How to Create or Update Records with the Google Sheets API in Python
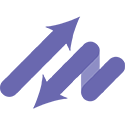
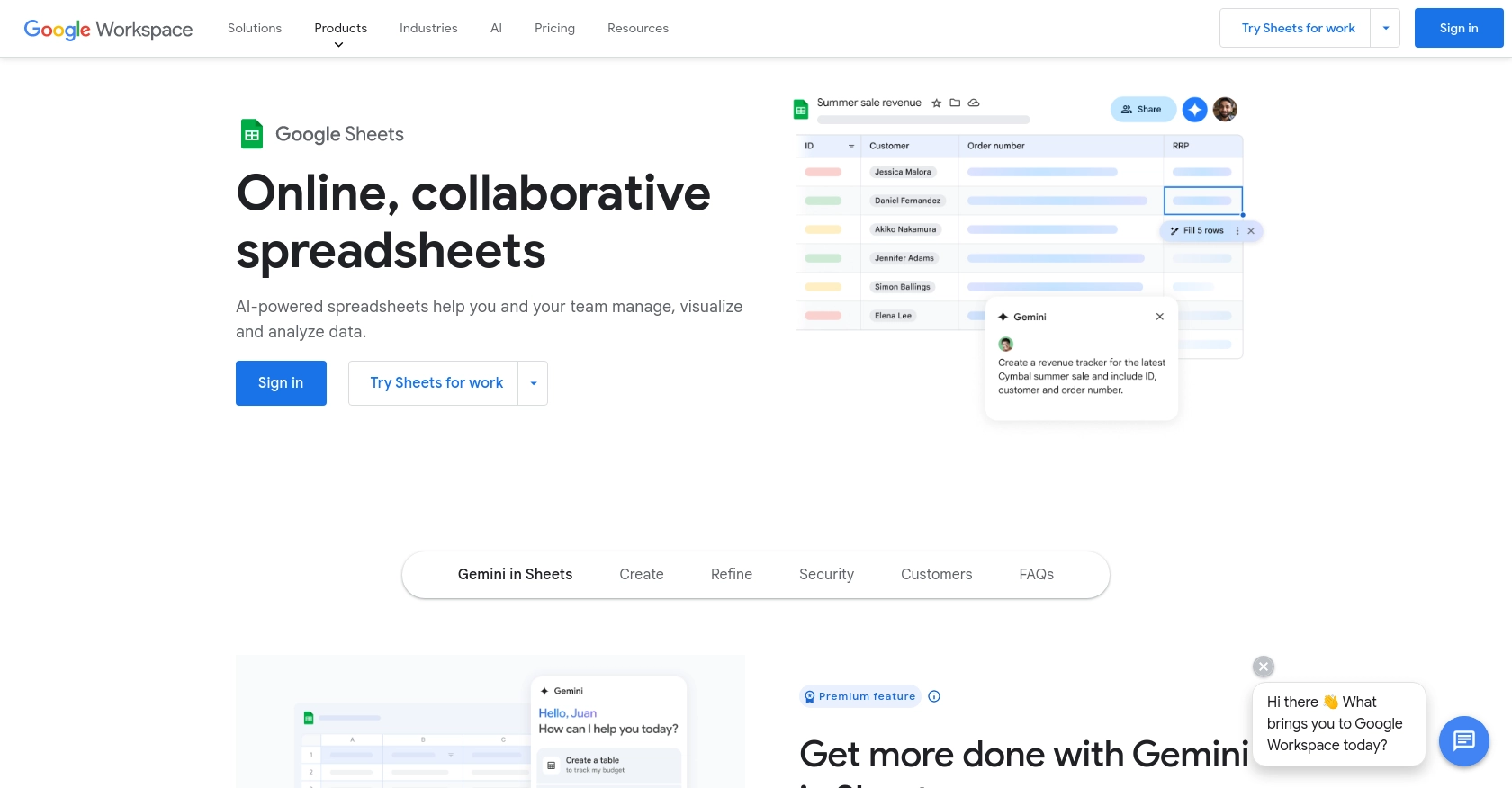
Introduction to Google Sheets API Integration
Google Sheets is a powerful cloud-based spreadsheet application that allows users to create, edit, and collaborate on spreadsheets in real-time. It is part of the Google Workspace suite and offers a wide range of functionalities for data management and analysis.
For developers, integrating with the Google Sheets API can unlock numerous possibilities for automating data workflows. By connecting to Google Sheets, developers can programmatically create, update, and manage spreadsheet data, enabling seamless data synchronization and manipulation across applications.
One common use case for the Google Sheets API is to automate the process of updating records in a spreadsheet. For example, a developer might want to update sales data in a Google Sheet from an external database, ensuring that the spreadsheet always reflects the most current information.
Setting Up Your Google Sheets API Test Account
Before you can start integrating with the Google Sheets API, you'll need to set up a Google Cloud project and configure the necessary credentials. This setup allows you to authenticate and authorize your application to interact with Google Sheets programmatically.
Step 1: Create a Google Cloud Project
To begin, you need a Google Cloud project. This project will serve as the foundation for enabling APIs and managing credentials.
- Go to the Google Cloud Console.
- Click on the Menu icon, navigate to IAM & Admin, and select Create a Project.
- Enter a descriptive name for your project and click Create.
Step 2: Enable the Google Sheets API
Once your project is created, you need to enable the Google Sheets API to allow your application to access it.
- In the Google Cloud Console, navigate to APIs & Services and select Library.
- Search for Google Sheets API and click Enable.
Step 3: Configure OAuth Consent Screen
Next, configure the OAuth consent screen, which is required for user authentication.
- In the Google Cloud Console, go to APIs & Services and select OAuth consent screen.
- Select the user type and fill out the required information, then click Save and Continue.
Step 4: Create OAuth 2.0 Credentials
To authenticate users, you'll need to create OAuth 2.0 credentials.
- Navigate to APIs & Services and select Credentials.
- Click Create Credentials and choose OAuth client ID.
- Select the application type (e.g., Web application) and configure the authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Step 5: Obtain Access Token
With your OAuth credentials, you can now obtain an access token to authenticate API requests.
- Use the client ID and client secret to request an access token from Google's OAuth 2.0 server.
- Refer to the OAuth 2.0 documentation for detailed steps on obtaining an access token.
Once you have completed these steps, your Google Sheets API test account is ready. You can now proceed to make API calls to create or update records in Google Sheets using Python.
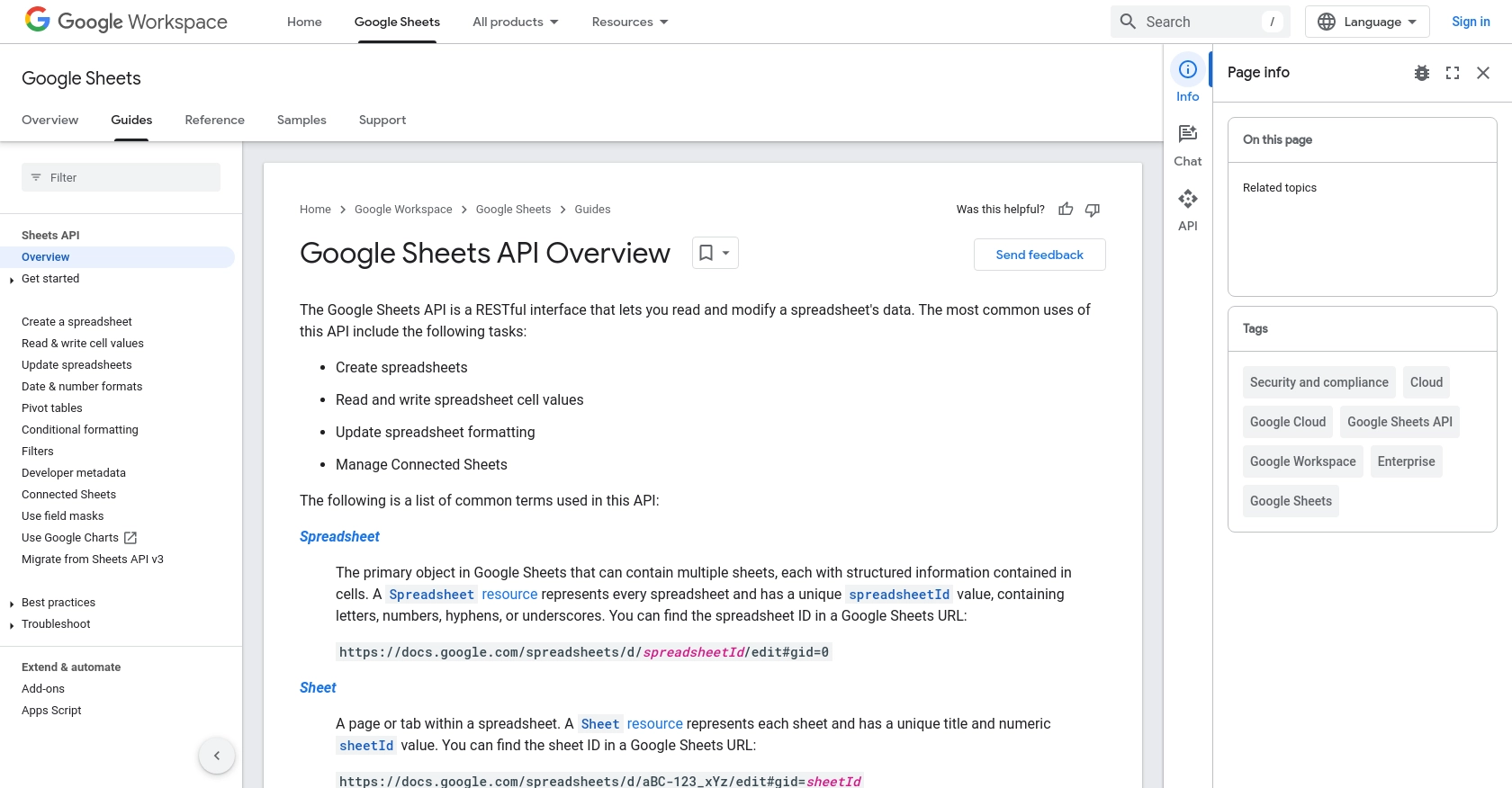
sbb-itb-96038d7
Making API Calls to Create or Update Records in Google Sheets Using Python
In this section, we will explore how to interact with the Google Sheets API using Python to create or update records. This involves setting up your Python environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Google Sheets API
Before making API calls, ensure you have the correct version of Python and the required libraries installed. This guide uses Python 3.11.1 and the Google Client Library for Python.
- Ensure Python 3.11.1 is installed on your machine.
- Install the Google Client Library using pip:
pip install --upgrade google-api-python-client google-auth-httplib2 google-auth-oauthlib
Writing Python Code to Interact with Google Sheets API
Now, let's write the Python code to create or update records in a Google Sheet. We'll use the googleapiclient.discovery
module to build the service and make API requests.
from google.oauth2 import service_account
from googleapiclient.discovery import build
# Define the scope and credentials
SCOPES = ['https://www.googleapis.com/auth/spreadsheets']
SERVICE_ACCOUNT_FILE = 'path/to/your/service-account-file.json'
# Authenticate and build the service
credentials = service_account.Credentials.from_service_account_file(
SERVICE_ACCOUNT_FILE, scopes=SCOPES)
service = build('sheets', 'v4', credentials=credentials)
# Specify the spreadsheet ID and range
spreadsheet_id = 'your_spreadsheet_id'
range_name = 'Sheet1!A1:D5'
# Data to be updated
values = [
['Name', 'Age', 'City', 'Occupation'],
['Alice', '30', 'New York', 'Engineer'],
['Bob', '25', 'Los Angeles', 'Designer']
]
# Prepare the request body
body = {
'values': values
}
# Make the API call to update the sheet
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheet_id, range=range_name,
valueInputOption='RAW', body=body).execute()
print(f"{result.get('updatedCells')} cells updated.")
Replace path/to/your/service-account-file.json
with the path to your service account JSON file and your_spreadsheet_id
with your actual spreadsheet ID.
Verifying API Call Success and Handling Errors
After running the code, you should see the number of cells updated printed in the console. To verify, check your Google Sheet for the updated data.
Handle potential errors by catching exceptions and checking the response status. Here's an example of error handling:
try:
result = service.spreadsheets().values().update(
spreadsheetId=spreadsheet_id, range=range_name,
valueInputOption='RAW', body=body).execute()
print(f"{result.get('updatedCells')} cells updated.")
except Exception as e:
print(f"An error occurred: {e}")
For more detailed error codes and handling strategies, refer to the Google Sheets API documentation.
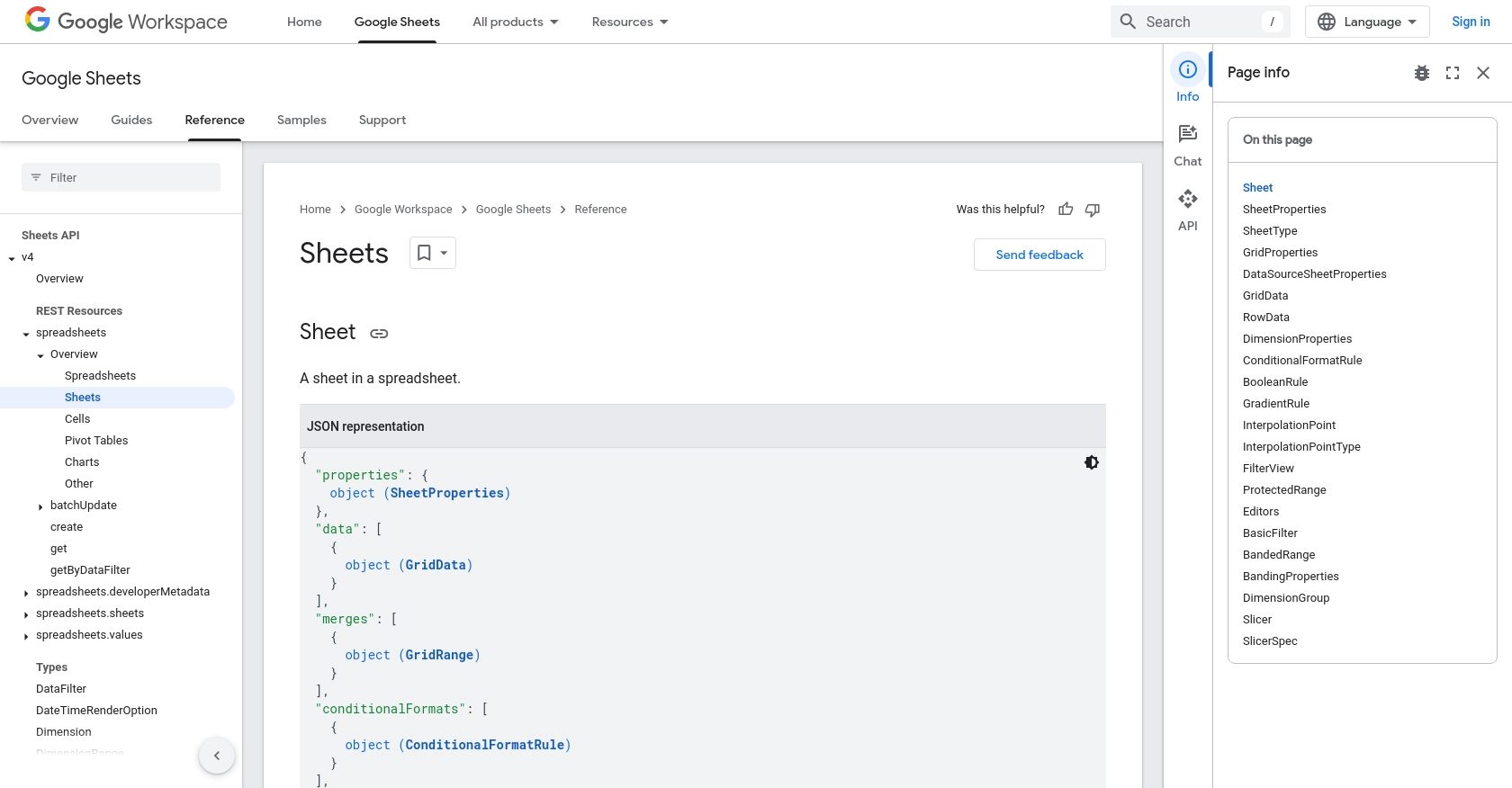
Conclusion and Best Practices for Google Sheets API Integration
Integrating with the Google Sheets API using Python provides a powerful way to automate and manage spreadsheet data efficiently. By following the steps outlined in this guide, you can create or update records in Google Sheets seamlessly, enhancing your data workflows and ensuring real-time synchronization across platforms.
Best Practices for Secure and Efficient Google Sheets API Usage
- Securely Store Credentials: Always store your OAuth credentials and service account files securely. Avoid hardcoding sensitive information in your codebase. Consider using environment variables or secure vaults to manage credentials.
- Handle Rate Limiting: Google Sheets API has usage limits. To avoid hitting these limits, implement exponential backoff strategies and monitor your API usage. For more details, refer to the Google Sheets API documentation.
- Optimize Data Handling: When dealing with large datasets, consider batching requests to minimize API calls and improve performance. Use the
batchUpdate
method for efficient data manipulation. - Data Transformation: Standardize and transform data fields as needed before updating Google Sheets. This ensures consistency and accuracy in your spreadsheets.
Leverage Endgrate for Streamlined Integrations
While integrating with Google Sheets API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Google Sheets.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/googlesheets
- https://developers.google.com/sheets/api/guides/concepts
- https://developers.google.com/sheets/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/sheets
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/cells
Ready to get started?