How to Create or Update Companies with the Teamwork CRM API in Python
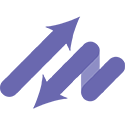
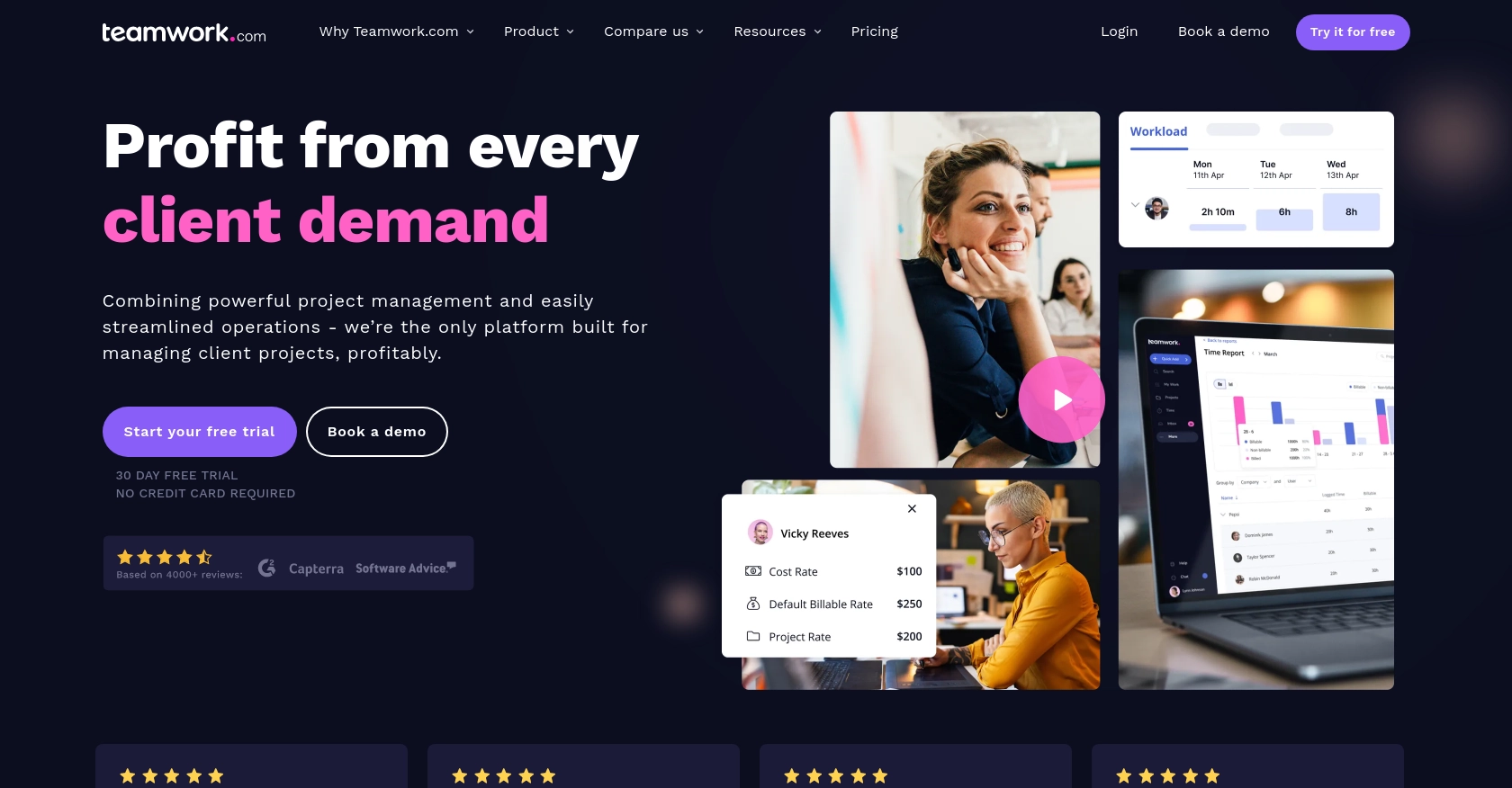
Introduction to Teamwork CRM API
Teamwork CRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. It offers a comprehensive suite of tools for tracking leads, managing customer interactions, and optimizing sales workflows. With its intuitive interface and powerful features, Teamwork CRM is a popular choice for businesses looking to enhance their customer relationship management.
Integrating with the Teamwork CRM API allows developers to automate and streamline various CRM tasks, such as creating or updating company records. For example, a developer might use the API to automatically update company information in Teamwork CRM based on data changes in another system, ensuring that all customer data remains consistent and up-to-date across platforms.
Setting Up a Teamwork CRM Test or Sandbox Account
Before you can start integrating with the Teamwork CRM API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Teamwork CRM Account
If you don't already have a Teamwork CRM account, you can sign up for a free trial on the Teamwork CRM website. This will give you access to all the features you need to test the API integration.
- Visit the Teamwork CRM website and click on the "Start Free Trial" button.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your new Teamwork CRM account.
Generate API Credentials for Teamwork CRM
To authenticate API requests, you'll need to generate API credentials. Teamwork CRM supports both Basic Authentication and OAuth 2.0. For this tutorial, we'll focus on Basic Authentication.
- Navigate to the "Settings" section of your Teamwork CRM account.
- Find the "API & Webhooks" section and click on it.
- Generate an API key by following the on-screen instructions.
- Store your API key securely, as you'll need it to authenticate your API requests.
Set Up OAuth 2.0 (Optional)
If you prefer using OAuth 2.0 for authentication, you'll need to register your application in the Teamwork Developer Portal:
- Visit the Teamwork Developer Portal.
- Register your application to obtain a client ID and client secret.
- Implement the OAuth 2.0 flow in your application to obtain an access token.
- Use the access token in the Authorization header of your API requests.
For more details on authentication, refer to the Teamwork CRM Authentication Documentation.
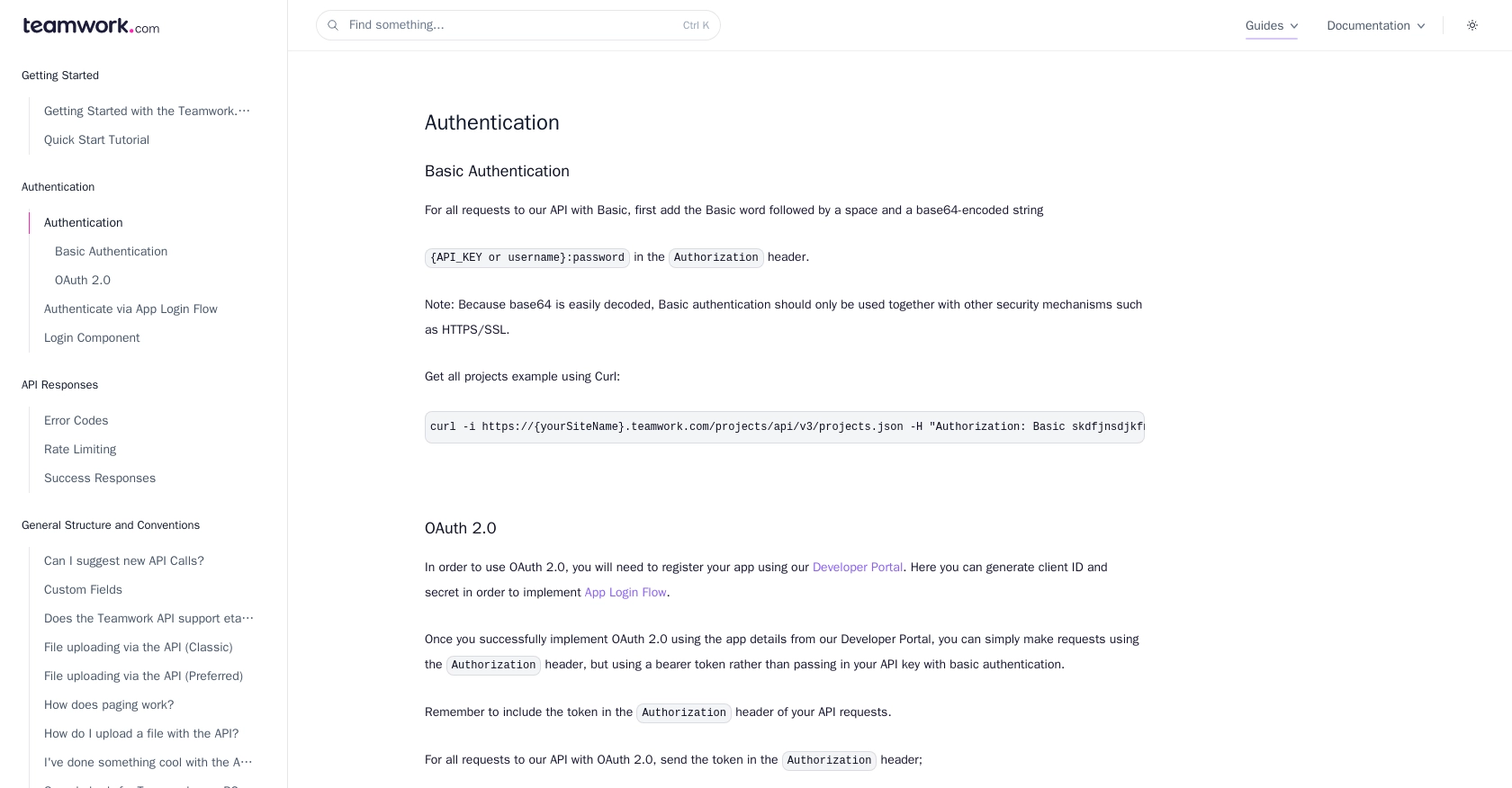
sbb-itb-96038d7
Making API Calls to Create or Update Companies in Teamwork CRM Using Python
To interact with the Teamwork CRM API for creating or updating company records, you'll need to use Python. This section will guide you through the process, including setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your Python Environment for Teamwork CRM API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Next, install the requests
library, which will help you make HTTP requests:
pip install requests
Creating or Updating Companies with Teamwork CRM API
Now, let's write the Python code to create or update a company in Teamwork CRM. We'll use the requests
library to send HTTP requests to the API.
import requests
import base64
# Set your Teamwork CRM API credentials
api_key = 'your_api_key'
site_name = 'yourSiteName'
# Encode the API key for Basic Authentication
auth_header = base64.b64encode(f'{api_key}:'.encode()).decode()
# Define the API endpoint for creating or updating a company
url = f'https://{site_name}.teamwork.com/crm/v2/companies.json'
# Set the request headers
headers = {
'Authorization': f'Basic {auth_header}',
'Content-Type': 'application/json'
}
# Define the company data
company_data = {
"company": {
"name": "New Company Name",
"address": "1234 Example St",
"city": "Example City",
"state": "Example State",
"zip": "12345"
}
}
# Send the request to create or update the company
response = requests.post(url, json=company_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Company created successfully.")
elif response.status_code == 200:
print("Company updated successfully.")
else:
print(f"Failed to create or update company. Status code: {response.status_code}")
print("Response:", response.json())
Replace your_api_key
and yourSiteName
with your actual API key and site name. The code above sets up the necessary headers for authentication and sends a POST request to the Teamwork CRM API to create or update a company.
Verifying API Call Success in Teamwork CRM
After running the code, you can verify the success of the API call by checking the response status code. A status code of 201
indicates successful creation, while 200
indicates a successful update. You can also log in to your Teamwork CRM account to confirm that the company record has been created or updated.
Handling Errors and Error Codes in Teamwork CRM API
When working with the Teamwork CRM API, you may encounter various error codes. Here are some common ones:
- 401 Unauthorized: Check your API key and ensure it's set up correctly.
- 422 Unprocessable Entity: Ensure the JSON data is correctly formatted.
- 429 Rate Limit Exceeded: The API allows up to 150 requests per minute for most plans. If you exceed this, you'll need to wait before making more requests.
For more details on error handling, refer to the Teamwork CRM Error Codes Documentation.
Conclusion and Best Practices for Teamwork CRM API Integration
Integrating with the Teamwork CRM API using Python can significantly enhance your ability to manage customer data efficiently. By automating the creation and updating of company records, you ensure that your CRM system remains consistent and up-to-date, reducing manual errors and saving valuable time.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Credentials: Always store your API keys and OAuth tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting Gracefully: Be mindful of the rate limits imposed by Teamwork CRM, which is 150 requests per minute for most plans. Implement retry logic with exponential backoff to handle HTTP 429 errors effectively.
- Data Transformation and Standardization: Ensure that data fields are transformed and standardized before sending them to the API. This helps maintain data integrity and consistency across systems.
- Error Handling and Logging: Implement robust error handling to capture and log API errors. This will aid in troubleshooting and ensure smooth operation of your integration.
Streamline Your Integrations with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Teamwork CRM, offering an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration efforts and enhance your product's capabilities.
Read More
Ready to get started?