Using the Confluence API to Get Space Pages in Python
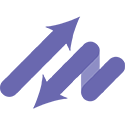
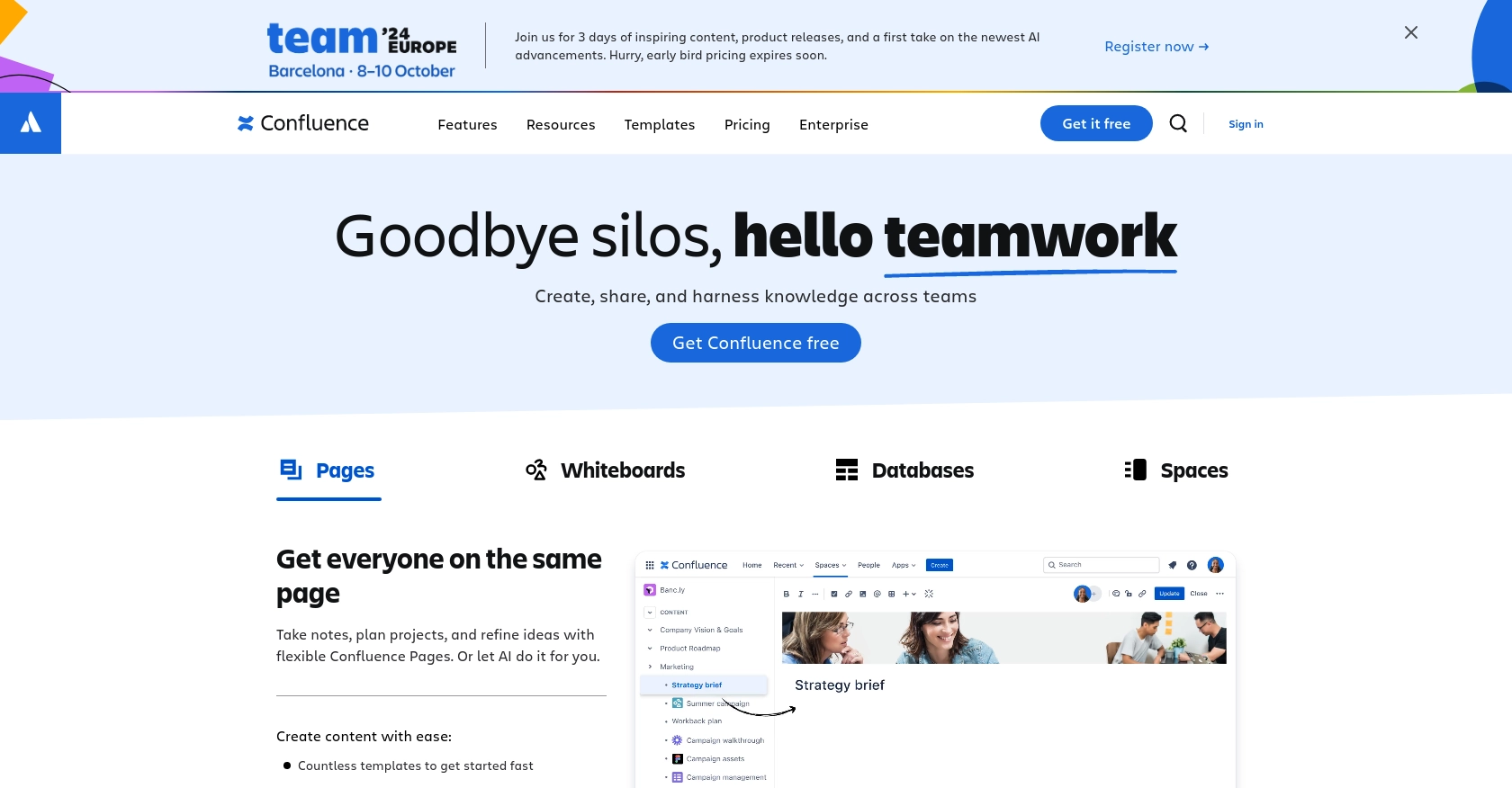
Introduction to Confluence API Integration
Confluence, developed by Atlassian, is a powerful collaboration tool that helps teams to create, share, and manage content seamlessly. It serves as a centralized platform for documentation, project collaboration, and knowledge sharing, making it an essential tool for businesses aiming to enhance productivity and communication.
Integrating with the Confluence API allows developers to automate and streamline content management processes. For example, you might want to retrieve all pages within a specific space to generate reports or synchronize content with other systems. This capability can significantly enhance the efficiency of content management workflows.
Setting Up Your Confluence Test or Sandbox Account
Before you can start interacting with the Confluence API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Confluence Account
If you don't already have a Confluence account, you can sign up for a free trial or use a demo account provided by Atlassian. Visit the Confluence website and follow the instructions to create your account. Once your account is set up, you can log in to access the Confluence dashboard.
Generating OAuth Credentials for Confluence API Access
To authenticate your API requests, you'll need to use OAuth 2.0, which requires you to create an app in your Confluence account. Follow these steps to generate the necessary credentials:
- Navigate to the Settings section in your Confluence dashboard.
- Under Integrations, select OAuth 2.0 to create a new app.
- Fill in the required details, such as the app name and description.
- Specify the necessary scopes for your app. For accessing pages, you will need the
read:page:confluence
scope. - Once your app is created, you will receive a Client ID and Client Secret. Keep these credentials secure as they will be used to authenticate your API requests.
Obtaining Access Tokens for API Requests
With your OAuth credentials ready, you can now obtain access tokens to make API calls. Here's a basic outline of how to get an access token:
- Send a POST request to the Confluence OAuth token endpoint with your Client ID and Client Secret.
- Include the required parameters, such as
grant_type
andscope
. - Upon successful authentication, you will receive an access token, which you can use in the Authorization header of your API requests.
For detailed instructions, refer to the Confluence API documentation.
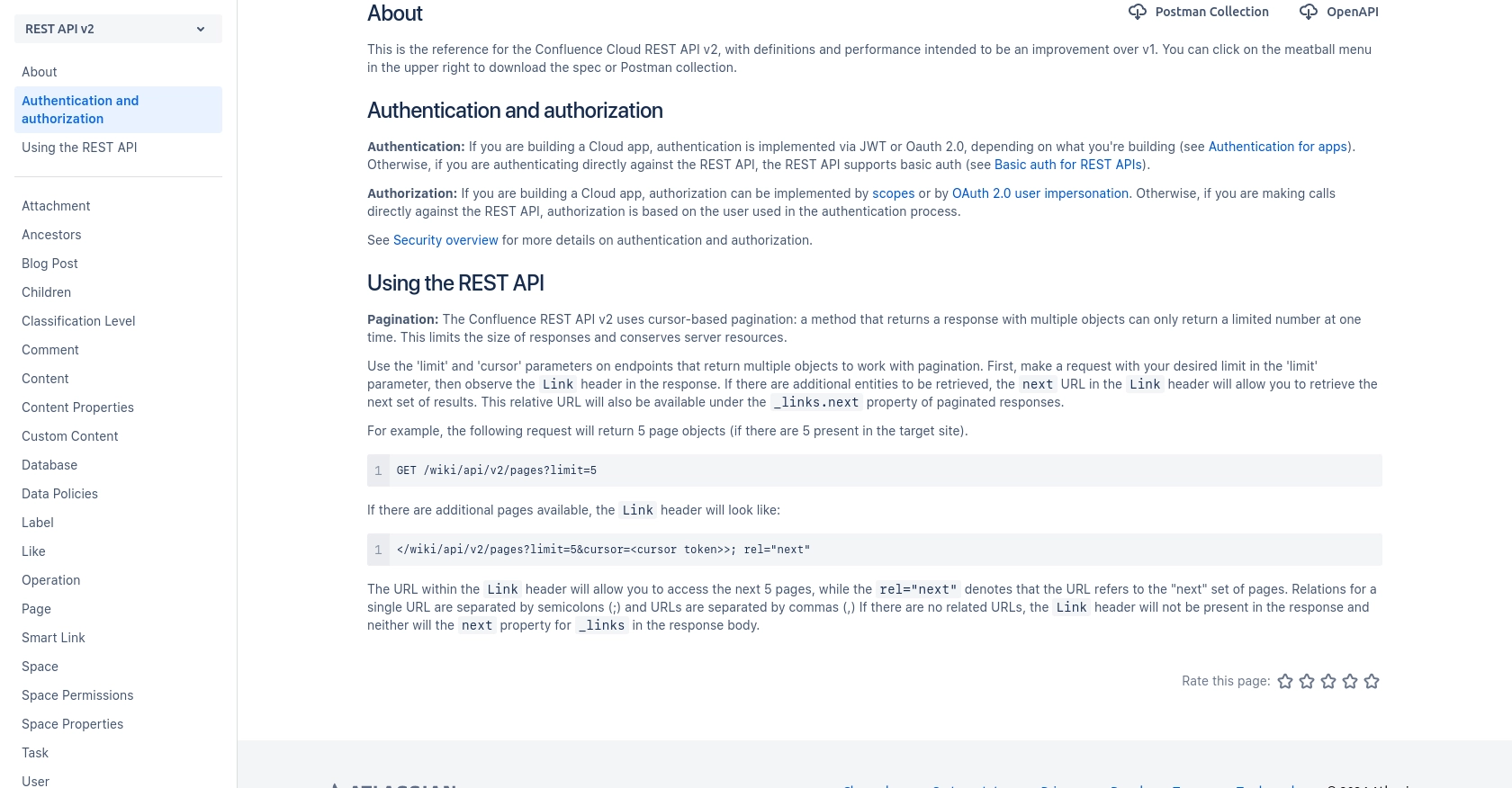
sbb-itb-96038d7
Making API Calls to Retrieve Confluence Space Pages Using Python
To interact with the Confluence API and retrieve pages from a specific space, you'll need to use Python. This section will guide you through setting up your environment, making the API call, and handling the response effectively.
Setting Up Your Python Environment for Confluence API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Writing Python Code to Get Pages from a Confluence Space
Create a new Python file named get_confluence_pages.py
and add the following code to retrieve pages from a specific space:
import requests
# Set the API endpoint and headers
space_id = "your_space_id"
url = f"https://your-domain.atlassian.net/wiki/api/v2/spaces/{space_id}/pages"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for page in data["results"]:
print(f"Page ID: {page['id']}, Title: {page['title']}")
else:
print(f"Failed to retrieve pages: {response.status_code} {response.text}")
Replace your_space_id
with the ID of the space you want to query and YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup.
Understanding the API Response and Handling Errors
The API response will include a JSON object containing the pages within the specified space. The code above iterates through the results and prints the page ID and title. If the request fails, the error message and status code will be displayed.
Common error codes include:
- 400 Bad Request: The request was invalid. Check your parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The specified space or pages do not exist.
For more details on error handling, refer to the Confluence API documentation.
Verifying Successful API Calls in Confluence
After running your script, you should see the list of pages printed in your terminal. You can verify the results by checking the Confluence dashboard to ensure the pages match those retrieved by your script.
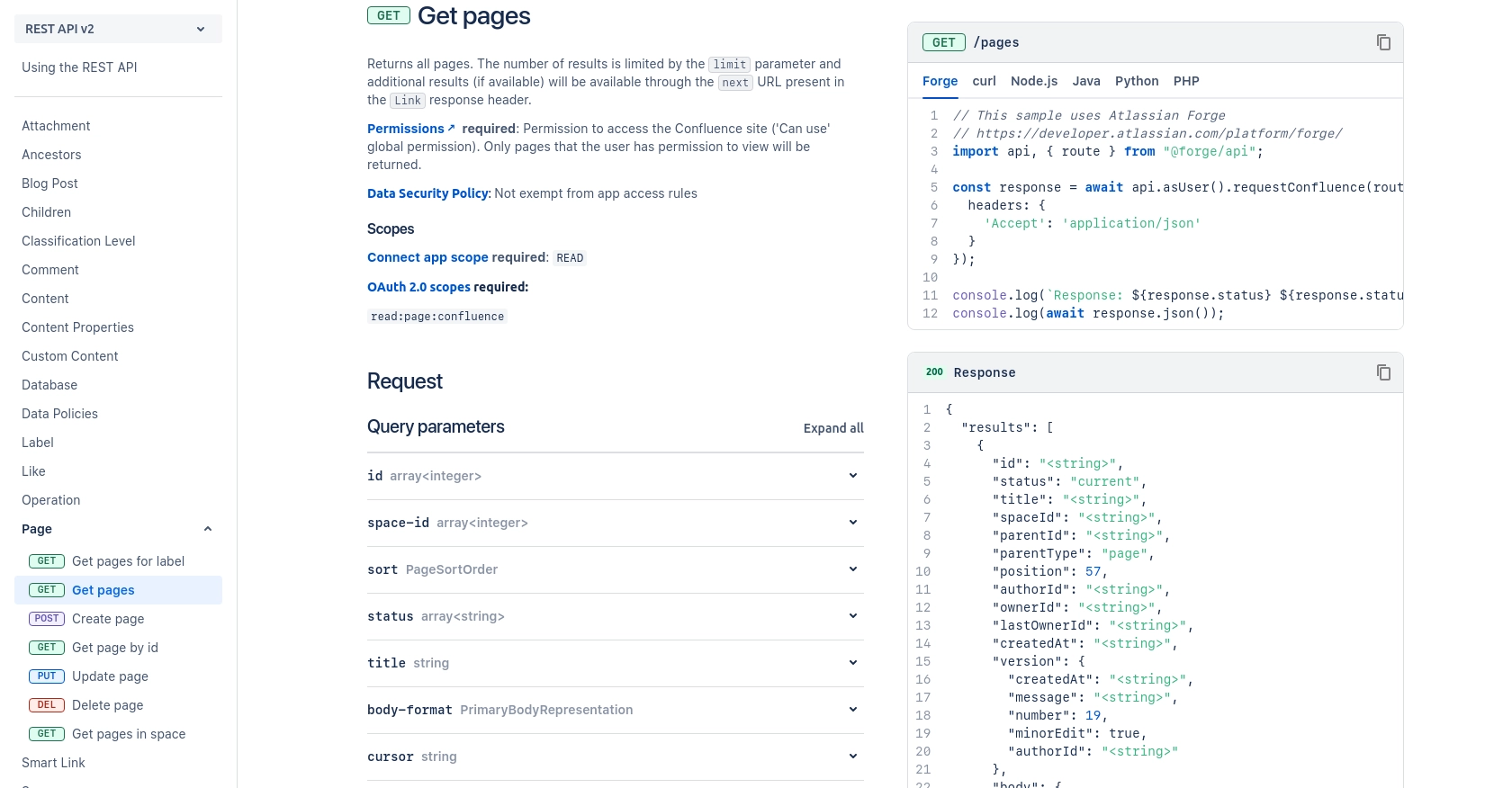
Best Practices for Confluence API Integration
When integrating with the Confluence API, it's crucial to follow best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth credentials and access tokens securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Confluence's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully. For more details, refer to the Confluence API documentation.
- Paginate API Requests: Use cursor-based pagination to efficiently handle large datasets. This ensures that your application remains responsive and does not overwhelm the server.
- Standardize Data Fields: When synchronizing data with other systems, ensure that data fields are standardized to maintain consistency across platforms.
Enhance Your Integration Strategy with Endgrate
Building and maintaining multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Confluence. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration development and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
Ready to get started?