Using the Microsoft Dynamics 365 API to Get Accounts (with PHP examples)
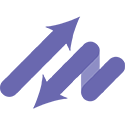
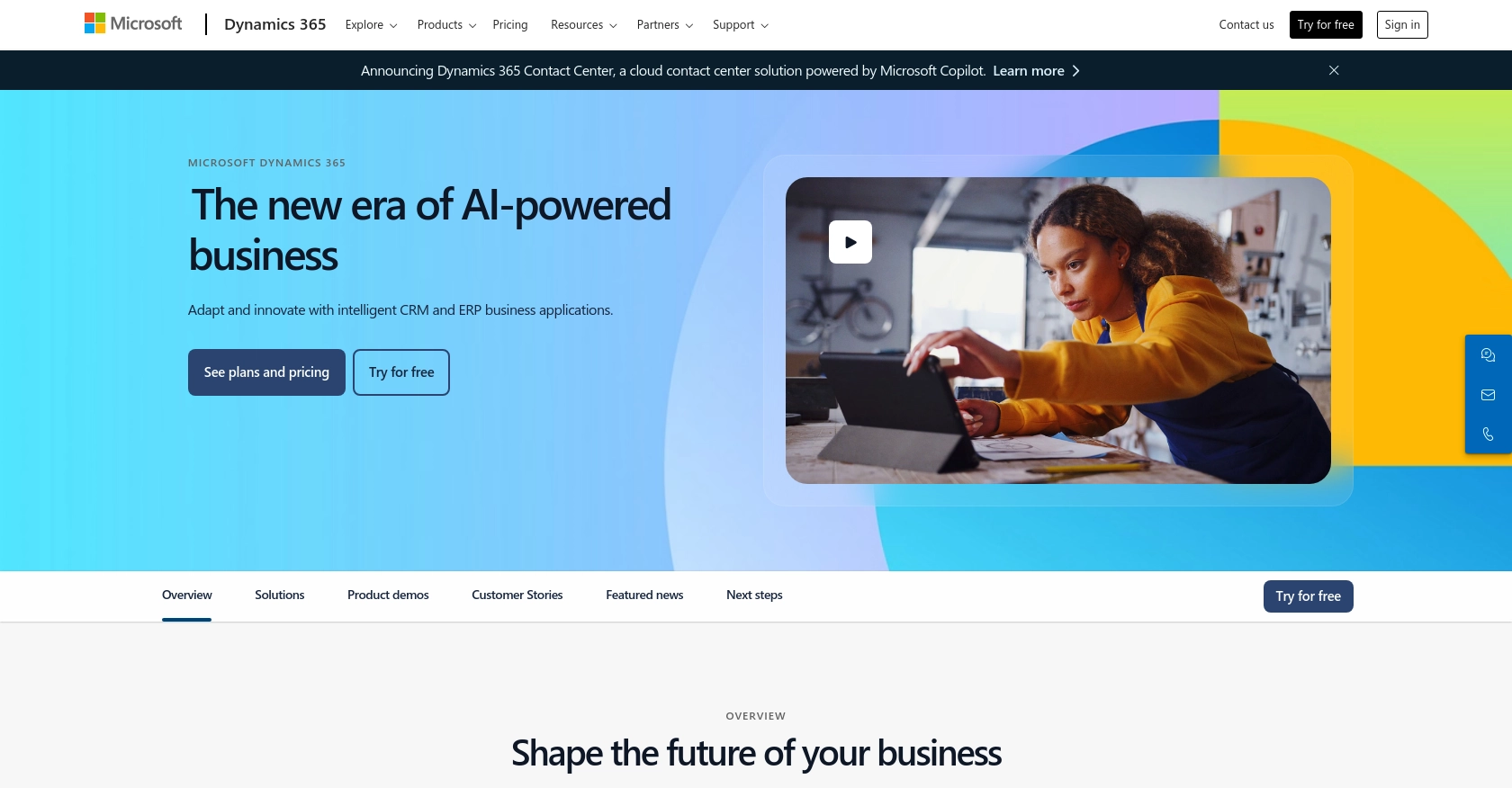
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a flexible and scalable platform that integrates seamlessly with other Microsoft services, making it a popular choice for businesses looking to enhance their operational efficiency.
Developers often seek to integrate with Microsoft Dynamics 365 to access and manage business data, such as accounts, to automate workflows and improve data accuracy. For example, a developer might use the Dynamics 365 API to retrieve account information and synchronize it with another business system, ensuring that all platforms reflect the most current data.
Setting Up a Microsoft Dynamics 365 Test or Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Microsoft Dynamics 365 Free Trial Account
If you don't already have a Microsoft Dynamics 365 account, you can sign up for a free trial. This trial provides access to the full suite of Dynamics 365 applications, allowing you to explore its features and capabilities.
- Visit the Microsoft Dynamics 365 website and click on "Try for free."
- Follow the on-screen instructions to create your account. You'll need to provide some basic information and verify your email address.
- Once your account is set up, you can access the Dynamics 365 dashboard and begin exploring the platform.
Setting Up a Microsoft Dynamics 365 Sandbox Environment
A sandbox environment is essential for testing and development. It allows you to test API interactions without impacting your production data.
- Log in to your Microsoft Dynamics 365 account.
- Navigate to the "Admin Center" and select "Environments."
- Click on "New" to create a new environment and choose "Sandbox" as the type.
- Configure the environment settings as needed and click "Create."
Registering an Application for OAuth Authentication
To interact with the Microsoft Dynamics 365 API, you'll need to register an application in your Microsoft Entra ID tenant. This registration will provide you with the necessary credentials for OAuth authentication.
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to "Azure Active Directory" and select "App registrations."
- Click "New registration" and fill in the required details, such as the application name and redirect URI.
- Once registered, note the "Application (client) ID" and "Directory (tenant) ID" for later use.
- Under "Certificates & secrets," create a new client secret and save it securely.
For more detailed instructions, refer to the official documentation on OAuth authentication with Microsoft Dataverse.
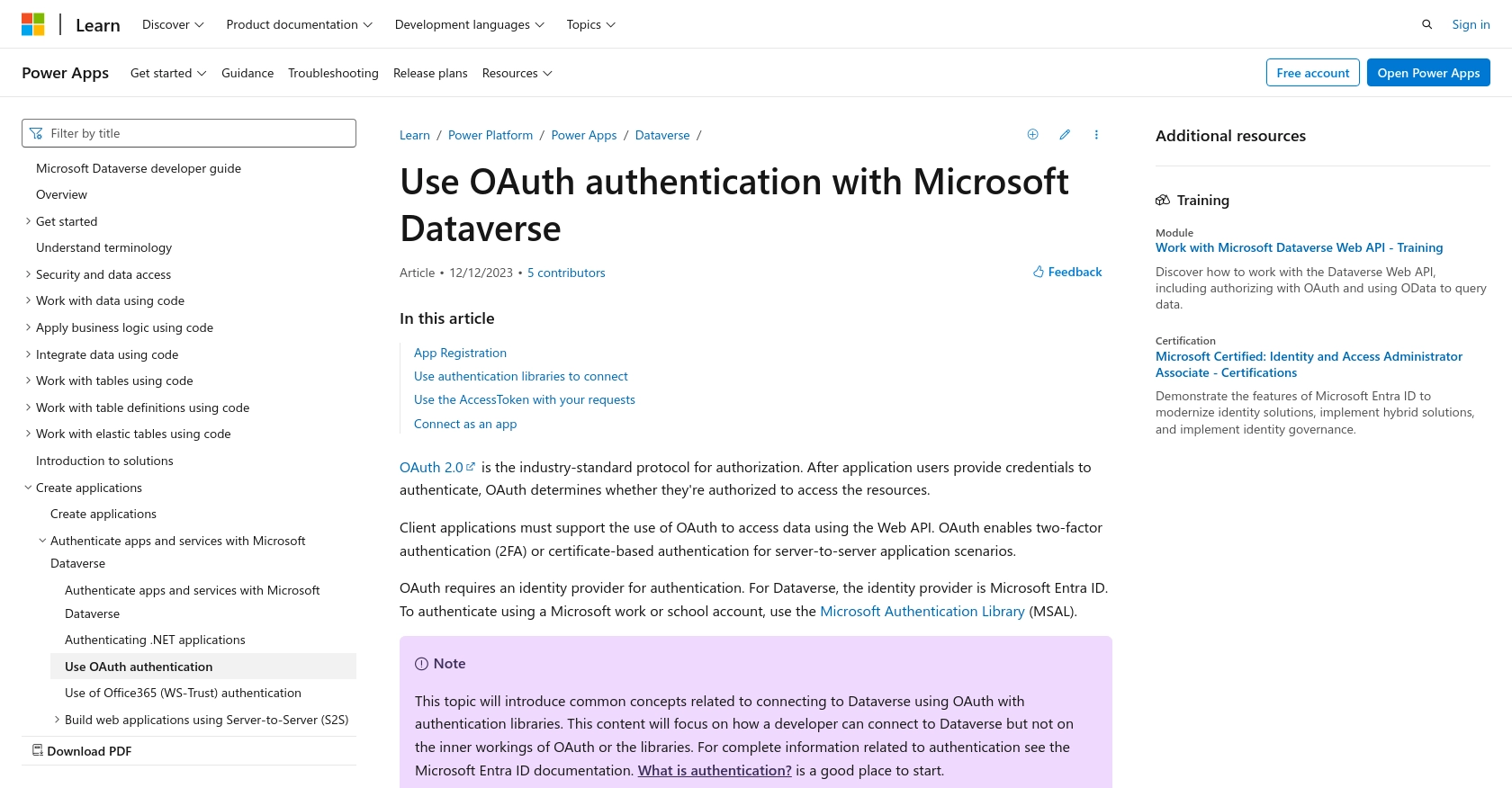
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Using PHP
To interact with the Microsoft Dynamics 365 API and retrieve account information, you'll need to use PHP to make HTTP requests. This section will guide you through setting up your PHP environment and executing the necessary API calls.
Setting Up Your PHP Environment for Microsoft Dynamics 365 API
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL
extension enabled. You can verify your PHP version and extensions by running the following command:
php -v
php -m | grep curl
If cURL
is not enabled, you can enable it by modifying your php.ini
file and restarting your web server.
Installing Required PHP Dependencies
To handle OAuth authentication and make HTTP requests, you'll need to install the guzzlehttp/guzzle
library. Use Composer to install it:
composer require guzzlehttp/guzzle
Authenticating with Microsoft Dynamics 365 API Using OAuth
First, authenticate with the Microsoft Dynamics 365 API using OAuth to obtain an access token. Here's a sample PHP script to perform the authentication:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://login.microsoftonline.com/{tenant_id}/oauth2/v2.0/token', [
'form_params' => [
'client_id' => '{client_id}',
'client_secret' => '{client_secret}',
'scope' => 'https://{resource}.api.crm.dynamics.com/.default',
'grant_type' => 'client_credentials',
]
]);
$body = json_decode($response->getBody(), true);
$accessToken = $body['access_token'];
?>
Replace {tenant_id}
, {client_id}
, {client_secret}
, and {resource}
with your specific values. This script will return an access token that you can use for subsequent API requests.
Retrieving Accounts from Microsoft Dynamics 365
With the access token, you can now retrieve account information from Microsoft Dynamics 365. Here's how to make the API call:
<?php
$response = $client->get('https://{businesscentralPrefix}/api/v2.0/companies({company_id})/accounts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
]
]);
$accounts = json_decode($response->getBody(), true);
foreach ($accounts['value'] as $account) {
echo 'Account ID: ' . $account['id'] . '<br>';
echo 'Account Name: ' . $account['displayName'] . '<br>';
}
?>
Replace {businesscentralPrefix}
and {company_id}
with your specific values. This script will output the account IDs and names retrieved from Microsoft Dynamics 365.
Handling Errors and Verifying API Call Success
To ensure your API calls are successful, check the HTTP status code and handle any errors appropriately. Here's an example of error handling:
<?php
try {
$response = $client->get('https://{businesscentralPrefix}/api/v2.0/companies({company_id})/accounts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
]
]);
if ($response->getStatusCode() === 200) {
$accounts = json_decode($response->getBody(), true);
// Process accounts
} else {
echo 'Error: ' . $response->getReasonPhrase();
}
} catch (Exception $e) {
echo 'Exception: ' . $e->getMessage();
}
?>
By checking the status code and catching exceptions, you can handle errors gracefully and ensure your application responds appropriately to any issues.
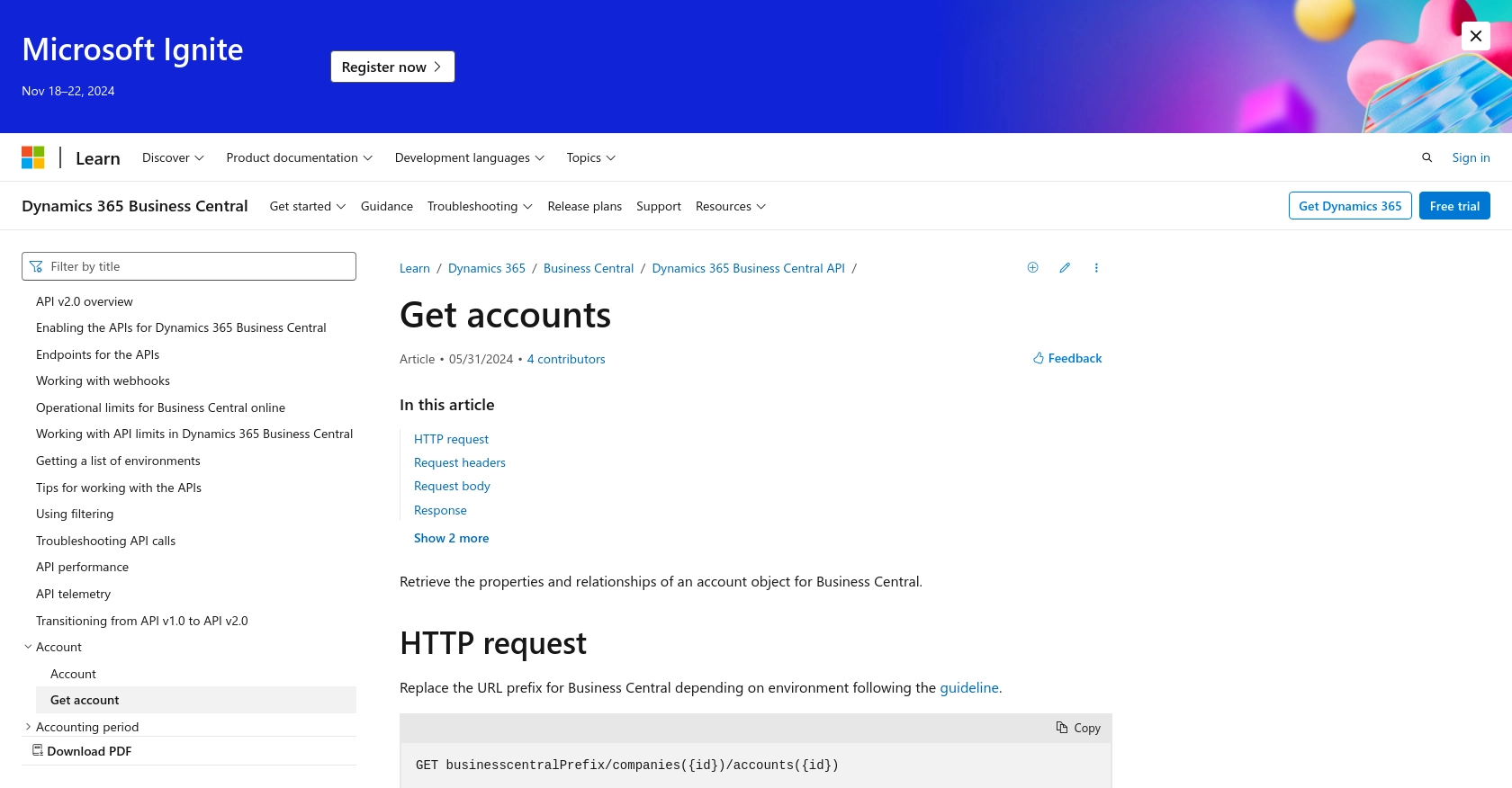
Conclusion and Best Practices for Microsoft Dynamics 365 API Integration
Integrating with the Microsoft Dynamics 365 API using PHP provides a powerful way to access and manage business data, enhancing your application's capabilities and streamlining workflows. By following the steps outlined in this guide, you can effectively authenticate and interact with the API to retrieve account information.
Best Practices for Secure and Efficient API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Microsoft Graph API documentation.
- Data Transformation and Standardization: Ensure that data retrieved from Microsoft Dynamics 365 is transformed and standardized to match your application's data structures, facilitating seamless integration.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Streamlining Integrations with Endgrate
While building integrations with Microsoft Dynamics 365 can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365. This allows developers to focus on core product development while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_account_get
Ready to get started?