Using the Younium API to Create or Update Sales Orders (with Javascript examples)
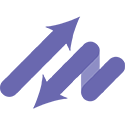
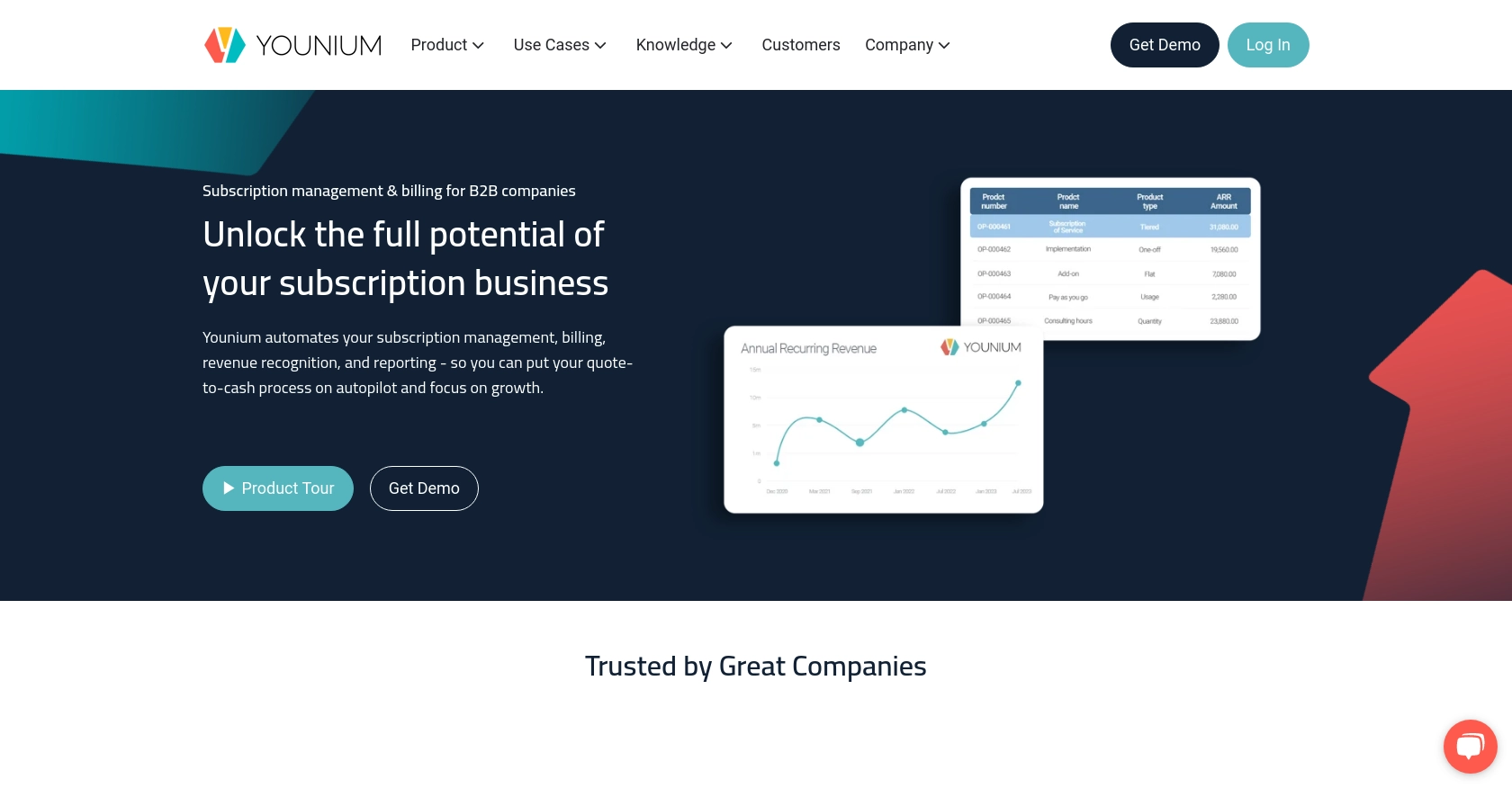
Introduction to Younium API for Sales Order Management
Younium is a comprehensive subscription management platform designed to streamline billing, invoicing, and financial operations for B2B SaaS companies. It offers robust tools to manage complex subscription models, automate billing processes, and gain insights into financial performance.
Integrating with Younium's API allows developers to efficiently manage sales orders, enabling seamless automation of order creation and updates. For example, a developer might use the Younium API to automatically generate sales orders from an external CRM system, ensuring that all sales data is synchronized and up-to-date.
Setting Up Your Younium Sandbox Account for API Integration
Before diving into the Younium API for managing sales orders, it's essential to set up a sandbox account. This environment allows developers to test and experiment with API calls without affecting live data, ensuring a smooth integration process.
Creating a Younium Sandbox Account
If you don't already have a Younium account, you can sign up for a sandbox account on the Younium website. This account will provide you with access to a testing environment where you can safely develop and test your API integrations.
- Visit the Younium Developer Portal.
- Follow the instructions to create a sandbox account.
- Once your account is set up, log in to access the sandbox environment.
Generating API Tokens and Client Credentials
To authenticate API requests, you'll need to generate an API token and client credentials. Follow these steps to obtain the necessary credentials:
- Log in to your Younium account and open the user profile menu by clicking your name in the top right corner.
- Select "Privacy & Security" from the dropdown menu.
- Navigate to "Personal Tokens" and click "Generate Token".
- Provide a relevant description for the token and click "Create".
- Copy the generated Client ID and Secret Key. These credentials will only be visible once, so ensure you store them securely.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token to authenticate your API requests:
// Example POST request to acquire JWT token
const axios = require('axios');
const getToken = async () => {
try {
const response = await axios.post('https://api.sandbox.younium.com/auth/token', {
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
}, {
headers: {
'Content-Type': 'application/json'
}
});
console.log('Access Token:', response.data.accessToken);
} catch (error) {
console.error('Error acquiring token:', error.response.data);
}
};
getToken();
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated. This script will return a JWT access token, valid for 24 hours, which you will use to authenticate API calls.
For more detailed information on authentication, refer to the Younium Authentication Guide.
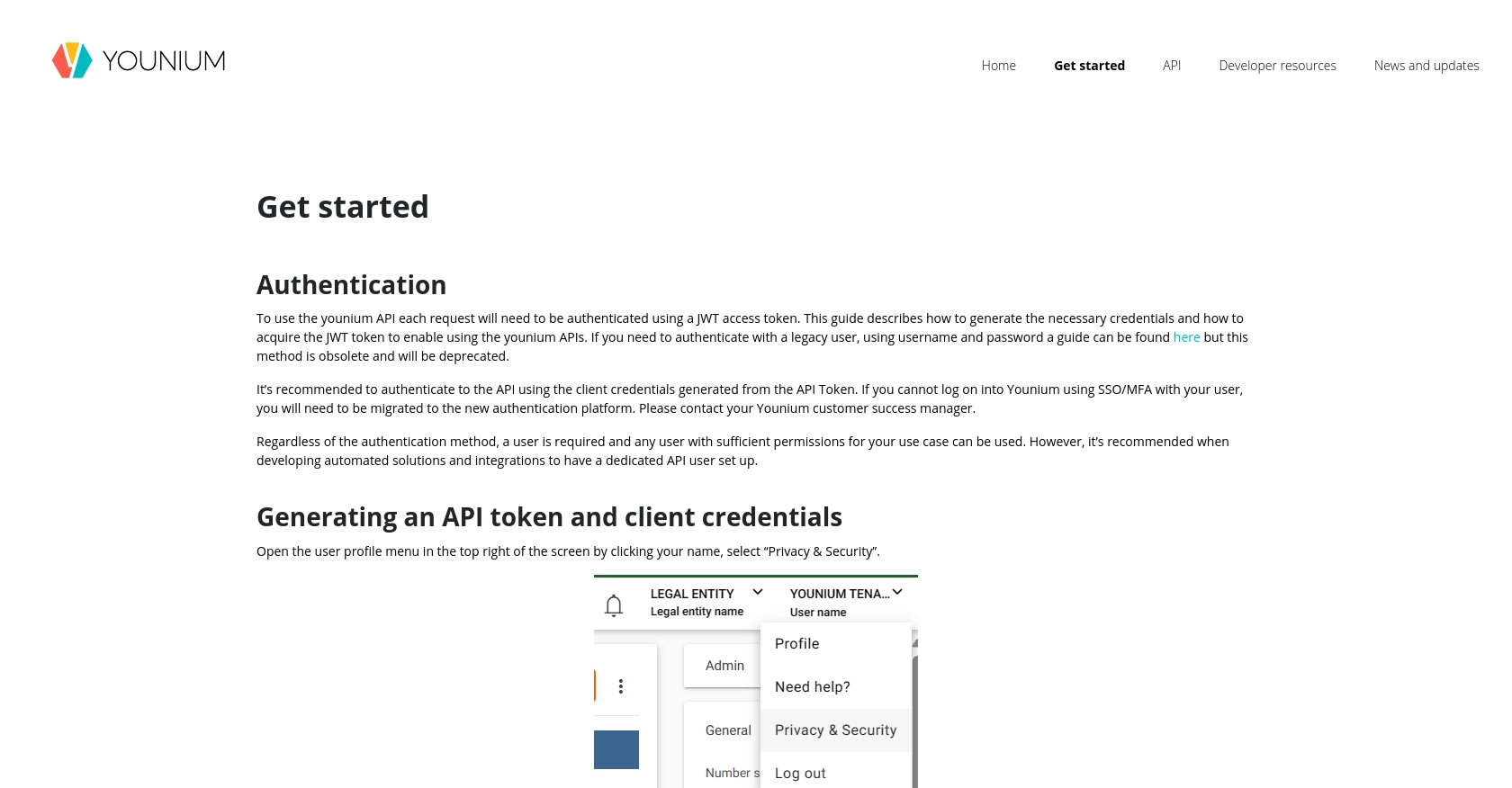
sbb-itb-96038d7
Making API Calls to Younium for Sales Order Management Using JavaScript
Once you have your JWT access token, you're ready to interact with the Younium API to create or update sales orders. This section will guide you through the process of making authenticated API calls using JavaScript, ensuring seamless integration with Younium's platform.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. You'll also need the axios
library to handle HTTP requests. You can install it using npm:
npm install axios
Creating a Sales Order with Younium API
To create a sales order, you'll need to send a POST request to the Younium API's sales order endpoint. Here's an example of how to do this using JavaScript:
// Example POST request to create a sales order
const axios = require('axios');
const createSalesOrder = async () => {
try {
const response = await axios.post('https://api.sandbox.younium.com/salesorders', {
// Replace with your sales order data
orderNumber: 'SO12345',
customer: 'Customer_ID',
items: [
{
productId: 'Product_ID',
quantity: 10,
price: 100
}
]
}, {
headers: {
'Authorization': 'Bearer Your_JWT_Token',
'Content-Type': 'application/json'
}
});
console.log('Sales Order Created:', response.data);
} catch (error) {
console.error('Error creating sales order:', error.response.data);
}
};
createSalesOrder();
Replace Your_JWT_Token
with your access token and fill in the sales order data as needed. This script will create a new sales order in the Younium sandbox environment.
Updating a Sales Order with Younium API
To update an existing sales order, send a PUT request to the Younium API with the updated data. Here's how you can do it:
// Example PUT request to update a sales order
const updateSalesOrder = async (orderId) => {
try {
const response = await axios.put(`https://api.sandbox.younium.com/salesorders/${orderId}`, {
// Replace with updated sales order data
items: [
{
productId: 'Updated_Product_ID',
quantity: 5,
price: 90
}
]
}, {
headers: {
'Authorization': 'Bearer Your_JWT_Token',
'Content-Type': 'application/json'
}
});
console.log('Sales Order Updated:', response.data);
} catch (error) {
console.error('Error updating sales order:', error.response.data);
}
};
updateSalesOrder('Order_ID');
Replace Order_ID
with the ID of the sales order you wish to update. This script will update the specified sales order in the Younium sandbox environment.
Verifying API Call Success and Handling Errors
After making an API call, check the response to verify success. A successful creation or update will return a 201 status code along with the sales order details. If an error occurs, the response will include an error message and a status code such as 400 for bad requests or 401 for unauthorized access.
For more information on handling errors, refer to the Younium API Documentation.
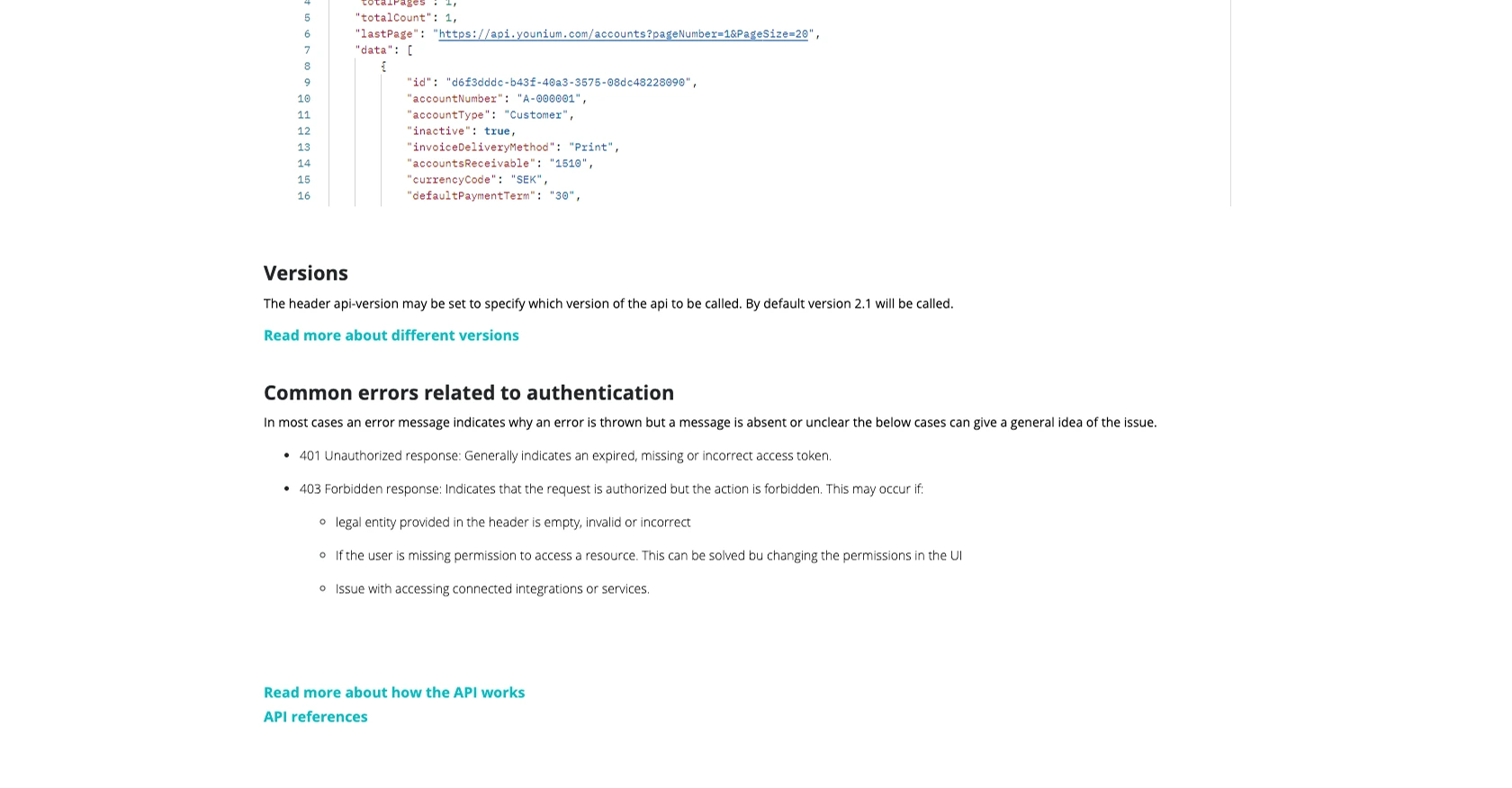
Conclusion: Best Practices for Using Younium API in Sales Order Management
Integrating with the Younium API for sales order management can significantly enhance your business operations by automating and streamlining order processes. To ensure a successful integration, consider the following best practices:
Securely Storing User Credentials
Always store your API credentials, such as the Client ID and Secret Key, securely. Use environment variables or secure vaults to prevent unauthorized access. Never hard-code these credentials in your source code.
Handling Younium API Rate Limits
Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully. For specific rate limit details, refer to the Younium API Documentation.
Transforming and Standardizing Data Fields
Ensure that the data you send and receive from the Younium API is correctly formatted and standardized. This will help maintain data integrity and consistency across your systems.
Utilizing Endgrate for Simplified Integrations
Consider using Endgrate to streamline your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Younium, offering an intuitive integration experience for your customers.
By following these best practices, you can maximize the efficiency and reliability of your Younium API integrations, ensuring seamless sales order management for your business.
Read More
Ready to get started?