Using the Google Analytics API to Get Analytics By Page (with Javascript examples)
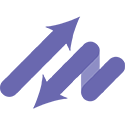
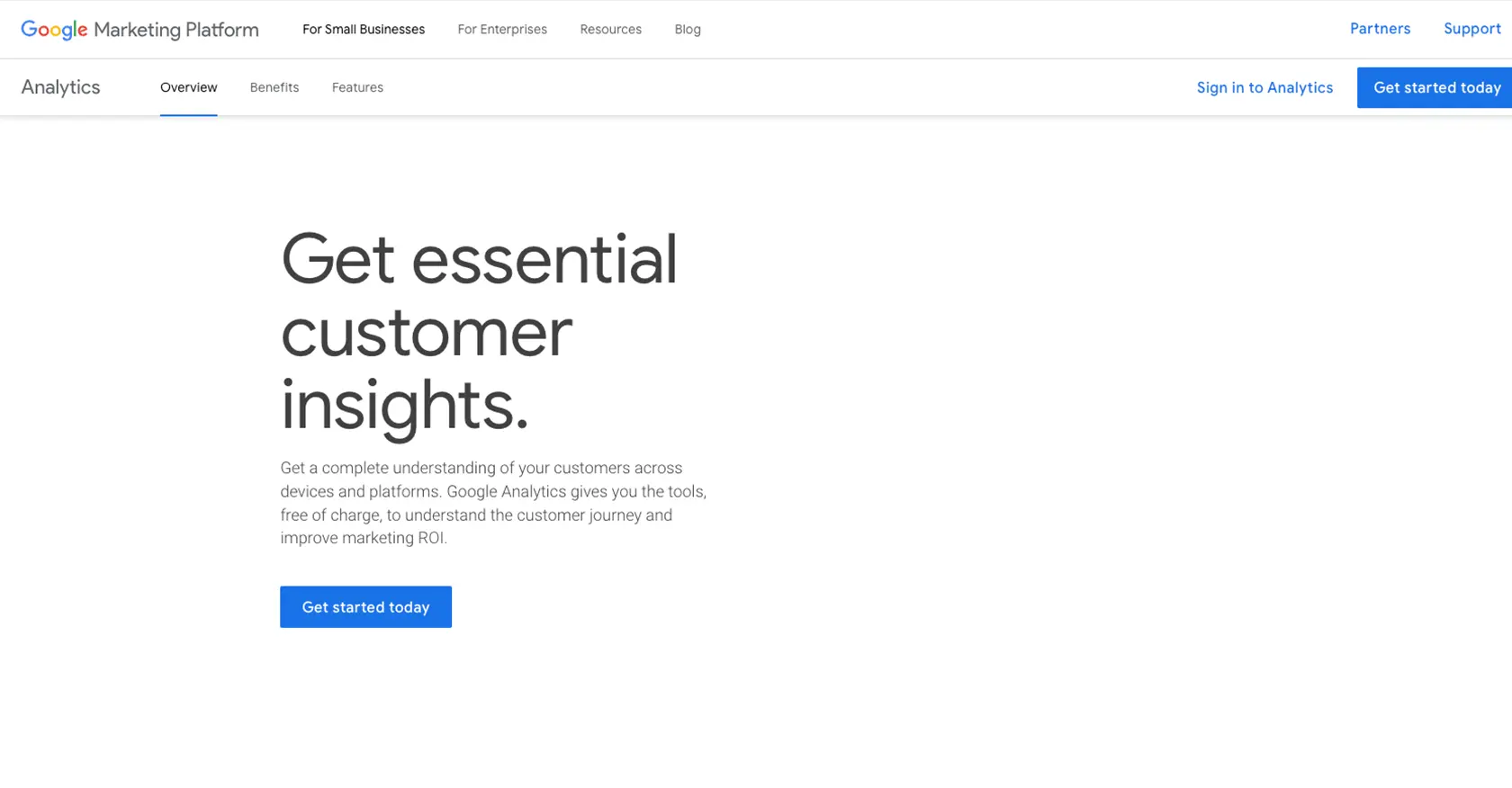
Introduction to Google Analytics API
Google Analytics is a powerful tool that provides insights into website traffic and user behavior. It enables businesses to track and analyze data to make informed decisions about their online presence. The Google Analytics API allows developers to programmatically access this data, offering flexibility in how it is used and integrated with other applications.
Integrating with the Google Analytics API can help developers automate reporting and data analysis tasks. For example, a developer might use the API to retrieve page-specific analytics data, enabling a deeper understanding of user interactions on different pages of a website. This can be particularly useful for optimizing content and improving user engagement.
Setting Up Your Google Analytics Test Account
Before you can start using the Google Analytics API, you need to set up a Google Cloud project and enable the necessary APIs. This will provide you with the credentials required to authenticate your requests.
Create a Google Cloud Project for Google Analytics API
- Go to the Google Cloud Console.
- Click on the Menu icon and select IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Analytics API
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for Google Analytics API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen
- Go to APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields and click Save and Continue.
Create OAuth Credentials for Google Analytics API
- Navigate to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for the credentials and add your authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate your API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
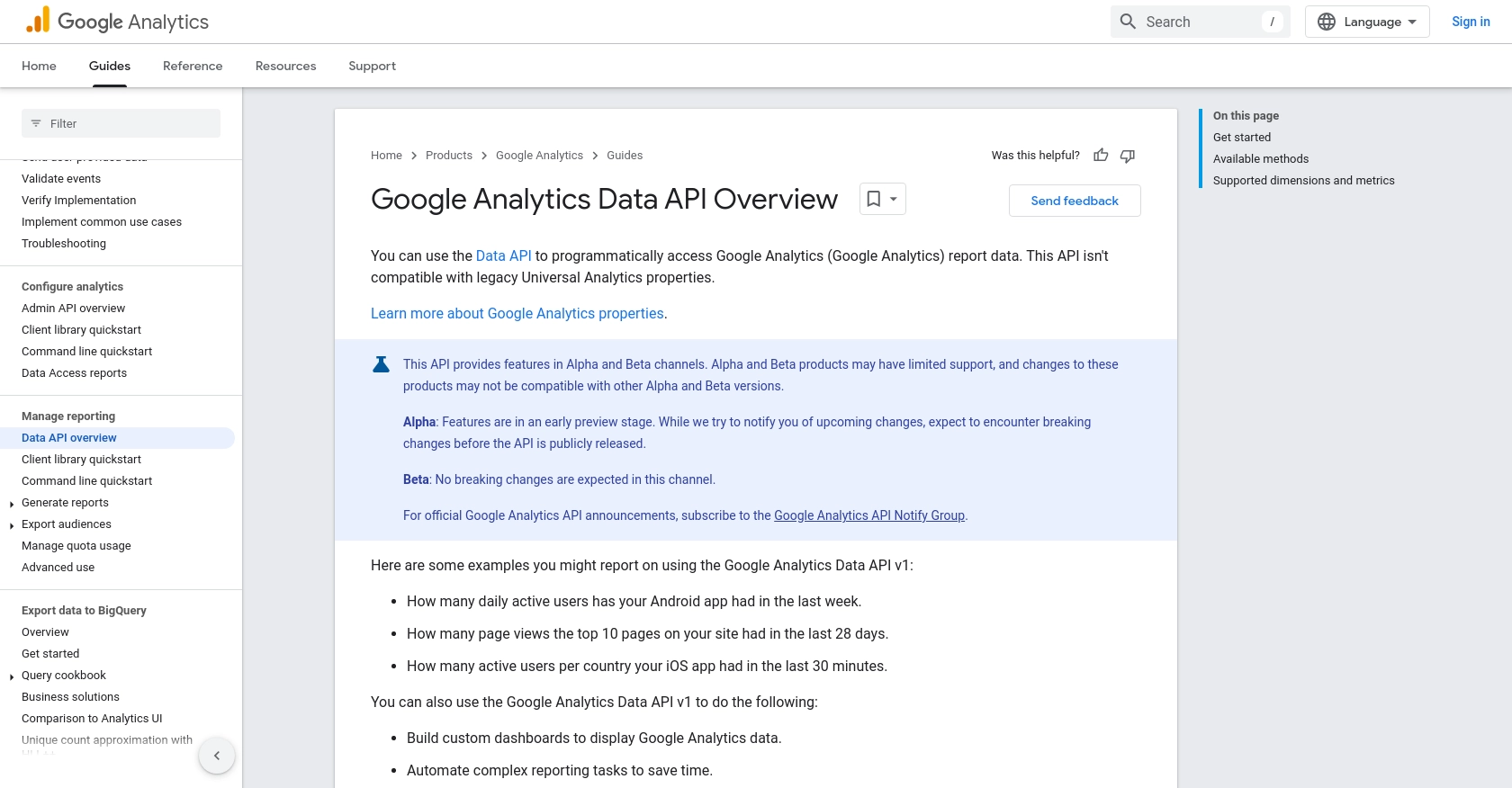
sbb-itb-96038d7
Making API Calls to Google Analytics Using JavaScript
To interact with the Google Analytics API using JavaScript, you'll need to set up your environment and write code to make API requests. This section will guide you through the necessary steps and provide example code to retrieve analytics data by page.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, which is essential for server-side API interactions.
- Download and install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Google Analytics Data API client library by running
npm install @google-analytics/data
.
Authenticating with Google Analytics API
To authenticate your requests, you'll need to use the OAuth 2.0 credentials you created earlier. Ensure your environment variables are set up correctly to access these credentials.
// Load environment variables
require('dotenv').config();
// Set up Google Analytics client
const { BetaAnalyticsDataClient } = require('@google-analytics/data');
const analyticsDataClient = new BetaAnalyticsDataClient();
Example: Retrieving Page Analytics Data
Below is an example of how to make a request to the Google Analytics API to retrieve analytics data by page. This example demonstrates how to use the runReport
method to get page views for specific pages.
// Define your Google Analytics property ID
const propertyId = 'YOUR-GA4-PROPERTY-ID';
// Function to run the report
async function getPageAnalytics() {
const [response] = await analyticsDataClient.runReport({
property: `properties/${propertyId}`,
dimensions: [{ name: 'pagePath' }],
metrics: [{ name: 'pageviews' }],
dateRanges: [{ startDate: '30daysAgo', endDate: 'today' }],
});
// Print the report results
console.log('Page Analytics Report:');
response.rows.forEach(row => {
console.log(`Page: ${row.dimensionValues[0].value}, Views: ${row.metricValues[0].value}`);
});
}
// Execute the function
getPageAnalytics().catch(console.error);
Handling API Responses and Errors
After making an API call, it's crucial to handle the response and any potential errors. The example above logs the page path and view count for each page. If an error occurs, it will be caught and logged to the console.
To verify the request's success, check the console output for the expected data. If the request fails, ensure your credentials are correct and that the Google Analytics API is enabled for your project.
For more details on error handling and response structure, refer to the Google Analytics API documentation.
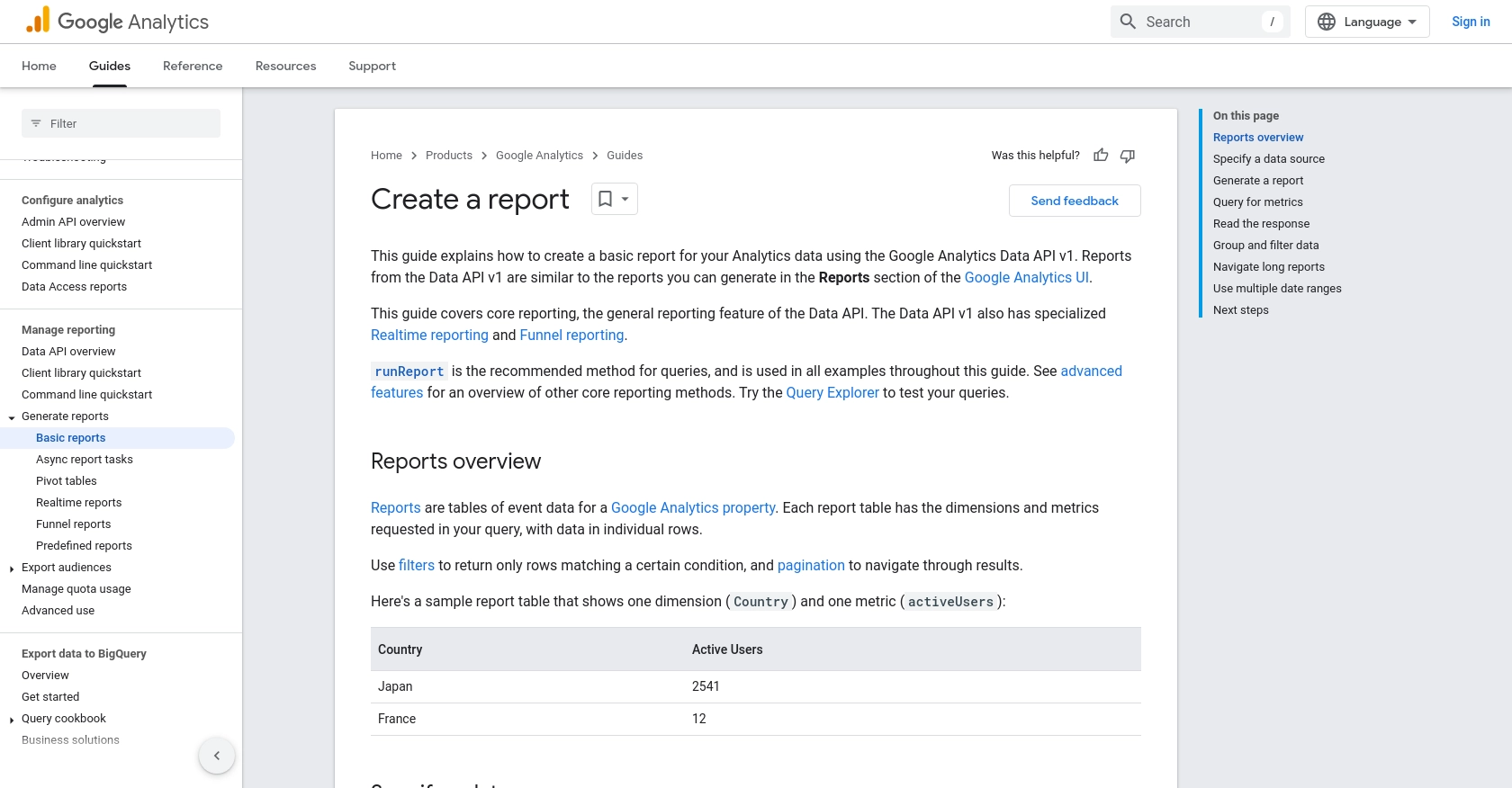
Conclusion and Best Practices for Using Google Analytics API with JavaScript
Integrating with the Google Analytics API using JavaScript provides a powerful way to automate data retrieval and analysis, enabling developers to gain deeper insights into user behavior on specific pages. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate requests, and handle API responses to retrieve valuable analytics data.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth client ID and client secret securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage request limits effectively.
- Optimize Data Requests: Use filters and dimensions wisely to limit the amount of data retrieved, ensuring faster response times and reduced load on the API.
- Monitor API Usage: Regularly monitor your API usage and adjust your requests as needed to stay within quota limits and optimize performance.
Enhance Your Integration with Endgrate
For developers looking to streamline their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case instead of multiple times for different integrations, and provide an intuitive integration experience for your customers. Learn more about how Endgrate can simplify your integration needs by visiting Endgrate.
By leveraging the Google Analytics API and following best practices, you can unlock the full potential of your analytics data, driving informed decisions and enhancing user engagement on your website.
Read More
- https://endgrate.com/provider/googleanalytics
- https://developers.google.com/analytics/devguides/reporting/data/v1
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/analytics/devguides/reporting/data/v1/basics
Ready to get started?