Using the Commerce7 API to Get Customers (with PHP examples)
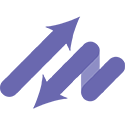
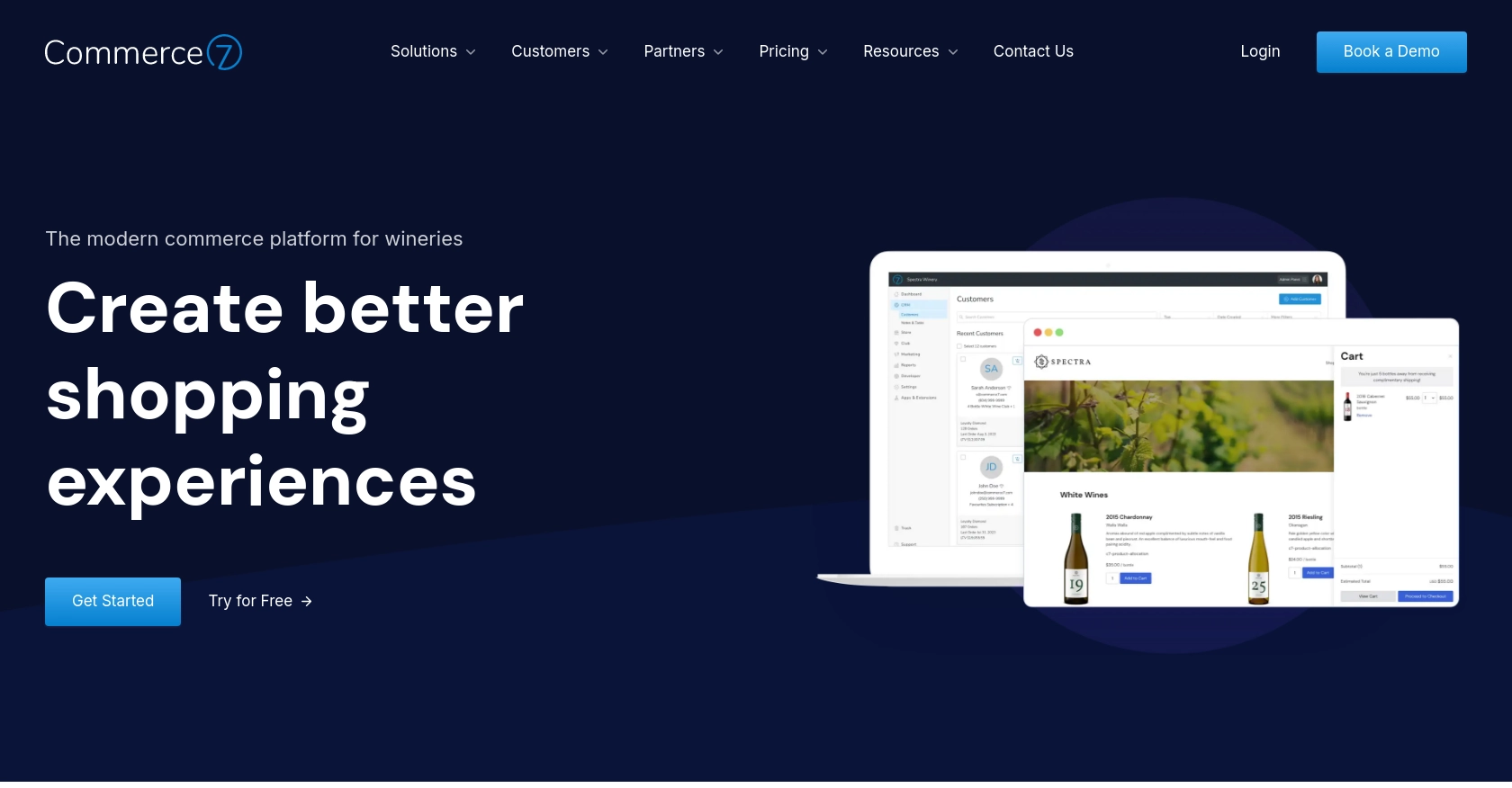
Introduction to Commerce7 API Integration
Commerce7 is a powerful eCommerce platform tailored for the wine industry, offering a comprehensive suite of tools to enhance customer experiences and streamline operations. With features like club memberships, reservations, and personalized marketing, Commerce7 empowers wineries to manage their business efficiently.
Developers might want to integrate with Commerce7's API to access and manage customer data, enabling seamless interactions and personalized experiences. For example, retrieving customer information using the Commerce7 API in PHP can help automate marketing campaigns or enhance customer relationship management by syncing data with other systems.
Setting Up a Commerce7 Test Account for API Integration
Before you begin interacting with the Commerce7 API, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Commerce7 provides a robust environment for developers to test their integrations.
Creating a Commerce7 Developer Account
To start, you'll need to create a developer account on Commerce7. Follow these steps:
- Visit the Commerce7 Developer Center.
- Click on the "Sign Up" button to create a new account.
- Fill in the required details, including your email and password, and submit the form.
- Verify your email address by clicking on the link sent to your inbox.
Creating a Commerce7 App for API Access
Once your developer account is set up, you need to create an app to access the API:
- Log into the Commerce7 Developer Center.
- Navigate to the "App Dev Center" and click on "Add App".
- Provide a name and description for your app.
- Select the API endpoints you wish to access, such as customer data.
- Save your app to generate an App ID and App Secret Key.
Keep your App Secret Key secure, as it is crucial for authenticating API requests.
Understanding Commerce7 API Authentication
Commerce7 uses Basic Auth for API requests, requiring your App ID and App Secret Key:
- Use your App ID as the username.
- Use your App Secret Key as the password.
Ensure these credentials are stored securely and not exposed in client-side code.
Generating API Credentials
To authenticate API requests, follow these steps:
- Log into your Commerce7 Developer account.
- Access the app you created and locate the App Secret Key.
- Use these credentials to authenticate your API requests.
For more detailed instructions, refer to the Commerce7 App Creation Guide and the Commerce7 API Documentation.
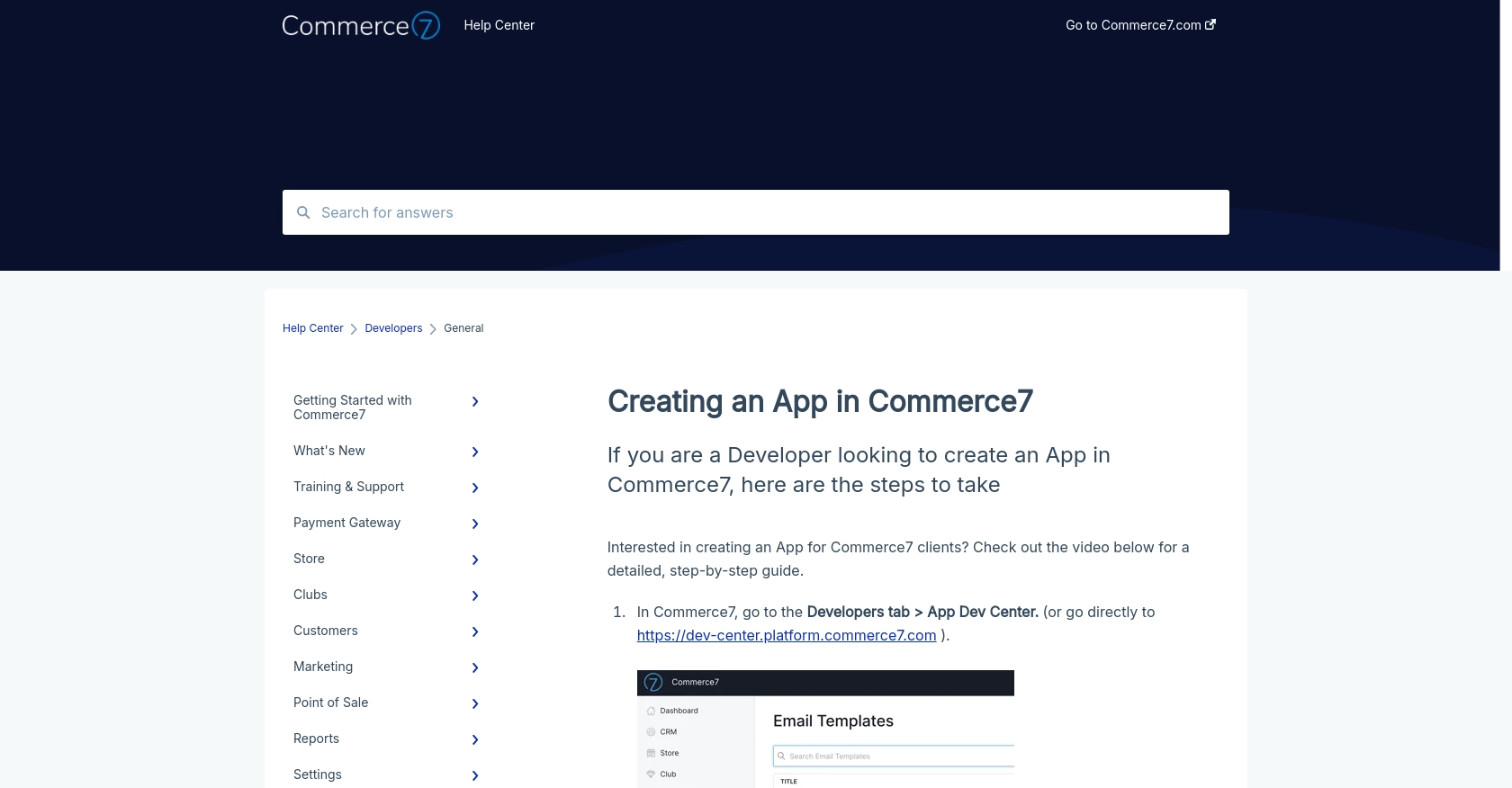
sbb-itb-96038d7
Making API Calls to Retrieve Customers Using Commerce7 API with PHP
To interact with the Commerce7 API and retrieve customer data, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses effectively.
Setting Up Your PHP Environment for Commerce7 API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP version 7.4 or higher.
- Ensure the
cURL
extension is enabled in yourphp.ini
file. - Install Composer, a dependency manager for PHP, to manage any additional libraries.
Installing Required PHP Libraries for Commerce7 API
To simplify HTTP requests, you can use the Guzzle library. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Customers from Commerce7 API
Now, let's write the PHP code to make a GET request to the Commerce7 API and retrieve customer data:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('GET', 'https://api.commerce7.com/v1/customer', [
'auth' => ['Your_App_ID', 'Your_App_Secret_Key'],
'headers' => [
'Content-Type' => 'application/json',
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data['customers'] as $customer) {
echo 'Customer Name: ' . $customer['firstName'] . ' ' . $customer['lastName'] . "\n";
echo 'Email: ' . $customer['emails'][0]['email'] . "\n\n";
}
Replace Your_App_ID
and Your_App_Secret_Key
with your actual Commerce7 app credentials.
Understanding the API Response and Verifying Success
After running the script, you should see a list of customer names and emails printed to the console. This confirms that the API call was successful. You can verify the data by checking the Commerce7 sandbox environment to ensure it matches the retrieved information.
Handling Errors and API Rate Limits in Commerce7
It's crucial to handle potential errors and respect API rate limits:
- Check the response status code. A 200 status indicates success, while other codes may indicate errors.
- Commerce7 API has a rate limit of 100 requests per minute per tenant. Implement logic to handle rate limiting, such as retrying requests after a delay.
For more details on error codes and rate limits, refer to the Commerce7 API Documentation.
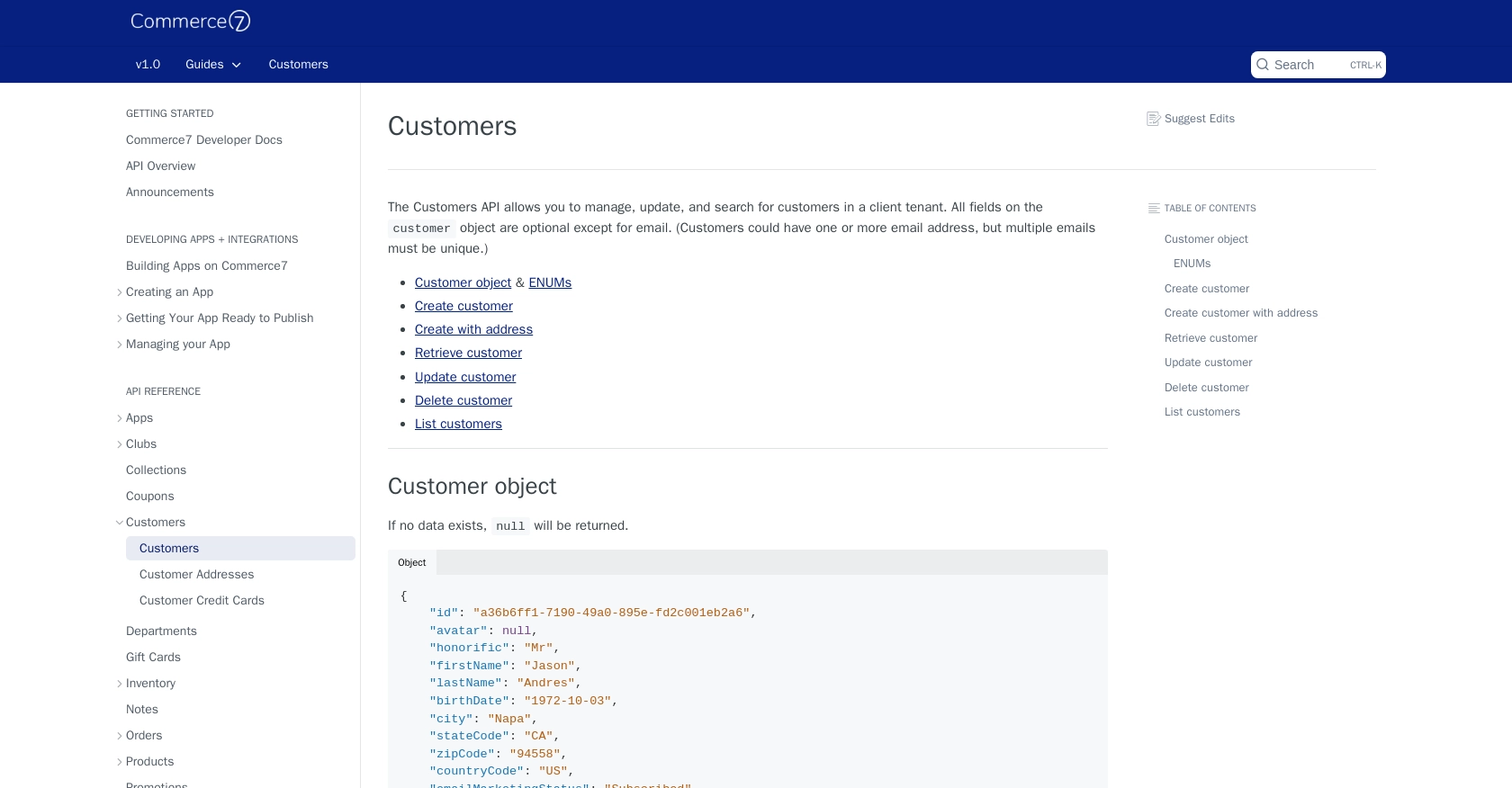
Best Practices for Commerce7 API Integration and Data Management
When integrating with the Commerce7 API, it's essential to follow best practices to ensure secure and efficient data management. Here are some recommendations:
- Secure Storage of Credentials: Always store your App ID and App Secret Key securely. Avoid exposing them in client-side code to prevent unauthorized access.
- Efficient Error Handling: Implement robust error handling to manage API response codes effectively. This includes retry logic for transient errors and logging for debugging purposes.
- Respecting API Rate Limits: Commerce7 enforces a rate limit of 100 requests per minute per tenant. Implement logic to handle rate limiting, such as exponential backoff, to avoid hitting these limits.
- Data Transformation and Standardization: Ensure that data retrieved from the API is transformed and standardized to match your application's requirements, especially when dealing with different time zones and currency formats.
Enhancing Integration Efficiency with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Commerce7. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while outsourcing integrations to Endgrate.
- Streamline Integration Processes: Build once for each use case instead of multiple times for different integrations.
- Improve Customer Experience: Offer an easy and intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?