How to Get Items with the Sap Business One API in Python
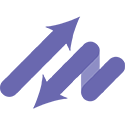
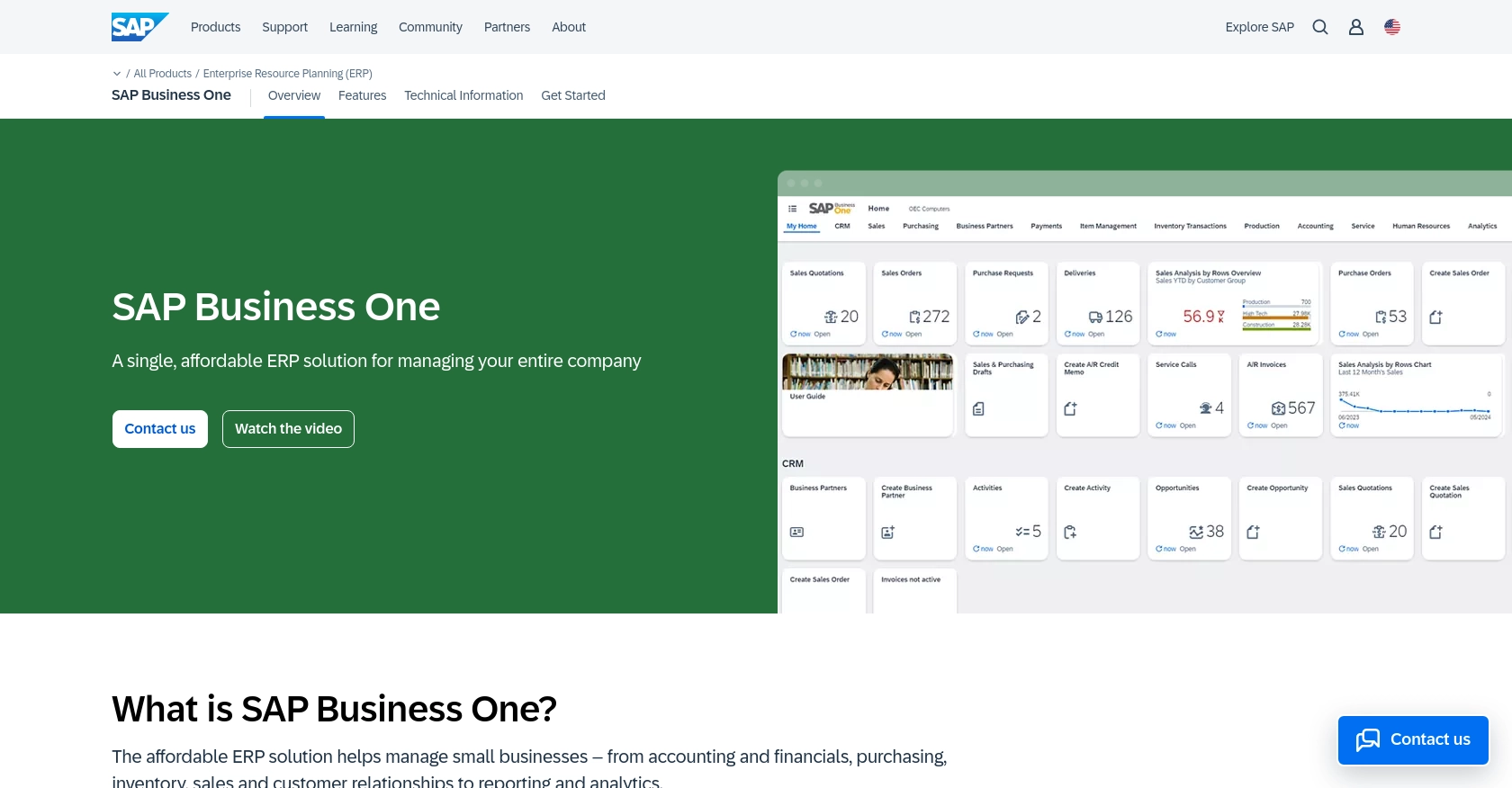
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control. By providing a unified platform, SAP Business One helps businesses streamline their operations and improve efficiency.
Integrating with the SAP Business One API allows developers to access and manage various business data programmatically. For example, a developer might want to retrieve item information from the SAP Business One database to synchronize inventory data with an e-commerce platform. This integration can enhance data accuracy and ensure that inventory levels are consistently updated across systems.
Setting Up a Test or Sandbox Account for SAP Business One API
Before you can start interacting with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your production data. Here's how you can get started:
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a test environment from your system administrator. If not, you may need to contact SAP or a certified SAP partner to set up a trial or demo account.
Configuring API Access in SAP Business One
Once you have access to the sandbox environment, follow these steps to configure API access:
- Log in to the SAP Business One Service Layer using your sandbox credentials.
- Navigate to the Administration module and select Setup > General > Service Layer Configuration.
- Ensure that the Service Layer is enabled and note down the Service Layer URL, which you will use in your API requests.
Generating API Credentials for SAP Business One
SAP Business One uses a custom authentication method. Follow these steps to generate the necessary credentials:
- In the SAP Business One interface, go to Administration > Setup > Users.
- Select the user account you want to use for API access or create a new user specifically for this purpose.
- Assign the necessary permissions to the user to ensure they have access to the data you wish to retrieve.
- Note down the username and password for this user, as these will be used for authentication in your API calls.
With your sandbox account set up and API credentials in hand, you're ready to start making API calls to the SAP Business One Service Layer. In the next section, we'll cover how to perform these calls using Python.
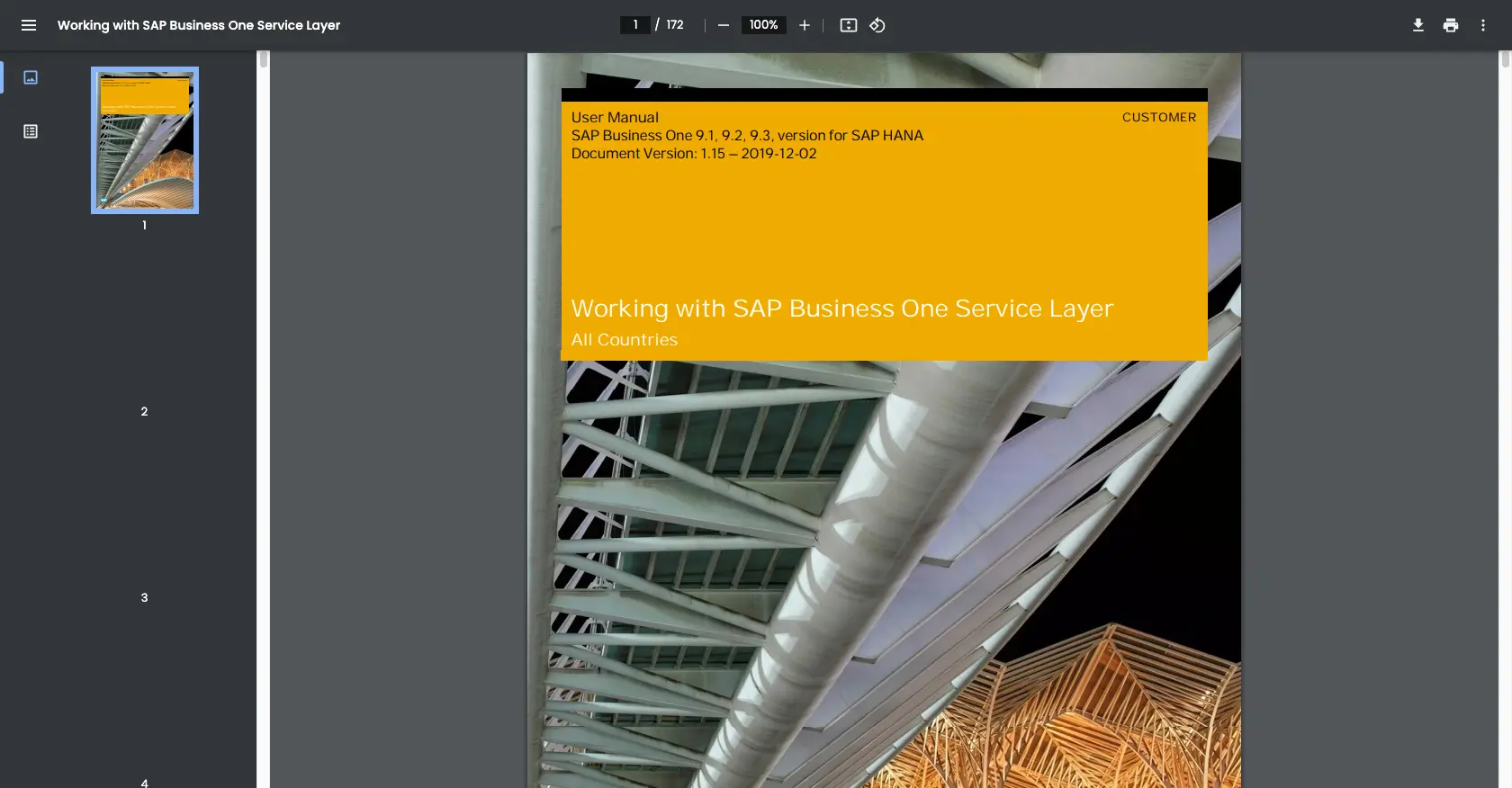
sbb-itb-96038d7
Making API Calls to Retrieve Items from SAP Business One Using Python
To interact with the SAP Business One API and retrieve item data, you'll need to use Python. This section will guide you through the process, including setting up your Python environment, writing the code to make API calls, and handling potential errors.
Setting Up Your Python Environment for SAP Business One API
Before you begin, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer
pip
Once these are installed, open your terminal or command line and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Retrieve Items from SAP Business One
Create a new Python file named get_sap_items.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://your-sap-service-layer-url/b1s/v1/Items"
headers = {
"Content-Type": "application/json",
"Authorization": "Basic Your_Base64_Encoded_Credentials"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
items = response.json()
for item in items['value']:
print(f"Item Code: {item['ItemCode']}, Item Name: {item['ItemName']}")
else:
print(f"Failed to retrieve items. Status Code: {response.status_code}, Error: {response.text}")
Replace Your_Base64_Encoded_Credentials
with your base64-encoded username and password for SAP Business One.
Running the Python Script and Verifying the Output
Run the script from your terminal or command line using the following command:
python get_sap_items.py
If successful, you should see a list of items with their codes and names printed in the console. If there are any errors, the script will output the status code and error message.
Handling Errors and Troubleshooting API Calls
When making API calls, you may encounter various errors. Here are some common error codes and their meanings:
- 401 Unauthorized: Check your credentials and ensure they are correctly encoded.
- 404 Not Found: Verify the endpoint URL and ensure it is correct.
- 500 Internal Server Error: This may indicate an issue with the SAP Business One server. Check the server logs for more details.
For more detailed error handling, refer to the SAP Business One API documentation: SAP Business One Service Layer Documentation.
Conclusion and Best Practices for Using SAP Business One API in Python
Integrating with the SAP Business One API using Python can significantly enhance your business operations by providing seamless access to critical data. By following the steps outlined in this guide, you can efficiently retrieve item information and synchronize it with other platforms, ensuring data consistency and accuracy.
Best Practices for Secure and Efficient API Integration with SAP Business One
- Securely Store Credentials: Always store your API credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the SAP Business One API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields retrieved from SAP Business One are standardized and transformed as needed to match the requirements of your target systems.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or optimizations.
Streamline Your Integration Process with Endgrate
While integrating with SAP Business One API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?