How to Create or Update Contacts with the FreshDesk API in Python
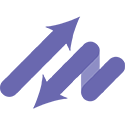
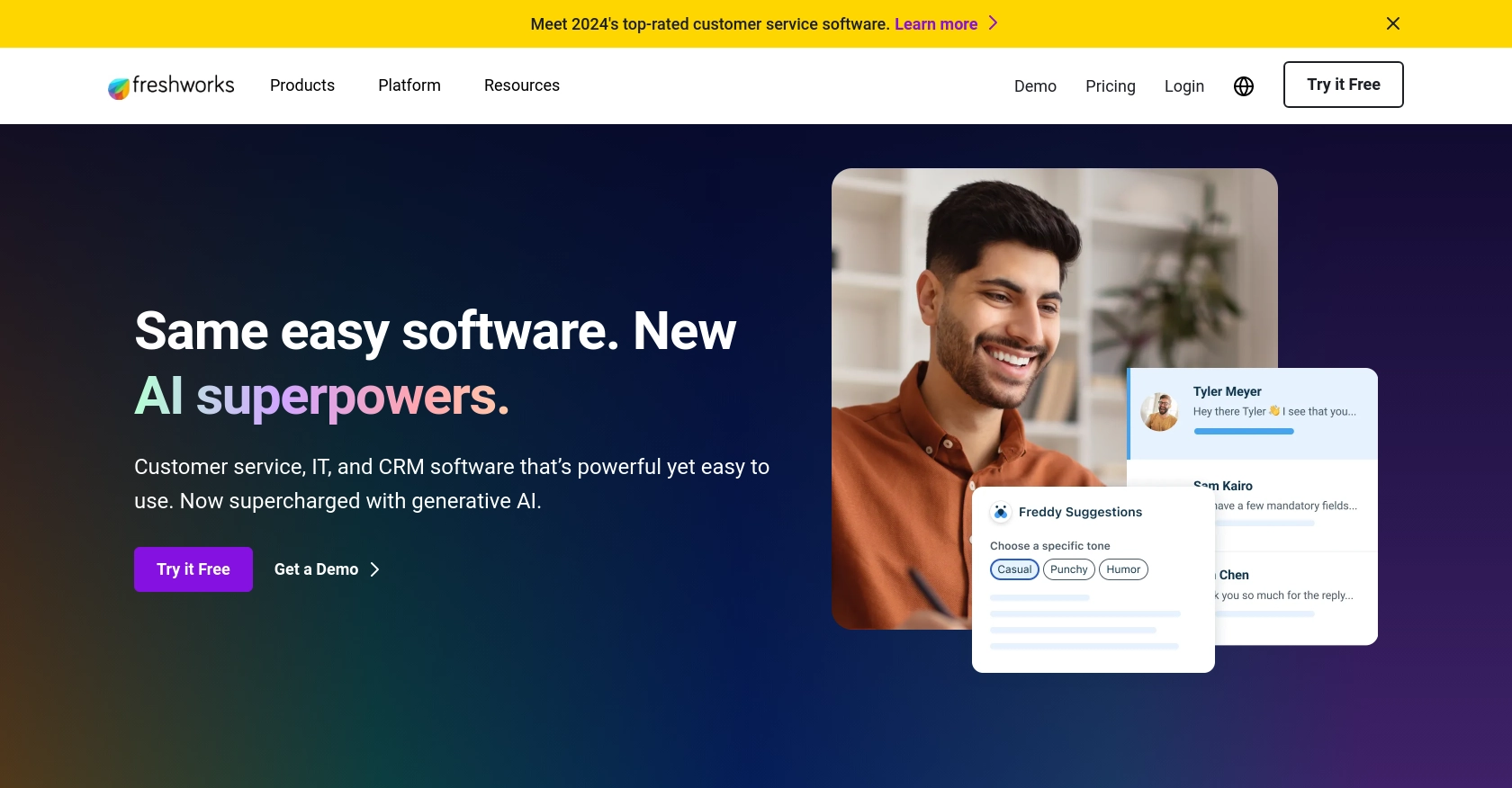
Introduction to FreshDesk API Integration
FreshDesk is a powerful customer support software that enables businesses to streamline their customer service operations. With features like ticketing, automation, and reporting, FreshDesk helps organizations manage customer interactions efficiently and effectively.
Integrating with the FreshDesk API allows developers to automate and enhance customer support processes. For example, you can create or update contact information directly from your application, ensuring that your customer data is always up-to-date and accessible. This integration can be particularly useful for businesses looking to personalize customer interactions or synchronize data across multiple platforms.
Setting Up Your FreshDesk Test Account for API Integration
Before you can start integrating with the FreshDesk API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. FreshDesk offers a free trial that you can use to access the necessary features for development and testing.
Creating a FreshDesk Account
To get started, visit the FreshDesk signup page and create a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you will have access to the FreshDesk dashboard, where you can manage your customer support operations.
Generating API Credentials for FreshDesk
FreshDesk uses a custom authentication method for API access. To generate the necessary credentials, follow these steps:
- Log in to your FreshDesk account and navigate to the Admin section.
- Under the Security category, click on the API section.
- Here, you will find your API key. Copy this key, as you will need it to authenticate your API requests.
Ensure that you store your API key securely and do not share it publicly, as it provides access to your FreshDesk account.
Setting Up a Test Environment
With your FreshDesk account and API key ready, you can now set up a test environment. This involves creating sample contacts and data that you can use to test your API calls. Follow these steps:
- In the FreshDesk dashboard, navigate to the Contacts section.
- Create a few test contacts by clicking on the "New Contact" button and filling in the necessary details.
- These contacts will be used to test the create and update functionalities of the FreshDesk API.
By setting up a test environment, you can ensure that your API interactions are functioning correctly before deploying them to a live environment.
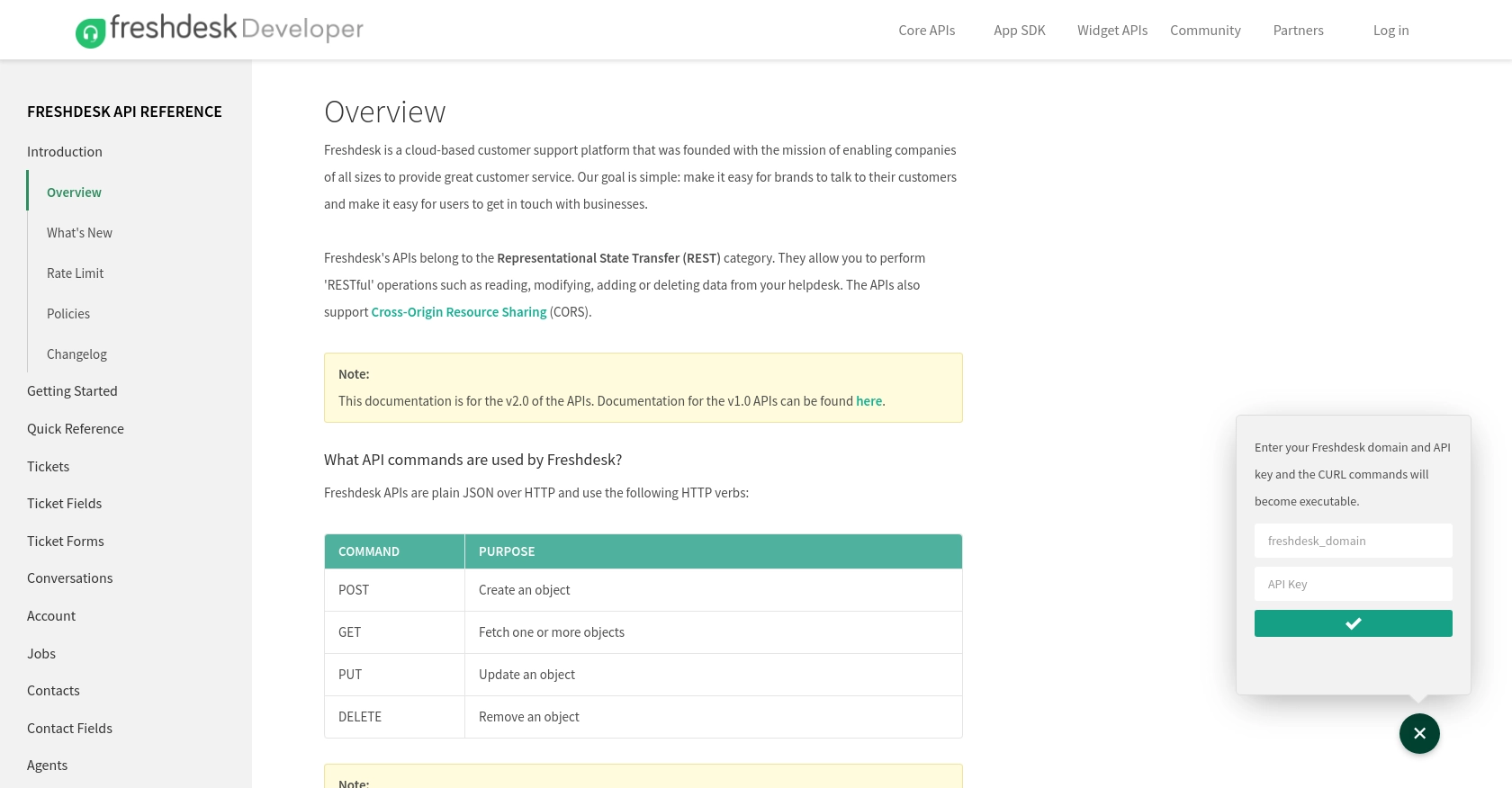
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with FreshDesk API in Python
To interact with the FreshDesk API using Python, you'll need to ensure you have the correct version of Python installed and the necessary dependencies. This section will guide you through the process of making API calls to create or update contacts in FreshDesk.
Setting Up Your Python Environment for FreshDesk API Integration
Before you begin, make sure you have Python 3.7 or later installed on your machine. You'll also need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Creating or Updating Contacts Using FreshDesk API
With your environment set up, you can now create or update contacts in FreshDesk. Below is a sample Python script to perform these actions:
import requests
# FreshDesk API endpoint for contacts
url = "https://yourdomain.freshdesk.com/api/v2/contacts"
# Your FreshDesk API key
api_key = "Your_API_Key"
# Headers for the request
headers = {
"Content-Type": "application/json"
}
# Contact data to create or update
contact_data = {
"name": "John Doe",
"email": "john.doe@example.com",
"phone": "1234567890"
}
# Make a POST request to create or update a contact
response = requests.post(url, auth=(api_key, "X"), headers=headers, json=contact_data)
# Check the response status
if response.status_code == 201:
print("Contact created successfully.")
elif response.status_code == 200:
print("Contact updated successfully.")
else:
print(f"Failed to create or update contact. Status code: {response.status_code}")
Replace Your_API_Key
with the API key you obtained from your FreshDesk account. The script uses the requests.post
method to send a request to the FreshDesk API, either creating a new contact or updating an existing one based on the provided data.
Verifying API Request Success in FreshDesk
After running the script, you can verify the success of your API request by checking the FreshDesk dashboard. Navigate to the Contacts section to see if the contact has been created or updated as expected.
Handling Errors and Troubleshooting FreshDesk API Calls
It's important to handle potential errors when making API calls. The script checks the response status code to determine if the operation was successful. If the status code is not 200 or 201, it prints an error message. You can further investigate by examining the response content:
if response.status_code not in [200, 201]:
print(f"Error: {response.json()}")
For more detailed error handling, refer to the FreshDesk API documentation.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API using Python can significantly enhance your customer support operations by automating contact management. By following the steps outlined in this article, you can efficiently create or update contacts within your FreshDesk account, ensuring your customer data remains accurate and up-to-date.
Best Practices for Secure and Efficient FreshDesk API Usage
- Secure Storage of API Credentials: Always store your FreshDesk API key securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of FreshDesk's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit errors gracefully.
- Data Standardization: Ensure that contact data is standardized before making API calls to maintain consistency across your systems.
- Error Handling: Implement robust error handling to manage API call failures. Use logging to capture error details for troubleshooting.
Streamlining Integrations with Endgrate
While integrating with FreshDesk can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including FreshDesk. This allows you to focus on your core product while Endgrate handles the intricacies of integration.
By leveraging Endgrate, you can save time and resources, build once for each use case, and offer an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
Ready to get started?