Using the Sap Business One API to Get Vendors in PHP
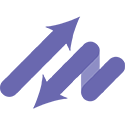
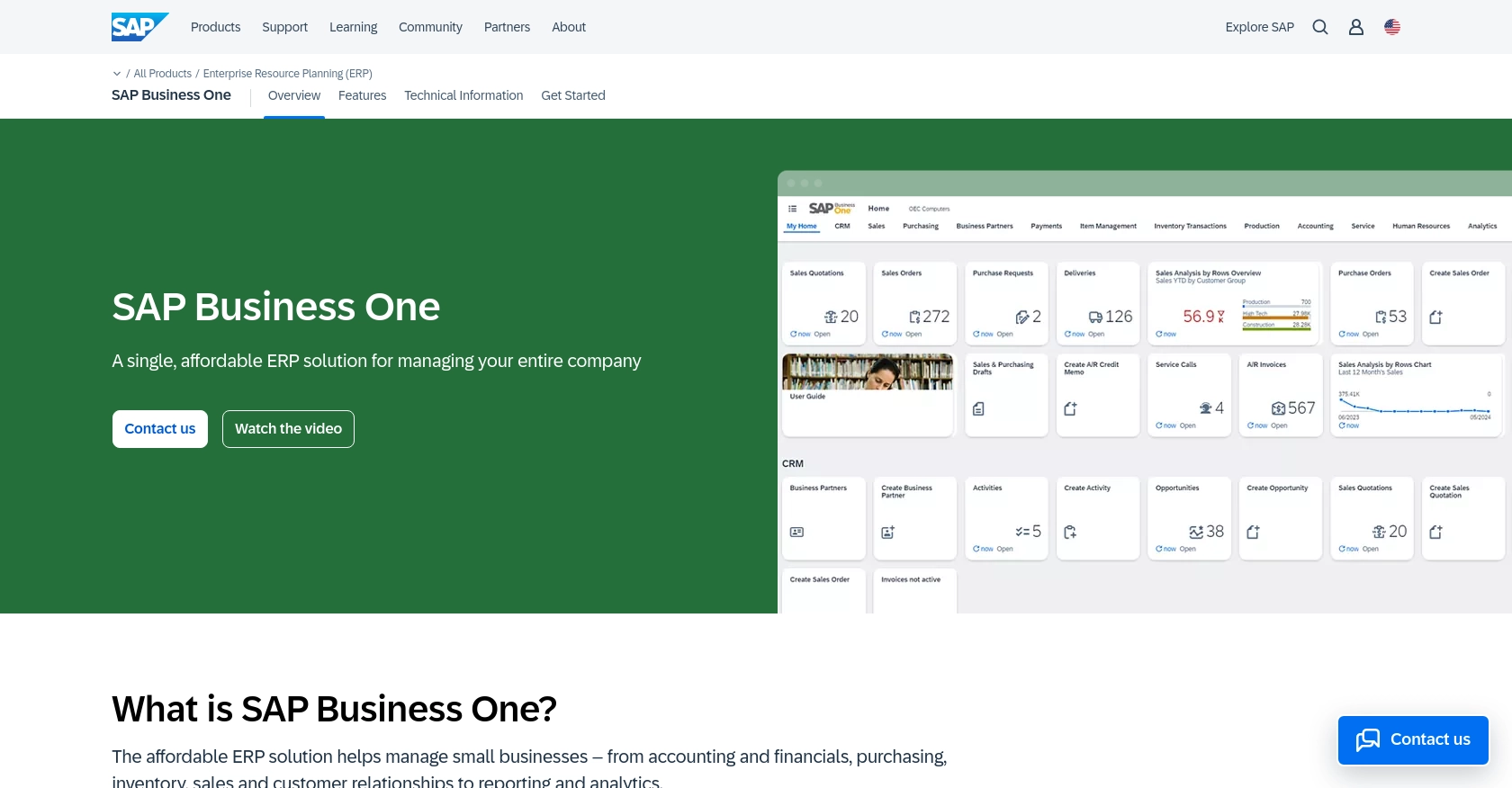
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers an integrated suite of tools to manage various business functions, including finance, sales, customer relationship management, and supply chain operations.
Developers often seek to integrate with SAP Business One's API to streamline business processes and enhance data management capabilities. For example, accessing vendor information through the SAP Business One API can help automate procurement workflows, ensuring that vendor data is up-to-date and easily accessible for decision-making.
Setting Up Your SAP Business One Test/Sandbox Account
Before you can start interacting with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a SAP Business One Sandbox Account
To begin, you'll need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a sandbox instance from your system administrator. If not, you may need to contact SAP or a certified SAP partner to set up a trial or demo account.
Generating API Credentials for SAP Business One
SAP Business One uses a custom authentication method for API access. Follow these steps to generate the necessary credentials:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer section, which is responsible for handling API requests.
- Create a new user specifically for API access, ensuring that it has the necessary permissions to interact with vendor data.
- Obtain the Company Database Name, User Name, and Password for this API user. These will be used to authenticate your API requests.
For more detailed instructions, refer to the SAP Business One Service Layer documentation.
Configuring Your Development Environment
Once you have your sandbox account and API credentials, configure your development environment to interact with the SAP Business One API:
- Ensure you have PHP installed on your machine. The recommended version is PHP 7.4 or higher.
- Install any necessary PHP extensions, such as
cURL
, to facilitate HTTP requests. - Set up a local development server or use a cloud-based environment to test your API interactions.
With your sandbox account and development environment ready, you can proceed to make API calls to retrieve vendor information from SAP Business One.
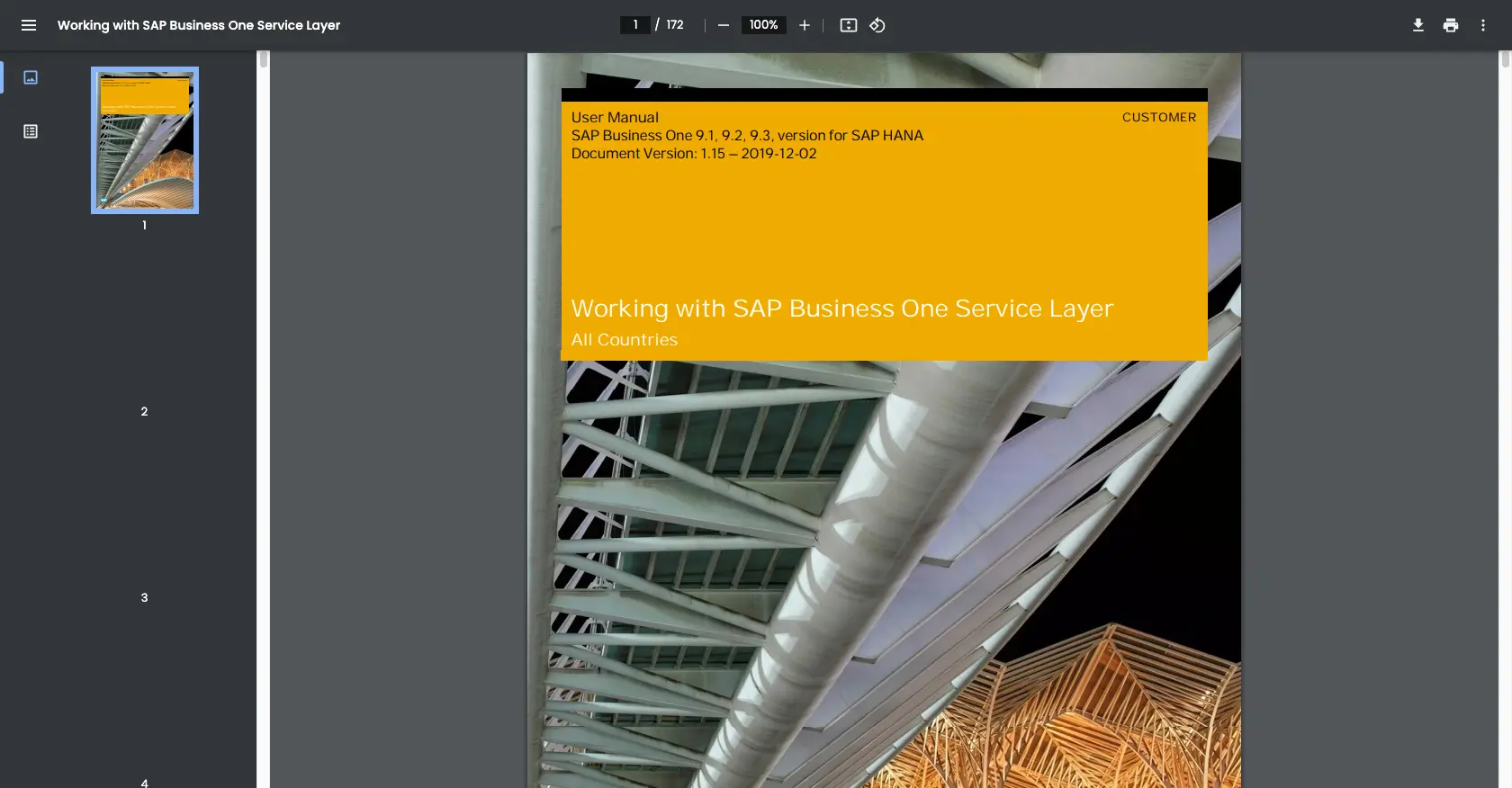
sbb-itb-96038d7
Making API Calls to Retrieve Vendor Information from SAP Business One Using PHP
To interact with the SAP Business One API and retrieve vendor information, you'll need to set up your PHP environment and make HTTP requests to the appropriate endpoints. This section will guide you through the process of making these API calls.
Setting Up Your PHP Environment for SAP Business One API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Verify that PHP 7.4 or higher is installed on your system.
- Ensure the
cURL
extension is enabled in your PHP configuration, as it is essential for making HTTP requests. - Set up a local or cloud-based development server to test your API interactions.
Writing PHP Code to Authenticate and Fetch Vendor Data from SAP Business One
With your environment ready, you can now write the PHP code to authenticate and retrieve vendor information from SAP Business One:
<?php
// Define the SAP Business One Service Layer URL
$serviceLayerUrl = "https://your-sap-server:50000/b1s/v1/";
// Set up authentication credentials
$companyDB = "Your_Company_DB";
$username = "Your_Username";
$password = "Your_Password";
// Initialize cURL session
$ch = curl_init();
// Set cURL options for authentication
curl_setopt($ch, CURLOPT_URL, $serviceLayerUrl . "Login");
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode([
"CompanyDB" => $companyDB,
"UserName" => $username,
"Password" => $password
]));
curl_setopt($ch, CURLOPT_HTTPHEADER, ["Content-Type: application/json"]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request and decode the response
$response = curl_exec($ch);
$sessionData = json_decode($response, true);
// Check if authentication was successful
if (isset($sessionData['SessionId'])) {
// Set the session ID for subsequent requests
$sessionId = $sessionData['SessionId'];
// Fetch vendor data
curl_setopt($ch, CURLOPT_URL, $serviceLayerUrl . "BusinessPartners?\$filter=CardType eq 'V'");
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
"Cookie: B1SESSION=" . $sessionId
]);
// Execute the request and decode the response
$vendorResponse = curl_exec($ch);
$vendorData = json_decode($vendorResponse, true);
// Output vendor information
foreach ($vendorData['value'] as $vendor) {
echo "Vendor Code: " . $vendor['CardCode'] . " - Vendor Name: " . $vendor['CardName'] . "<br>";
}
} else {
echo "Authentication failed. Please check your credentials.";
}
// Close the cURL session
curl_close($ch);
?>
In this code snippet, you start by defining the SAP Business One Service Layer URL and setting up your authentication credentials. The cURL
library is used to handle HTTP requests. First, you authenticate by sending a POST request with your credentials to the Login
endpoint. Upon successful authentication, you receive a session ID, which is then used to make a GET request to fetch vendor data.
Verifying API Call Success and Handling Errors
After executing the API call, verify the success of your request by checking the response data. If the request is successful, the vendor information will be displayed. If there are errors, ensure to handle them gracefully by checking the response status and error messages.
For further details on error codes and handling, refer to the SAP Business One Service Layer documentation.
Best Practices for Using the SAP Business One API in PHP
When integrating with the SAP Business One API, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store Credentials: Always store your SAP Business One credentials securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the SAP Business One API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a specified delay.
- Standardize Data Fields: Ensure that data fields retrieved from the API are standardized and transformed as needed to fit your application's data model.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for debugging and provide meaningful feedback to users when issues arise.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including SAP Business One. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing development overhead.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified integration solution.
Read More
Ready to get started?