Using the Mailshake API to Create Leads in Python
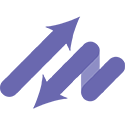
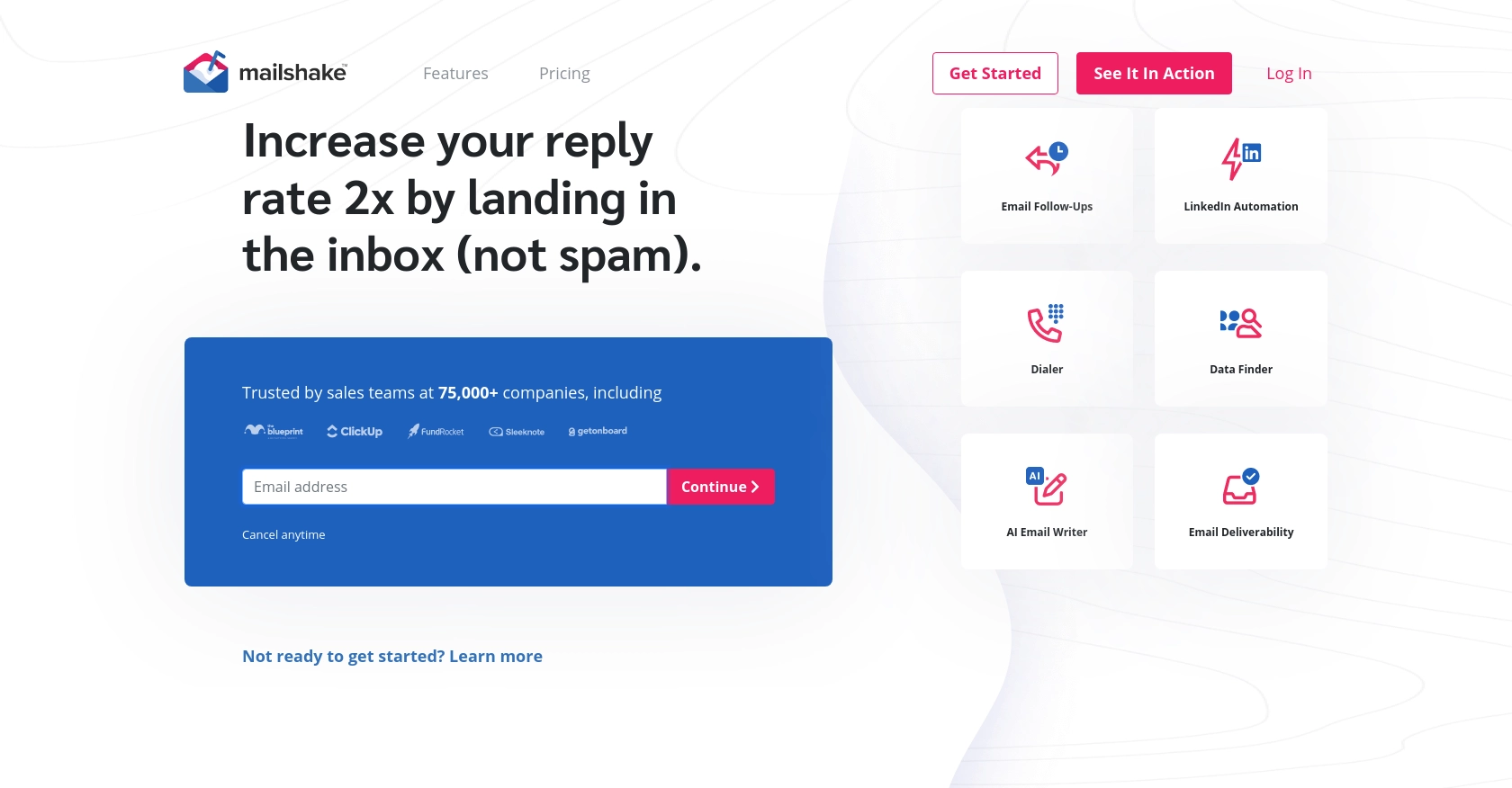
Introduction to Mailshake API
Mailshake is a powerful sales engagement platform designed to streamline outreach efforts through email campaigns, social media, and phone calls. It offers a comprehensive suite of tools to help sales teams automate and personalize their communication strategies, making it easier to connect with prospects and close deals.
Integrating with the Mailshake API allows developers to automate lead management processes, such as creating new leads directly from email campaigns. For example, you can use the Mailshake API to automatically generate leads from recipients who engage with your email content, enhancing your sales pipeline efficiency.
Setting Up Your Mailshake API Test Account
To begin integrating with the Mailshake API, you'll need to set up a test account. This involves creating an API key, which will allow you to authenticate your requests and interact with the Mailshake platform programmatically.
Creating a Mailshake Account
If you don't already have a Mailshake account, you can sign up for a free trial on the Mailshake website. This will give you access to the platform's features and allow you to test API interactions.
- Visit the Mailshake website and click on "Sign Up."
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating Your Mailshake API Key
Mailshake uses API keys for simple authentication. Follow these steps to generate your API key:
- Navigate to the "Extensions" section in the Mailshake dashboard.
- Select "API" from the menu options.
- Click on "Create API Key" to generate a new key.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
Make sure to replace my-api-key
in your code with the actual API key you generated.
Testing Your Mailshake API Connection
Before proceeding with API calls, it's a good idea to test your connection to ensure that your API key is working correctly. You can do this by making a simple request to the Mailshake API:
import requests
# Set the API endpoint and headers
url = "https://api.mailshake.com/2017-04-01/me"
headers = {
"Authorization": "Basic [base-64 encoded version of your api key]"
}
# Make a GET request to test the connection
response = requests.get(url, headers=headers)
# Check the response
if response.status_code == 200:
print("Connection successful!")
else:
print("Failed to connect:", response.json())
Replace [base-64 encoded version of your api key]
with your actual API key encoded in base64 format. A successful connection will confirm that your API key is valid and ready for use.
For more details on authentication, refer to the Mailshake API documentation.
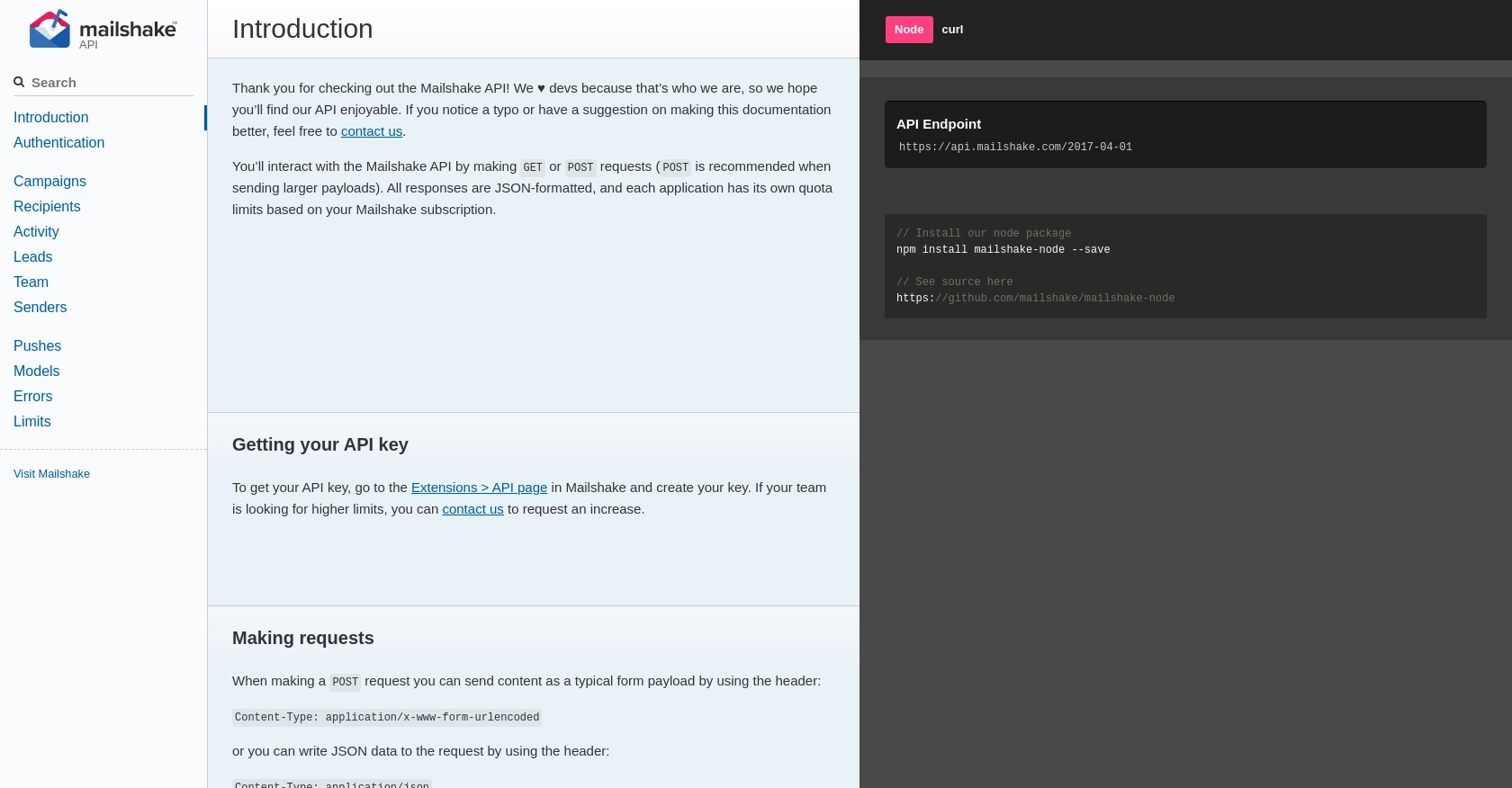
sbb-itb-96038d7
Making API Calls to Create Leads with Mailshake in Python
To interact with the Mailshake API and create leads, you'll need to use Python to send HTTP requests. This section will guide you through setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your Python Environment for Mailshake API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Install the
requests
library using pip:
pip install requests
Writing Python Code to Create Leads Using Mailshake API
Now, let's write a Python script to create leads using the Mailshake API. This involves sending a POST request to the appropriate endpoint with the necessary data.
import requests
# Set the API endpoint
url = "https://api.mailshake.com/2017-04-01/leads/create"
# Set the request headers
headers = {
"Authorization": "Basic [base-64 encoded version of your api key]",
"Content-Type": "application/x-www-form-urlencoded"
}
# Set the data for creating leads
data = {
"campaignID": 1, # Replace with your campaign ID
"emailAddresses": [
"example1@domain.com",
"example2@domain.com"
]
}
# Make a POST request to create leads
response = requests.post(url, headers=headers, data=data)
# Check the response
if response.status_code == 200:
print("Leads created successfully:", response.json())
else:
print("Failed to create leads:", response.json())
Replace [base-64 encoded version of your api key]
with your actual API key encoded in base64 format. Also, update the campaignID
and emailAddresses
with your specific data.
Verifying Successful Lead Creation in Mailshake
After running the script, you should verify that the leads have been successfully created in your Mailshake account. You can do this by checking the Mailshake dashboard for the new leads under the specified campaign.
Handling Errors and Understanding Mailshake API Responses
It's crucial to handle errors gracefully when interacting with the Mailshake API. Common error codes include:
invalid_api_key
: The API key is missing or invalid.missing_parameter
: A required parameter is missing from the request.limit_reached
: You've exceeded your quota and need to wait before making more requests.
For a complete list of error codes, refer to the Mailshake API documentation.
By following these steps, you can efficiently create leads using the Mailshake API and Python, streamlining your sales engagement processes.
Conclusion and Best Practices for Using Mailshake API in Python
Integrating with the Mailshake API to create leads using Python can significantly enhance your sales engagement processes by automating lead management and streamlining communication strategies. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and handle API interactions effectively.
Best Practices for Secure and Efficient Mailshake API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mailshake's rate limits and implement logic to handle
limit_reached
errors gracefully. Consider implementing exponential backoff strategies to retry requests. - Data Standardization: Ensure that the data you send to Mailshake is standardized and validated to avoid errors like
invalid_parameter
ormissing_parameter
. - Error Handling: Implement robust error handling to manage API responses effectively, logging errors for further analysis and troubleshooting.
Enhancing Integration Capabilities with Endgrate
If you're looking to simplify and expand your integration capabilities beyond Mailshake, consider using Endgrate. Endgrate offers a unified API endpoint that connects to multiple platforms, allowing you to manage integrations more efficiently. By leveraging Endgrate, you can save time and resources, focusing on your core product while providing an intuitive integration experience for your customers.
For more information on how Endgrate can help streamline your integration processes, visit Endgrate.
Read More
Ready to get started?