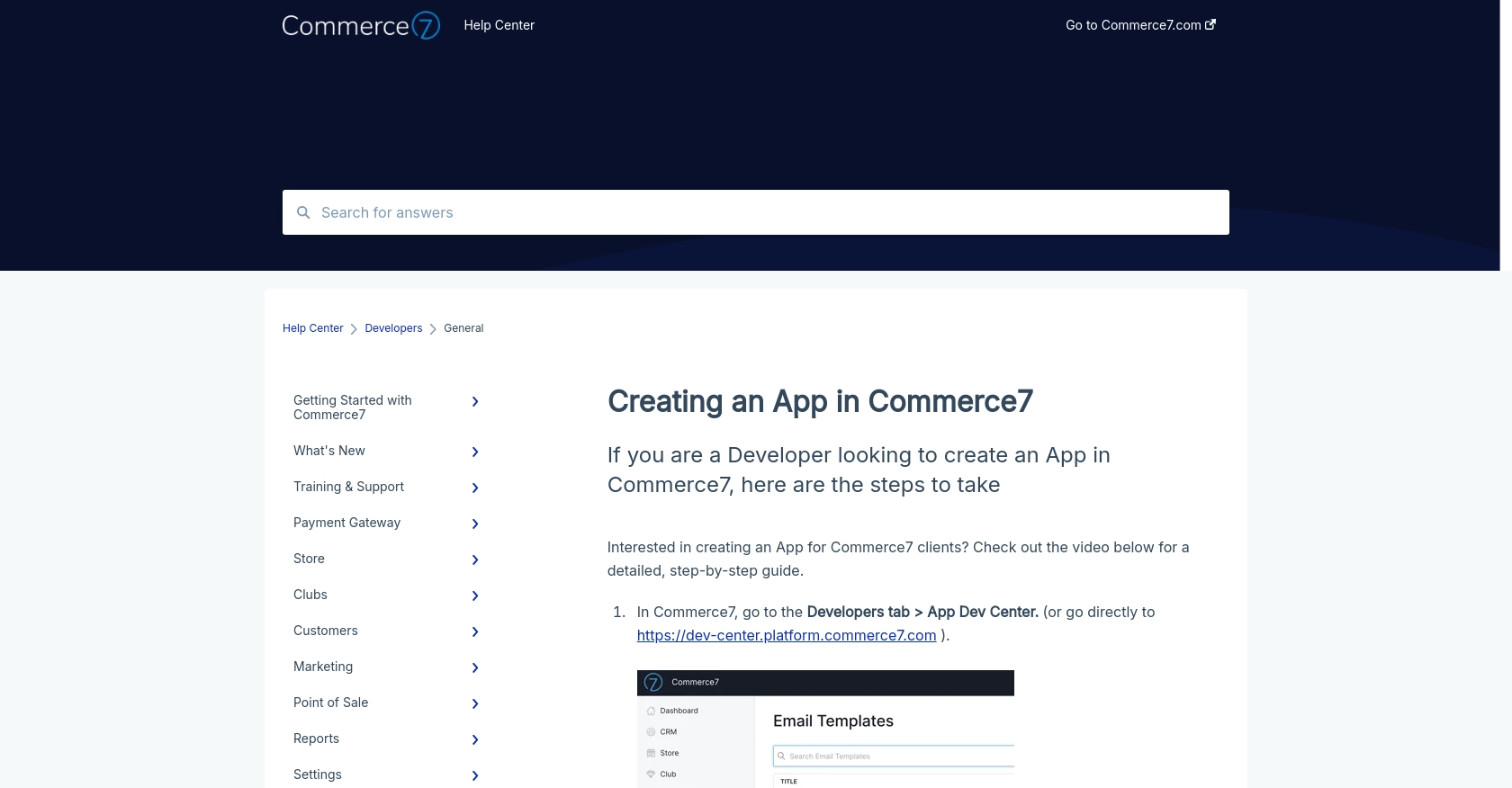
sbb-itb-96038d7
Making API Calls to Create or Update Customers with Commerce7 in JavaScript
To interact with the Commerce7 API for creating or updating customer records, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your JavaScript Environment for Commerce7 API Integration
Before making API calls, ensure you have Node.js installed on your machine. You will also need the axios
library to handle HTTP requests. Install it using npm:
npm install axios
Creating a New Customer with Commerce7 API
To create a new customer, you'll make a POST request to the Commerce7 API. Here's a sample code snippet:
const axios = require('axios');
const createCustomer = async () => {
const url = 'https://api.commerce7.com/v1/customer';
const auth = {
username: 'Your_App_ID',
password: 'Your_App_Secret_Key'
};
const customerData = {
firstName: 'Andrew',
lastName: 'Kamphuis',
birthDate: '1973-11-15',
city: 'Vancouver',
stateCode: 'BC',
zipCode: 'V6A1C2',
countryCode: 'CA',
emailMarketingStatus: 'Subscribed',
phones: [{ phone: '+16046135343' }],
emails: [{ email: 'andrewkamphuis@gmail.com' }]
};
try {
const response = await axios.post(url, customerData, { auth });
console.log('Customer Created:', response.data);
} catch (error) {
console.error('Error Creating Customer:', error.response.data);
}
};
createCustomer();
Replace Your_App_ID
and Your_App_Secret_Key
with your actual credentials. This code sends a POST request to create a customer and logs the response or any errors encountered.
Updating an Existing Customer with Commerce7 API
To update an existing customer, use a PUT request. Here's how you can do it:
const updateCustomer = async (customerId) => {
const url = `https://api.commerce7.com/v1/customer/${customerId}`;
const auth = {
username: 'Your_App_ID',
password: 'Your_App_Secret_Key'
};
const updateData = {
birthDate: '1973-11-15'
};
try {
const response = await axios.put(url, updateData, { auth });
console.log('Customer Updated:', response.data);
} catch (error) {
console.error('Error Updating Customer:', error.response.data);
}
};
updateCustomer('customer_id_here');
Replace customer_id_here
with the actual customer ID you wish to update. This code updates the customer's birth date and handles any errors that may occur.
Verifying API Requests and Handling Errors
After making API calls, verify the changes by checking the Commerce7 dashboard. If you encounter errors, refer to the error messages returned by the API. Common issues include incorrect credentials or missing required fields.
For more detailed error handling, you can inspect the error.response
object in the catch block to understand the specific issue.
For further details on API endpoints and error codes, refer to the Commerce7 Customers API Documentation.
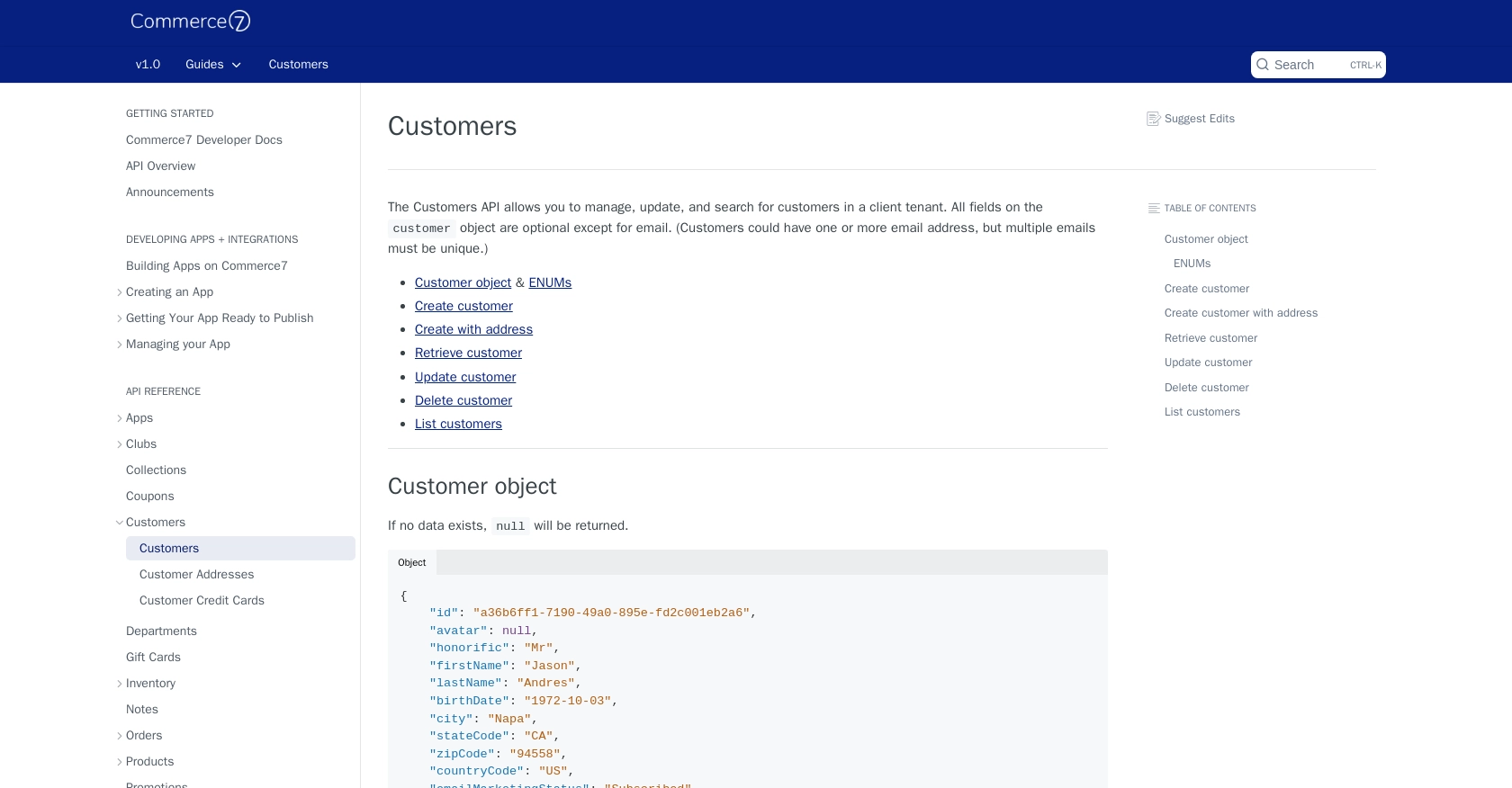
Best Practices for Using the Commerce7 API in JavaScript
When integrating with the Commerce7 API, it's crucial to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Secure Storage of Credentials: Always store your App ID and App Secret Key securely. Avoid hardcoding them in your frontend code to prevent unauthorized access.
- Handle Rate Limiting: Commerce7 API has a rate limit of 100 requests per minute per tenant. Implement logic to handle rate limits gracefully, such as retrying requests after a delay.
- Data Standardization: Ensure that data fields are standardized, especially when dealing with dates and currency. Commerce7 stores currency in cents and dates in UTC format.
Leveraging Endgrate for Seamless Commerce7 Integrations
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Commerce7.
With Endgrate, you can:
- Focus on your core product by outsourcing integration tasks.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?