How to Get Applicants with the JazzHR API in PHP
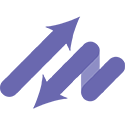
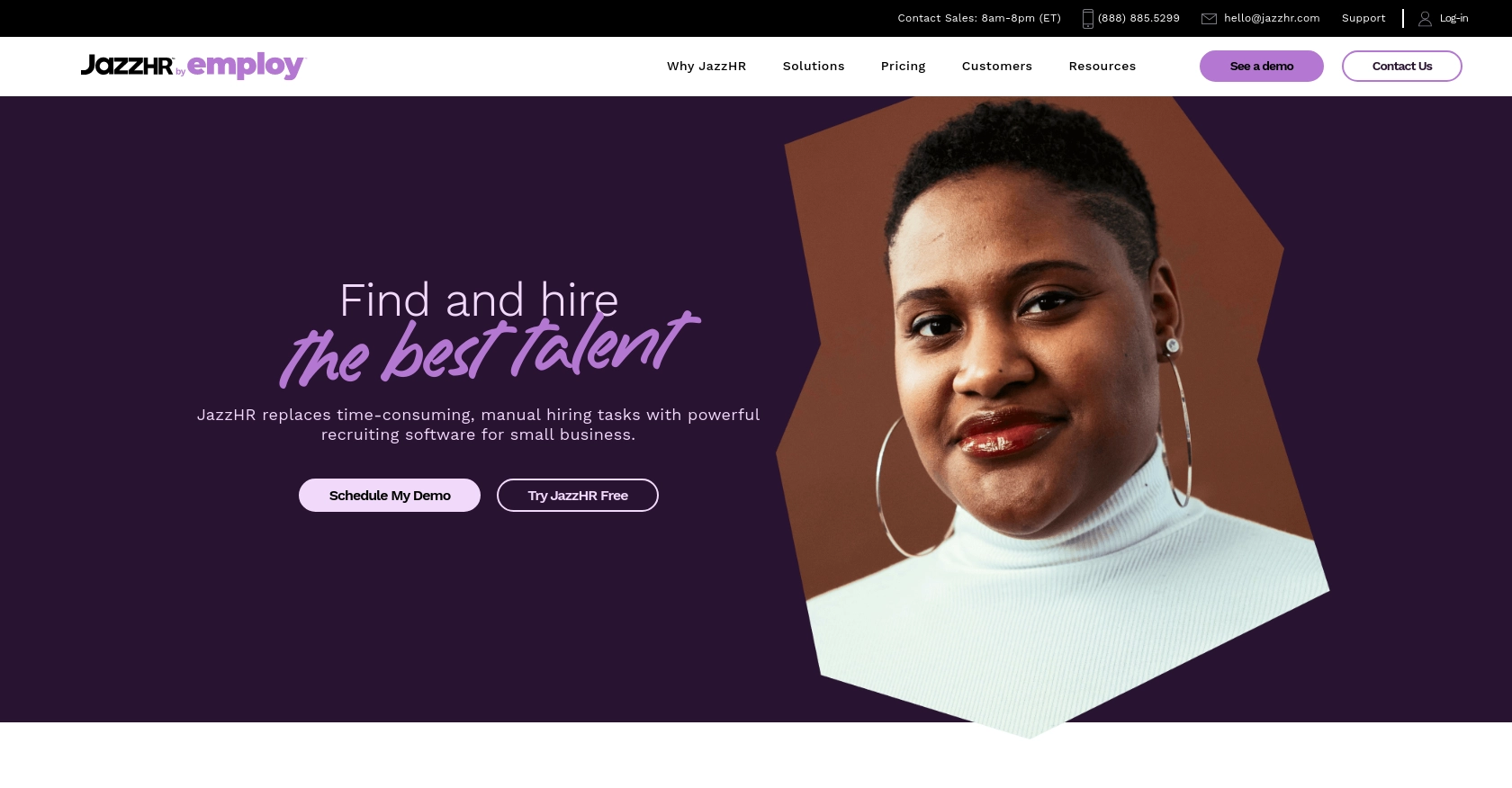
Introduction to JazzHR
JazzHR is a powerful recruiting software designed to simplify the hiring process for businesses of all sizes. It offers a comprehensive suite of tools to help companies manage job postings, track applicants, and streamline the recruitment workflow.
Developers may want to integrate with JazzHR's API to automate and enhance their recruitment processes. For example, by accessing applicant data through the JazzHR API, a developer can create custom dashboards or reports that provide insights into the hiring pipeline, improving decision-making and efficiency.
Setting Up Your JazzHR Account for API Access
Before you can start interacting with the JazzHR API, you'll need to set up your JazzHR account and obtain the necessary API key. This key will allow you to authenticate your requests and access applicant data.
Creating a JazzHR Account
If you don't already have a JazzHR account, you can sign up for a free trial or a demo account on the JazzHR website. Follow the instructions to complete the registration process. Once your account is created, log in to access the dashboard.
Generating Your JazzHR API Key
To interact with the JazzHR API, you'll need an API key. Follow these steps to generate your API key:
- Log in to your JazzHR account.
- Navigate to the Integrations section in the account settings.
- Locate the API key section and generate a new API key if one is not already available.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed information, you can refer to the JazzHR API Overview.
Understanding API Rate Limits
JazzHR imposes a limit of 100 results per page for API calls. If you need to retrieve more results, you can paginate through the data by appending /page/#
to the end of the request URI, where #
is the page number. This ensures efficient data retrieval without overwhelming the server.
For further details, you can explore the JazzHR API Documentation.
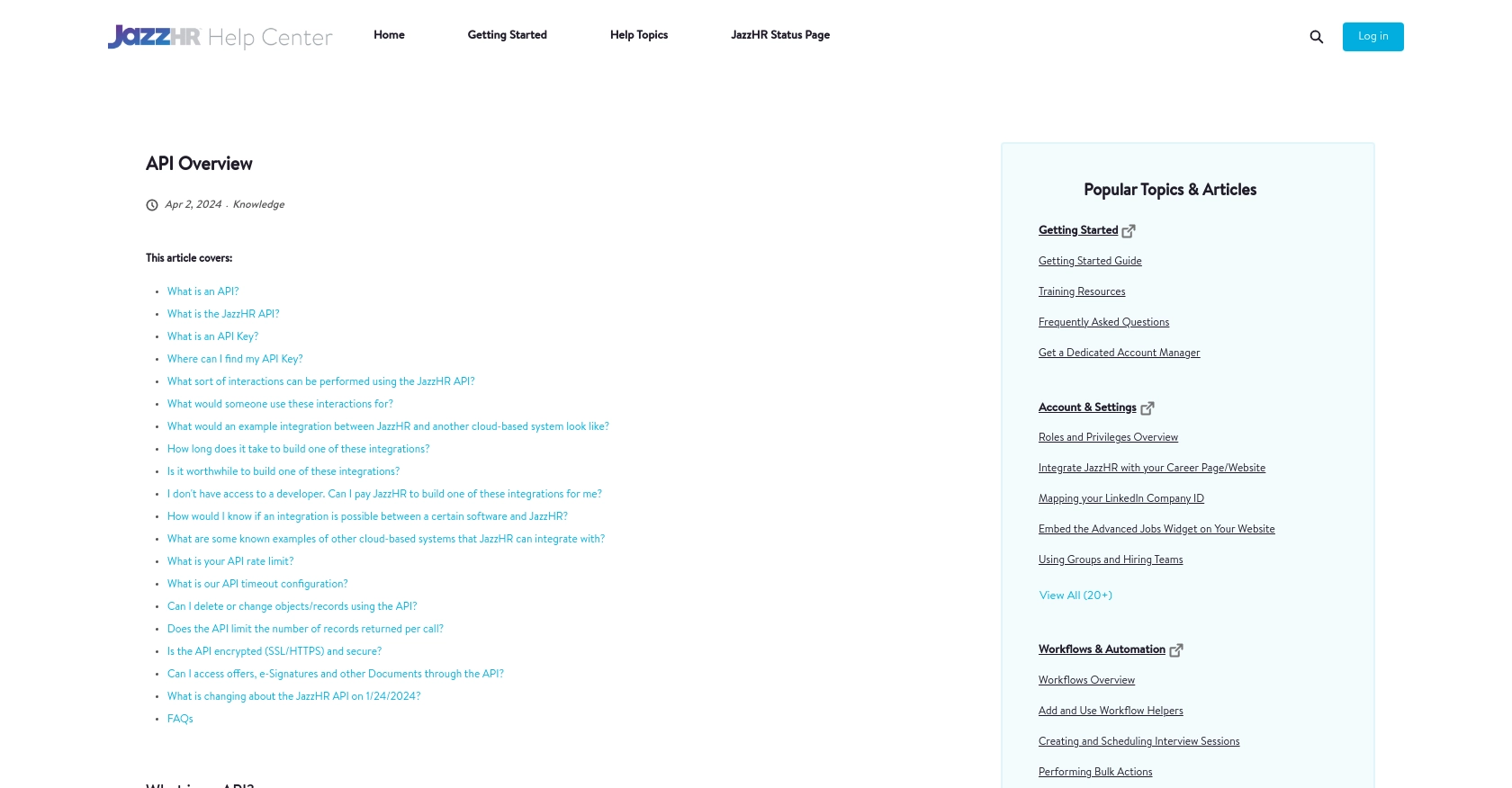
sbb-itb-96038d7
Making API Calls to Retrieve Applicants from JazzHR Using PHP
To interact with the JazzHR API and retrieve applicant data, you'll need to use PHP to make HTTP requests. This section will guide you through setting up your PHP environment, installing necessary dependencies, and executing the API call to fetch applicants.
Setting Up Your PHP Environment for JazzHR API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, open your terminal and install the guzzlehttp/guzzle
library, which simplifies HTTP requests in PHP:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Applicants from JazzHR
Create a new PHP file named get_applicants.php
and add the following code to it:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle HTTP client
$client = new Client();
// Set the API endpoint and your API key
$endpoint = 'https://api.resumatorapi.com/v1/applicants';
$apiKey = 'Your_API_Key';
// Make a GET request to the JazzHR API
$response = $client->request('GET', $endpoint, [
'query' => ['apikey' => $apiKey]
]);
// Parse the JSON response
$data = json_decode($response->getBody(), true);
// Loop through the applicants and print their information
foreach ($data as $applicant) {
echo 'Name: ' . $applicant['first_name'] . ' ' . $applicant['last_name'] . "\n";
echo 'Email: ' . $applicant['email'] . "\n\n";
}
?>
Replace Your_API_Key
with the API key you obtained from your JazzHR account.
Running the PHP Script to Retrieve JazzHR Applicants
Execute the script from the terminal using the following command:
php get_applicants.php
You should see a list of applicants with their names and email addresses printed in the terminal.
Handling Errors and Verifying API Call Success
To ensure your API call is successful, check the HTTP status code of the response. A status code of 200
indicates success. You can modify the script to handle errors as follows:
if ($response->getStatusCode() === 200) {
// Success logic
} else {
echo 'Failed to retrieve applicants. Status code: ' . $response->getStatusCode();
}
For more information on error codes, refer to the JazzHR API Overview.
Conclusion and Best Practices for Using JazzHR API with PHP
Integrating with the JazzHR API using PHP can significantly enhance your recruitment processes by automating data retrieval and management. By following the steps outlined in this guide, you can efficiently access applicant data and leverage it to improve your hiring workflow.
Best Practices for Secure and Efficient JazzHR API Integration
- Securely Store API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of JazzHR's rate limits, which allow 100 results per page. Implement pagination to manage large datasets efficiently.
- Error Handling: Implement robust error handling to manage API call failures gracefully. Check HTTP status codes and handle exceptions appropriately.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications and systems.
Streamline Your Integrations with Endgrate
While integrating with JazzHR's API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including JazzHR.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can manage multiple integrations seamlessly, providing an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?