How to Get Contacts with the Freshsales API in Javascript
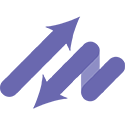
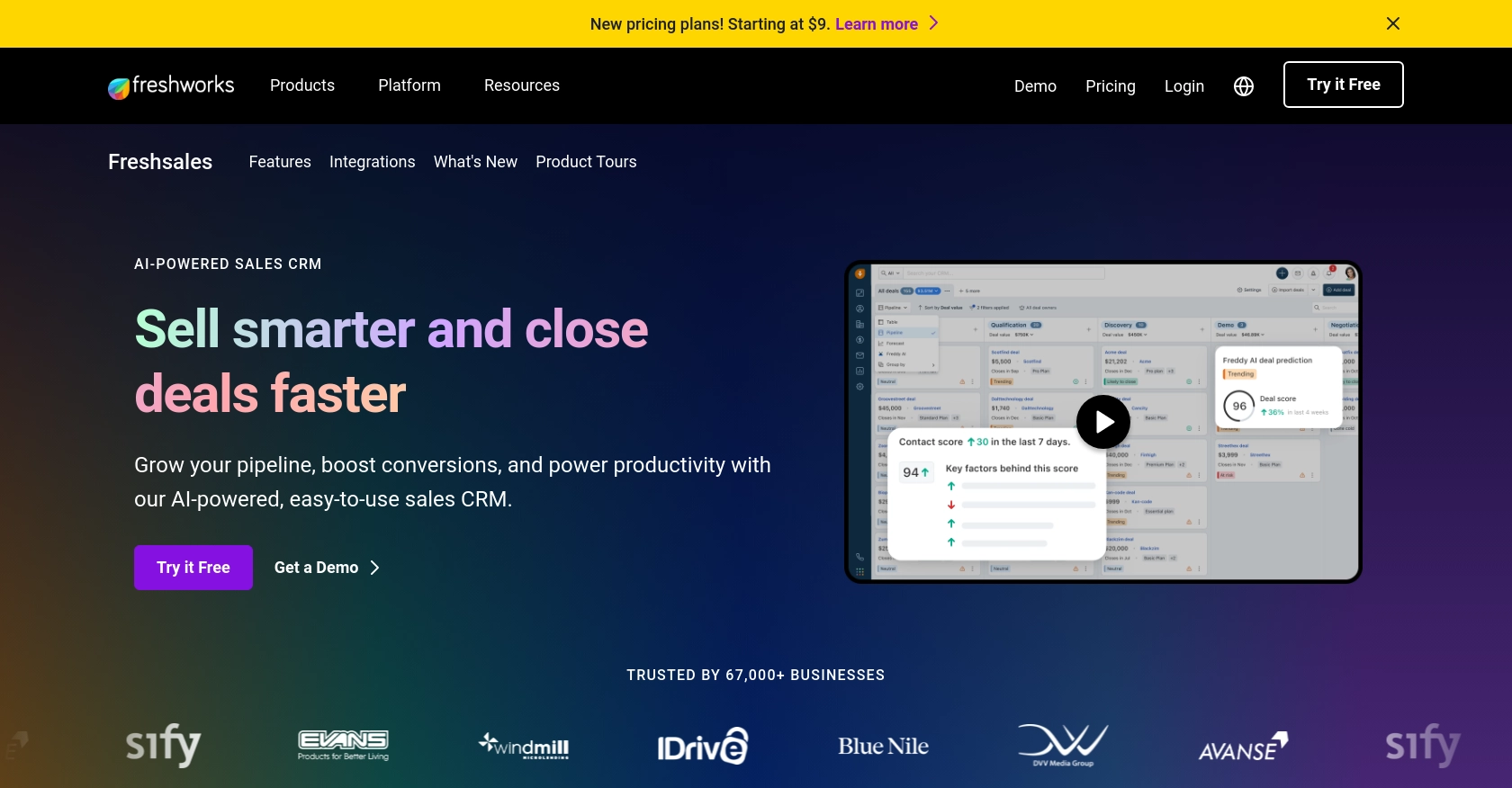
Introduction to Freshsales CRM
Freshsales is a powerful customer relationship management (CRM) platform designed to help businesses manage their sales processes more effectively. With features like lead scoring, email tracking, and built-in phone capabilities, Freshsales provides a comprehensive solution for sales teams to streamline their workflows and enhance customer interactions.
Integrating with the Freshsales API allows developers to access and manage customer data programmatically, enabling automation and customization of sales processes. For example, a developer might use the Freshsales API to retrieve contact information and integrate it with other business tools, enhancing data synchronization and improving sales efficiency.
Setting Up Your Freshsales Test Account for API Integration
Before you can start interacting with the Freshsales API using JavaScript, you'll need to set up a Freshsales account. This will allow you to access the necessary credentials and test your API calls in a controlled environment.
Creating a Freshsales Account
If you don't already have a Freshsales account, you can sign up for a free trial on the Freshsales website. This will give you access to the platform's features and allow you to experiment with the API.
- Visit the Freshsales website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating API Key for Freshsales API Access
Freshsales uses a custom authentication method that requires an API key to authorize requests. Follow these steps to generate your API key:
- Log in to your Freshsales account.
- Navigate to the "Settings" section, usually found in the top navigation bar.
- Under "API Settings," find the option to generate an API key.
- Click on "Generate API Key" and copy the key provided. Store it securely, as you'll need it to authenticate your API requests.
Configuring Your Freshsales Sandbox Environment
To ensure your API calls do not affect live data, it's best to use a sandbox environment:
- Check if Freshsales offers a sandbox or test environment as part of your account setup.
- If available, switch to the sandbox environment through the account settings.
- Create sample contacts and data within the sandbox to test your API interactions without impacting real customer data.
With your Freshsales account and API key ready, you're all set to start making API calls using JavaScript. In the next section, we'll explore how to perform these calls effectively.
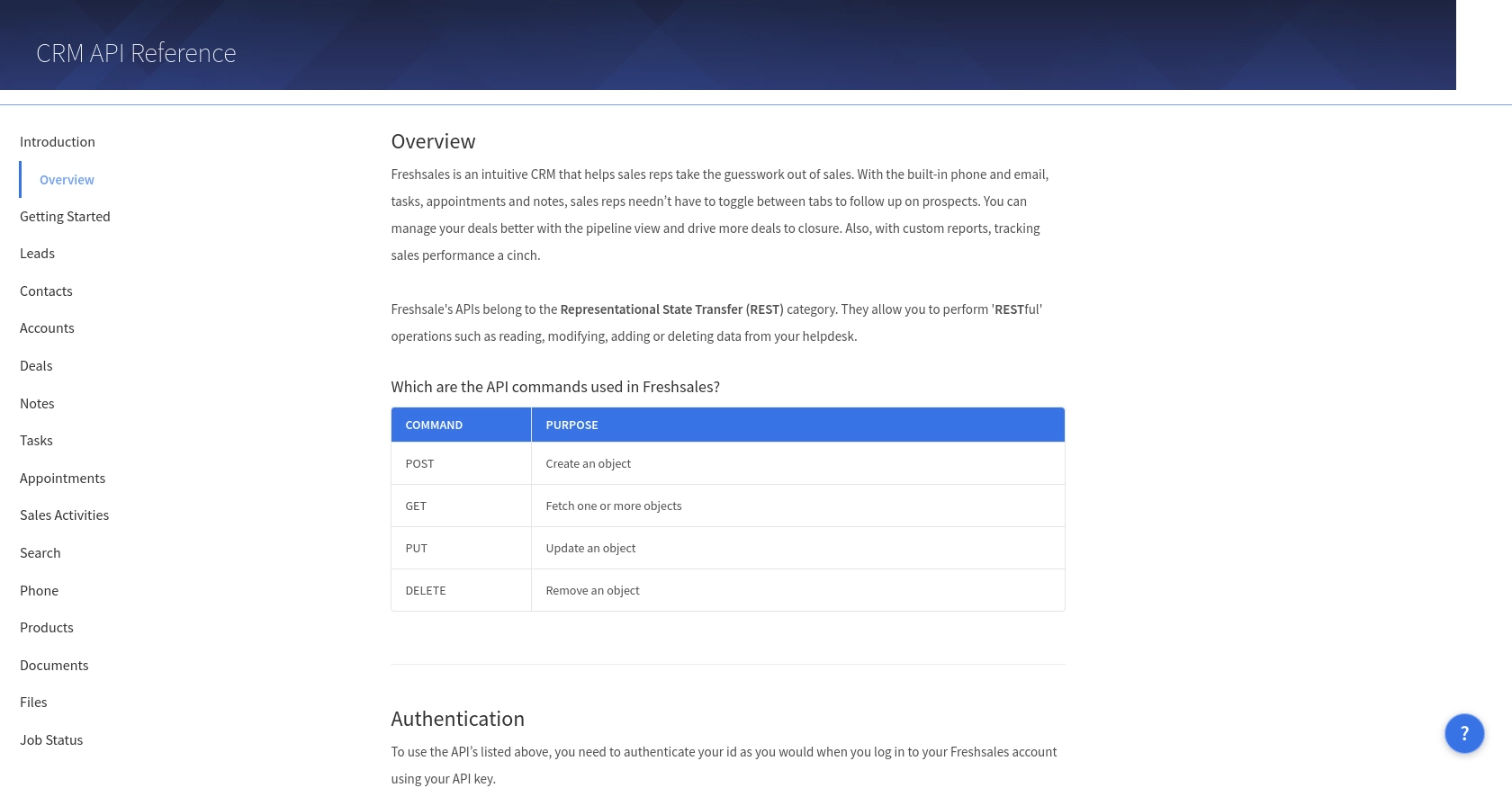
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Freshsales Using JavaScript
To interact with the Freshsales API and retrieve contact information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment and writing the code necessary to fetch contacts from Freshsales.
Setting Up Your JavaScript Environment for Freshsales API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code to write and execute your JavaScript code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Writing JavaScript Code to Fetch Contacts from Freshsales
With your environment set up, you can now write the JavaScript code to retrieve contacts from Freshsales. Create a new file named get_freshsales_contacts.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://yourdomain.freshsales.io/api/contacts';
const apiKey = 'Your_API_Key'; // Replace with your actual API key
// Configure the request headers
const headers = {
'Authorization': `Token token=${apiKey}`,
'Content-Type': 'application/json'
};
// Function to fetch contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data.contacts;
// Display the contacts
contacts.forEach(contact => {
console.log(`Name: ${contact.first_name} ${contact.last_name}, Email: ${contact.email}`);
});
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getContacts();
In this code, you use the axios
library to make a GET request to the Freshsales API endpoint. The API key is included in the headers for authentication. The response is parsed to extract and display contact information.
Running Your JavaScript Code to Retrieve Freshsales Contacts
To execute the code and fetch contacts, run the following command in your terminal:
node get_freshsales_contacts.js
If successful, you should see a list of contacts printed in the console, including their names and email addresses.
Handling Errors and Verifying API Call Success
It's important to handle potential errors when making API calls. The code above includes a try-catch
block to catch and log errors. Common error codes include:
- 401 Unauthorized: Check your API key and ensure it's correctly included in the headers.
- 404 Not Found: Verify the API endpoint URL.
- 500 Internal Server Error: This may indicate an issue with the Freshsales server. Try again later.
To verify the success of your API call, you can log into your Freshsales account and check the contacts section to ensure the data matches the retrieved information.
Best Practices for Freshsales API Integration and Conclusion
Integrating with the Freshsales API can significantly enhance your sales processes by automating data retrieval and synchronization. To ensure a smooth and efficient integration, consider the following best practices:
Securely Storing Freshsales API Credentials
Always store your Freshsales API key securely. Avoid hardcoding it directly in your code. Instead, use environment variables or a secure vault to manage sensitive information. This practice helps prevent unauthorized access and potential security breaches.
Handling Freshsales API Rate Limits
Be mindful of any rate limits imposed by Freshsales. While specific rate limit details were not available in the documentation, it's crucial to implement error handling and retry logic to manage rate-limited responses gracefully. This ensures your application remains robust and responsive.
Standardizing and Transforming Data
When retrieving contact data from Freshsales, consider transforming and standardizing the data fields to match your application's requirements. This can include formatting names, normalizing email addresses, or converting timestamps to a preferred format.
Conclusion and Call to Action
By following this guide, you can effectively integrate with the Freshsales API using JavaScript to retrieve contact information. This integration empowers your sales team with up-to-date data, enhancing decision-making and customer interactions.
If you're looking to streamline your integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API experience, enabling you to build once for each use case and easily connect with multiple platforms, including Freshsales.
Explore how Endgrate can simplify your integration needs by visiting Endgrate today.
Read More
Ready to get started?