Using the Sap Business One API to Get Orders in PHP
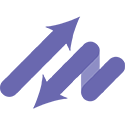
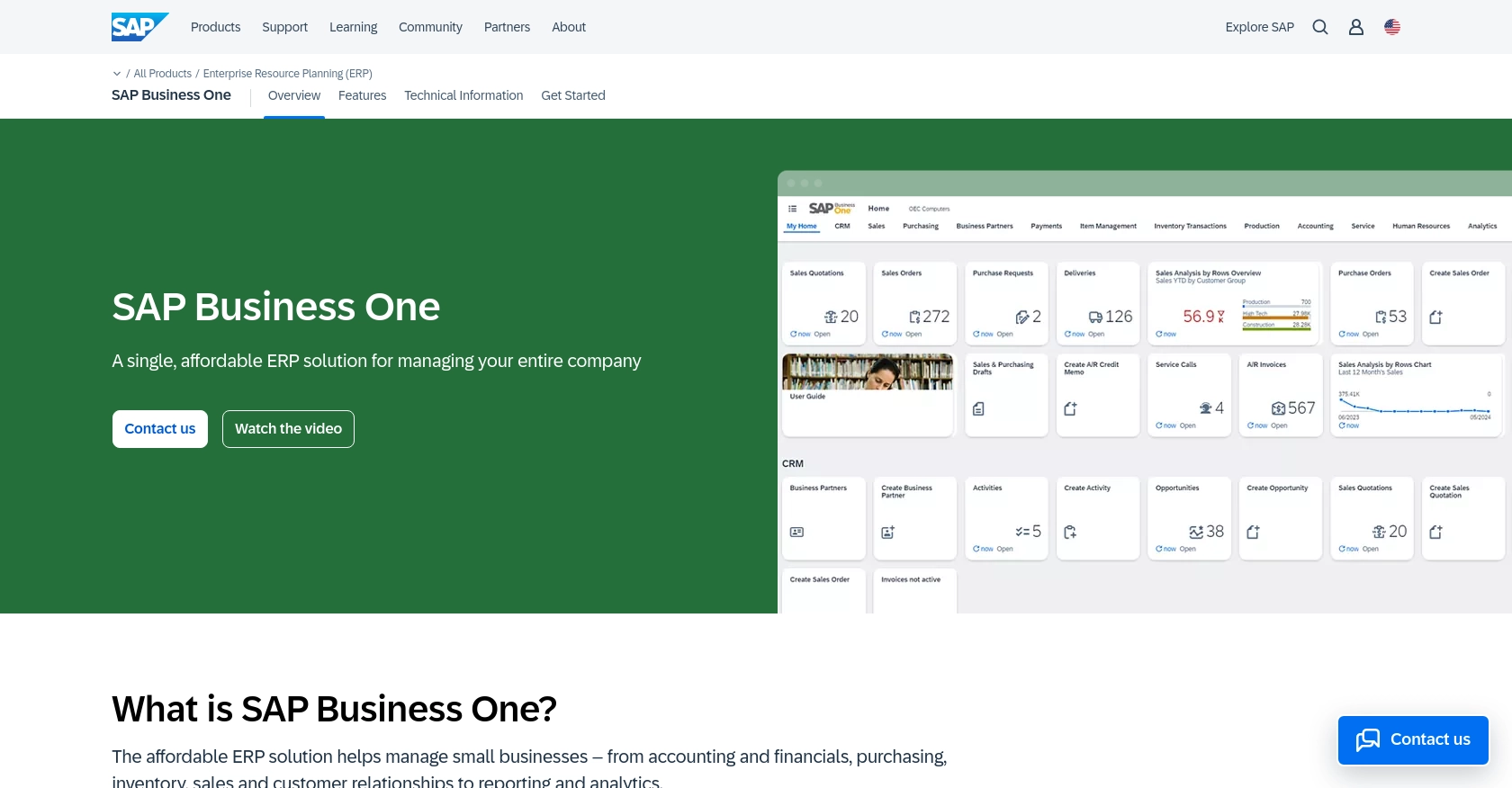
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed specifically for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single, integrated platform.
Developers may want to connect with the SAP Business One API to streamline business processes and enhance data management capabilities. For example, retrieving order information using the SAP Business One API in PHP can help automate order processing, improve accuracy, and provide real-time insights into sales activities.
Setting Up a Test/Sandbox Account for SAP Business One API
Before you can start interacting with the SAP Business One API using PHP, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting your live data. SAP Business One provides a service layer that facilitates this process.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One environment. If you don't have one, you can request a demo or trial version from SAP's official website. This will provide you with a sandbox environment where you can test API interactions.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to set up authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer Management section.
- Create a new application and note down the client ID and client secret provided.
- Set the necessary scopes and permissions required for accessing order data.
Ensure you store the client ID and client secret securely, as they will be used in your PHP code to authenticate API requests.
Generating API Keys for SAP Business One
If your SAP Business One setup requires API key-based access, follow these steps:
- Go to the API Management section in your SAP Business One account.
- Generate a new API key and assign it the necessary permissions for order retrieval.
- Store the API key securely for use in your PHP application.
For more detailed information on authentication, refer to the SAP Business One Service Layer documentation.
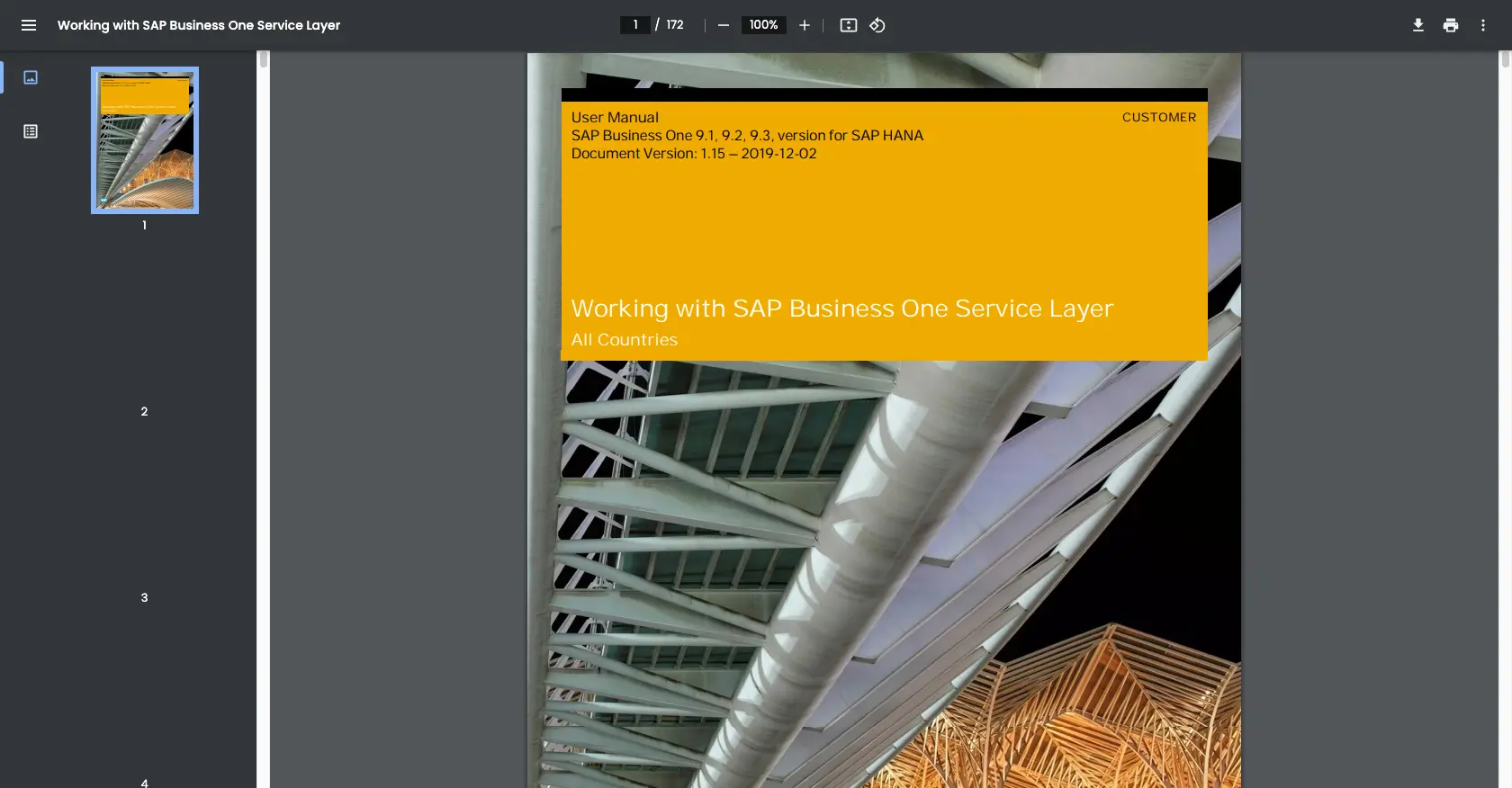
sbb-itb-96038d7
Making API Calls to Retrieve Orders from SAP Business One Using PHP
To interact with the SAP Business One API and retrieve order data using PHP, you'll need to set up your development environment and write code to make the necessary API calls. This section will guide you through the process, including setting up PHP, installing dependencies, and writing the code to fetch orders.
Setting Up Your PHP Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, open your terminal and install the Guzzle HTTP client, which will help you make HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Orders from SAP Business One API
With your environment set up, you can now write the PHP code to retrieve orders. Create a file named get_orders.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle HTTP client
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Orders';
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer Your_Access_Token'
];
// Make a GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Check if the request was successful
if ($response->getStatusCode() === 200) {
// Parse the JSON data from the response
$orders = json_decode($response->getBody(), true);
// Loop through the orders and print their details
foreach ($orders['value'] as $order) {
echo 'Order ID: ' . $order['DocEntry'] . "\n";
echo 'Customer: ' . $order['CardName'] . "\n";
echo 'Total: ' . $order['DocTotal'] . "\n\n";
}
} else {
echo 'Failed to retrieve orders. Status code: ' . $response->getStatusCode();
}
?>
Replace Your_Access_Token
with the access token obtained during the authentication setup. This code initializes the Guzzle client, sets the API endpoint and headers, and makes a GET request to retrieve orders. If successful, it parses and displays the order details.
Verifying API Call Success and Handling Errors
After running the script, you should see the order details printed in your terminal. To verify the success of the API call, you can cross-check the returned data with the orders in your SAP Business One sandbox account.
If the request fails, the script will output the status code. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it has the necessary permissions.
- 404 Not Found: Verify the API endpoint URL.
- 500 Internal Server Error: This may indicate an issue with the SAP Business One server.
For more detailed error handling, refer to the SAP Business One Service Layer API Reference.
Conclusion and Best Practices for Using SAP Business One API in PHP
Integrating with the SAP Business One API using PHP can significantly enhance your business processes by automating order retrieval and providing real-time insights. By following the steps outlined in this article, you can efficiently set up your environment, authenticate your requests, and handle API interactions.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and potential API errors effectively.
Streamline Your Integration Process with Endgrate
While integrating with SAP Business One API can be rewarding, it can also be time-consuming and complex, especially if you need to manage multiple integrations. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including SAP Business One. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can help streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?