How to Create or Update Companies with the FreshDesk API in PHP
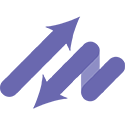
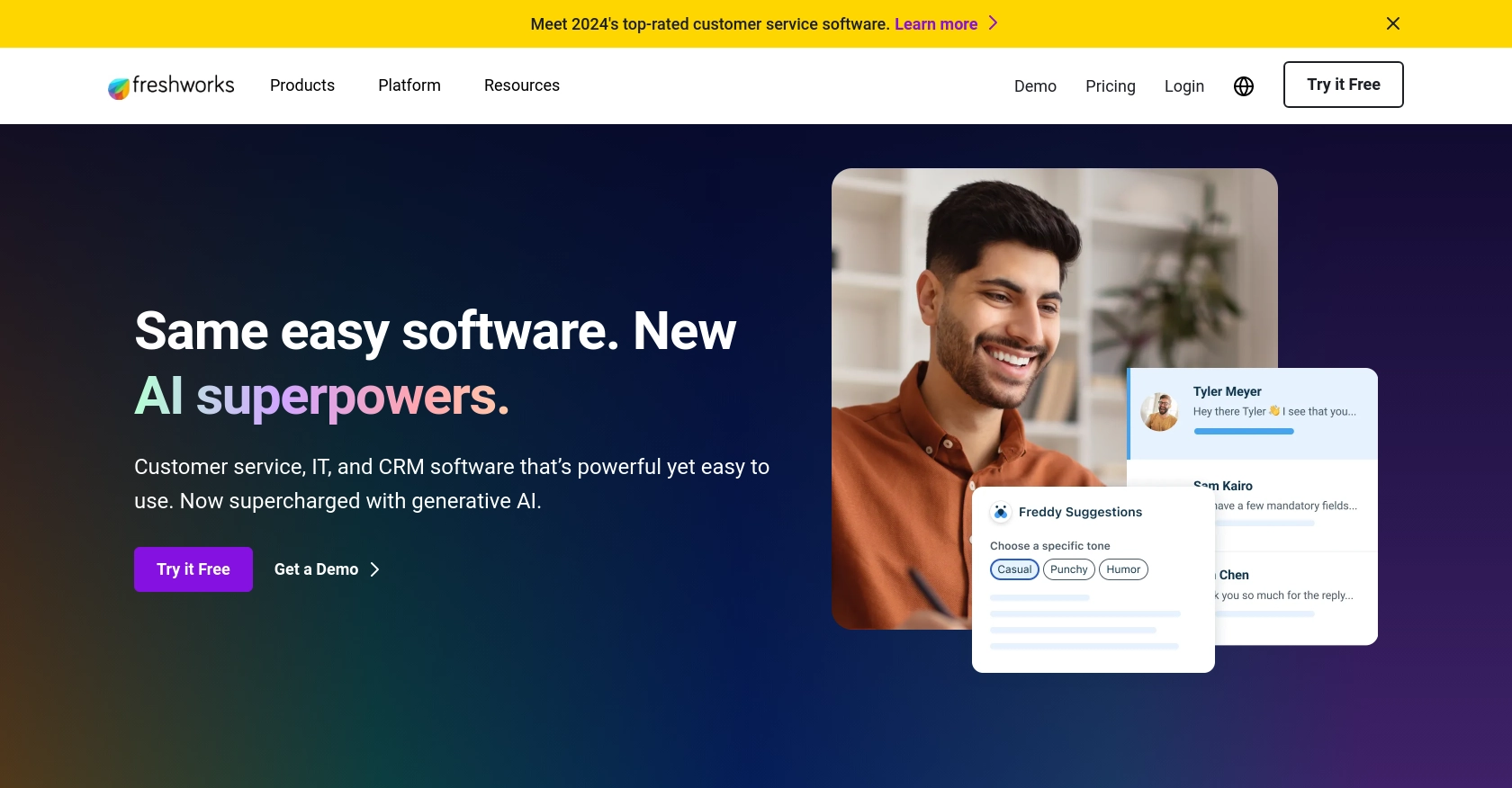
Introduction to FreshDesk API Integration
FreshDesk is a powerful customer support software that enables businesses to streamline their customer service operations. With features like ticketing, automation, and analytics, FreshDesk helps companies deliver exceptional customer experiences.
Developers may want to integrate with the FreshDesk API to automate and enhance customer support processes. For example, using the FreshDesk API, you can create or update company records directly from your application, ensuring that your customer data is always up-to-date and accessible.
This article will guide you through the process of creating or updating companies using the FreshDesk API with PHP, providing step-by-step instructions and code examples to help you efficiently manage company data within the FreshDesk platform.
Setting Up Your FreshDesk Test or Sandbox Account
Before you can start integrating with the FreshDesk API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. FreshDesk offers a free trial that you can use to access their API and test your integration.
Creating a FreshDesk Account
To get started, visit the FreshDesk sign-up page and create a new account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you'll have access to the FreshDesk dashboard where you can manage your customer support operations.
Generating API Key for FreshDesk Authentication
FreshDesk uses a custom authentication method that involves an API key. To generate your API key, follow these steps:
- Log in to your FreshDesk account.
- Navigate to your profile settings by clicking on your profile icon in the top right corner.
- Select "Profile Settings" from the dropdown menu.
- In the "API" section, you will find your API key. Copy this key and store it securely, as you will need it to authenticate your API requests.
Creating a FreshDesk App for API Access
To interact with the FreshDesk API, you may need to create an app within your FreshDesk account. This app will allow you to manage permissions and access specific API endpoints.
- Go to the "Admin" section in your FreshDesk dashboard.
- Under "Apps," click on "Create App."
- Fill in the required details, such as the app name and description.
- Set the necessary permissions for accessing and managing company data.
- Save your app settings to complete the setup.
With your FreshDesk account and API key ready, you can now proceed to make API calls to create or update companies using PHP. This setup ensures that you have the necessary credentials and permissions to interact with the FreshDesk API effectively.
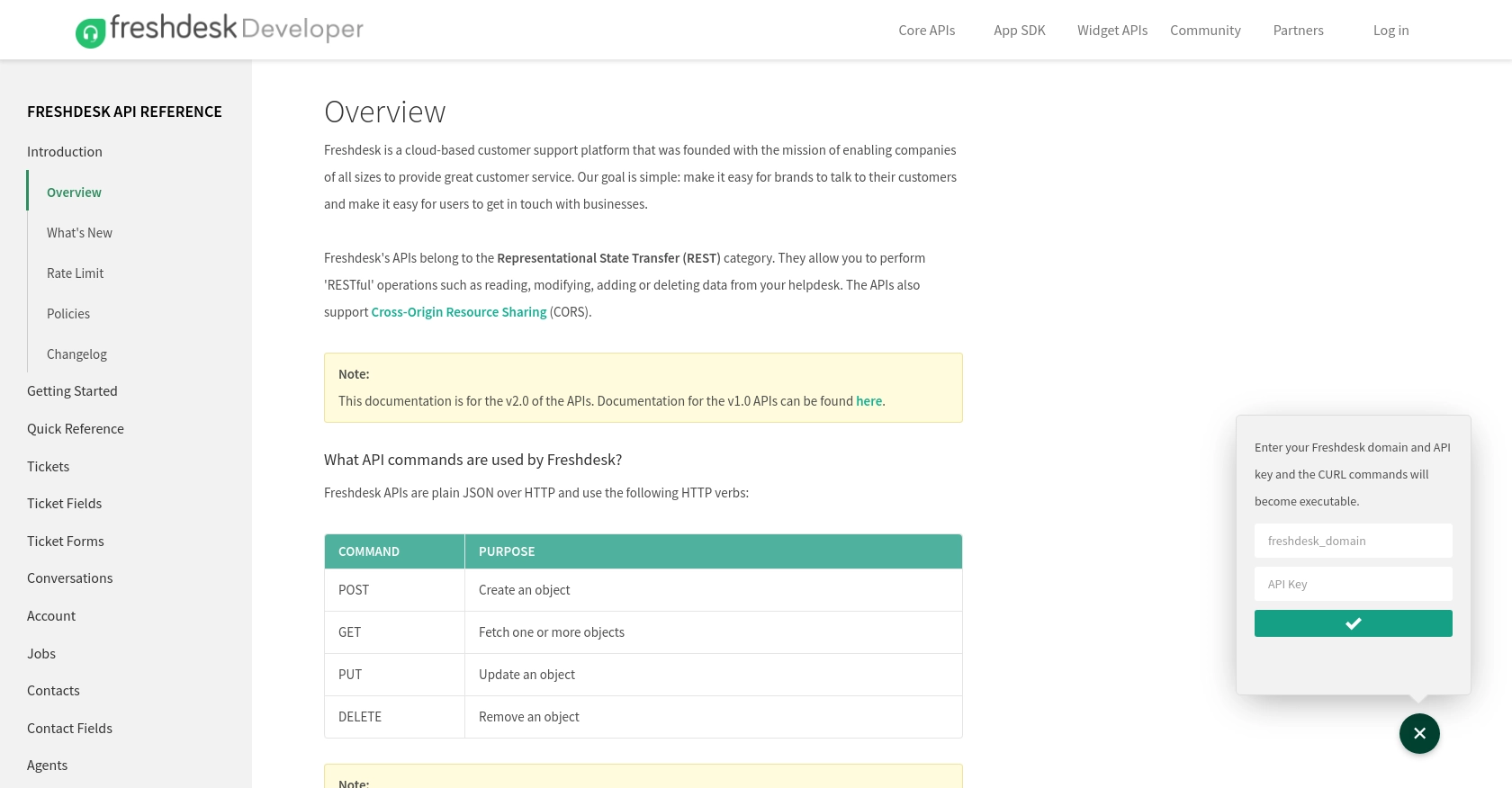
sbb-itb-96038d7
Making API Calls to Create or Update Companies with FreshDesk in PHP
To interact with the FreshDesk API using PHP, you'll need to make HTTP requests to the appropriate endpoints. This section will guide you through the process of creating or updating company records in FreshDesk using PHP, including setting up your environment and handling API responses.
Setting Up Your PHP Environment for FreshDesk API Integration
Before you begin, ensure that you have PHP installed on your machine. You can verify this by running the following command in your terminal:
php -v
Additionally, you'll need the cURL
extension enabled in PHP to make HTTP requests. You can check if it's enabled by running:
php -m | grep curl
If it's not enabled, you may need to install or enable it in your PHP configuration.
Creating a Company with the FreshDesk API Using PHP
To create a new company in FreshDesk, you'll need to make a POST request to the FreshDesk API endpoint for companies. Here's an example of how you can do this using PHP:
<?php
$api_key = 'your_api_key';
$domain = 'your_domain';
$url = "https://$domain.freshdesk.com/api/v2/companies";
$data = array(
"name" => "New Company",
"domains" => ["newcompany.com"],
"description" => "A description of the new company"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_USERPWD, "$api_key:X");
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array("Content-Type: application/json"));
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
$response = curl_exec($ch);
$status_code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($status_code == 201) {
echo "Company created successfully.";
} else {
echo "Failed to create company. Status code: $status_code";
}
curl_close($ch);
?>
Replace your_api_key
and your_domain
with your actual FreshDesk API key and domain. This script sends a POST request to create a new company with the specified details.
Updating a Company with the FreshDesk API Using PHP
To update an existing company, you'll need to make a PUT request to the FreshDesk API. Here's how you can update a company's information:
<?php
$api_key = 'your_api_key';
$domain = 'your_domain';
$company_id = 'company_id_to_update';
$url = "https://$domain.freshdesk.com/api/v2/companies/$company_id";
$data = array(
"name" => "Updated Company Name",
"domains" => ["updatedcompany.com"],
"description" => "Updated description"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_USERPWD, "$api_key:X");
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array("Content-Type: application/json"));
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
$response = curl_exec($ch);
$status_code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($status_code == 200) {
echo "Company updated successfully.";
} else {
echo "Failed to update company. Status code: $status_code";
}
curl_close($ch);
?>
Replace company_id_to_update
with the ID of the company you wish to update. This script sends a PUT request to update the company's details.
Handling API Responses and Errors
When making API calls, it's important to handle responses and potential errors. The FreshDesk API will return status codes indicating the success or failure of your request. Common status codes include:
- 200: Success for GET and PUT requests.
- 201: Success for POST requests.
- 400: Bad request, often due to invalid input.
- 401: Unauthorized, check your API key.
- 404: Not found, check the endpoint URL or resource ID.
Ensure you check the status code and handle errors appropriately in your application to provide a robust integration experience.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API using PHP allows developers to efficiently manage company data, enhancing customer support operations. By following the steps outlined in this article, you can create or update company records seamlessly within the FreshDesk platform.
Best Practices for Secure and Efficient FreshDesk API Usage
- Secure API Key Storage: Always store your FreshDesk API key securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of FreshDesk's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure consistent data formats when creating or updating companies to maintain data integrity across your systems.
- Error Handling: Implement robust error handling to manage API response codes effectively, providing clear feedback to users or logging errors for troubleshooting.
Streamlining Integrations with Endgrate
While integrating with FreshDesk is powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including FreshDesk.
By leveraging Endgrate, you can focus on your core product development while outsourcing integration tasks. This approach not only saves time and resources but also provides a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?