Using the Sap Business One API to Create or Update Customers in Javascript
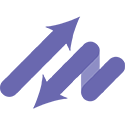
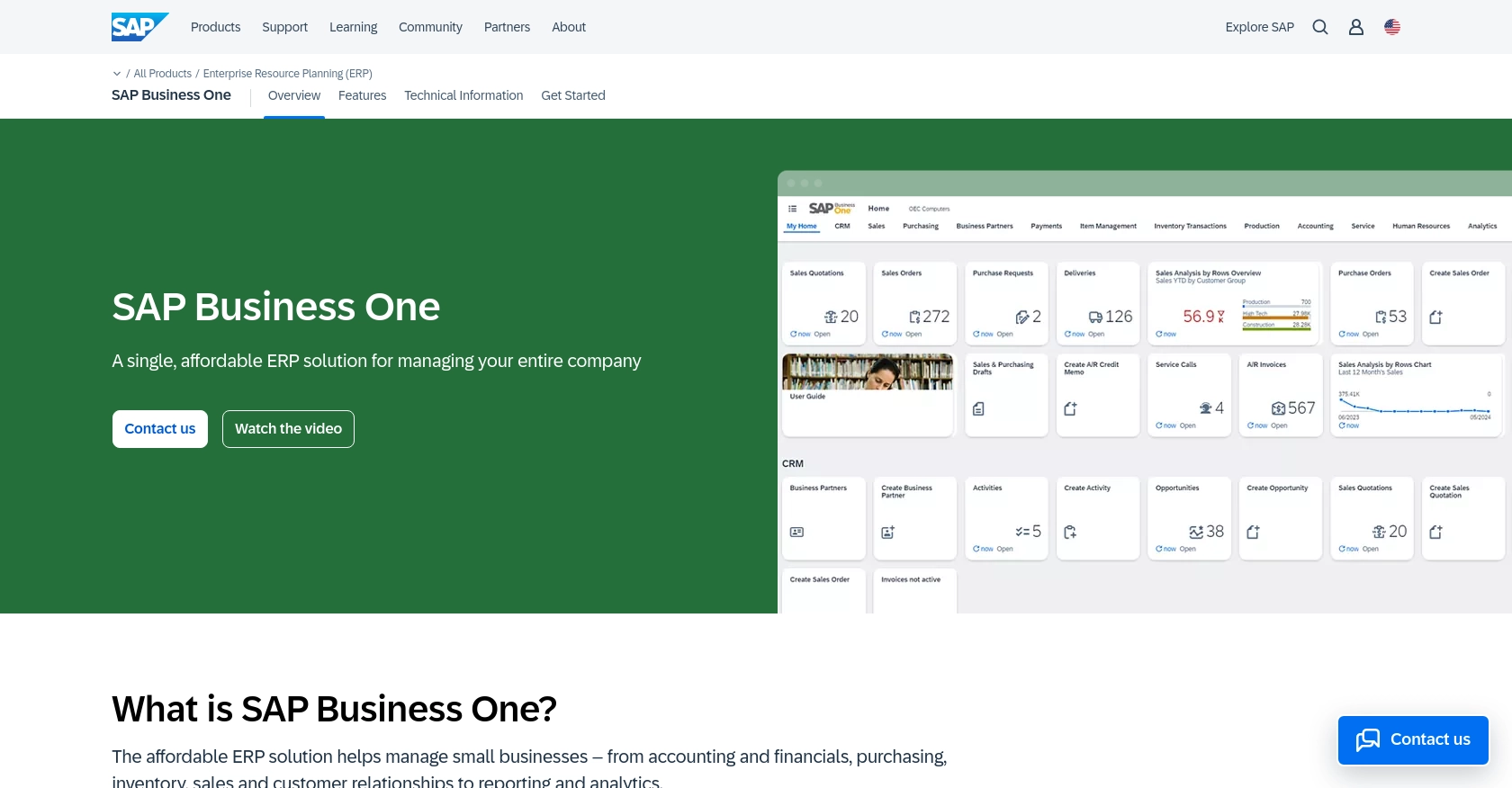
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small and medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all designed to streamline business operations and enhance productivity.
Integrating with the SAP Business One API allows developers to automate and manage customer data efficiently. For example, you can use the API to create or update customer records directly from your application, ensuring that your customer information is always up-to-date and consistent across platforms.
Setting Up a Test or Sandbox Account for SAP Business One API Integration
Before you can start integrating with the SAP Business One API, it's essential to set up a test or sandbox environment. This allows you to safely experiment with API calls without affecting your live data. SAP Business One provides a sandbox environment specifically for developers to test their integrations.
Creating a SAP Business One Sandbox Account
To begin, you'll need to create a sandbox account with SAP Business One. Follow these steps to set up your account:
- Visit the SAP Business One Service Layer Documentation for detailed instructions on accessing the sandbox environment.
- Sign up for a developer account if you haven't already. This will grant you access to the sandbox environment.
- Once your account is created, log in to the SAP Business One sandbox portal to begin configuring your environment.
Configuring OAuth-Based Authentication for SAP Business One API
SAP Business One uses a custom authentication method to secure API access. Follow these steps to configure authentication:
- Navigate to the API management section within your sandbox account.
- Create a new application to obtain your client ID and client secret. These credentials are necessary for authenticating API requests.
- Set the necessary permissions for your application to access customer data. Ensure you have both read and write permissions enabled.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need to generate API keys for certain operations. Here's how to do it:
- Go to the API keys section in your sandbox account dashboard.
- Click on "Generate New API Key" and follow the prompts to create a key.
- Store your API key securely, as you'll need it to authenticate your API calls.
With your sandbox environment and authentication credentials set up, you're ready to start making API calls to create or update customer records in SAP Business One using JavaScript.
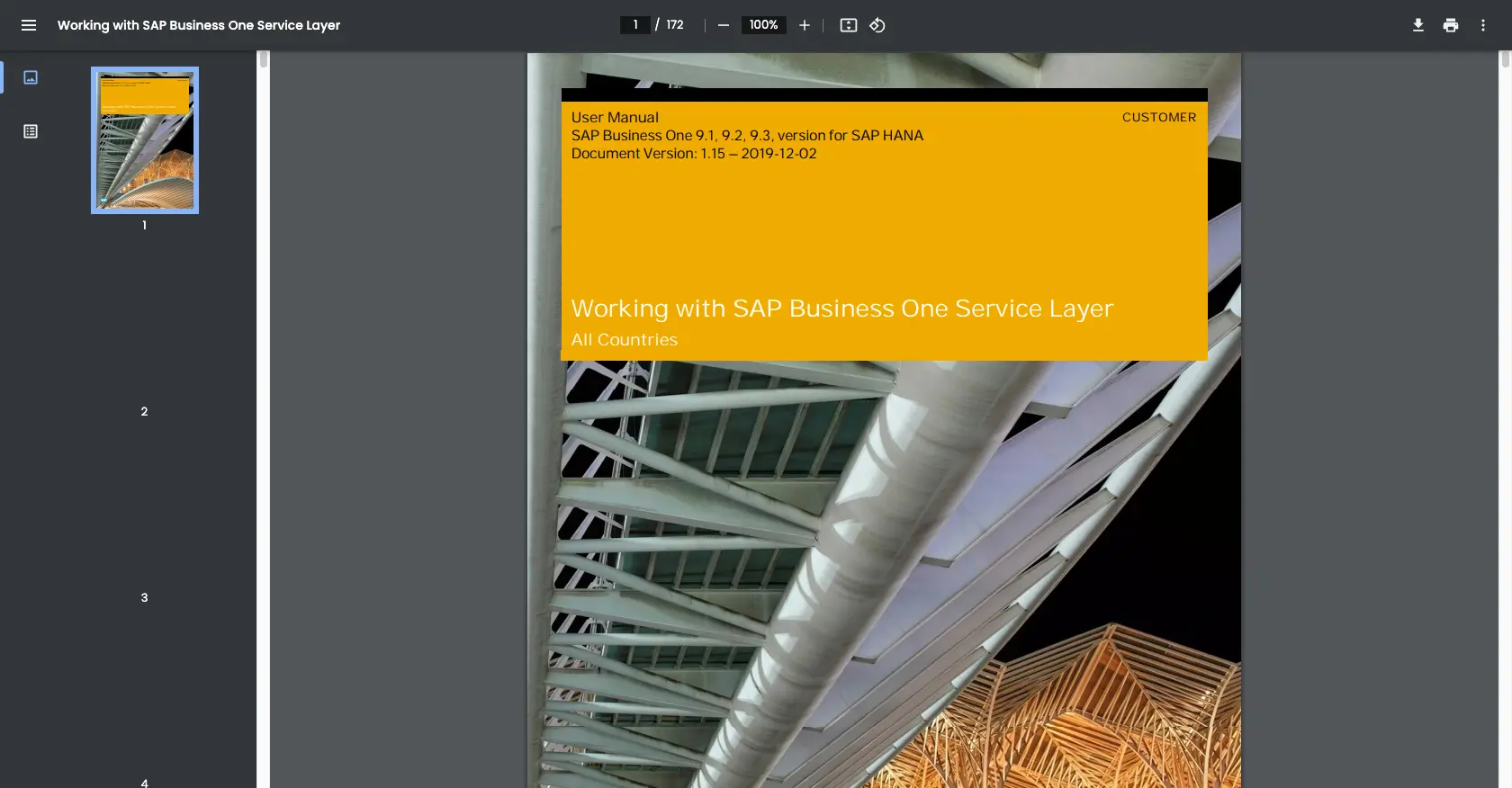
sbb-itb-96038d7
Making API Calls to Create or Update Customers in SAP Business One Using JavaScript
To interact with the SAP Business One API and manage customer records, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment
Before you begin coding, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor, such as Visual Studio Code, to write and manage your JavaScript files.
- The
axios
library for making HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Create or Update Customers
Now, let's write the JavaScript code to interact with the SAP Business One API. This example demonstrates how to create or update a customer record.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/BusinessPartners';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Token'
};
// Define the customer data
const customerData = {
CardCode: 'C12345',
CardName: 'New Customer',
CardType: 'C', // 'C' for Customer
EmailAddress: 'customer@example.com'
};
// Function to create or update a customer
async function createOrUpdateCustomer() {
try {
const response = await axios.post(endpoint, customerData, { headers });
console.log('Customer created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating customer:', error.response ? error.response.data : error.message);
}
}
// Execute the function
createOrUpdateCustomer();
Replace Your_Token
with the token obtained from your SAP Business One sandbox account.
Verifying API Call Success and Handling Errors
After executing the code, verify the success of your API call by checking the response data. If the customer is created or updated successfully, you should see the relevant details in the response.
To handle errors, ensure you catch exceptions and log the error messages. This will help you diagnose issues related to authentication, network connectivity, or data validation.
Testing in the SAP Business One Sandbox Environment
Once your API call is successful, log in to your SAP Business One sandbox environment to verify that the customer record has been created or updated. This ensures that your integration is functioning as expected.
Conclusion and Best Practices for SAP Business One API Integration
Integrating with the SAP Business One API using JavaScript allows developers to efficiently manage customer data, ensuring consistency and accuracy across platforms. By following the steps outlined in this guide, you can create or update customer records seamlessly within your applications.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your API keys and tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Validation: Ensure that the data you send to the API is validated and sanitized to prevent errors and maintain data integrity.
- Error Handling: Implement comprehensive error handling to capture and log errors, making it easier to troubleshoot issues related to API calls.
Streamlining Integrations with Endgrate
While integrating with SAP Business One can enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including SAP Business One.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate, you build once for each use case, reducing the need for multiple integrations and offering an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?