Using the Woodpecker API to Create or Update Prospects in Python
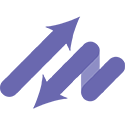
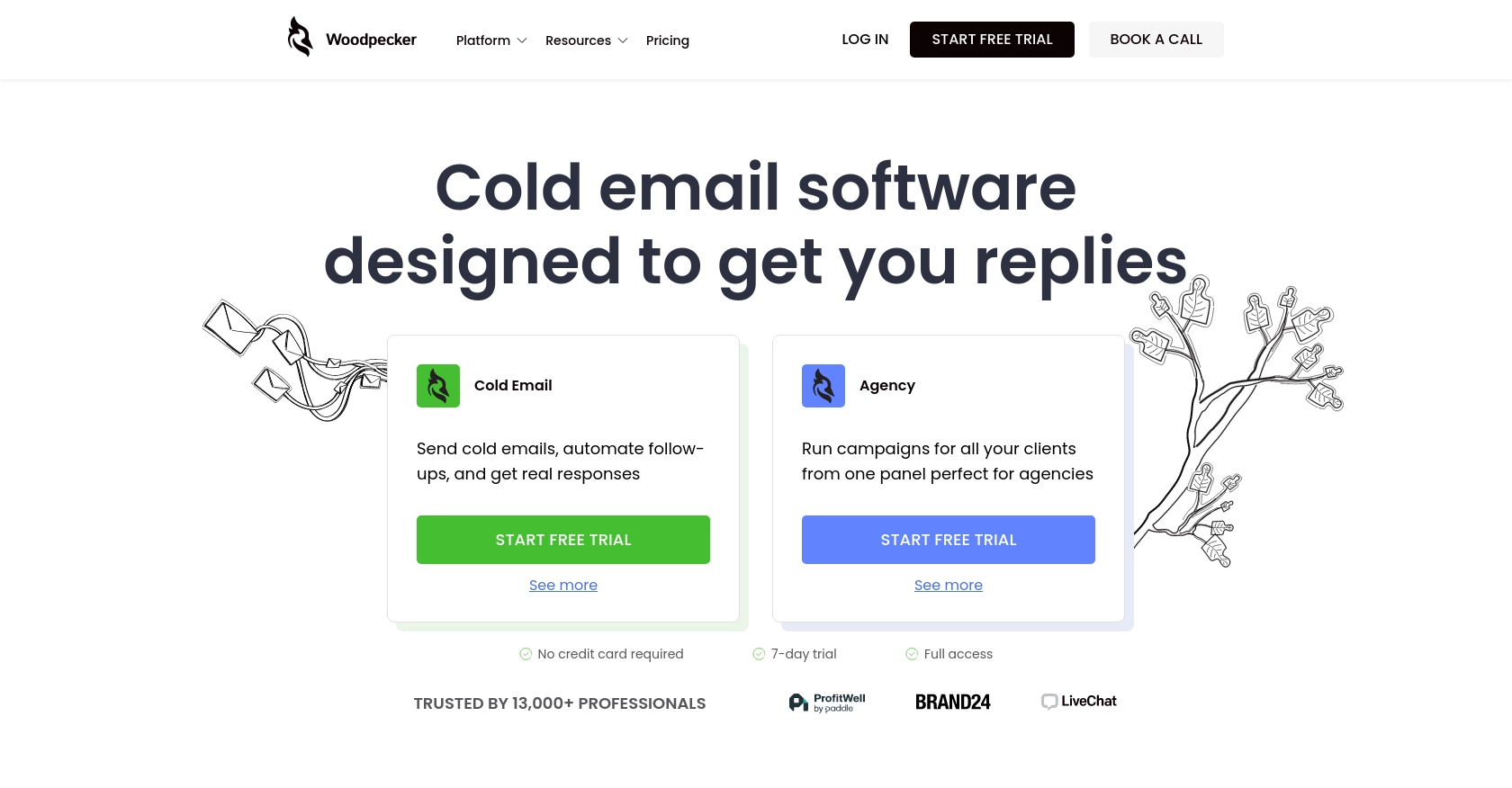
Introduction to Woodpecker API
Woodpecker is a powerful tool designed to enhance cold email outreach and automate follow-ups for businesses. It offers a comprehensive platform for managing prospects, campaigns, and email sequences, making it an essential tool for sales teams, lead generation agencies, and recruiters.
Integrating with the Woodpecker API allows developers to efficiently manage prospect data, automate email campaigns, and streamline communication processes. For example, you can use the API to create or update prospect information directly from your application, ensuring that your sales team always has the most up-to-date data.
Setting Up Your Woodpecker Test Account and Generating an API Key
Before you can start using the Woodpecker API to manage prospects, you'll need to set up a Woodpecker account and generate an API key. This key will allow you to authenticate your requests and interact with the API securely.
Step-by-Step Guide to Creating a Woodpecker Account
- Visit the Woodpecker website and sign up for a free trial or log in if you already have an account.
- Once logged in, navigate to the Marketplace section.
- Select Integrations and then click on API keys.
Generating Your Woodpecker API Key
- In the API keys section, click the green button labeled Create a Key.
- Your new API key will be generated. Make sure to copy and store it securely, as it will be used to authenticate your API requests.
For more detailed instructions, you can refer to the Woodpecker API Key Generation Guide.
Understanding API Key Authentication
Woodpecker uses API key-based authentication. When making API requests, include your API key in the request headers as follows:
headers = {
"Authorization": "Basic <Your_API_Key>"
}
Ensure your API key is encoded in Base64 format when using tools like cURL. For more information, visit the Woodpecker Authentication Documentation.
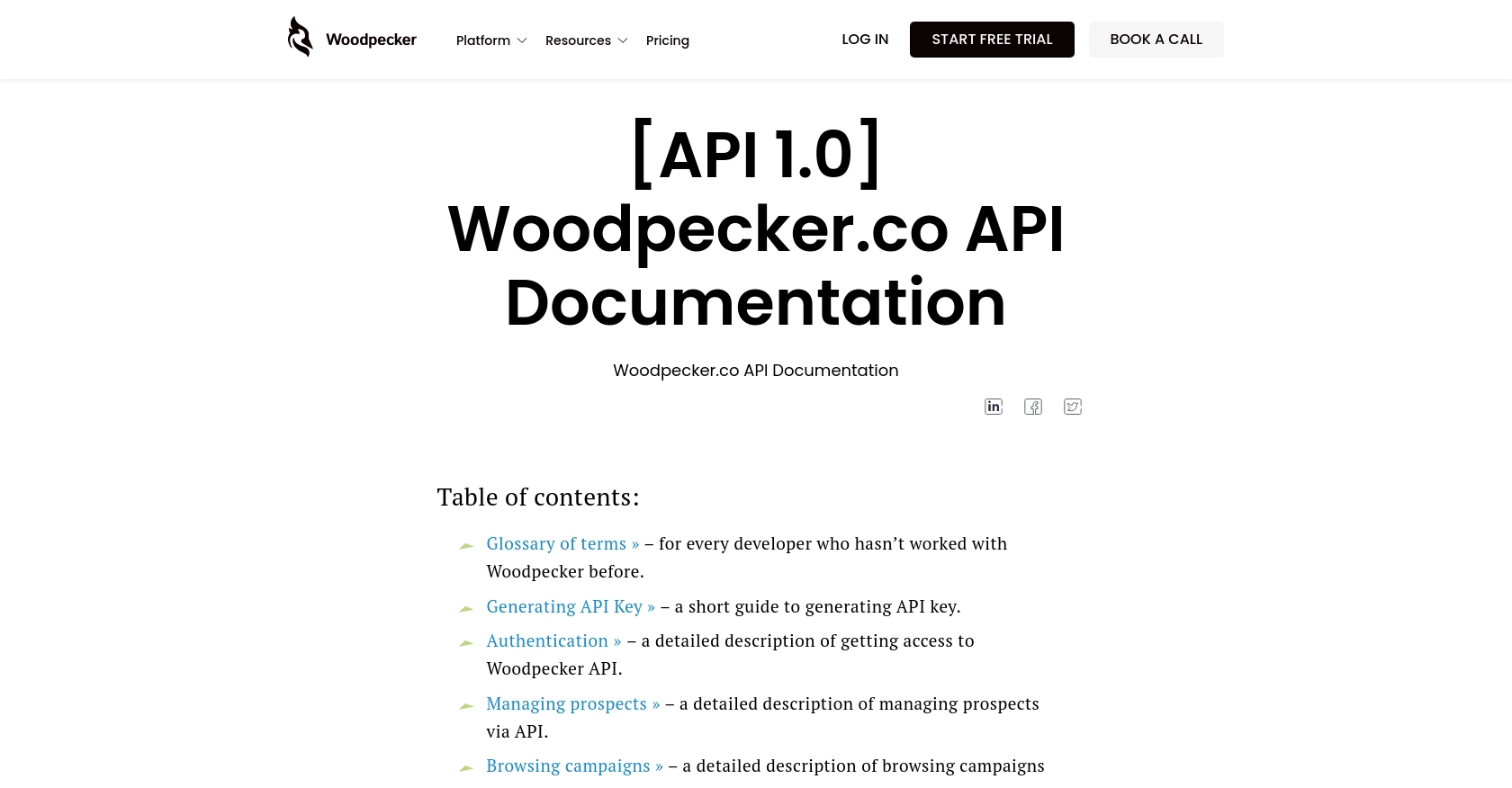
sbb-itb-96038d7
Making API Calls to Create or Update Prospects Using the Woodpecker API in Python
To interact with the Woodpecker API for creating or updating prospects, you'll need to use Python and the requests library. This section will guide you through setting up your environment, writing the code, and handling responses effectively.
Setting Up Your Python Environment for Woodpecker API Integration
Before making API calls, ensure you have Python 3.x installed on your machine. Additionally, you'll need the requests library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Create or Update Prospects in Woodpecker
Create a new Python file named manage_prospects.py
and add the following code:
import requests
# Set the API endpoint
url = "https://api.woodpecker.co/rest/v1/add_prospects_list"
# Set the request headers
headers = {
"Authorization": "Basic <Your_API_Key>",
"Content-Type": "application/json"
}
# Define the prospect data
prospect_data = {
"update": "true",
"prospects": [
{
"email": "example@company.com",
"first_name": "John",
"last_name": "Doe",
"company": "Example Corp",
"industry": "Technology",
"website": "http://www.example.com",
"linkedin_url": "https://www.linkedin.com/in/johndoe/",
"title": "Software Engineer"
}
]
}
# Make the POST request to create or update the prospect
response = requests.post(url, json=prospect_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Prospect created or updated successfully.")
else:
print(f"Failed to create or update prospect. Status code: {response.status_code}")
print(response.json())
Replace <Your_API_Key>
with your actual API key. This code sends a POST request to the Woodpecker API to create or update a prospect. The response is checked to ensure the request was successful.
Verifying the API Request Success in Woodpecker
After running the script, log in to your Woodpecker account and navigate to the prospects section. You should see the newly created or updated prospect listed there. This confirms that the API call was successful.
Handling Errors and Understanding Woodpecker API Response Codes
It's crucial to handle errors effectively. The Woodpecker API provides various error codes that you should be aware of. For instance, a 400 status code indicates a bad request, while a 401 indicates unauthorized access. Always check the response and handle errors gracefully in your application.
For more details on error codes, refer to the Woodpecker API Documentation.
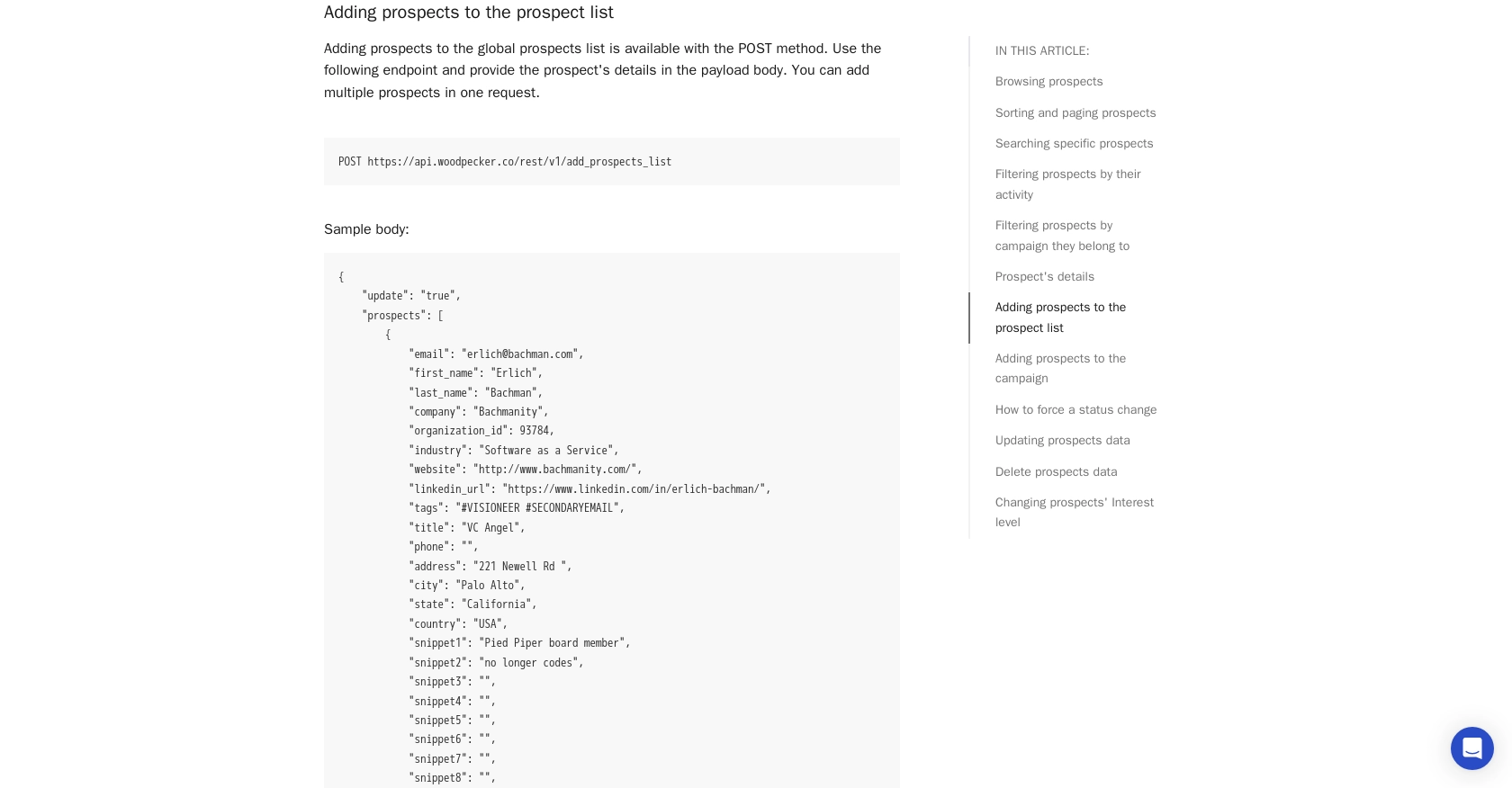
Conclusion and Best Practices for Using the Woodpecker API in Python
Integrating with the Woodpecker API allows developers to efficiently manage and update prospect data, enhancing the effectiveness of sales and marketing campaigns. By following the steps outlined in this guide, you can seamlessly create or update prospects using Python, ensuring your data is always current and actionable.
Best Practices for Secure and Efficient Woodpecker API Integration
- Secure Storage of API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Although Woodpecker allows unlimited API calls per month, remember that only one request can be processed at a time, with a maximum of six queued requests. Plan your API calls accordingly to avoid dropped requests.
- Data Standardization: Ensure that the data you send to the API is standardized and validated to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling in your application to manage different response codes effectively. This will help in diagnosing issues and maintaining a smooth user experience.
Enhance Your Integration Experience with Endgrate
While integrating with individual APIs like Woodpecker can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple services, including Woodpecker. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover the benefits of a unified API approach.
Read More
Ready to get started?