Using the SendGrid API to Get Contacts in PHP
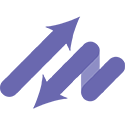
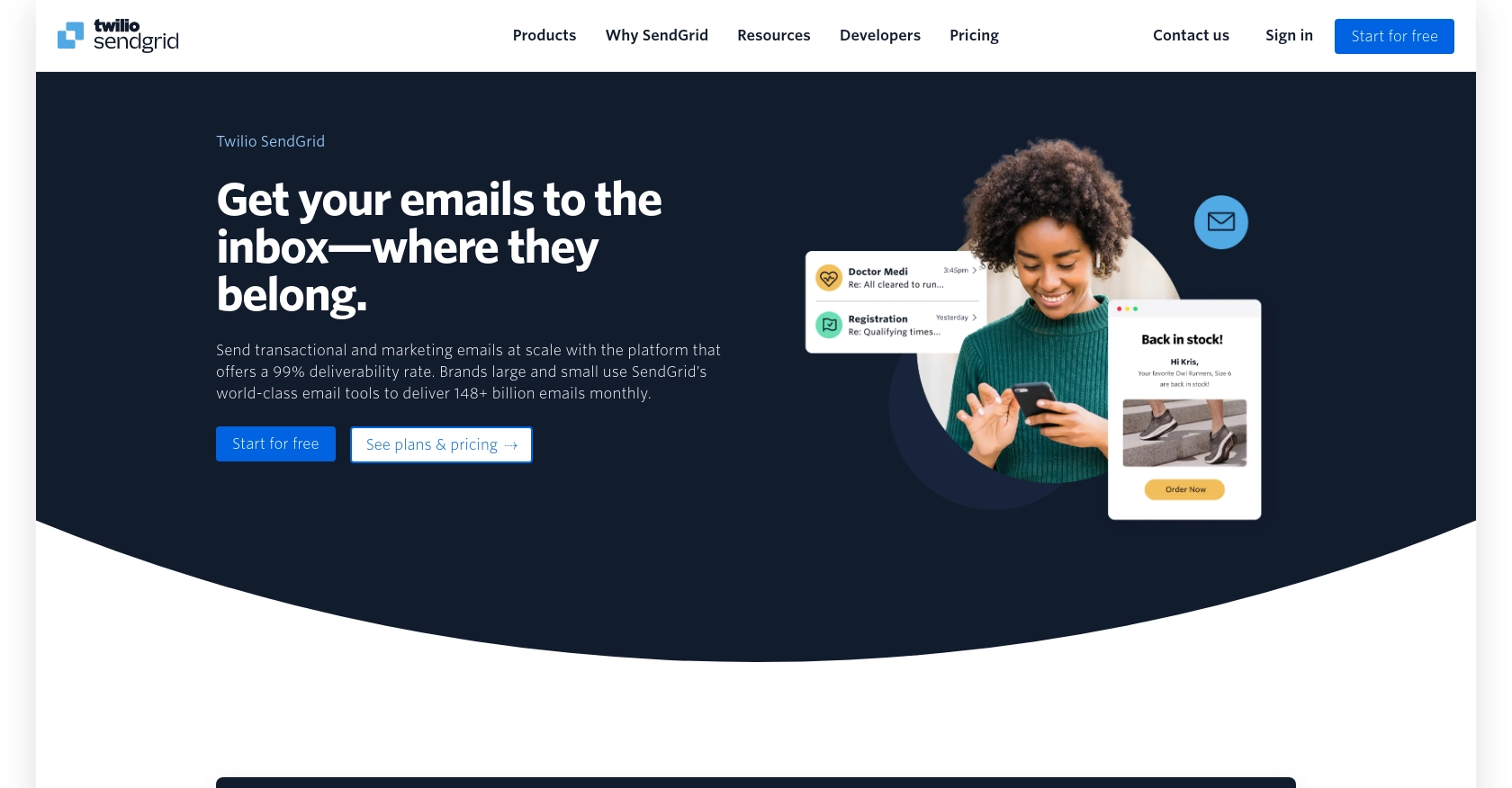
Introduction to SendGrid API for Contact Management
SendGrid, a cloud-based email delivery service, is renowned for its robust API that enables businesses to manage email communications effectively. It offers a suite of tools for sending transactional and marketing emails, ensuring high deliverability rates and comprehensive analytics.
For developers, integrating with SendGrid's API can streamline the process of managing contact lists, automating email campaigns, and personalizing communications. For example, you might want to retrieve contact details from SendGrid to synchronize with your CRM system, ensuring that your marketing efforts are always up-to-date and targeted.
Setting Up Your SendGrid Account for API Access
Before you can start using the SendGrid API to manage contacts, you'll need to set up your SendGrid account and obtain the necessary API key for authentication. This section will guide you through the process of setting up a test or sandbox account and generating an API key.
Create a SendGrid Account
If you don't already have a SendGrid account, you can sign up for a free account on the SendGrid website. The free plan offers a generous number of emails per month, which is ideal for testing and development purposes.
- Visit the SendGrid website and click on "Sign Up".
- Fill in the required information, such as your name, email, and password.
- Verify your email address to activate your account.
Generate an API Key for SendGrid
Once your account is set up, you'll need to generate an API key to authenticate your API requests. Follow these steps to create an API key:
- Log in to your SendGrid account.
- Navigate to the "Settings" section in the left-hand menu.
- Select "API Keys" from the dropdown menu.
- Click on "Create API Key".
- Provide a name for your API key, such as "Contact Management Key".
- Select the appropriate permissions for your API key. For managing contacts, ensure you have the necessary scopes enabled.
- Click "Create & View" to generate your API key.
- Copy the API key and store it securely, as it will not be displayed again.
For more detailed information on API key generation, refer to the SendGrid API Authentication Documentation.
Test Your API Key
To ensure your API key is working correctly, you can perform a simple test by making a request to the SendGrid API. Use the following PHP code snippet to verify your API key:
$apiKey = 'YOUR_API_KEY_HERE';
$endpoint = 'https://api.sendgrid.com/v3/marketing/contacts';
$headers = [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Replace YOUR_API_KEY_HERE
with the API key you generated. If the request is successful, you should receive a response from the SendGrid API.
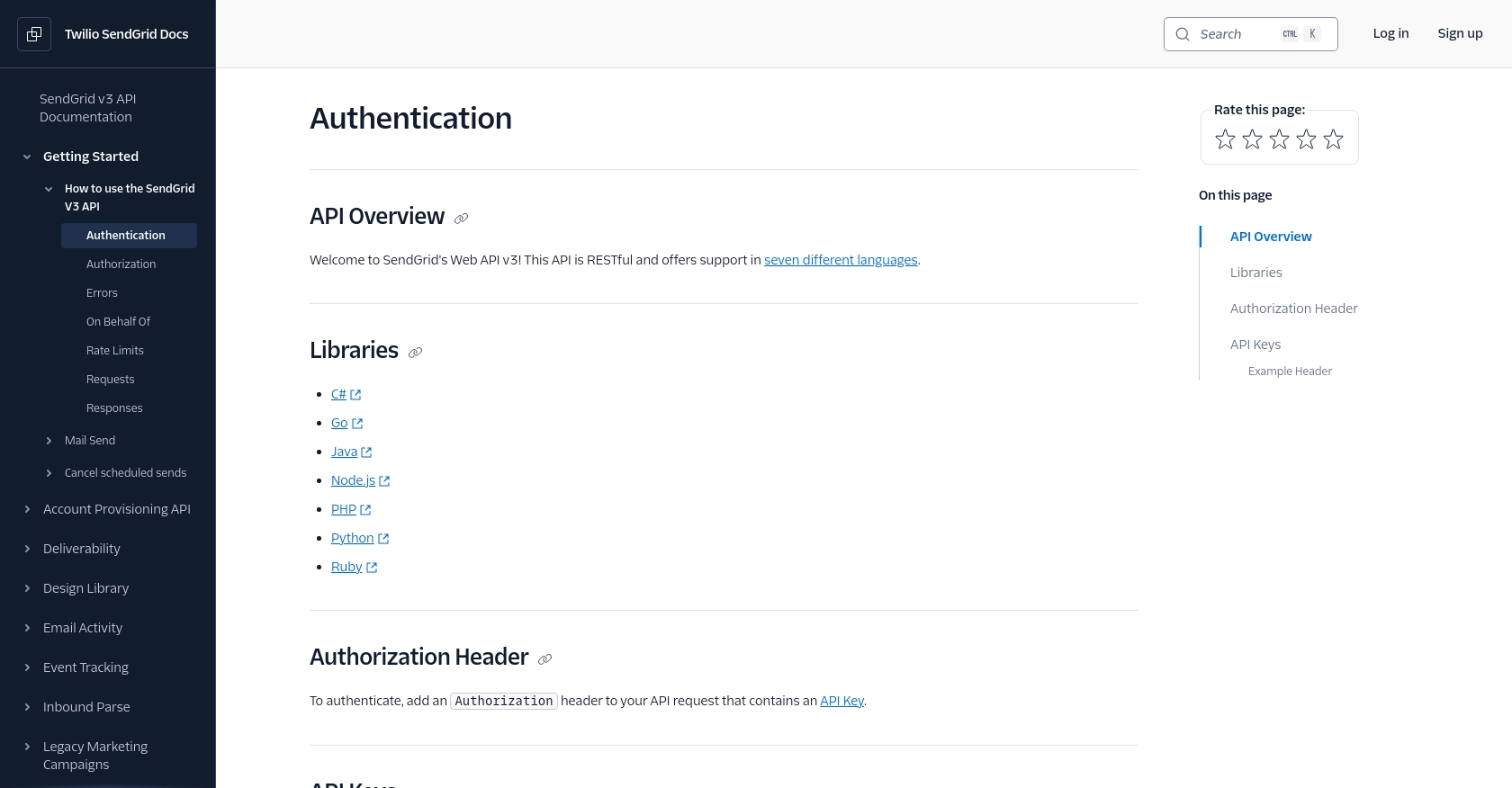
sbb-itb-96038d7
Making API Calls to Retrieve Contacts Using SendGrid in PHP
To effectively interact with the SendGrid API and retrieve contact information, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses from the API.
Setting Up Your PHP Environment for SendGrid API Integration
Before making API calls, ensure your PHP environment is properly configured. You will need:
- PHP 7.4 or higher
- cURL extension enabled
Verify your PHP version and cURL installation by running the following commands in your terminal:
php -v
php -m | grep curl
Installing Necessary PHP Dependencies for SendGrid API
To interact with the SendGrid API, you need to ensure that the cURL extension is enabled in your PHP configuration. This extension allows you to make HTTP requests from your PHP scripts.
Writing PHP Code to Retrieve Contacts from SendGrid
Now, let's write the PHP code to make a POST request to the SendGrid API to retrieve contacts by email. Create a file named get_sendgrid_contacts.php
and add the following code:
$apiKey = 'YOUR_API_KEY_HERE';
$endpoint = 'https://api.sendgrid.com/v3/marketing/contacts/search/emails';
$emails = ['jane_doe@example.com', 'john_doe@example.com'];
$data = json_encode(['emails' => $emails]);
$headers = [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Replace YOUR_API_KEY_HERE
with your actual API key. This script sends a POST request to the SendGrid API to retrieve contacts by their email addresses.
Understanding the SendGrid API Response
Upon executing the script, you should receive a JSON response containing the contact details for the specified email addresses. Here's an example of what the response might look like:
{
"result": {
"jane_doe@example.com": {
"contact": {
"email": "jane_doe@example.com",
"first_name": "Jane",
"last_name": "Doe",
"created_at": "2021-03-02T15:25:47Z",
"updated_at": "2021-03-30T15:26:16Z"
}
},
"john_doe@example.com": {
"contact": {
"email": "john_doe@example.com",
"first_name": "John",
"last_name": "Doe",
"created_at": "2020-01-02T15:25:47Z",
"updated_at": "2020-12-20T15:26:16Z"
}
}
}
}
Handling Errors and Rate Limits in SendGrid API
It's crucial to handle potential errors and respect the API's rate limits. If you exceed the rate limit, the API will return a status code 429. To handle errors, you can modify the PHP script to check the HTTP status code:
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($httpCode === 200) {
echo "Contacts retrieved successfully.";
} elseif ($httpCode === 429) {
echo "Rate limit exceeded. Please try again later.";
} else {
echo "An error occurred: " . $response;
}
For more information on rate limits, refer to the SendGrid Rate Limits Documentation.
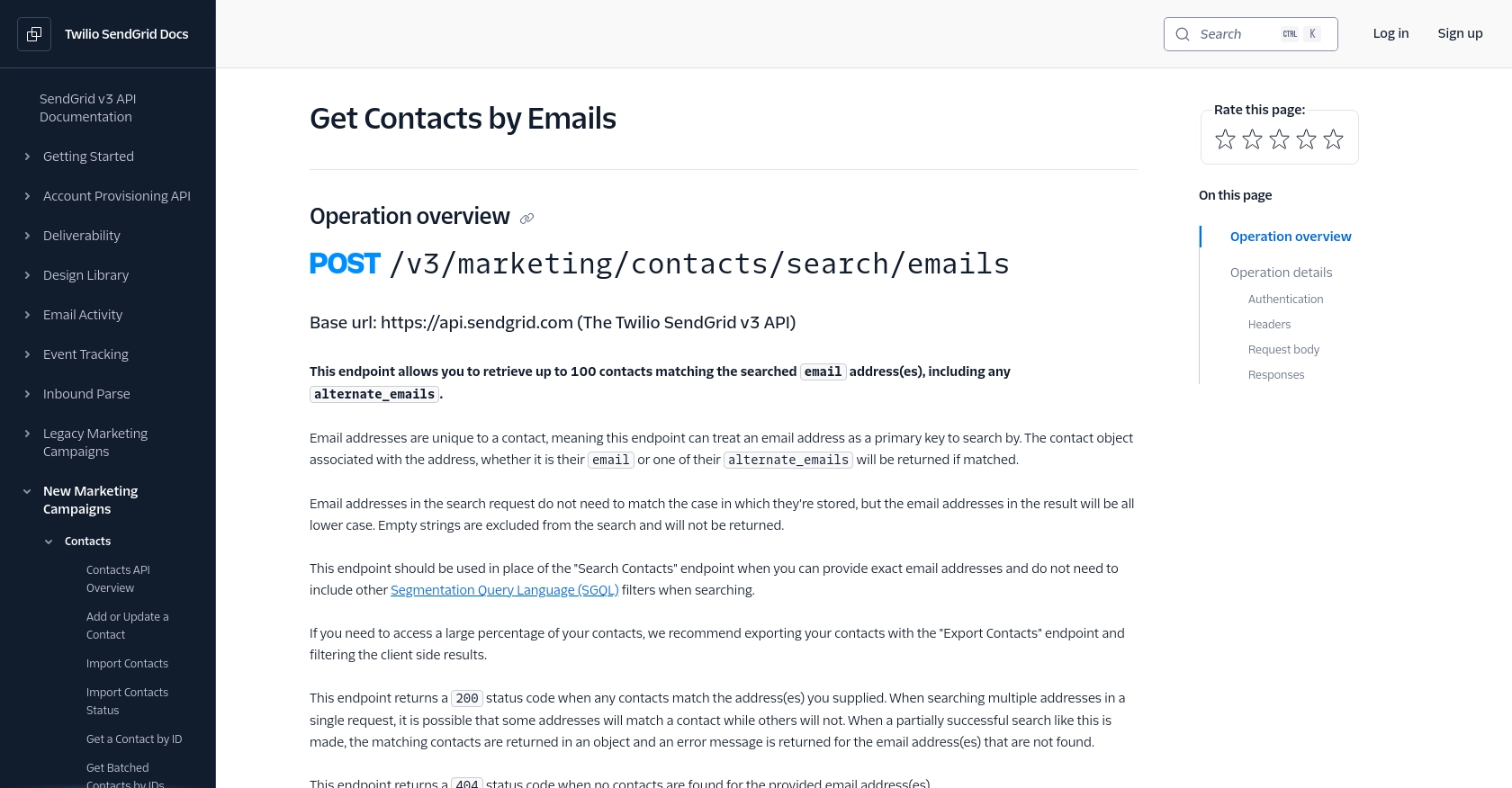
Conclusion and Best Practices for Using SendGrid API in PHP
Integrating the SendGrid API into your PHP applications can significantly enhance your ability to manage contacts and automate email communications. By following the steps outlined in this guide, you can efficiently retrieve contact information and ensure your marketing efforts are well-targeted and up-to-date.
Best Practices for Secure and Efficient SendGrid API Integration
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of SendGrid's rate limits to avoid disruptions. Implement logic to handle status code 429 and retry requests after a delay. For more details, refer to the SendGrid Rate Limits Documentation.
- Data Transformation: Consider normalizing and transforming data fields to maintain consistency across your systems. This can help in seamless data integration and reporting.
- Error Handling: Implement robust error handling to manage different HTTP status codes and potential API errors. This ensures your application can gracefully handle unexpected issues.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with SendGrid's API can be straightforward, managing multiple integrations across various platforms can become complex and time-consuming. This is where Endgrate can be a valuable asset.
Endgrate offers a unified API endpoint that simplifies the integration process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and avoid the hassle of maintaining multiple integrations. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
By adopting these best practices and leveraging tools like Endgrate, you can ensure a smooth and efficient integration experience, providing your customers with a seamless and intuitive interaction with your services.
Read More
- https://endgrate.com/provider/sendgrid
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/authentication
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/authorization
- https://www.twilio.com/docs/sendgrid/api-reference/how-to-use-the-sendgrid-v3-api/rate-limits
- https://www.twilio.com/docs/sendgrid/api-reference/contacts/get-contacts-by-emails
Ready to get started?