Using the Microsoft Dynamics 365 Business Central API to Get Contacts in Javascript
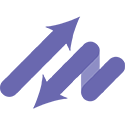
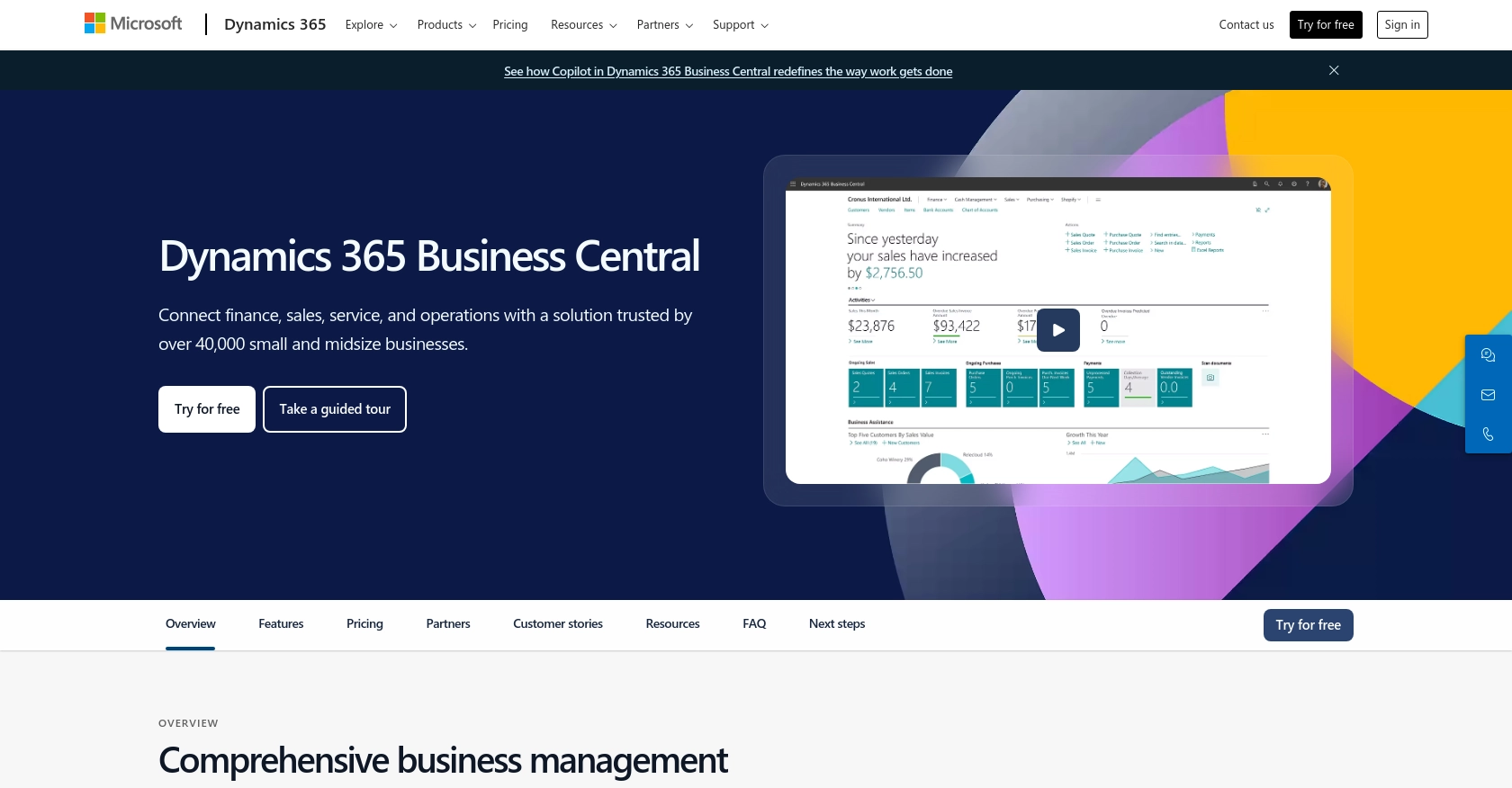
Introduction to Microsoft Dynamics 365 Business Central
Microsoft Dynamics 365 Business Central is a comprehensive business management solution designed for small to medium-sized enterprises. It offers a wide range of functionalities, including finance, sales, service, and operations management, all integrated into a single platform. This enables businesses to streamline their processes, improve customer interactions, and make informed decisions based on real-time data.
For developers, integrating with Microsoft Dynamics 365 Business Central's API provides the opportunity to automate and enhance business workflows. By accessing and managing data such as contacts, developers can create custom solutions that improve efficiency and productivity. For example, retrieving contact information through the API can help in synchronizing customer data across different platforms, ensuring consistency and accuracy.
Setting Up a Microsoft Dynamics 365 Business Central Sandbox Account
To begin integrating with the Microsoft Dynamics 365 Business Central API, you'll need to set up a sandbox account. This allows you to test your API interactions in a controlled environment without affecting live data.
Creating a Microsoft Dynamics 365 Business Central Sandbox Account
Follow these steps to create a sandbox account:
- Visit the Microsoft Dynamics 365 Business Central website and sign up for a free trial if you don't already have an account.
- Once logged in, navigate to the Admin Center.
- Select Environments from the menu, then click on New to create a new sandbox environment.
- Choose Sandbox as the type and fill in the necessary details.
- Click Create to set up your sandbox environment.
Registering an Application for OAuth Authentication
Since the Microsoft Dynamics 365 Business Central API uses OAuth for authentication, you'll need to register an application in Microsoft Entra ID:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to Azure Active Directory and select App registrations.
- Click on New registration and fill in the application details.
- Set the Redirect URI to
https://localhost
for local development. - Click Register to create the application.
Generating Client ID and Client Secret
After registering your application, you need to generate the client credentials:
- In the App registrations section, select your newly created app.
- Navigate to Certificates & secrets and click on New client secret.
- Provide a description and set an expiration period, then click Add.
- Copy the client secret value immediately as it will be hidden later.
Assigning API Permissions
To allow your application to access the Business Central API, assign the necessary permissions:
- In your app's settings, go to API permissions and click Add a permission.
- Select Dynamics 365 Business Central and choose the appropriate permissions for accessing contacts.
- Click Add permissions and ensure admin consent is granted.
With your sandbox environment and application registration complete, you're ready to start making API calls to Microsoft Dynamics 365 Business Central.
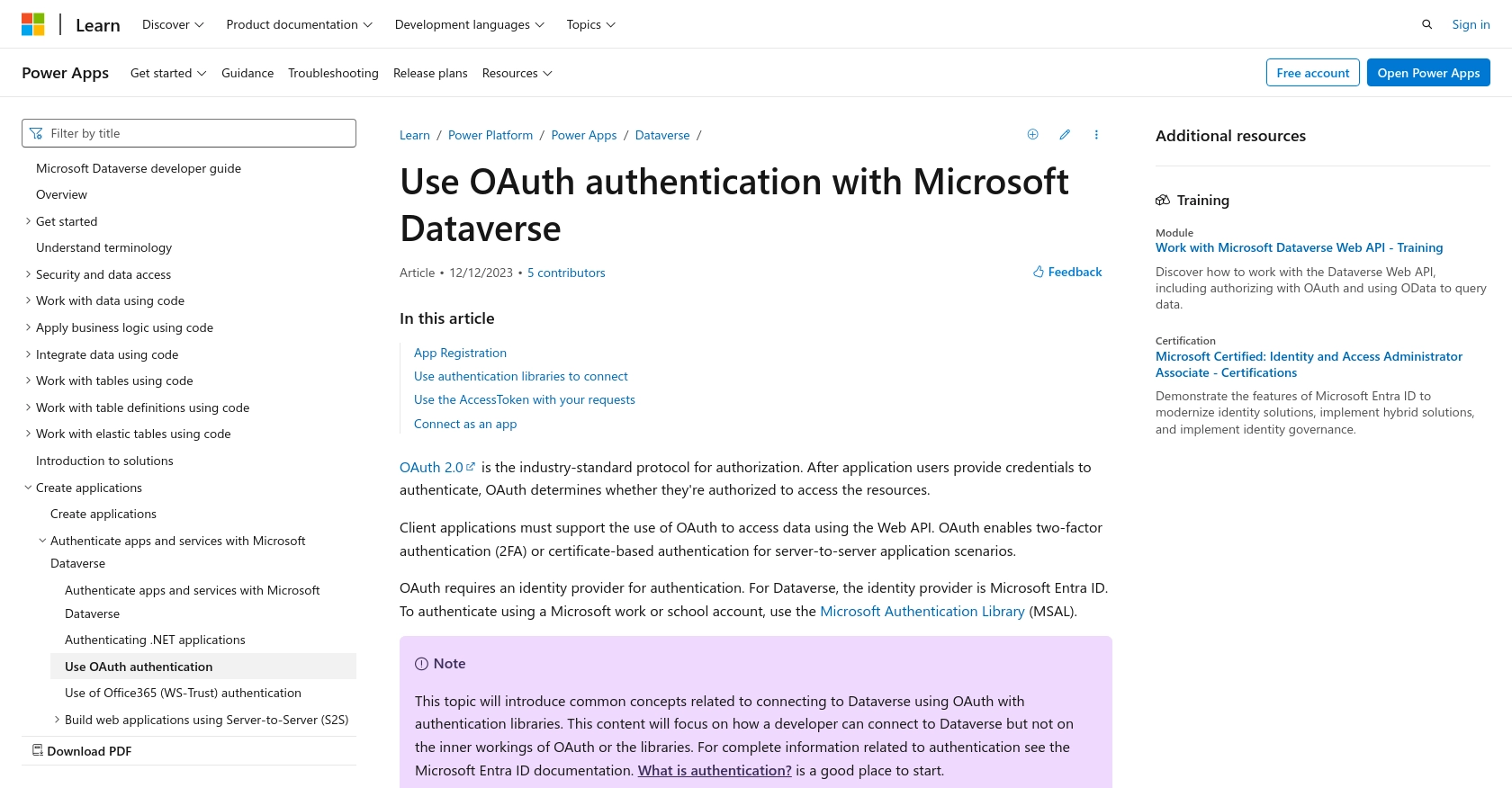
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Microsoft Dynamics 365 Business Central Using JavaScript
Setting Up Your JavaScript Environment
Before you begin making API calls to Microsoft Dynamics 365 Business Central, ensure you have a suitable JavaScript environment set up. You will need Node.js installed on your machine to run JavaScript outside the browser. Additionally, you will require the axios
library to handle HTTP requests.
- Install Node.js from the official website if you haven't already.
- Open your terminal and run the following command to install
axios
:
npm install axios
Writing JavaScript Code to Access Microsoft Dynamics 365 Business Central API
Now, let's write the JavaScript code to retrieve contact information from Microsoft Dynamics 365 Business Central. The following example demonstrates how to make a GET request to the API using OAuth authentication.
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://{businesscentralPrefix}/api/v2.0/companies({id})/contacts';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
console.log('Contacts retrieved successfully:', response.data);
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getContacts();
Replace Your_Access_Token
with the token obtained during the OAuth authentication process. The endpoint
URL should be updated with your specific Business Central environment details.
Verifying API Call Success and Handling Errors
After running the script, you should see the contact data printed in the console if the request is successful. Verify the retrieved data by checking it against the contacts in your Microsoft Dynamics 365 Business Central sandbox environment.
In case of errors, the script will log the error message. Common issues may include incorrect tokens or endpoint URLs. Refer to the official documentation for error code details and troubleshooting tips.
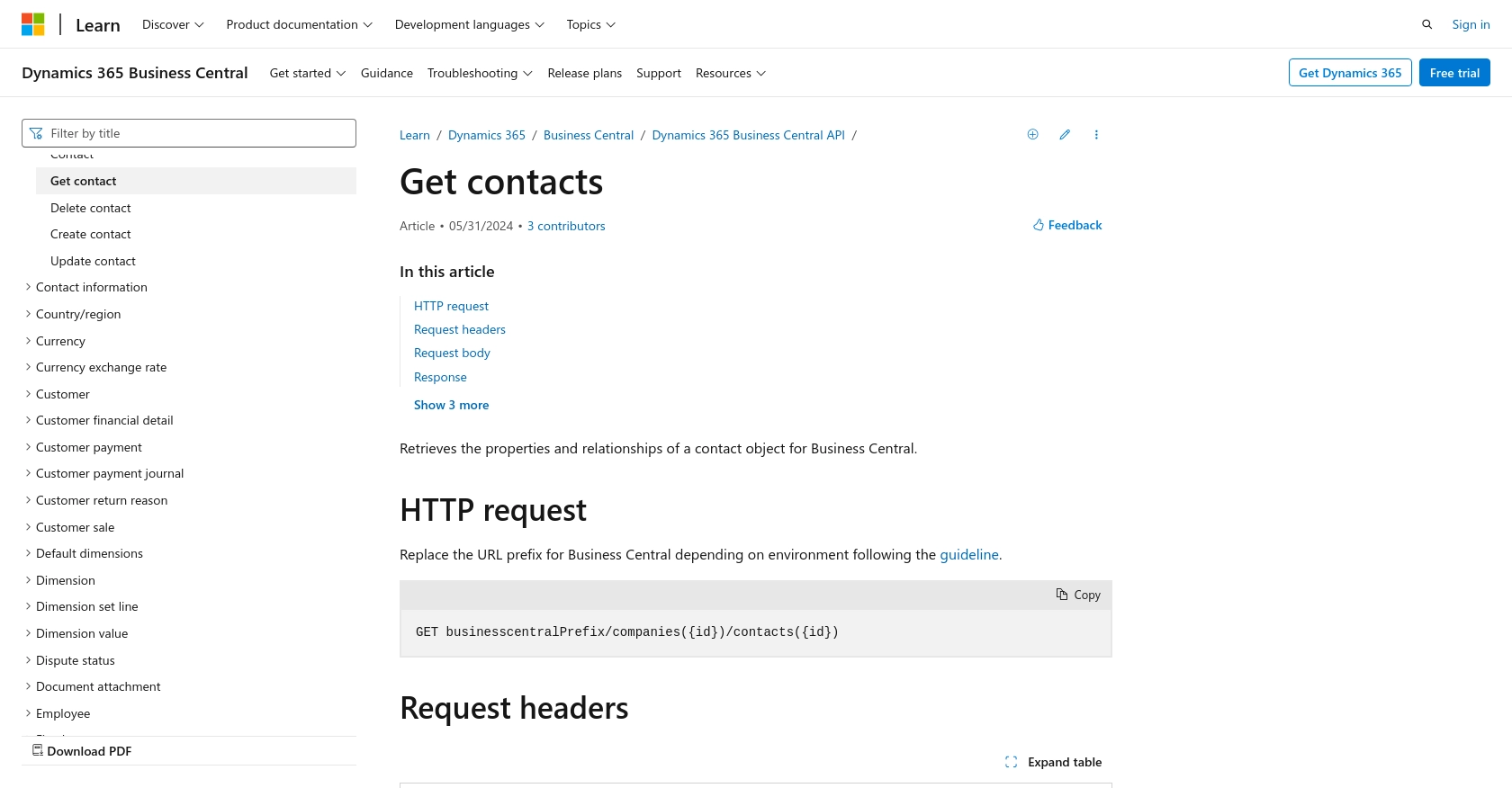
Conclusion and Best Practices for Using Microsoft Dynamics 365 Business Central API with JavaScript
Integrating with the Microsoft Dynamics 365 Business Central API using JavaScript offers developers a powerful way to automate and enhance business processes. By accessing contact data programmatically, you can ensure data consistency across platforms and streamline workflows.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your OAuth tokens and client secrets securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the API. Implement retry logic with exponential backoff to handle HTTP 429 status codes gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from the API is transformed and standardized to match your application's data structures for seamless integration.
Leverage Endgrate for Simplified Integration Management
While integrating with Microsoft Dynamics 365 Business Central can be rewarding, managing multiple integrations can become complex. Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Microsoft Dynamics 365 Business Central.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365businesscentral
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_contact_get
Ready to get started?