Using the OnePageCRM API to Get Contacts in PHP
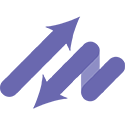
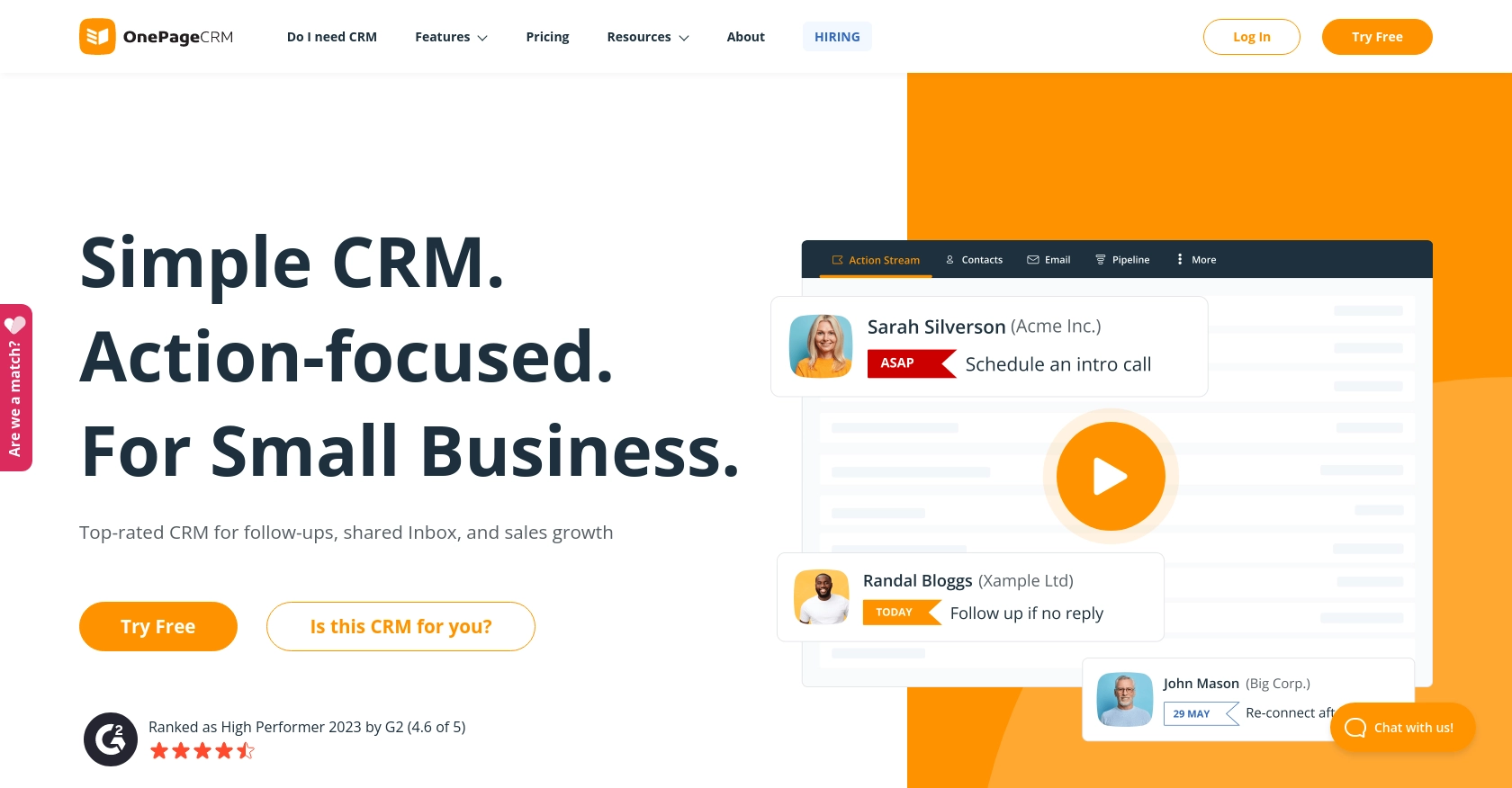
Introduction to OnePageCRM
OnePageCRM is a dynamic customer relationship management (CRM) platform designed to simplify sales processes for businesses. It offers a streamlined approach to managing contacts, deals, and tasks, making it an ideal choice for sales teams looking to enhance their productivity and efficiency.
Integrating with OnePageCRM's API allows developers to access and manage contact data programmatically, enabling seamless integration with other systems and applications. For example, a developer might use the OnePageCRM API to retrieve contact information and synchronize it with a custom sales dashboard, providing real-time insights and updates.
Setting Up Your OnePageCRM Test Account
Before you can start interacting with the OnePageCRM API, you'll need to set up a test account. This will allow you to experiment with API calls without affecting any live data. Follow these steps to get started:
Create a OnePageCRM Account
- Visit the OnePageCRM website and sign up for a free trial account.
- Follow the on-screen instructions to complete the registration process. Once registered, you'll have access to the OnePageCRM dashboard.
Generate API Credentials for OnePageCRM
To interact with the OnePageCRM API, you'll need to generate API credentials. These credentials will be used to authenticate your requests.
- Log in to your OnePageCRM account and navigate to the settings section.
- Find the API settings or developer section where you can create a new API key.
- Generate a new API key and make sure to copy it. You'll need this key to authenticate your API requests.
Understanding OnePageCRM Custom Authentication
OnePageCRM uses a custom authentication method. Ensure you have your API key ready, as it will be required for making API calls. Refer to the OnePageCRM API documentation for detailed information on authentication.
With your test account and API credentials set up, you're ready to start making API calls to OnePageCRM and explore its capabilities.
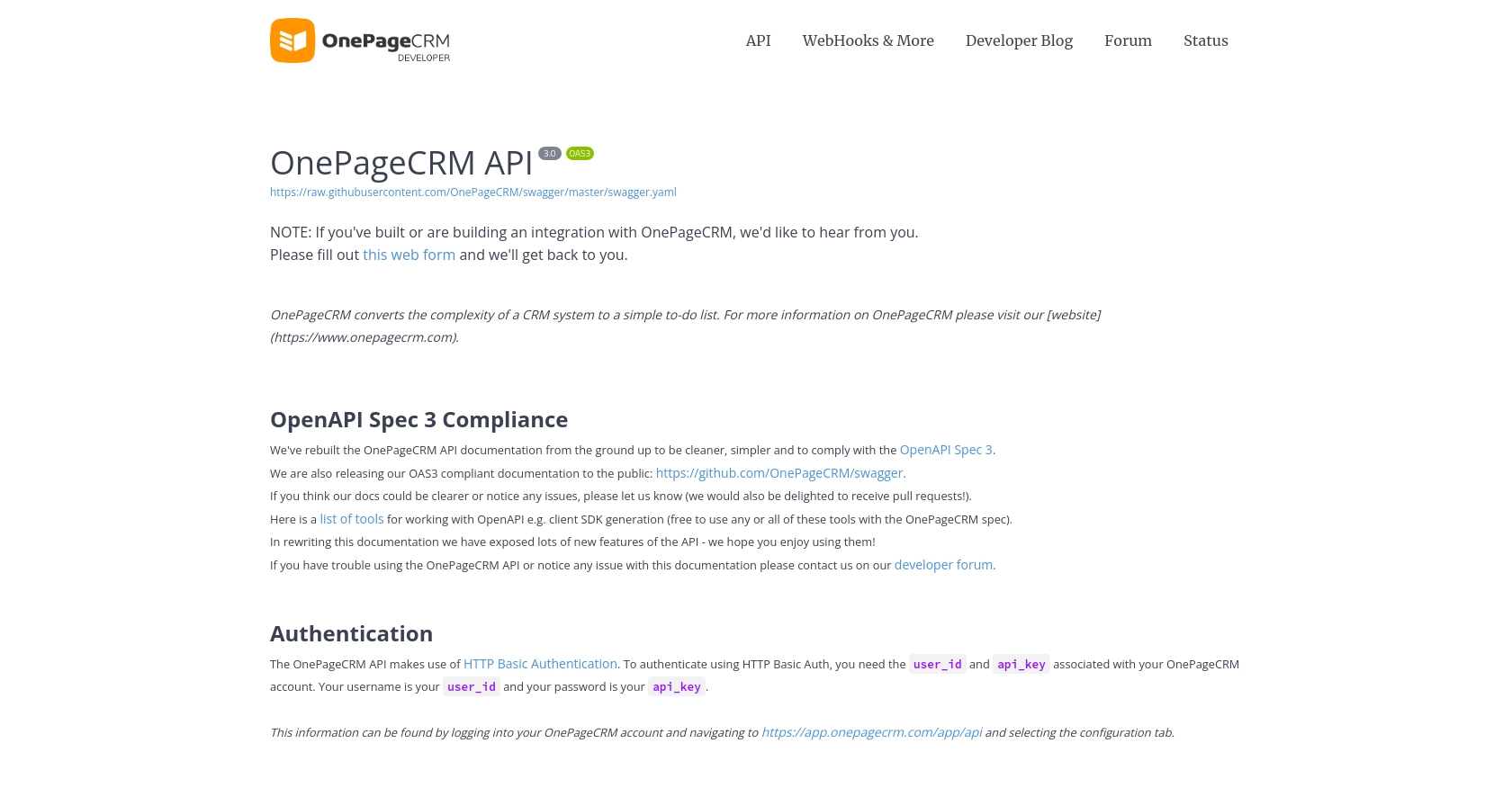
sbb-itb-96038d7
How to Make API Calls to Retrieve Contacts from OnePageCRM Using PHP
To interact with the OnePageCRM API and retrieve contacts, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment for OnePageCRM API Integration
Before you begin, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer, the PHP package manager
Once you have these installed, open your terminal and install the Guzzle HTTP client, which will be used to make HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Make OnePageCRM API Calls
Create a new PHP file named get_contacts.php
and add the following code to it:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key'; // Replace with your actual API key
try {
$response = $client->request('GET', 'https://app.onepagecrm.com/api/v3/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Accept' => 'application/json',
]
]);
$contacts = json_decode($response->getBody(), true);
foreach ($contacts['data'] as $contact) {
echo 'Name: ' . $contact['first_name'] . ' ' . $contact['last_name'] . '<br>';
echo 'Email: ' . $contact['email'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This script uses Guzzle to send a GET request to the OnePageCRM API to retrieve contacts. It then decodes the JSON response and prints out the contact names and emails.
Running the PHP Script and Verifying OnePageCRM API Response
Run the script from your terminal using the following command:
php get_contacts.php
If successful, you should see a list of contacts printed in your terminal. This confirms that the API call was successful and that you are able to retrieve contact information from OnePageCRM.
Handling Errors and Understanding OnePageCRM API Response Codes
When making API calls, it's important to handle potential errors. The OnePageCRM API may return various HTTP status codes, such as:
- 200 OK: The request was successful.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Ensure your code handles these errors gracefully, providing meaningful messages to help diagnose issues.
Best Practices for Using OnePageCRM API in PHP
When working with the OnePageCRM API, it's essential to follow best practices to ensure efficient and secure integration. Here are some key recommendations:
- Securely Store API Credentials: Always store your API key securely, preferably in environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid being throttled. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from OnePageCRM is transformed and standardized to fit your application's requirements, maintaining consistency across systems.
- Error Handling: Implement robust error handling to manage different HTTP status codes, providing clear messages and logging errors for troubleshooting.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple platforms. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including OnePageCRM.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while outsourcing integrations to Endgrate, reducing development time and costs.
- Build Once, Use Everywhere: Create a single integration for each use case, eliminating the need for multiple implementations across different platforms.
- Enhance User Experience: Provide your customers with an intuitive and seamless integration experience, improving satisfaction and engagement.
Explore how Endgrate can simplify your integration process by visiting Endgrate and discover the benefits of a unified integration solution.
Read More
Ready to get started?