How to Get Prospects with the Woodpecker API in Python
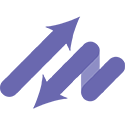
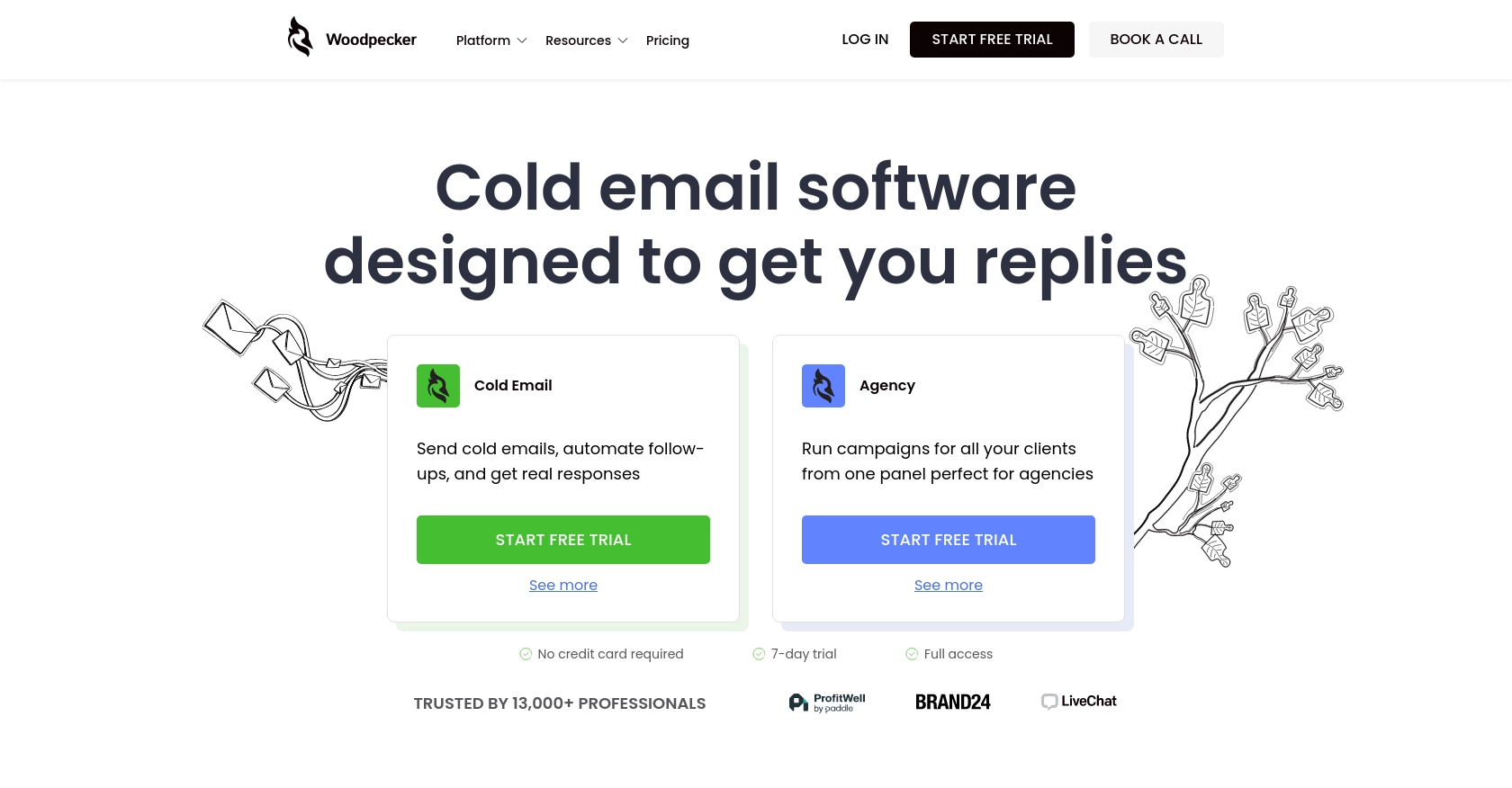
Introduction to Woodpecker API
Woodpecker is a powerful tool designed to enhance cold email outreach and lead generation for businesses. It offers a suite of features that streamline the process of managing prospects, automating follow-ups, and integrating with other platforms.
Developers may want to integrate with the Woodpecker API to efficiently manage and retrieve prospect data. For example, using the Woodpecker API, a developer can automate the process of fetching prospect information to analyze engagement metrics or integrate with CRM systems for a seamless sales workflow.
Setting Up Your Woodpecker Account for API Access
Before you can start using the Woodpecker API to manage prospects, you'll need to set up your account and generate an API key. This key will allow you to authenticate your requests and access the necessary data.
Creating a Woodpecker Account
If you don't already have a Woodpecker account, you can sign up for a free trial on the Woodpecker website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Woodpecker website.
- Click on "Start free trial" and follow the registration process.
- Once your account is created, log in to access the dashboard.
Generating an API Key in Woodpecker
To interact with the Woodpecker API, you'll need to generate an API key. Follow these steps to create your key:
- Log into your Woodpecker account at app.woodpecker.co.
- Navigate to the "Marketplace" section and select "INTEGRATIONS".
- Click on "API keys" and then use the green button to "CREATE A KEY".
- Copy the generated API key and store it securely, as you'll need it for authentication.
For more detailed instructions, refer to the Woodpecker API Key Generation Guide.
Understanding API Key Authentication
Woodpecker uses API key-based authentication to secure access to its API. When making requests, include your API key in the headers as follows:
headers = {
"Authorization": "Basic <API_KEY>"
}
Ensure your API key is encoded in Base64 format before using it in requests. For more information, visit the Woodpecker Authentication Documentation.
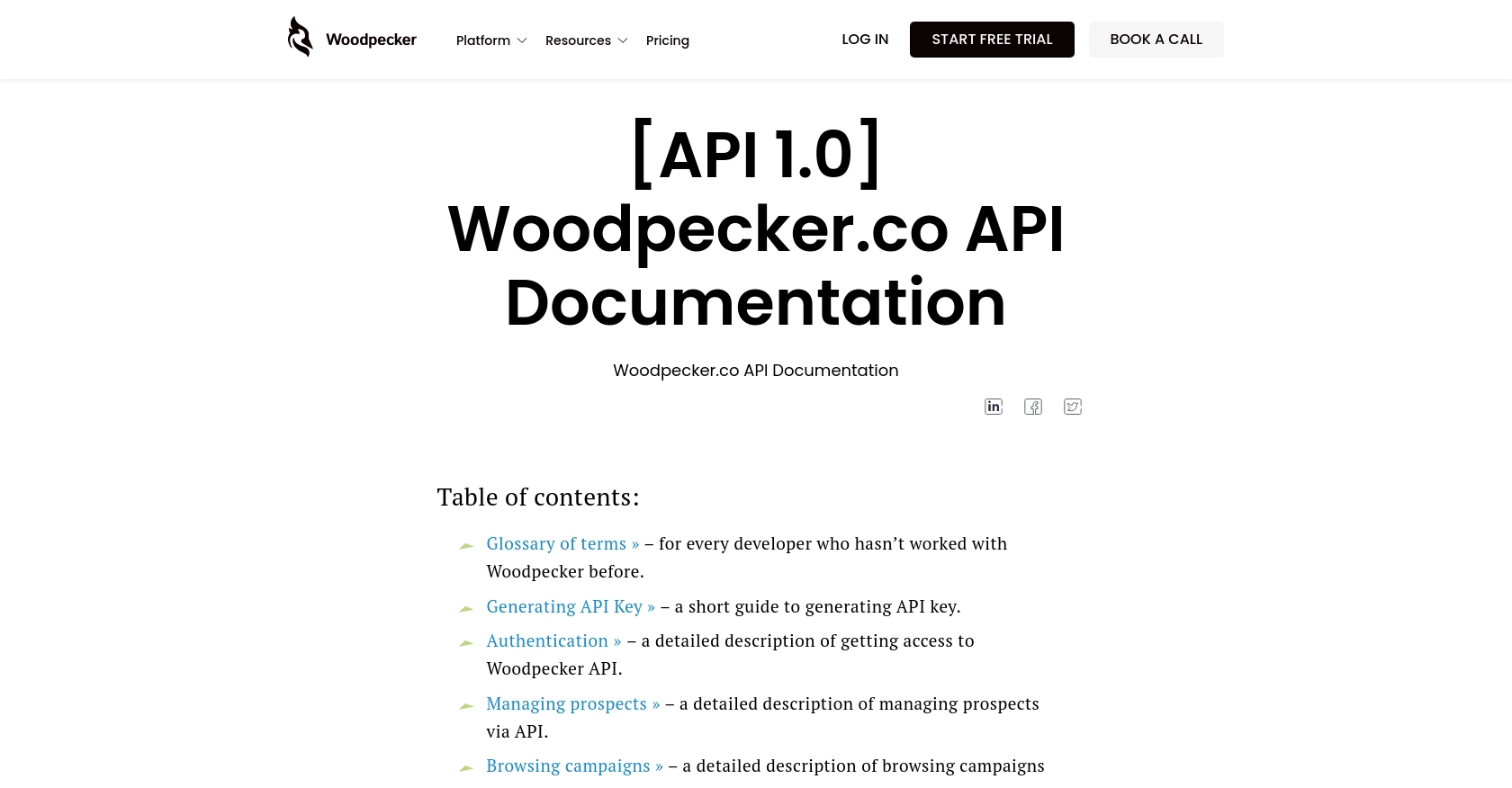
sbb-itb-96038d7
Making API Calls to Retrieve Prospects with Woodpecker API in Python
To interact with the Woodpecker API and retrieve prospect data, you'll need to use Python. This section will guide you through setting up your environment and making the necessary API calls.
Setting Up Python Environment for Woodpecker API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website.
- Use pip to install the
requests
library by running the following command:
pip install requests
Writing Python Code to Fetch Prospects from Woodpecker
Create a Python script named get_woodpecker_prospects.py
and add the following code to retrieve prospects:
import requests
# Define the API endpoint and headers
endpoint = "https://api.woodpecker.co/rest/v1/prospects"
headers = {
"Authorization": "Basic <API_KEY>"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
prospects = response.json()
# Loop through the prospects and print their information
for prospect in prospects:
print(prospect)
else:
print(f"Failed to retrieve prospects: {response.status_code} - {response.text}")
Replace <API_KEY>
with your actual API key. This script sends a GET request to the Woodpecker API to fetch prospect data and prints it to the console.
Verifying Successful API Requests in Woodpecker
After running the script, you should see a list of prospects printed in the console. To verify the request's success, you can cross-check the data with the prospects listed in your Woodpecker account.
Handling Errors and Understanding Woodpecker API Error Codes
When making API calls, it's crucial to handle potential errors. The Woodpecker API provides error codes to help diagnose issues. If the request fails, the script prints the status code and error message. Refer to the Woodpecker API documentation for a detailed list of error codes and their meanings.
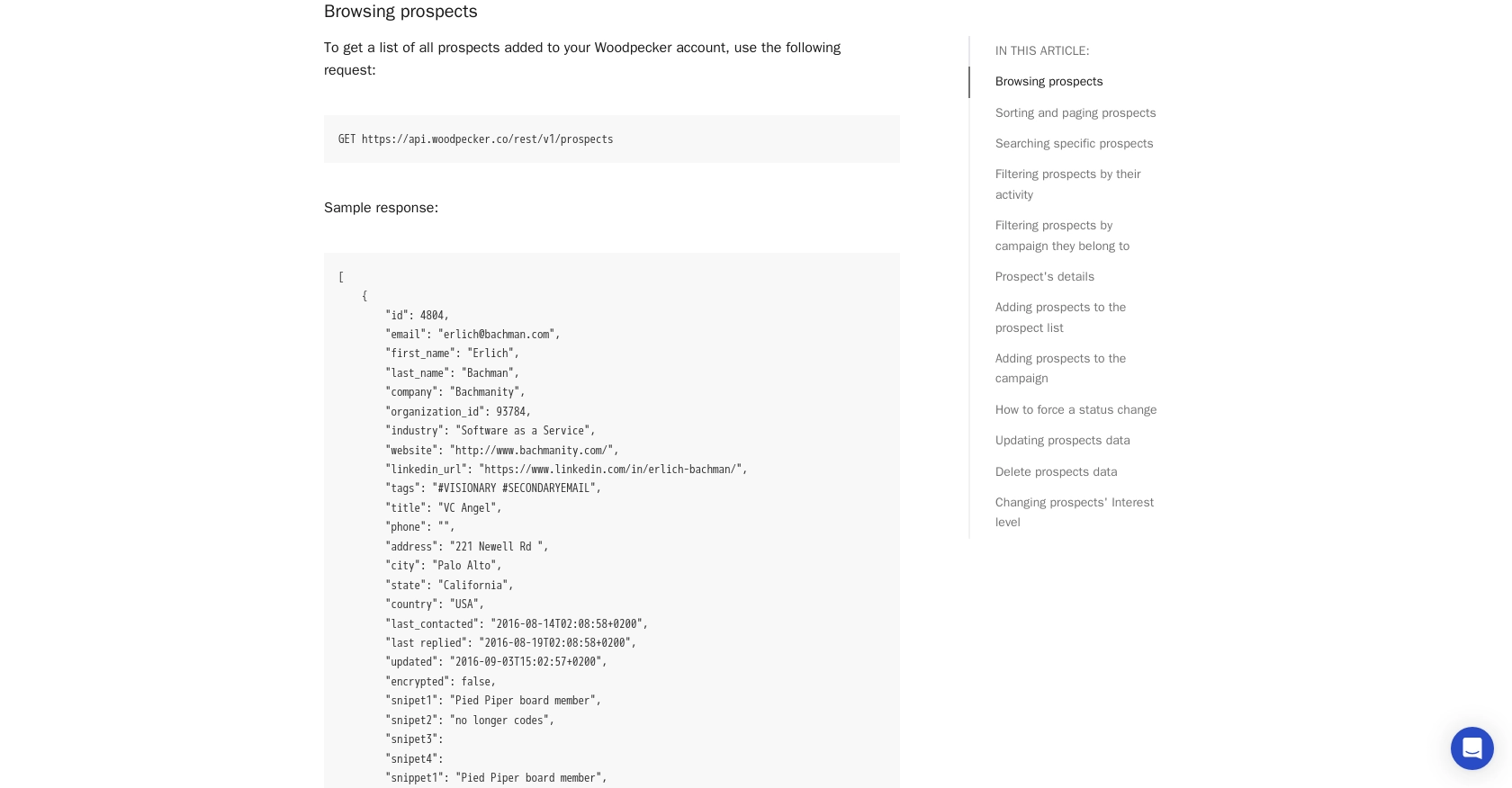
Conclusion and Best Practices for Using Woodpecker API in Python
Integrating with the Woodpecker API allows developers to efficiently manage and retrieve prospect data, enhancing the capabilities of cold email outreach and lead generation efforts. By following the steps outlined in this guide, you can seamlessly connect your applications to Woodpecker and automate various processes.
Best Practices for Secure and Efficient API Integration with Woodpecker
- Secure Storage of API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Although Woodpecker allows unlimited API calls per month, remember that only one request can be processed at a time, with a maximum queue of six requests. Plan your API calls accordingly to avoid dropped requests.
- Error Handling: Implement robust error handling in your scripts to manage potential issues gracefully. Refer to the Woodpecker API documentation for detailed error codes and troubleshooting tips.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to integrate seamlessly with your existing systems.
Enhancing Integration Capabilities with Endgrate
While integrating with Woodpecker is a powerful way to manage prospects, consider leveraging Endgrate for a more streamlined integration experience. Endgrate allows you to build once for each use case and connect to multiple platforms through a single API endpoint, saving time and resources. Focus on your core product while Endgrate handles the complexities of integration.
Explore how Endgrate can simplify your integration processes by visiting Endgrate and discover the benefits of a unified API solution.
Read More
Ready to get started?