How to Get Companies with the Affinity API in Python
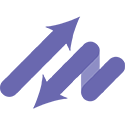
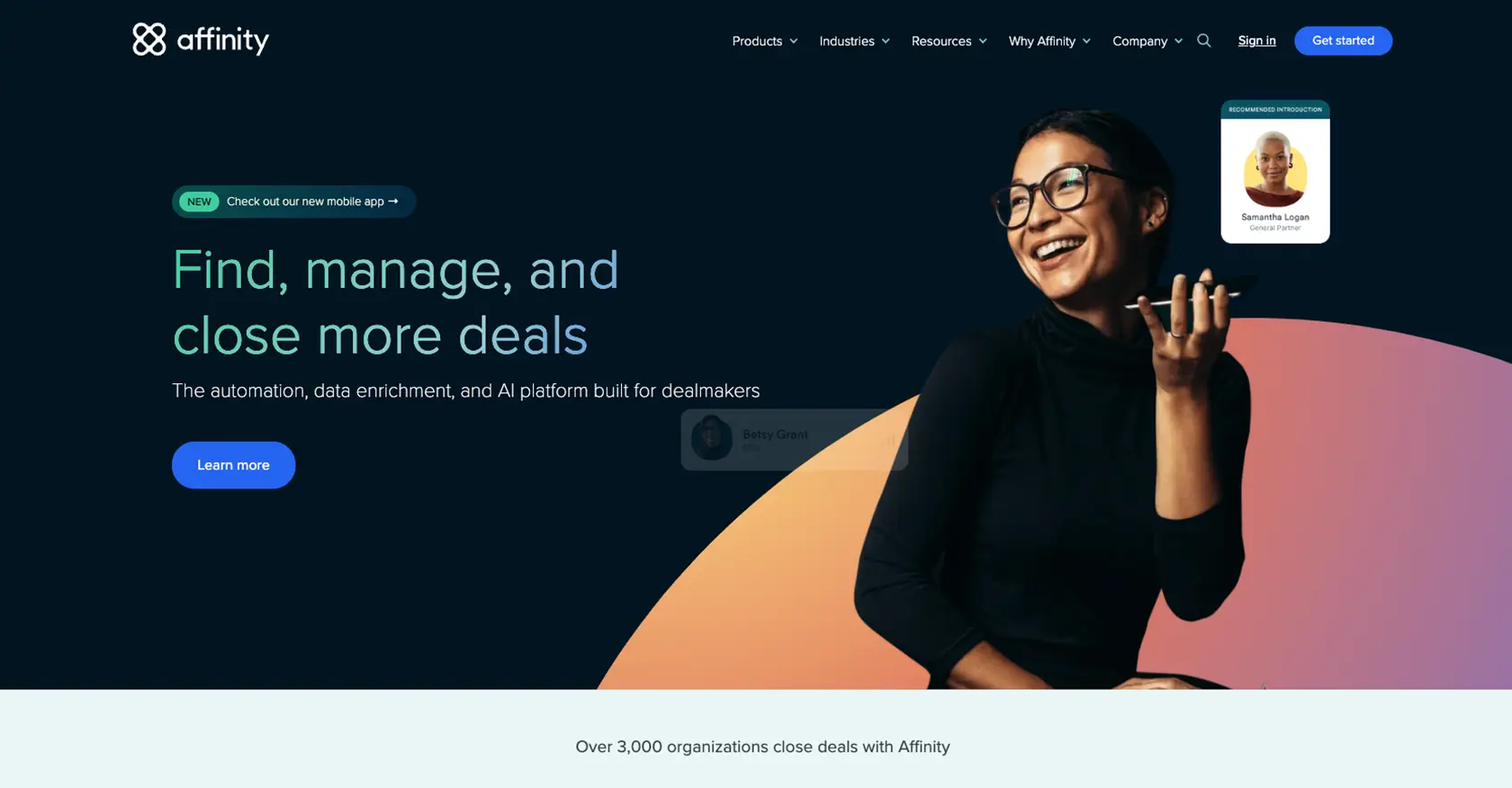
Introduction to Affinity API for Company Data Integration
Affinity is a powerful relationship intelligence platform designed to help businesses manage and leverage their professional networks. By providing insights into connections and interactions, Affinity enables organizations to build stronger relationships and drive growth.
Developers may want to integrate with Affinity's API to access and manage company data efficiently. For example, using the Affinity API, a developer can retrieve detailed information about organizations, helping sales teams to better understand potential clients and tailor their outreach strategies.
This guide will walk you through the process of using Python to interact with the Affinity API, specifically focusing on retrieving company data. By following this tutorial, you'll learn how to set up your environment, authenticate requests, and handle data effectively.
Setting Up Your Affinity API Test Account
Before you can start interacting with the Affinity API to retrieve company data, you need to set up a test account. This involves generating an API key, which will allow you to authenticate your requests securely.
Creating an Affinity Account
If you don't already have an Affinity account, you can sign up for one on the Affinity website. Follow the instructions to create your account, and once completed, log in to access the platform.
Generating Your Affinity API Key
To interact with the Affinity API, you'll need an API key. Follow these steps to generate your key:
- Log in to your Affinity account.
- Navigate to the Settings Panel via the left sidebar.
- Locate the API Key section.
- Click on Generate API Key to create a new key.
- Copy the generated API key and store it securely, as you'll need it for authentication in your API requests.
For more detailed instructions, refer to the Affinity API documentation.
Understanding Affinity API Authentication
The Affinity API uses HTTP Basic Auth for authentication. When making API requests, provide your API key as the password. You do not need to include a username. Here's an example of how to structure your request:
import requests
url = "https://api.affinity.co/api_endpoint"
headers = {
"Authorization": "Basic :Your_API_Key"
}
response = requests.get(url, headers=headers)
print(response.json())
Replace Your_API_Key
with the API key you generated earlier. This setup ensures that your requests are authenticated and processed correctly by Affinity.
Testing Your Affinity API Setup
Once your API key is set up, you can test your configuration by making a simple API call to verify that your authentication is working. Use the example code provided above to make a request and check the response. If successful, you'll be ready to proceed with retrieving company data using the Affinity API.
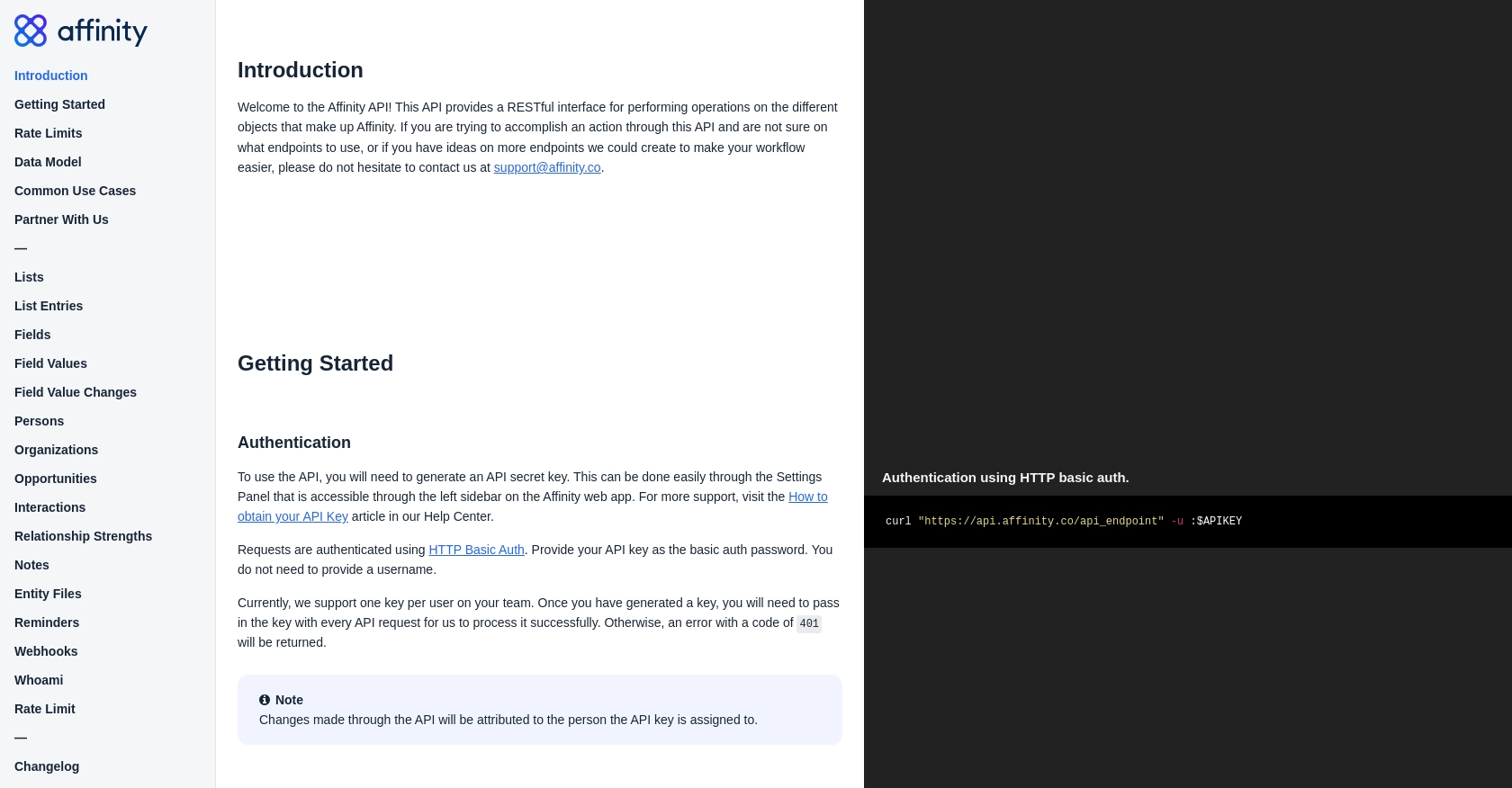
sbb-itb-96038d7
Making API Calls to Retrieve Company Data with Affinity API in Python
To effectively interact with the Affinity API and retrieve company data, you'll need to set up your Python environment and understand how to make API calls. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and executing API requests to fetch company information.
Setting Up Python Environment for Affinity API Integration
Before making API calls, ensure you have Python installed on your machine. This guide uses Python 3.11.1. You can download and install it from the official Python website.
Next, install the required dependencies using pip, the Python package installer. Open your terminal or command prompt and run the following command:
pip install requests
The requests
library is essential for making HTTP requests to the Affinity API.
Executing API Requests to Fetch Company Data from Affinity
With your environment set up, you can now make API calls to retrieve company data. Create a new Python file named get_affinity_companies.py
and add the following code:
import requests
# Define the API endpoint for retrieving companies
url = "https://api.affinity.co/organizations"
# Set up the headers with your API key for authentication
headers = {
"Authorization": "Basic :Your_API_Key"
}
# Make a GET request to the API endpoint
response = requests.get(url, headers=headers)
# Parse the JSON response
data = response.json()
# Print the company data
for company in data.get("organizations", []):
print(f"Company Name: {company['name']}, Domain: {company['domain']}")
Replace Your_API_Key
with the API key you generated earlier. This script sends a GET request to the Affinity API to fetch a list of companies and prints their names and domains.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of companies printed in your terminal. This indicates a successful API call. If you encounter errors, check the response status code and handle them accordingly. Here are some common error codes and their meanings:
- 401 Unauthorized: Your API key is invalid.
- 403 Forbidden: Insufficient rights to access the resource.
- 404 Not Found: The requested resource does not exist.
- 429 Too Many Requests: You have exceeded the rate limit.
For more detailed error handling, refer to the Affinity API documentation.
Conclusion and Best Practices for Using Affinity API in Python
Integrating with the Affinity API allows developers to efficiently access and manage company data, enhancing the ability to build and maintain professional relationships. By following the steps outlined in this guide, you can set up your environment, authenticate requests, and retrieve valuable company information using Python.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handling Rate Limits: Be mindful of the Affinity API's rate limits, which are set at 900 requests per user per minute. Implement retry logic to handle
429 Too Many Requests
errors gracefully. - Data Transformation and Standardization: Consider transforming and standardizing data fields to ensure consistency across different systems and integrations.
Leverage Endgrate for Streamlined Integration Solutions
For developers looking to simplify the integration process further, consider using Endgrate. Endgrate offers a unified API endpoint that connects to multiple platforms, including Affinity, allowing you to build integrations once and deploy them across various services. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?