Using the Google Forms API to Get Questions in Python
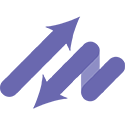
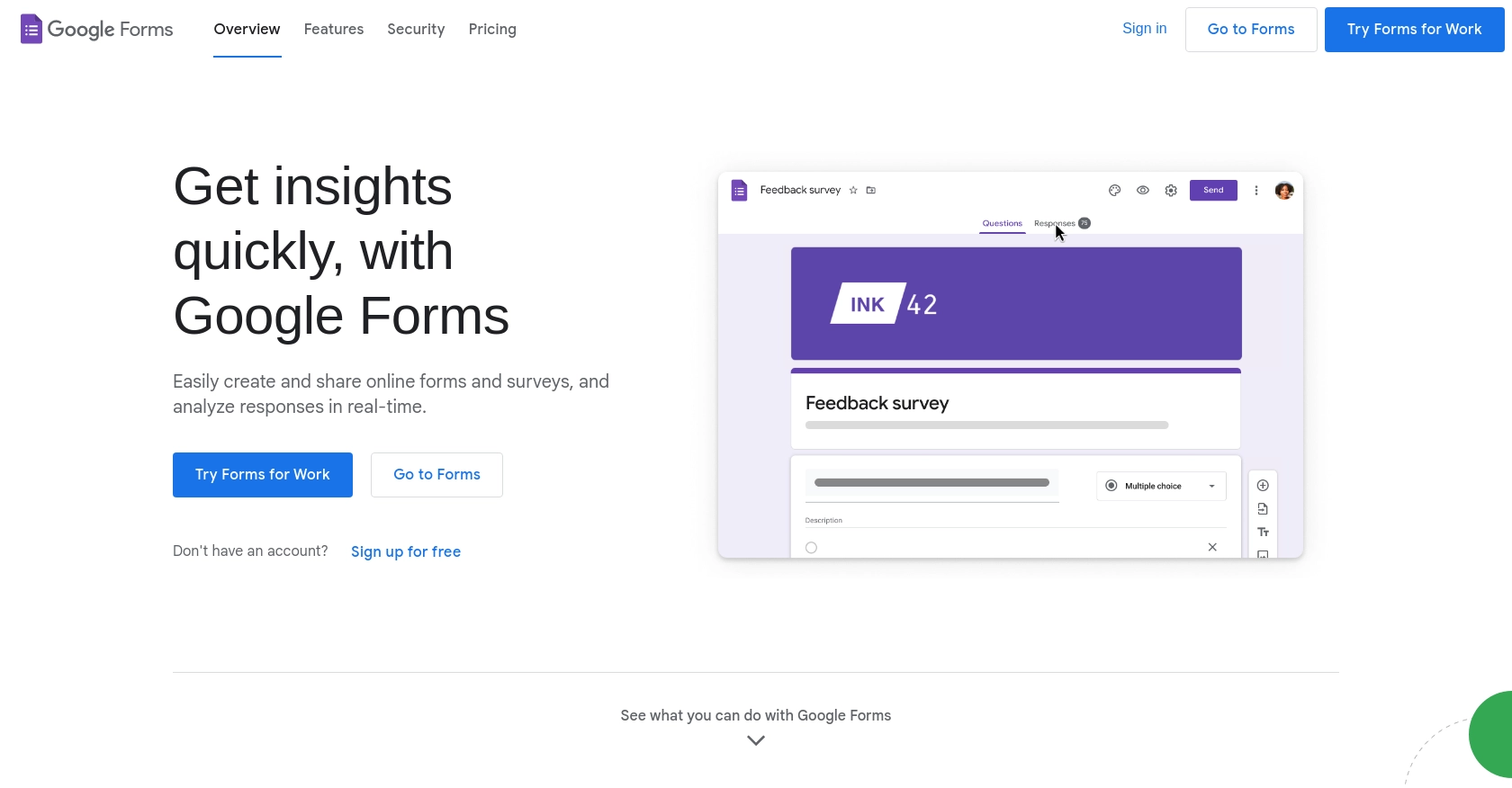
Introduction to Google Forms API
Google Forms is a versatile tool within the Google Workspace suite, allowing users to create surveys, quizzes, and forms with ease. Its integration capabilities make it a powerful asset for businesses and developers looking to streamline data collection and analysis.
By integrating with the Google Forms API, developers can automate the retrieval and management of form data, such as extracting questions for analysis or reporting. For example, a developer might use the API to fetch questions from a feedback form and analyze the responses to improve customer service strategies.
Setting Up Your Google Forms API Test Environment
Before you can start interacting with the Google Forms API, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows you to securely access and manage your Google Forms data programmatically.
Step 1: Create a Google Cloud Project
To begin, you need a Google Cloud project. This project will serve as the foundation for enabling APIs and managing credentials.
- Go to the Google Cloud Console.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Step 2: Enable Google Forms API
Next, you need to enable the Google Forms API for your project.
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Forms API" and click on it.
- Click Enable to activate the API for your project.
Step 3: Configure OAuth Consent Screen
Configuring the OAuth consent screen is crucial for defining how your application requests access to user data.
- In the Google Cloud Console, navigate to APIs & Services > OAuth consent screen.
- Select the user type and fill out the required fields, such as application name and support email.
- Click Save and Continue.
Step 4: Create OAuth 2.0 Credentials
To authenticate your application, you'll need to create OAuth 2.0 credentials.
- Go to APIs & Services > Credentials in the Google Cloud Console.
- Click Create Credentials > OAuth client ID.
- Select the application type (e.g., Web application) and configure the authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as they will be used to authenticate API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Access Credentials.
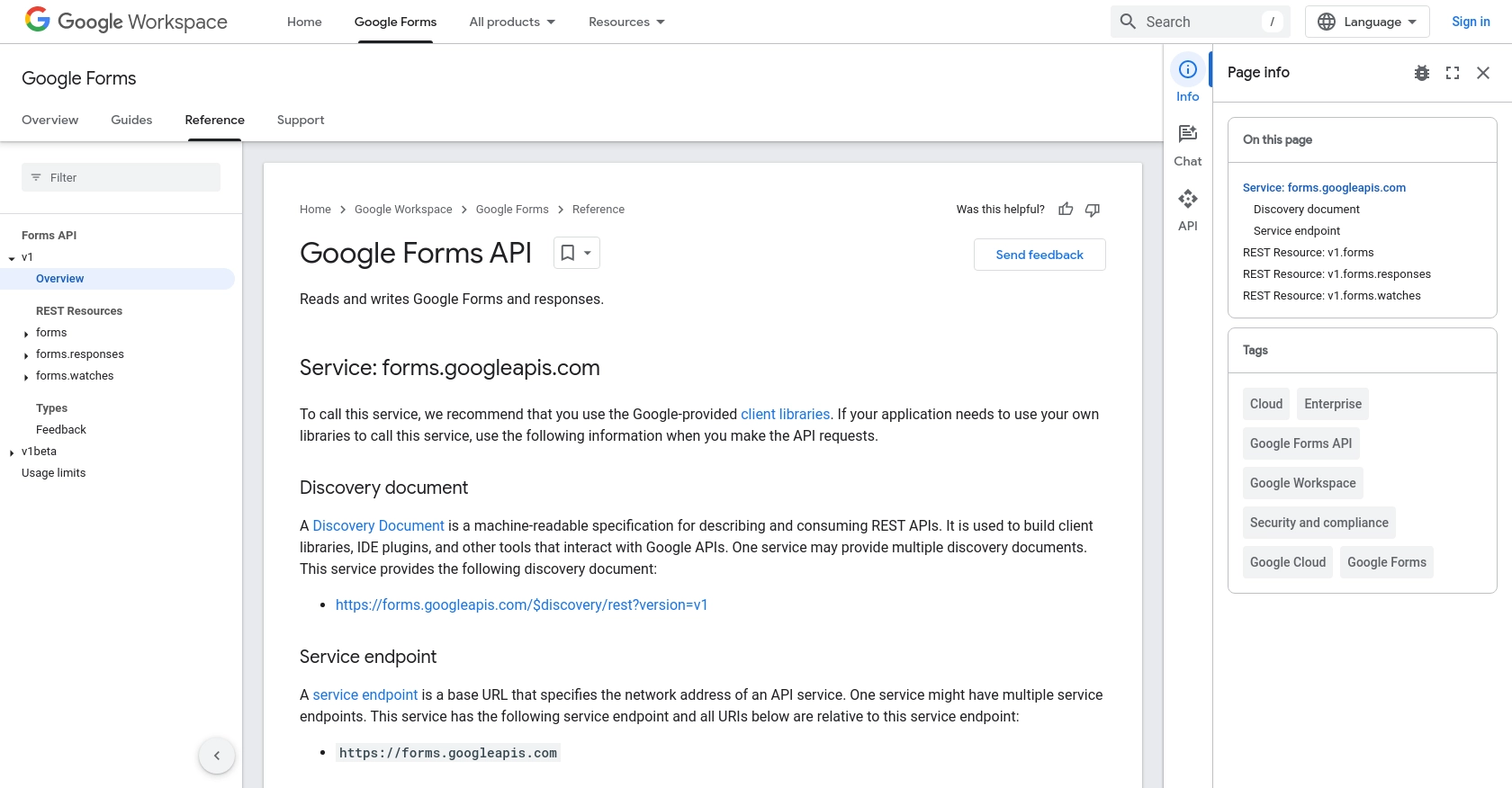
sbb-itb-96038d7
Making API Calls to Retrieve Google Forms Questions Using Python
To interact with the Google Forms API and retrieve questions, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of making API calls to fetch questions from a Google Form.
Prerequisites for Google Forms API Integration with Python
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Additionally, you'll need to install the google-auth
and google-auth-oauthlib
libraries to handle authentication:
pip install google-auth google-auth-oauthlib
Setting Up the Python Script to Fetch Google Forms Questions
Create a file named get_google_forms_questions.py
and add the following code:
from google.oauth2 import service_account
from googleapiclient.discovery import build
# Define the scope
SCOPES = ['https://www.googleapis.com/auth/forms.body.readonly']
# Load credentials from the JSON key file
creds = service_account.Credentials.from_service_account_file(
'path/to/credentials.json', scopes=SCOPES)
# Build the service
service = build('forms', 'v1', credentials=creds)
# Specify the form ID
form_id = 'your_form_id_here'
# Make the API call to get the form
form = service.forms().get(formId=form_id).execute()
# Print the form questions
for item in form['items']:
if 'questionItem' in item:
question = item['questionItem']['question']
print(f"Question ID: {question['questionId']}, Text: {question['textQuestion']}")
Replace 'path/to/credentials.json'
with the path to your service account JSON key file and 'your_form_id_here'
with the ID of the Google Form you wish to access.
Running the Python Script and Verifying Output
Execute the script using the following command:
python get_google_forms_questions.py
Upon successful execution, the script will output the questions from the specified Google Form. Verify the output by checking the questions listed in your Google Form.
Handling Errors and Google Forms API Error Codes
While making API calls, you might encounter errors. It's crucial to handle these gracefully. Common error codes include:
- 400 Bad Request: The request was invalid. Check your form ID and credentials.
- 401 Unauthorized: Authentication failed. Ensure your credentials are correct.
- 403 Forbidden: Access is denied. Verify your OAuth scopes and permissions.
For more detailed error handling, refer to the Google Forms API documentation.
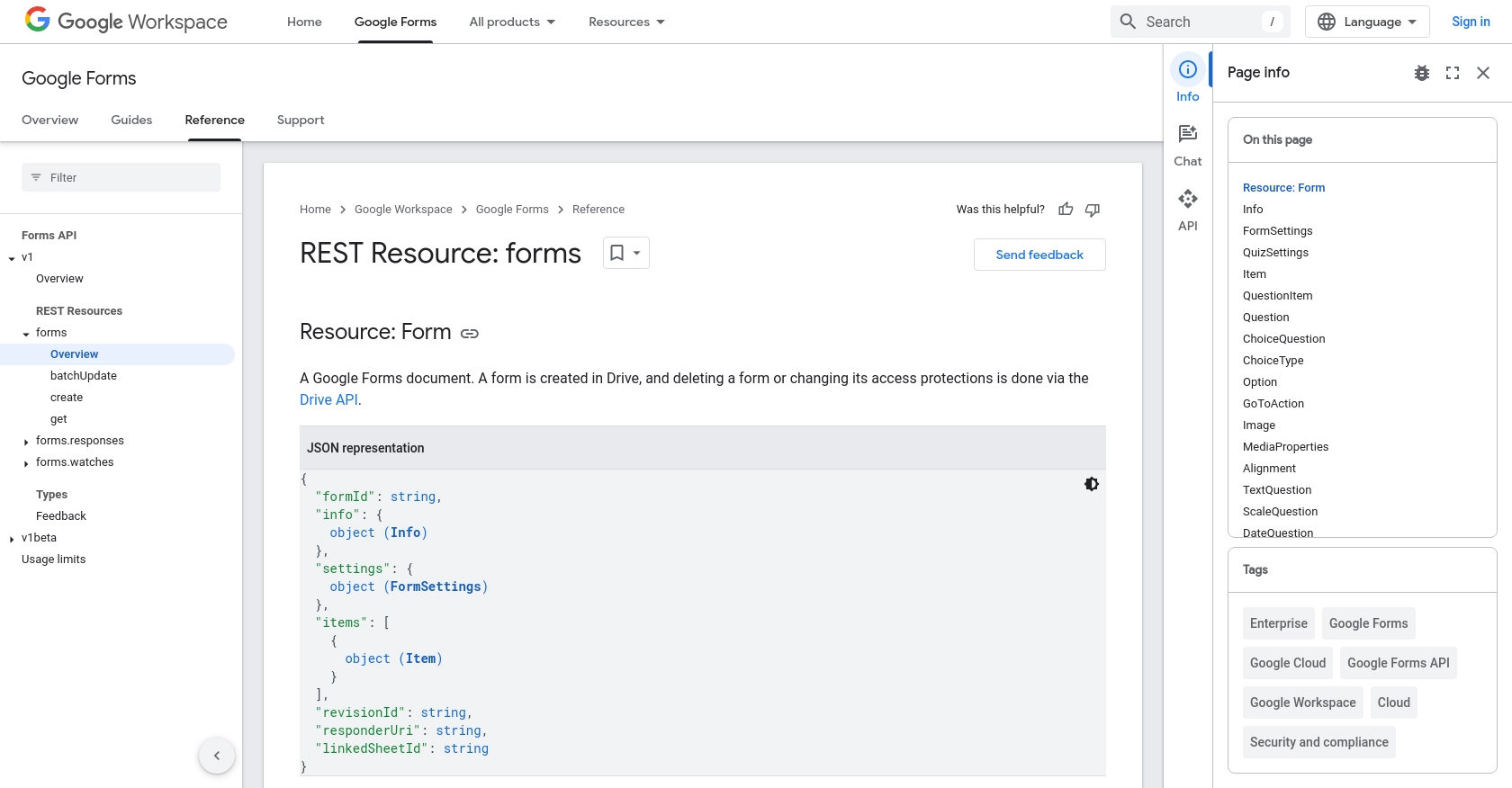
Conclusion and Best Practices for Using Google Forms API with Python
Integrating with the Google Forms API using Python allows developers to efficiently manage and analyze form data, enhancing data-driven decision-making processes. By automating the retrieval of form questions, you can streamline workflows and improve productivity.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials and service account keys securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Google Forms API has usage limits. To avoid hitting these limits, implement exponential backoff strategies for retrying requests. For more details, refer to the Google Forms API documentation.
- Data Standardization: Ensure that the data retrieved from Google Forms is standardized and transformed as needed for your application. This can help maintain consistency across different data sources.
Streamlining Integrations with Endgrate
While integrating with Google Forms API can be straightforward, managing multiple integrations across different platforms can become complex. This is where Endgrate can be a valuable asset.
Endgrate provides a unified API endpoint that simplifies the integration process, allowing you to focus on your core product. By using Endgrate, you can build once for each use case and avoid the hassle of maintaining multiple integrations. This not only saves time and resources but also enhances the integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
- https://endgrate.com/provider/googleforms
- https://developers.google.com/forms/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/forms/api/reference/rest/v1/forms
Ready to get started?