Using the FreshDesk API to Get Companies in PHP
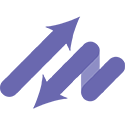
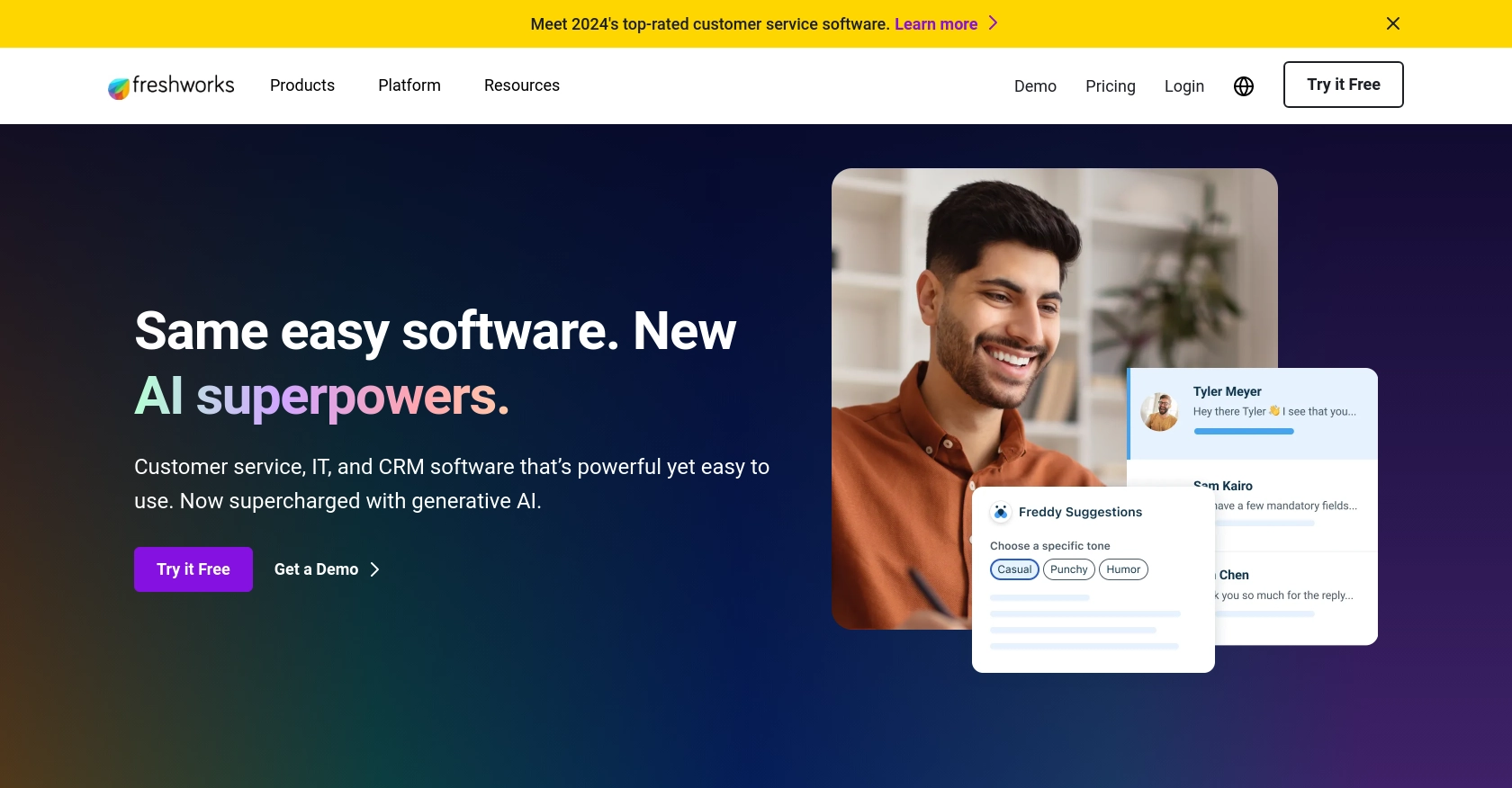
Introduction to FreshDesk API Integration
FreshDesk is a popular customer support software that offers a comprehensive suite of tools to help businesses manage customer interactions efficiently. With features such as ticketing, automation, and reporting, FreshDesk enables companies to deliver exceptional customer service.
Developers might want to integrate with FreshDesk's API to streamline customer support processes, such as retrieving company information to personalize support interactions. For example, a developer could use the FreshDesk API to fetch a list of companies and use this data to tailor support responses based on the company's profile and history.
Setting Up Your FreshDesk Test Account for API Integration
Before you can start using the FreshDesk API to retrieve company information, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a FreshDesk Account
If you don't already have a FreshDesk account, you can sign up for a free trial on the FreshDesk website. This trial provides access to most features, allowing you to test the API thoroughly.
- Visit the FreshDesk signup page.
- Fill out the registration form with your details.
- Verify your email address to activate your account.
Generating API Credentials for FreshDesk
FreshDesk uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your FreshDesk account.
- Navigate to your profile settings by clicking on your profile picture in the top right corner.
- Select Profile Settings from the dropdown menu.
- Under the API Key section, you will find your unique API key. Copy this key and store it securely, as you will need it to authenticate your API requests.
Configuring Your FreshDesk Sandbox Environment
To ensure that your API interactions do not interfere with live data, it's advisable to use a sandbox environment. FreshDesk provides a sandbox feature that allows you to test API calls safely:
- Contact FreshDesk support to request access to a sandbox environment if it's not available in your account settings.
- Once access is granted, you can configure the sandbox to mimic your production environment.
- Use the sandbox to test API calls and verify their behavior before deploying them to production.
With your FreshDesk account set up and API key in hand, you're ready to start making API calls to retrieve company information using PHP.
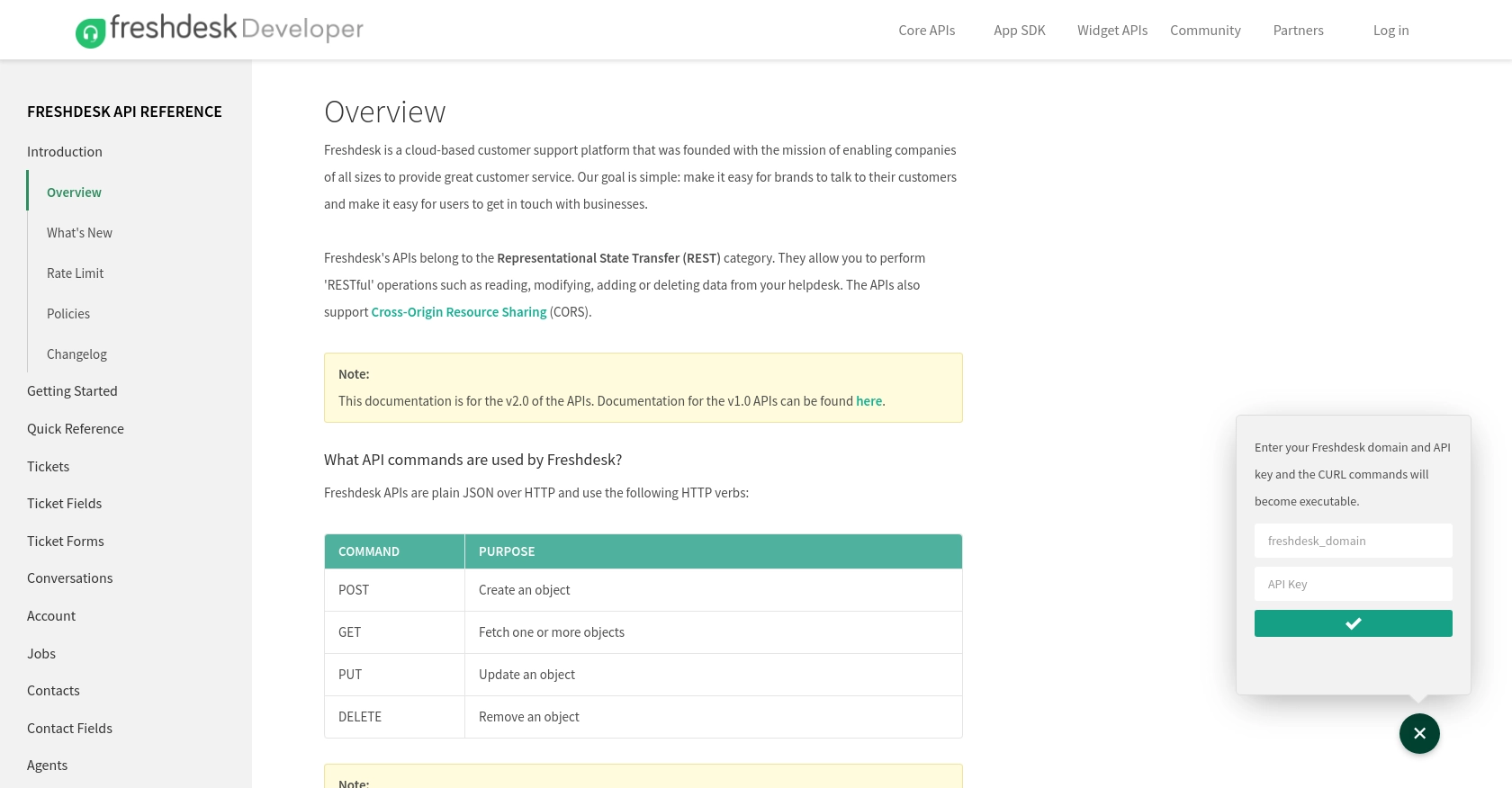
sbb-itb-96038d7
Making API Calls to Retrieve Companies from FreshDesk Using PHP
To interact with the FreshDesk API and retrieve company information, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for FreshDesk API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- The
cURL
extension enabled in your PHP configuration, as it is commonly used for making HTTP requests.
To verify that cURL is enabled, you can check your php.ini
file or run phpinfo()
in a PHP script.
Installing Required PHP Dependencies
While PHP's built-in cURL functions are sufficient for making API calls, you might find it easier to use a library like Guzzle for more complex requests. To install Guzzle, you can use Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Companies from FreshDesk
Now, let's write the PHP code to make a GET request to the FreshDesk API and retrieve company data. Create a new PHP file named get_freshdesk_companies.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$apiKey = 'Your_API_Key';
$domain = 'yourdomain.freshdesk.com';
$client = new Client([
'base_uri' => "https://$domain/api/v2/",
'auth' => [$apiKey, 'X']
]);
try {
$response = $client->request('GET', 'companies');
$companies = json_decode($response->getBody(), true);
foreach ($companies as $company) {
echo "Company ID: " . $company['id'] . "\n";
echo "Company Name: " . $company['name'] . "\n\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Replace Your_API_Key
and yourdomain.freshdesk.com
with your actual FreshDesk API key and domain.
Understanding the PHP Code for FreshDesk API Calls
In the code above, we use Guzzle to simplify the process of making HTTP requests. Here's a breakdown of the key steps:
- We initialize a Guzzle client with the base URI for the FreshDesk API and set up basic authentication using the API key.
- We make a GET request to the
companies
endpoint to fetch company data. - The response is decoded from JSON format, and we loop through the companies to display their IDs and names.
- Error handling is implemented using a try-catch block to catch any exceptions and display error messages.
Verifying the API Call and Handling Errors
After running the script, you should see a list of companies printed in the terminal. If the request is successful, the data should match the companies in your FreshDesk sandbox environment.
In case of errors, the catch block will output the error message. Common issues might include incorrect API keys, domain names, or network connectivity problems.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API using PHP allows developers to efficiently manage and retrieve company data, enhancing customer support processes. By following the steps outlined in this guide, you can set up your environment, make API calls, and handle responses effectively.
Best Practices for FreshDesk API Integration
- Securely Store API Credentials: Always store your FreshDesk API key securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: FreshDesk may impose rate limits on API requests. Implement logic to handle HTTP 429 status codes and retry requests after a delay. Refer to the FreshDesk documentation for specific rate limit details.
- Standardize Data Fields: Ensure that data retrieved from FreshDesk is transformed and standardized to match your application's data structures, improving data consistency.
- Implement Error Handling: Use try-catch blocks to manage exceptions and provide meaningful error messages to aid in debugging and user support.
Streamlining Integrations with Endgrate
While integrating with FreshDesk's API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with various platforms, including FreshDesk. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?