How to Create or Update Contacts with the Nimble API in Python
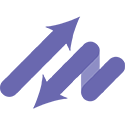
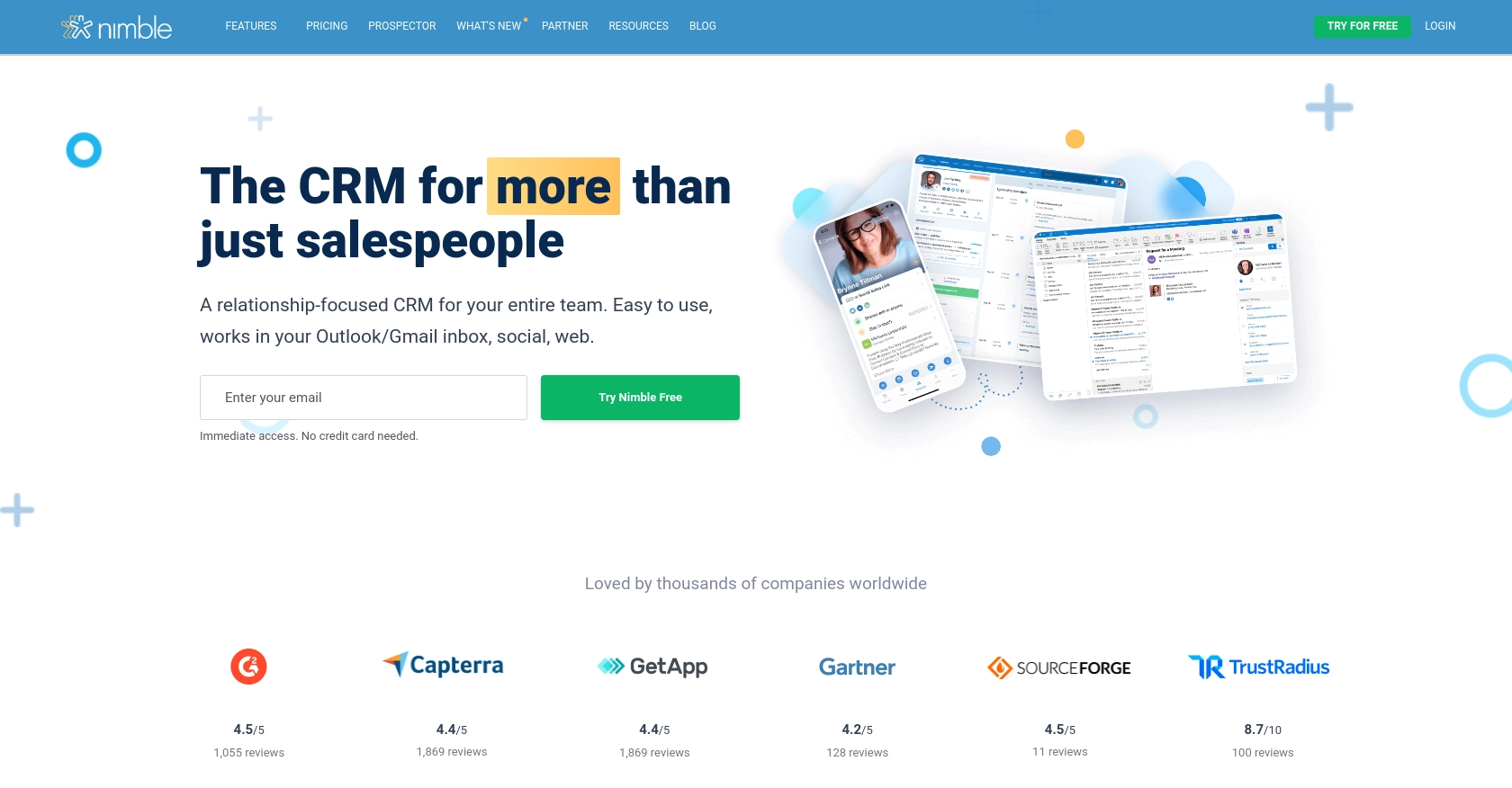
Introduction to Nimble CRM API
Nimble is a relationship-focused CRM platform that integrates seamlessly with Microsoft 365 and Google Workspace, offering businesses a streamlined way to manage contacts, leads, and deals. Its user-friendly interface and powerful automation tools make it an ideal choice for businesses looking to enhance their customer relationship management.
Developers may wish to integrate with the Nimble API to automate and manage contact data efficiently. For example, using the Nimble API, a developer can create or update contact information directly from an external application, ensuring that the CRM data is always up-to-date and accurate.
This article will guide you through the process of creating or updating contacts using the Nimble API with Python, providing step-by-step instructions and code examples to help you get started quickly.
Setting Up Your Nimble Test Account for API Integration
Before you can start creating or updating contacts using the Nimble API, you'll need to set up a Nimble account and obtain an API key. This section will guide you through the necessary steps to get your test environment ready.
Create a Nimble Account
If you don't already have a Nimble account, you can sign up for a free trial on the Nimble website. This will give you access to the CRM platform and allow you to explore its features.
- Visit the Nimble website and click on "Start Free Trial."
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Nimble dashboard.
Generate an API Key for Nimble API Access
To interact with the Nimble API, you'll need an API key. This key will authenticate your requests and allow you to manage contacts programmatically.
- Navigate to the "Settings" section in your Nimble dashboard.
- Under "API Token," select "Generate New Token."
- Provide a description for your token and click "Generate."
- Copy the generated API key and store it securely, as you'll need it for API requests.
Note: Only Nimble Account Administrators can generate API keys. Ensure you have the necessary permissions or contact your account admin for assistance.
Grant API Access Permissions
Ensure that API access is granted by the admin of your Nimble account. Admins can manage API access permissions in the "Users" section under "Settings."
With your Nimble account set up and API key generated, you're ready to start integrating with the Nimble API using Python. In the next section, we'll cover how to make API calls to create or update contacts.
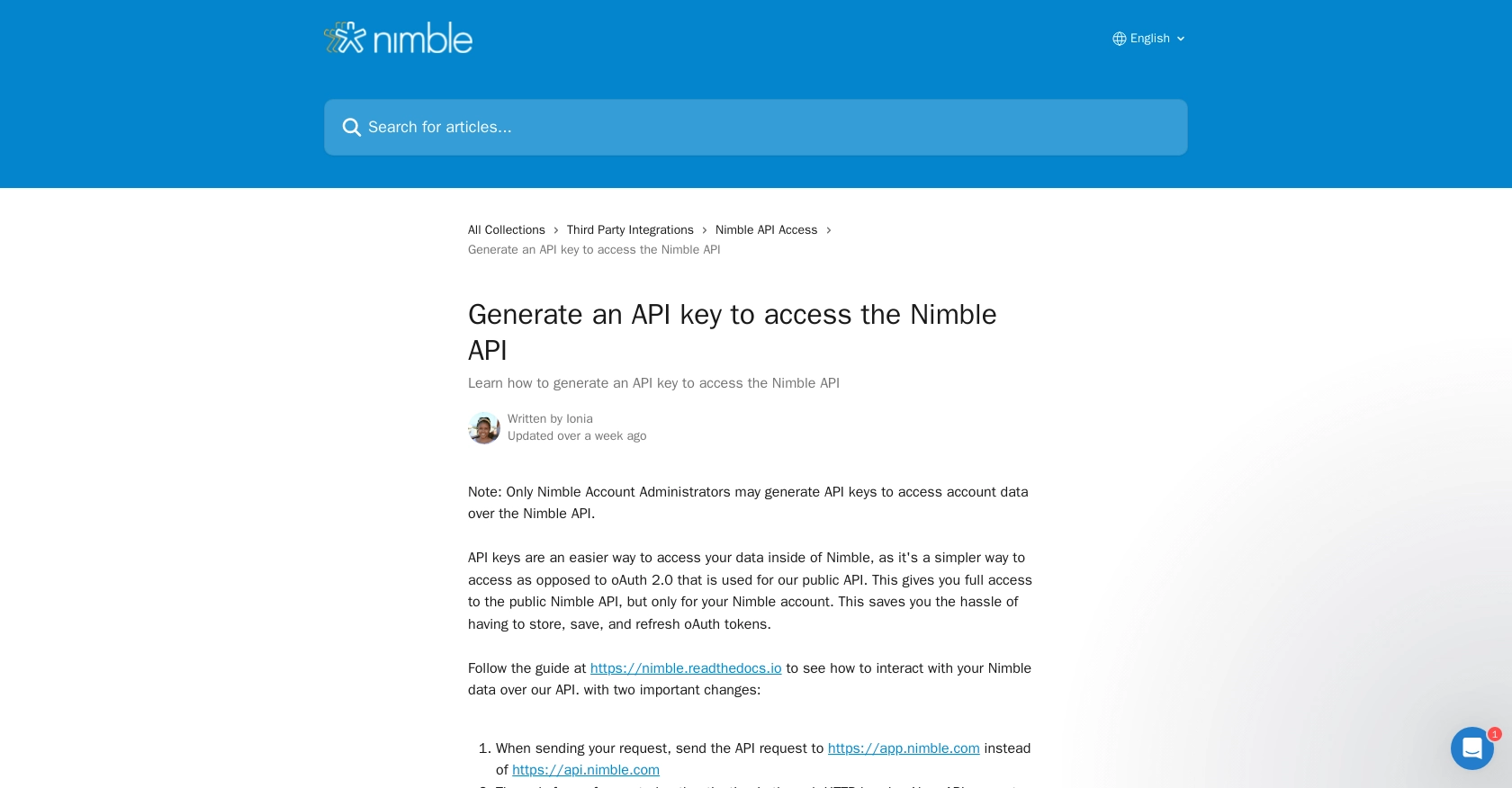
sbb-itb-96038d7
How to Make API Calls to Create or Update Contacts with Nimble API in Python
To interact with the Nimble API using Python, you'll need to ensure you have the right environment set up. This section will guide you through the necessary steps to make API calls for creating or updating contacts in Nimble.
Setting Up Python Environment for Nimble API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website.
- Use the following command to install the
requests
library:
pip install requests
Creating a Contact with Nimble API Using Python
To create a new contact in Nimble, you'll need to make a POST request to the appropriate endpoint. Here's a step-by-step guide:
import requests
# Set the API endpoint
url = "https://app.nimble.com/api/v1/contact"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_KEY"
}
# Define the contact data
contact_data = {
"fields": {
"first name": [{"value": "John"}],
"last name": [{"value": "Doe"}],
"email": [{"value": "john.doe@example.com"}]
},
"record_type": "person"
}
# Make the POST request
response = requests.post(url, json=contact_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Contact created successfully:", response.json())
else:
print("Failed to create contact:", response.status_code, response.json())
Replace YOUR_API_KEY
with the API key you generated earlier. The code above sends a POST request to create a new contact with the specified details. If successful, it prints the contact information.
Updating a Contact with Nimble API Using Python
To update an existing contact, you'll need to make a PUT request to the specific contact's endpoint. Here's how you can do it:
import requests
# Set the API endpoint with contact ID
contact_id = "CONTACT_ID"
url = f"https://app.nimble.com/api/v1/contact/{contact_id}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_KEY"
}
# Define the updated contact data
updated_data = {
"fields": {
"email": [{"value": "new.email@example.com"}]
}
}
# Make the PUT request
response = requests.put(url, json=updated_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Contact updated successfully:", response.json())
else:
print("Failed to update contact:", response.status_code, response.json())
Replace CONTACT_ID
with the ID of the contact you wish to update. This code updates the contact's email address. Ensure you handle errors by checking the response status code and message.
Verifying API Call Success in Nimble Dashboard
After making API calls, verify the changes in your Nimble dashboard. Newly created or updated contacts should reflect in the contact list. If you encounter errors, refer to the error codes provided in the response for troubleshooting.
By following these steps, you can efficiently manage contacts in Nimble using Python. For more information on handling errors and rate limits, refer to the Nimble API documentation.
Conclusion and Best Practices for Nimble API Integration
Integrating with the Nimble API using Python provides a powerful way to automate and manage your CRM data. By following the steps outlined in this guide, you can efficiently create and update contacts, ensuring your data remains accurate and up-to-date.
Best Practices for Secure and Efficient Nimble API Usage
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Nimble's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure consistent data formats when creating or updating contacts. This helps maintain data integrity across your CRM system.
- Error Handling: Implement robust error handling by checking response status codes and messages. Refer to the Nimble API documentation for detailed error code explanations.
Streamline Your Integrations with Endgrate
While integrating with Nimble API can enhance your CRM capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with various platforms, including Nimble. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?