How to Create or Update Contacts with the Hubspot API in Python
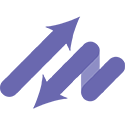
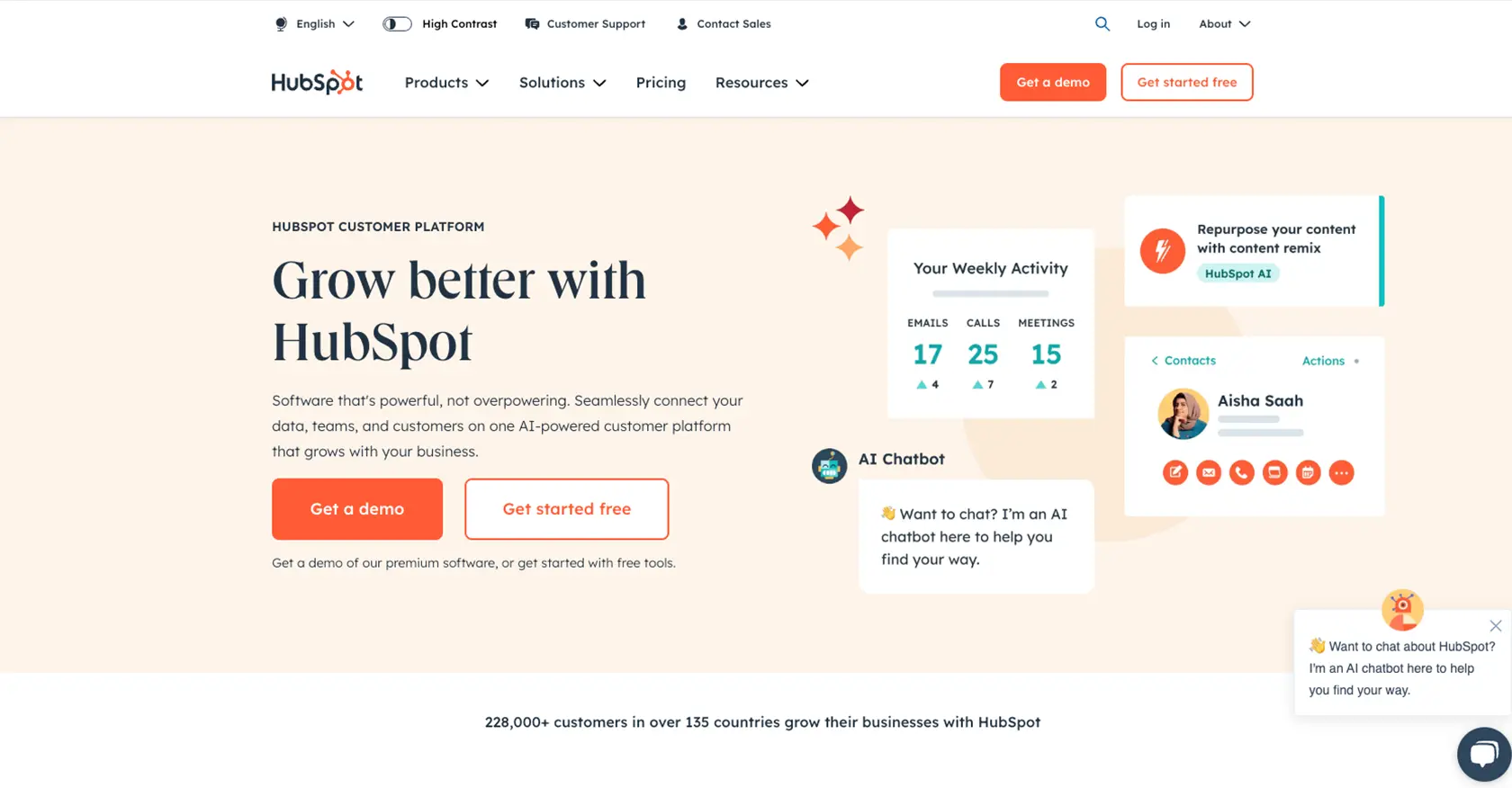
Introduction to HubSpot API Integration
HubSpot is a comprehensive CRM platform that offers a suite of tools to enhance marketing, sales, and customer service efforts. Its robust features and user-friendly interface make it a preferred choice for businesses aiming to streamline their customer relationship management.
Integrating with HubSpot's API allows developers to automate and manage customer data efficiently. For example, you can create or update contact information directly from your application, ensuring that your CRM data is always up-to-date and accurate. This capability is particularly useful for businesses looking to maintain personalized communication with their clients by leveraging real-time data updates.
Setting Up Your HubSpot Test or Sandbox Account for API Integration
Before you begin integrating with the HubSpot API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. HubSpot provides a developer-friendly setup to facilitate this process.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required details.
- Once registered, log in to your developer account to access the HubSpot dashboard.
Setting Up a HubSpot Sandbox Environment
HubSpot offers a sandbox environment for testing API integrations. Here's how to set it up:
- Navigate to the "Integrations" section in your HubSpot account.
- Select "Private Apps" and click on "Create a private app."
- Provide a name and description for your app, then proceed to configure the necessary scopes.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for secure API authentication. Follow these steps to configure OAuth:
- In your private app settings, navigate to the "Auth" tab.
- Select the required scopes such as
crm.objects.contacts.write
andcrm.objects.contacts.read
to manage contact data. - Save your settings to generate the client ID and client secret.
For more details on OAuth setup, refer to the HubSpot Authentication Documentation.
Generating and Storing OAuth Tokens
Once your app is configured, you'll need to generate OAuth tokens to authenticate API requests:
- Use the client ID and client secret to request an access token from HubSpot's OAuth server.
- Store the access token securely, as it will be used in the authorization header for API calls.
Remember to refresh the token periodically, as it has an expiration time. For more information, see the HubSpot API Usage Guidelines.
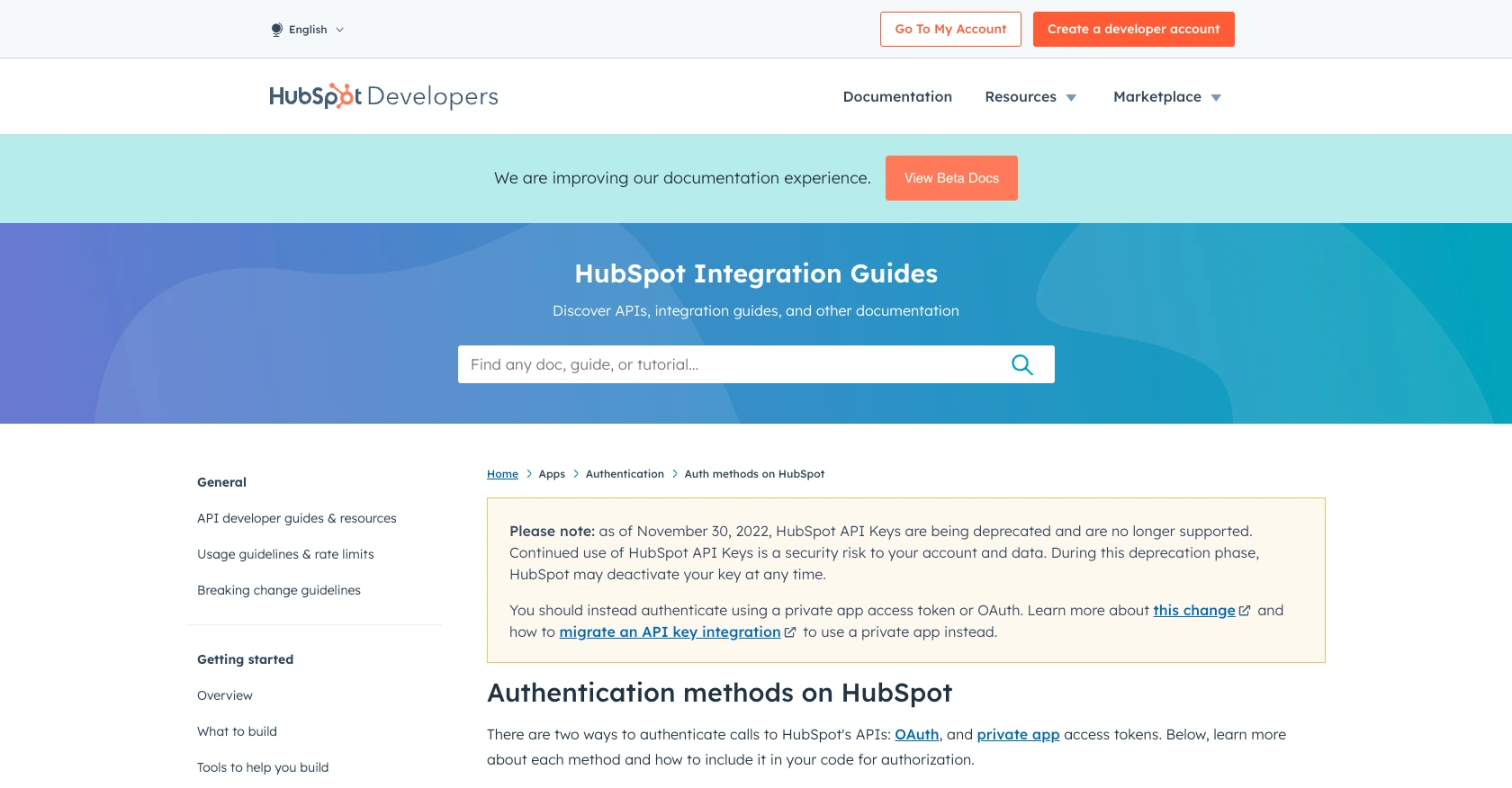
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with HubSpot API in Python
To interact with the HubSpot API for creating or updating contacts, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through setting up your Python environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for HubSpot API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Install the necessary Python library for making HTTP requests:
pip install requests
Creating a Contact Using HubSpot API with Python
To create a contact in HubSpot, you'll make a POST request to the HubSpot API. Here's a step-by-step guide:
import requests
# Set the API endpoint
url = "https://api.hubapi.com/crm/v3/objects/contacts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the contact information
contact = {
"properties": {
"email": "example@hubspot.com",
"firstname": "John",
"lastname": "Doe"
}
}
# Send the POST request
response = requests.post(url, json=contact, headers=headers)
# Check the response status
if response.status_code == 201:
print("Contact Created Successfully")
else:
print("Failed to Create Contact:", response.json())
Replace Your_Token
with your OAuth token. This code sends a POST request to create a new contact with the specified properties. If successful, it prints a confirmation message.
Updating a Contact Using HubSpot API with Python
To update an existing contact, you'll make a PATCH request. Here's how:
import requests
# Set the API endpoint with the contact ID
contact_id = "123456789"
url = f"https://api.hubapi.com/crm/v3/objects/contacts/{contact_id}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the updated contact information
updated_contact = {
"properties": {
"jobtitle": "Senior Developer"
}
}
# Send the PATCH request
response = requests.patch(url, json=updated_contact, headers=headers)
# Check the response status
if response.status_code == 200:
print("Contact Updated Successfully")
else:
print("Failed to Update Contact:", response.json())
Replace Your_Token
and contact_id
with your OAuth token and the contact's ID, respectively. This code updates the contact's job title and confirms the update upon success.
Handling API Response and Errors
It's crucial to handle API responses and errors effectively. Check the response status code to verify success or identify issues. Common error codes include:
- 401 Unauthorized: Check your OAuth token.
- 404 Not Found: Verify the contact ID.
- 429 Too Many Requests: You've hit the rate limit. Refer to the HubSpot API Usage Guidelines for rate limit details.
For more detailed error handling, refer to the HubSpot Contacts API Documentation.
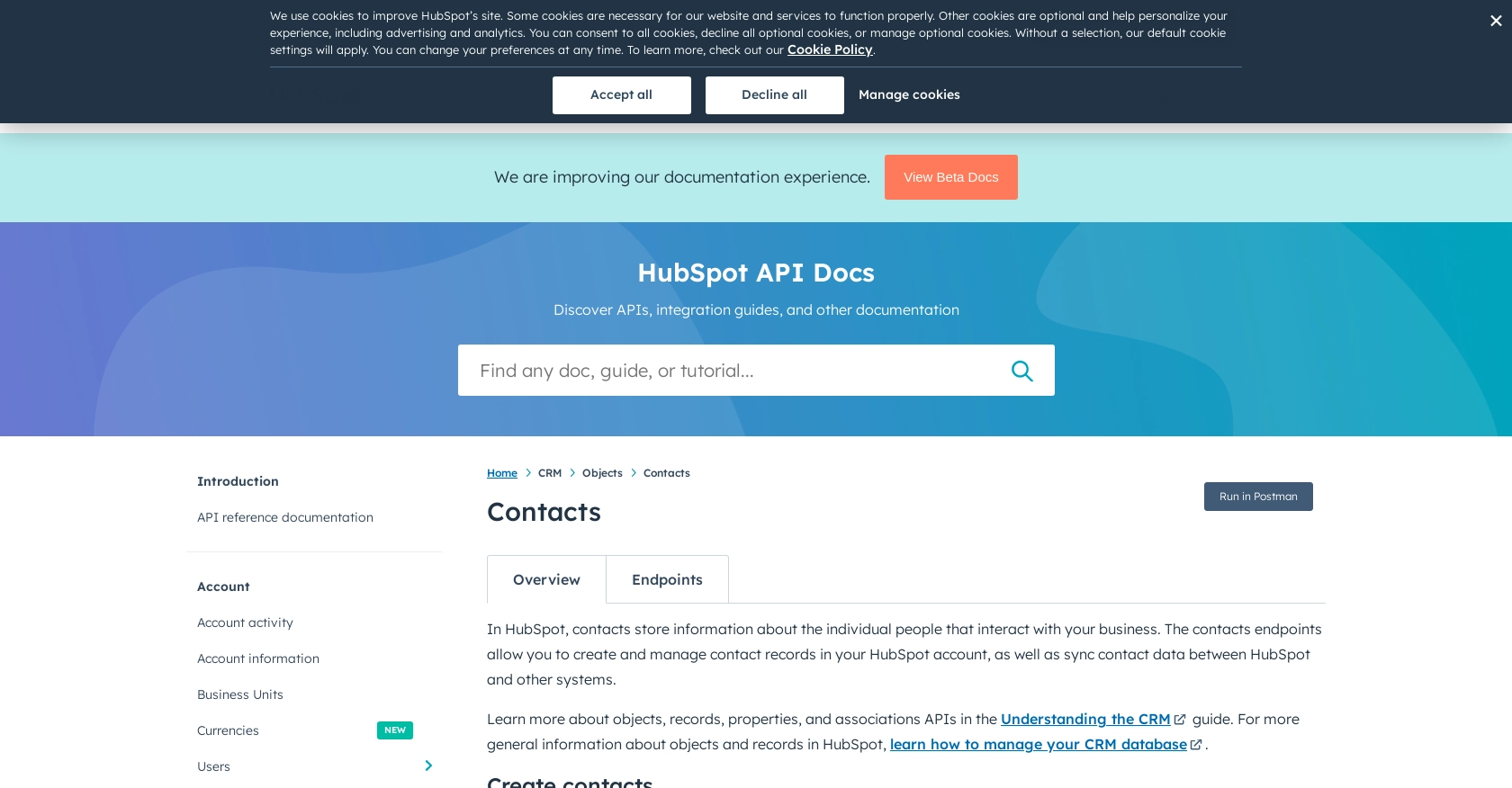
Conclusion and Best Practices for HubSpot API Integration
Integrating with the HubSpot API using Python provides a powerful way to manage and automate your CRM data. By following the steps outlined in this guide, you can efficiently create and update contact information, ensuring your business maintains accurate and up-to-date records.
Best Practices for Secure and Efficient HubSpot API Usage
- Securely Store OAuth Tokens: Always store your OAuth tokens securely and refresh them periodically to maintain uninterrupted access.
- Handle Rate Limits: Be mindful of HubSpot's rate limits, which are 100 requests every 10 seconds for OAuth apps. Implement throttling in your application to avoid hitting these limits. For more details, refer to the HubSpot API Usage Guidelines.
- Standardize Data Fields: Ensure consistency in data fields to avoid discrepancies and maintain data integrity across your applications.
- Error Handling: Implement robust error handling to manage API response codes effectively, ensuring your application can gracefully handle issues like authentication errors or rate limit breaches.
Streamline Your Integration Process with Endgrate
While integrating with HubSpot's API can significantly enhance your CRM capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case, rather than multiple times for different platforms.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/contacts
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?