How to Create or Update Leads with the Close API in PHP
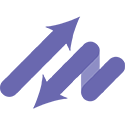
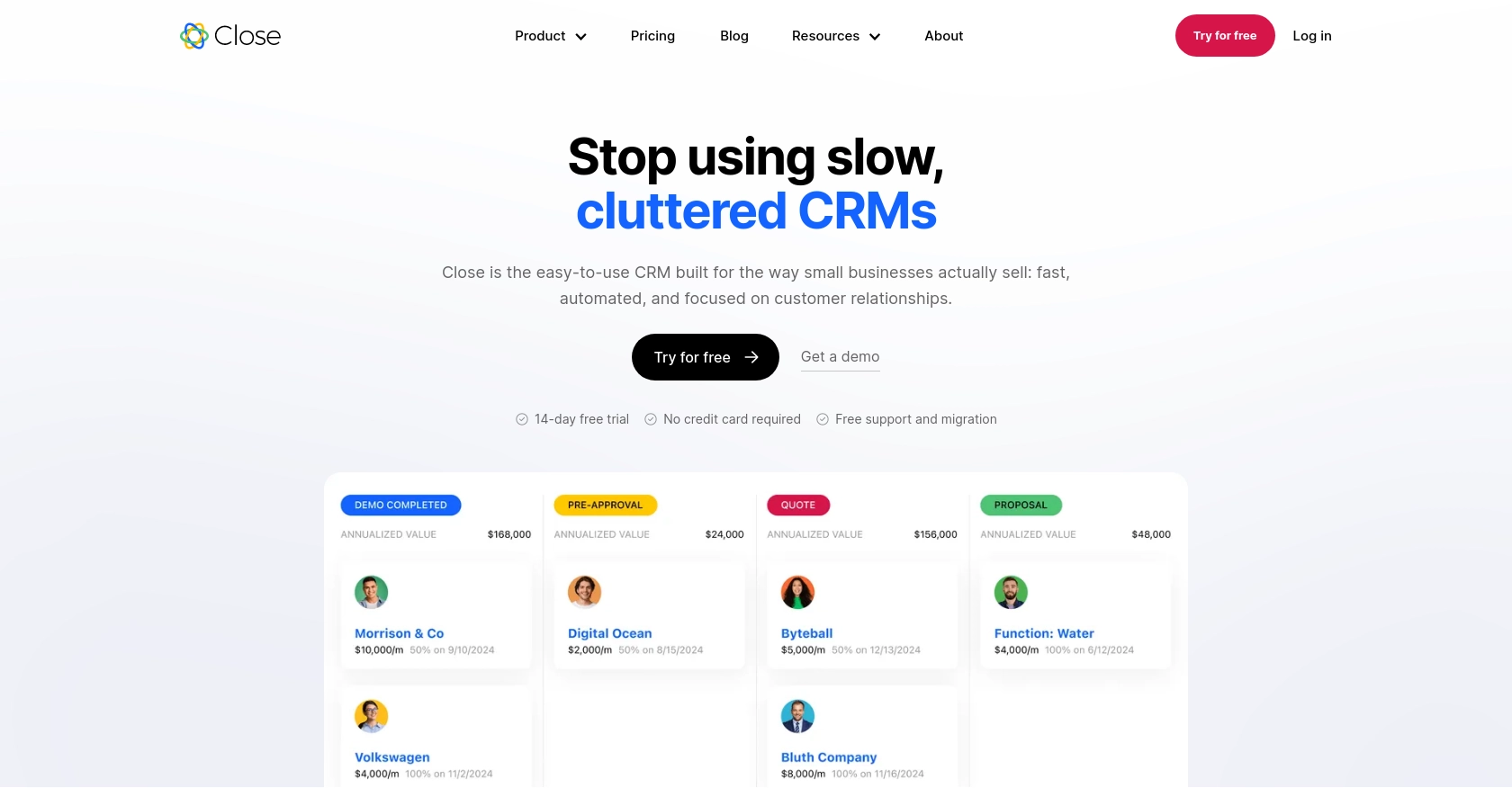
Introduction to Close CRM API
Close is a powerful CRM platform designed to help businesses streamline their sales processes and improve customer relationship management. With its robust set of features, Close enables teams to manage leads, contacts, and sales activities efficiently.
Integrating with the Close API allows developers to automate and enhance CRM functionalities, such as creating or updating leads programmatically. For example, a developer might use the Close API to automatically update lead information from an external data source, ensuring that the sales team always has the most current data at their fingertips.
Setting Up Your Close CRM Test Account
Before you can start integrating with the Close API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create a Close CRM Account
If you don't already have a Close account, you can sign up for a free trial on the Close website. This trial will give you access to all the features you need to test your integration.
- Visit the Close website and click on the "Try for Free" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Close dashboard.
Generate an API Key for Authentication
Close uses API keys for authentication, which are ideal for internal scripts and integrations. Follow these steps to generate your API key:
- Navigate to the "Settings" page in your Close dashboard.
- Under the "API Keys" section, click on "Generate API Key."
- Copy the generated API key and store it securely, as you'll need it for making API calls.
For more details, refer to the Close API Authentication Documentation.
Understanding API Key Authentication
Close API keys use HTTP Basic authentication. When making API requests, include an "Authorization" header with the word "Basic" followed by a space and a base64-encoded string of your API key followed by a colon. The API key acts as the username, and the password is left empty.
// Example of setting up the Authorization header in PHP
$apiKey = 'yourapikey:';
$authHeader = 'Authorization: Basic ' . base64_encode($apiKey);
For more information, visit the Close API Authentication Documentation.
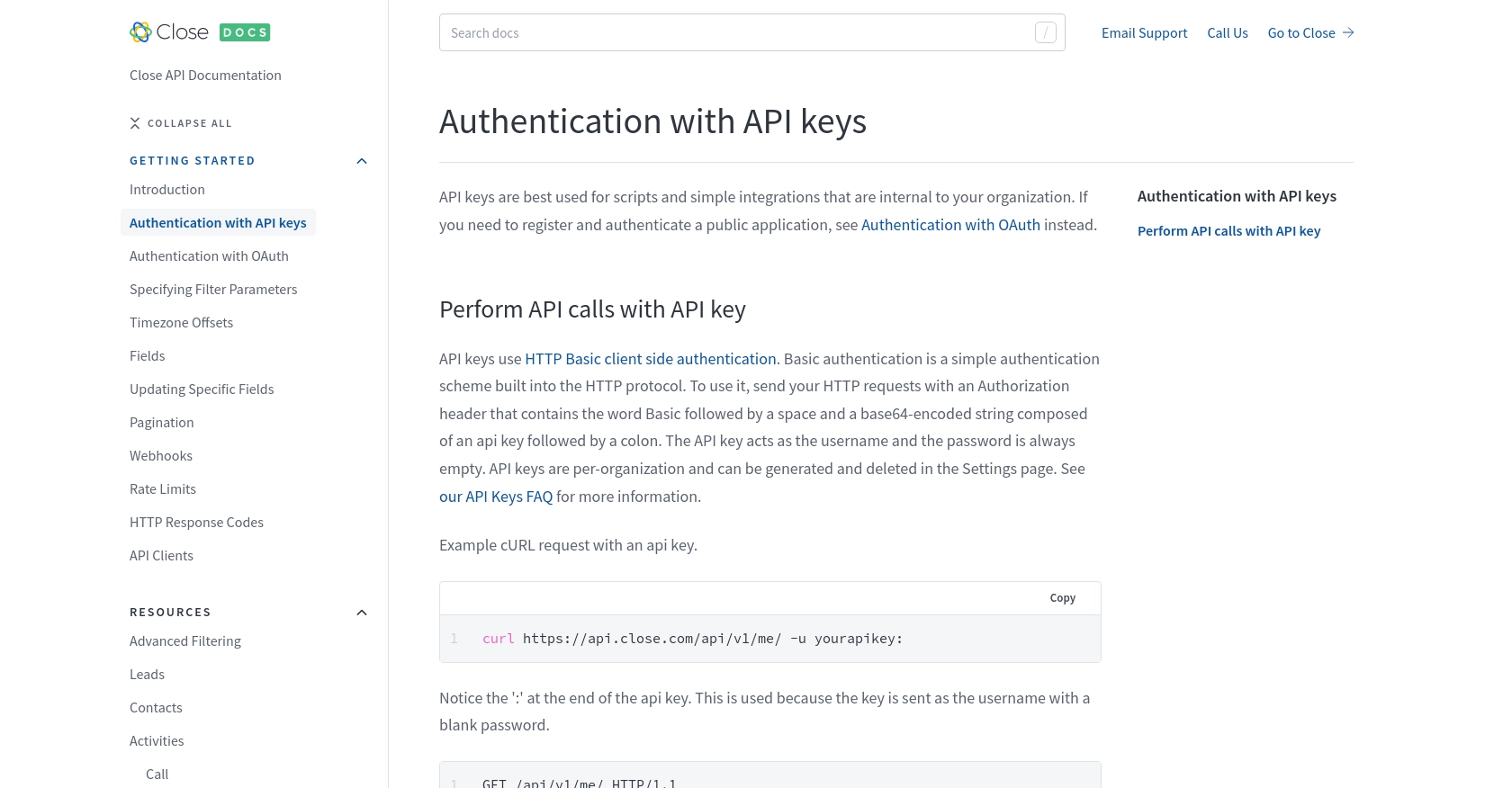
sbb-itb-96038d7
Making API Calls to Create or Update Leads in Close Using PHP
To interact with the Close API for creating or updating leads, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for Close API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- The
cURL
extension enabled
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Installing Dependencies for Close API Requests
You'll need to use the cURL
library to make HTTP requests in PHP. Ensure it is enabled in your php.ini
file:
extension=curl
Creating a New Lead with the Close API
To create a new lead, you'll send a POST request to the Close API endpoint. Here's an example of how to do this in PHP:
$apiKey = 'yourapikey:';
$authHeader = 'Authorization: Basic ' . base64_encode($apiKey);
$data = [
'name' => 'New Lead',
'contacts' => [
[
'name' => 'John Doe',
'emails' => [['email' => 'john.doe@example.com']]
]
]
];
$ch = curl_init('https://api.close.com/api/v1/lead/');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
$authHeader,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Replace yourapikey
with your actual API key. This code sends a POST request to create a new lead with a contact.
Updating an Existing Lead with the Close API
To update an existing lead, use a PUT request. Here's how you can update a lead's information:
$leadId = 'lead_id_here';
$apiKey = 'yourapikey:';
$authHeader = 'Authorization: Basic ' . base64_encode($apiKey);
$updateData = [
'name' => 'Updated Lead Name'
];
$ch = curl_init("https://api.close.com/api/v1/lead/$leadId/");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
$authHeader,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PUT');
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($updateData));
$response = curl_exec($ch);
curl_close($ch);
echo $response;
Ensure you replace lead_id_here
with the actual ID of the lead you wish to update.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. Check the HTTP status code to determine if the request was successful:
if ($response) {
$responseData = json_decode($response, true);
if (isset($responseData['id'])) {
echo "Lead operation successful. Lead ID: " . $responseData['id'];
} else {
echo "Error: " . $responseData['error'];
}
} else {
echo "Failed to connect to Close API.";
}
Refer to the Close API HTTP Response Codes Documentation for more details on handling different response codes.
Verifying API Call Success in Close Dashboard
After executing the API calls, verify the changes in your Close test account dashboard. Newly created or updated leads should reflect the changes made via the API.
For more information on API calls, visit the Close API Leads Documentation.
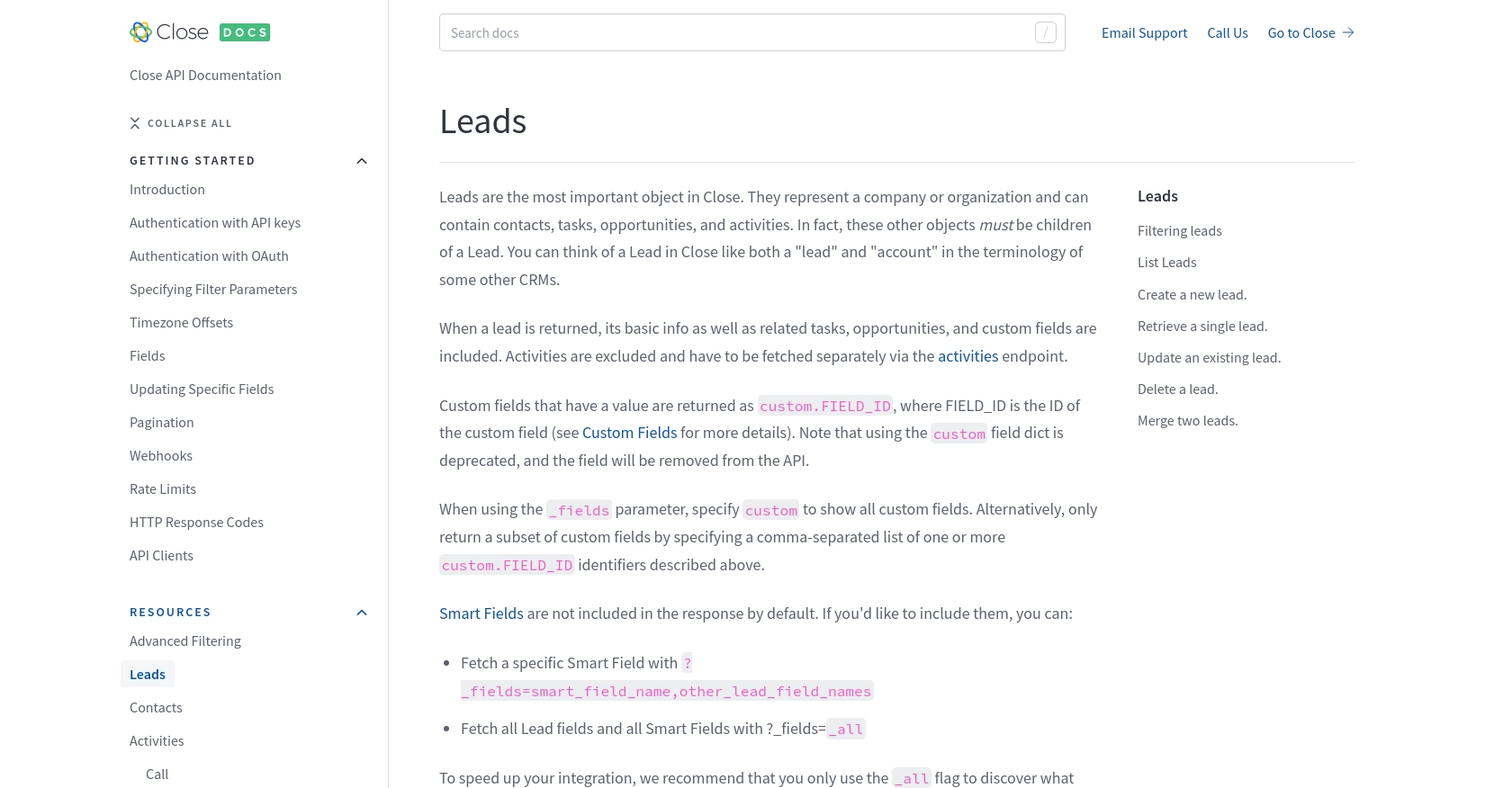
Best Practices for Using the Close API in PHP
When integrating with the Close API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store API Keys: Always store your API keys securely, preferably in environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be aware of Close's rate limits. If you receive a 429 status code, pause your requests for the duration specified in the
RateLimit
header. For more details, refer to the Close API Rate Limits Documentation. - Efficient Data Handling: Use pagination to handle large datasets efficiently. Refer to the Close API Pagination Documentation for guidance.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications.
Enhance Your Integration Experience with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Close. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration needs by visiting Endgrate.
Read More
- https://endgrate.com/provider/close
- https://developer.close.com/topics/authentication/
- https://developer.close.com/topics/authentication-oauth2/
- https://developer.close.com/topics/pagination/
- https://developer.close.com/topics/rate-limits/
- https://developer.close.com/topics/http-response-codes/
- https://developer.close.com/resources/leads/
Ready to get started?