How to Create Or Update Contacts with the Front API in Python
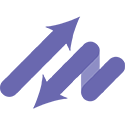
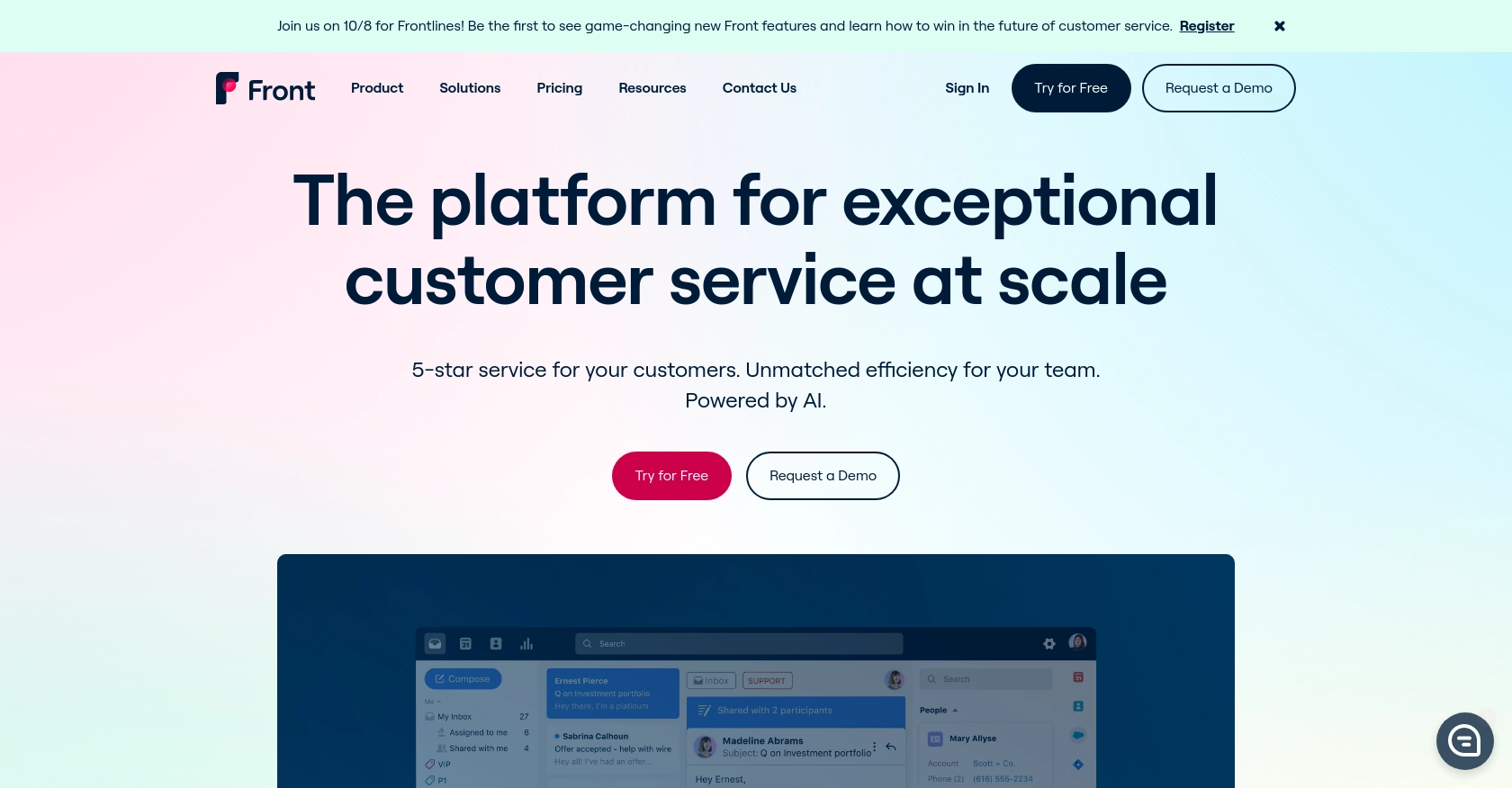
Introduction to Front API for Contact Management
Front is a powerful communication platform that centralizes customer interactions across various channels, including email, social media, and SMS. It offers a robust API that allows developers to seamlessly integrate and manage contact data, enhancing customer relationship management and streamlining communication workflows.
Integrating with Front's API is essential for developers looking to automate and optimize contact management processes. For example, you can create or update contact information directly from your CRM system into Front, ensuring that your team always has access to the most up-to-date customer data.
Setting Up Your Front Developer Account for API Integration
Before you can start creating or updating contacts using the Front API, you need to set up a developer account. This account will provide you with access to the necessary tools and environment to test your integration without affecting production data.
Creating a Front Developer Account
If you don't already have a Front account, follow these steps to create a developer account:
- Visit the Front Developer Portal.
- Sign up for a free developer account by clicking on the "Sign Up" button.
- Fill in the required information and complete the registration process.
- Once registered, log in to your developer account to access the sandbox environment.
This developer account will allow you to test your API calls and ensure everything works as expected before deploying to a live environment.
Generating an API Token for Authentication
The Front API uses API tokens for authentication. Follow these steps to generate your API token:
- Log in to your Front developer account.
- Navigate to the "API Tokens" section in the dashboard.
- Click on "Create API Token" and provide a name for your token.
- Select the appropriate scopes for your token, such as "Shared Resources" to access contact data.
- Click "Create" to generate your token. Make sure to copy and store it securely, as you will need it for API requests.
With your API token ready, you can now authenticate your requests to the Front API and begin integrating contact management features into your application.
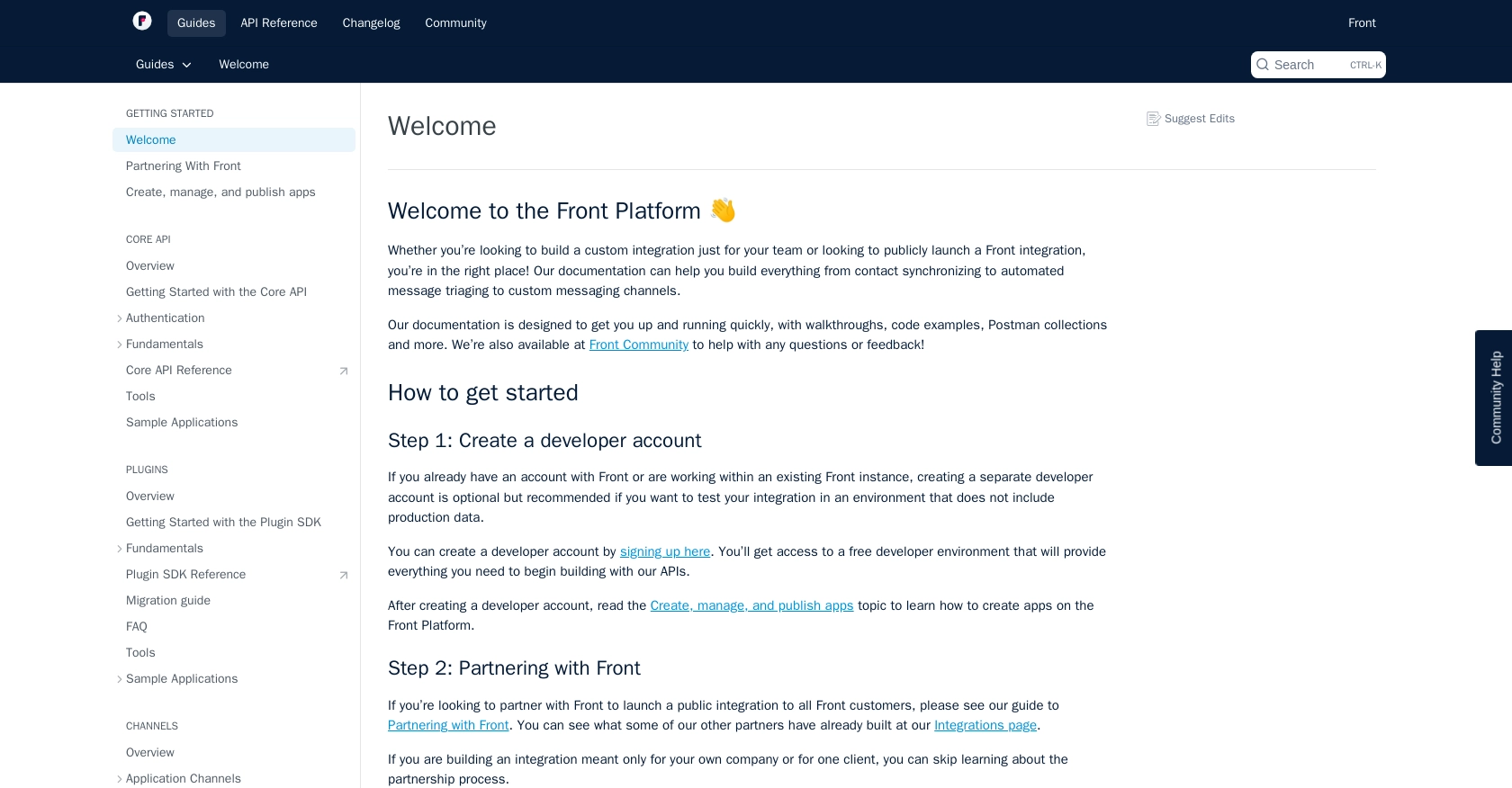
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with Front API in Python
To interact with the Front API for creating or updating contacts, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Python Environment for Front API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You will also need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Open your terminal or command prompt and install the
requests
library using pip:
pip install requests
Creating a Contact with Front API
To create a contact, you'll use the Front API's POST
method. Here's a step-by-step guide:
- Create a new Python file named
create_contact.py
. - Add the following code to the file:
import requests # Set the API endpoint and headers url = "https://api2.frontapp.com/contacts" headers = { "Authorization": "Bearer YOUR_API_TOKEN", "Content-Type": "application/json" } # Define the contact data contact_data = { "name": "John Doe", "description": "Sample contact for demonstration purposes", "handles": [{"source": "email", "handle": "john.doe@example.com"}] } # Make the POST request to create the contact response = requests.post(url, json=contact_data, headers=headers) # Check the response status if response.status_code == 201: print("Contact created successfully:", response.json()) else: print("Failed to create contact:", response.status_code, response.text)
Replace YOUR_API_TOKEN
with the API token you generated earlier. Run the script using the command:
python create_contact.py
If successful, you should see a confirmation message with the contact details.
Updating a Contact with Front API
To update an existing contact, use the PATCH
method. Follow these steps:
- Create a new Python file named
update_contact.py
. - Add the following code to the file:
import requests # Set the API endpoint and headers contact_id = "CONTACT_ID" # Replace with the actual contact ID url = f"https://api2.frontapp.com/contacts/{contact_id}" headers = { "Authorization": "Bearer YOUR_API_TOKEN", "Content-Type": "application/json" } # Define the updated contact data update_data = { "description": "Updated description for the contact" } # Make the PATCH request to update the contact response = requests.patch(url, json=update_data, headers=headers) # Check the response status if response.status_code == 204: print("Contact updated successfully.") else: print("Failed to update contact:", response.status_code, response.text)
Replace YOUR_API_TOKEN
and CONTACT_ID
with your API token and the contact ID you wish to update. Run the script using the command:
python update_contact.py
A successful update will return a status code of 204 with no content.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors. The Front API may return various status codes indicating success or failure. Here are some common ones:
- 201 Created: The contact was successfully created.
- 204 No Content: The contact was successfully updated.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Authentication failed, check your API token.
- 429 Too Many Requests: Rate limit exceeded. Retry after the specified time.
Always check the response status and handle errors appropriately to ensure robust integration.
For more details on rate limits, refer to the Front API rate limiting documentation.
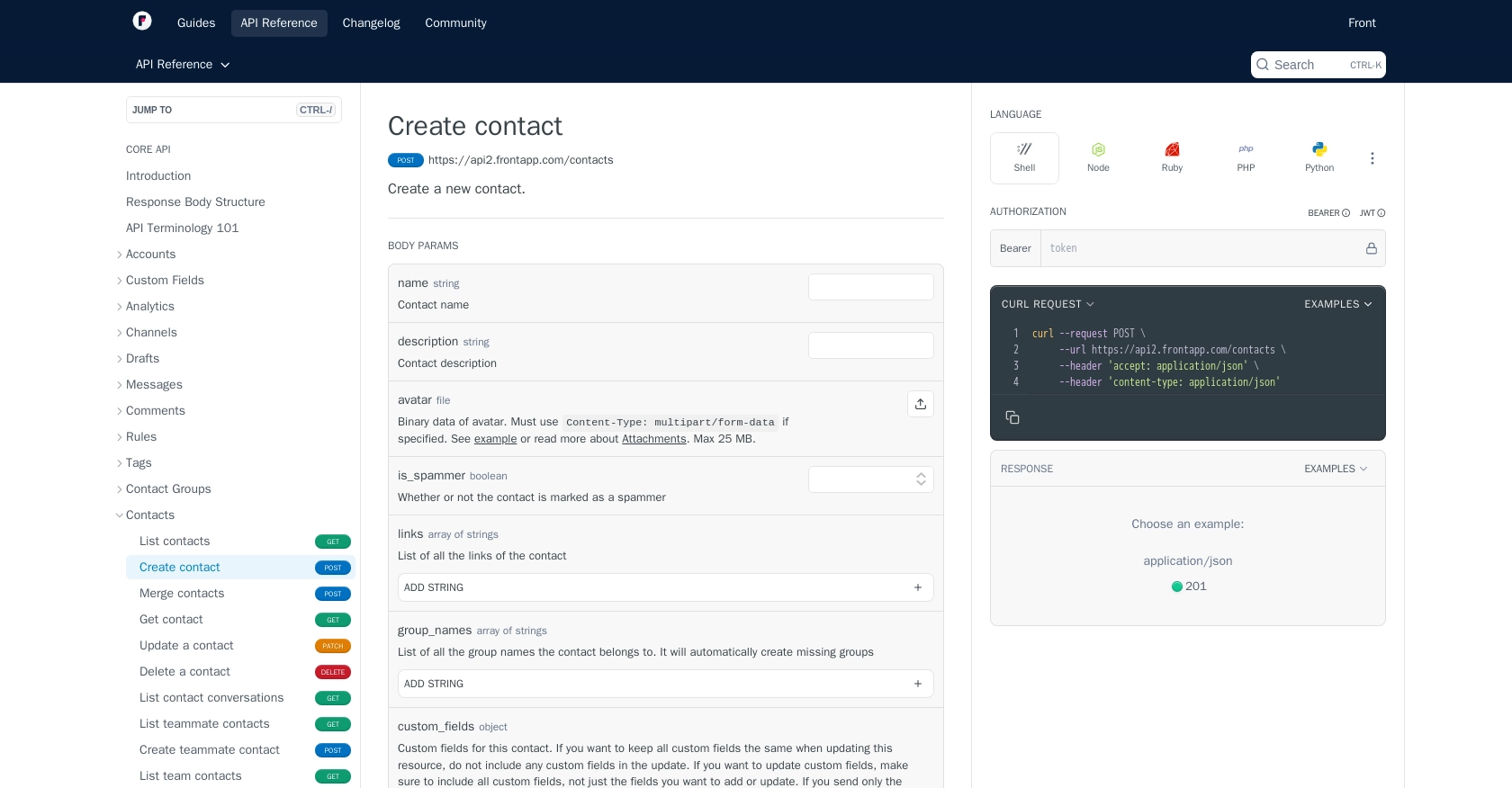
Conclusion and Best Practices for Using Front API in Python
Integrating with the Front API to manage contacts can significantly enhance your team's ability to maintain up-to-date customer information and streamline communication workflows. By following the steps outlined in this guide, you can efficiently create and update contacts using Python, ensuring seamless synchronization with your CRM systems.
Best Practices for Secure and Efficient API Integration with Front
- Securely Store API Tokens: Always store your API tokens securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of Front's API rate limits, which start at 50 requests per minute. Implement logic to handle the
429 Too Many Requests
status code and use theRetry-After
header to determine when to retry requests. For more information, refer to the rate limiting documentation. - Implement Error Handling: Always check the response status codes and handle errors gracefully. This ensures that your application can recover from unexpected issues without disrupting user experience.
- Optimize Data Synchronization: Regularly update and synchronize contact data to maintain consistency across platforms. Consider using webhooks for real-time updates if supported by Front.
Enhance Your Integration Capabilities with Endgrate
While integrating with Front API directly offers powerful capabilities, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Front. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management and focusing on your core product development.
- Build once for each use case, reducing the need for multiple integrations and minimizing maintenance efforts.
- Offer an intuitive integration experience for your customers, enhancing their interaction with your product.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website and discover the benefits of a unified integration solution.
Read More
- https://endgrate.com/provider/front
- https://dev.frontapp.com/docs/welcome
- https://dev.frontapp.com/docs/core-api-getting-started
- https://dev.frontapp.com/docs/oauth
- https://dev.frontapp.com/docs/rate-limiting
- https://dev.frontapp.com/docs/pagination
- https://dev.frontapp.com/reference/create-contact
- https://dev.frontapp.com/reference/update-a-contact
Ready to get started?