Using the Sap Business One API to Get Invoices in PHP
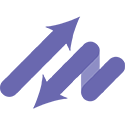
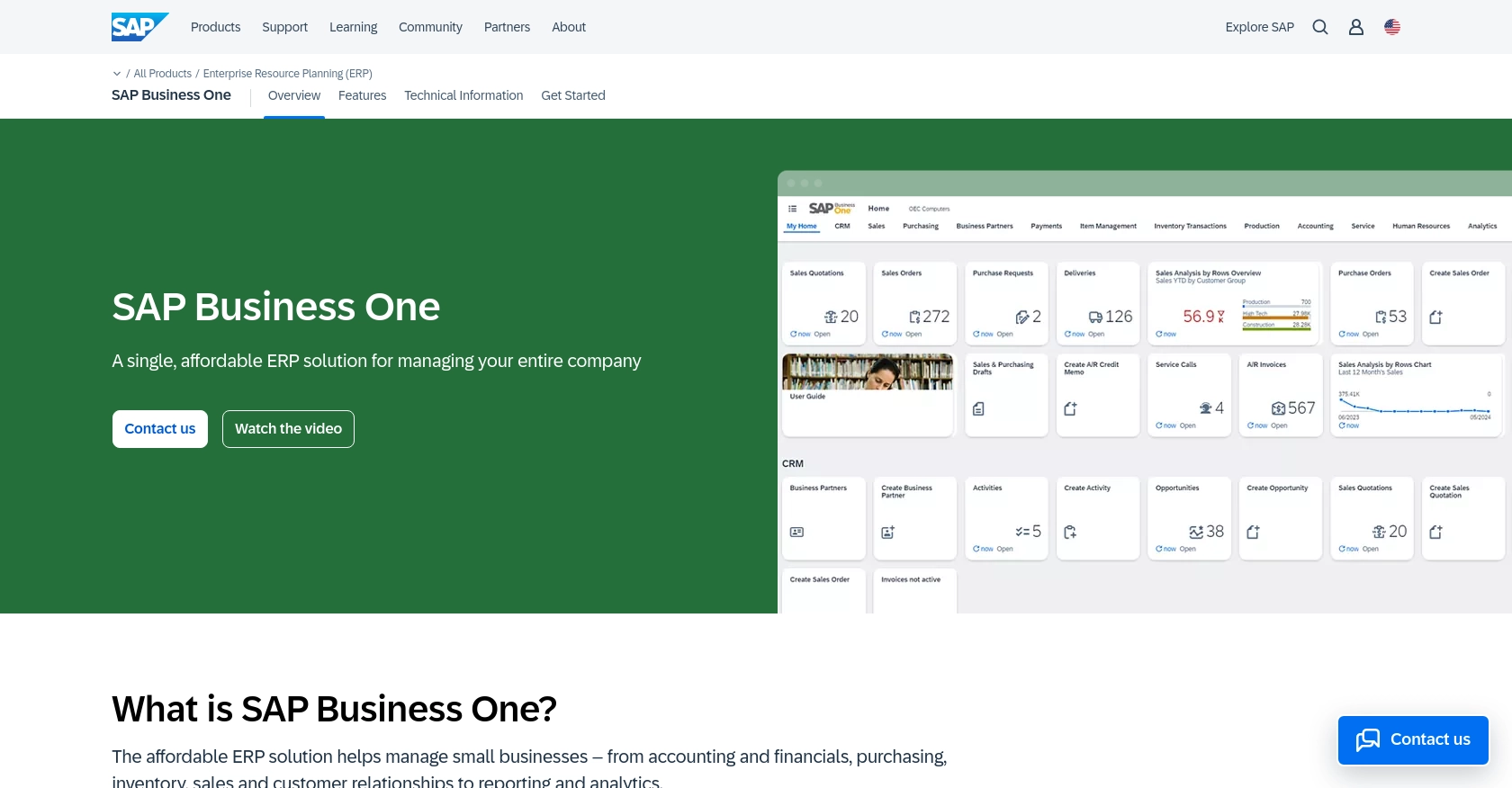
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed for small to mid-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all integrated into a single platform.
Developers often need to interact with SAP Business One's API to streamline business operations and enhance data accessibility. For example, retrieving invoices using the SAP Business One API in PHP can help automate financial reporting and improve the efficiency of accounting processes.
Setting Up Your SAP Business One Test/Sandbox Account
Before you begin integrating with the SAP Business One API, it's essential to set up a test or sandbox account. This environment allows you to safely test your API interactions without affecting live data.
Creating an SAP Business One Sandbox Account
To start, you'll need access to an SAP Business One sandbox environment. If you don't already have one, you can contact your SAP Business One provider to request access. Many providers offer trial or demo accounts specifically for development and testing purposes.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method for API access. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer settings, where you can manage API access.
- Create a new application to obtain your Client ID and Client Secret. These credentials are necessary for authenticating API requests.
- Set the appropriate permissions and scopes required for accessing invoice data.
For detailed guidance on setting up OAuth authentication, refer to the SAP Business One Service Layer documentation.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need an API key for certain operations. Here's how to generate it:
- Access the API management section within your SAP Business One sandbox account.
- Generate a new API key and note it down securely, as it will be used in your API requests.
With your sandbox account and authentication credentials set up, you're ready to start making API calls to retrieve invoices using PHP.
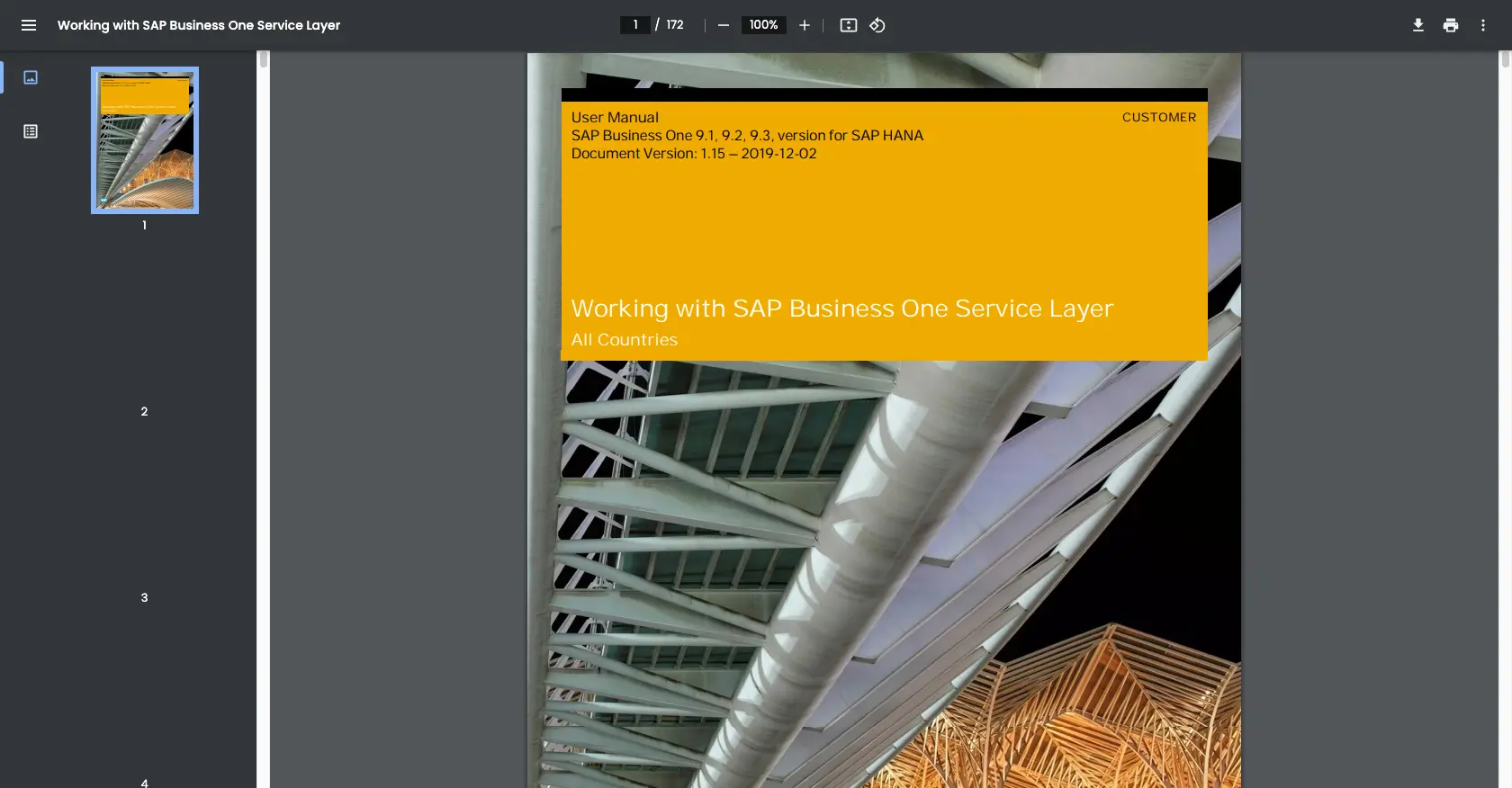
sbb-itb-96038d7
Making API Calls to Retrieve Invoices from SAP Business One Using PHP
To interact with the SAP Business One API and retrieve invoices, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment for SAP Business One API
Before you begin, ensure that you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer, the PHP package manager
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. You can install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Invoices from SAP Business One
Once your environment is set up, you can write the PHP code to make the API call. Create a new PHP file, for example, get_invoices.php
, and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Define the API endpoint and headers
$endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Invoices';
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer Your_Access_Token'
];
try {
// Make the GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Check if the request was successful
if ($response->getStatusCode() === 200) {
// Parse the JSON response
$invoices = json_decode($response->getBody(), true);
// Output the invoices
foreach ($invoices['value'] as $invoice) {
echo 'Invoice ID: ' . $invoice['DocEntry'] . "\n";
echo 'Invoice Total: ' . $invoice['DocTotal'] . "\n\n";
}
} else {
echo 'Failed to retrieve invoices. Status code: ' . $response->getStatusCode();
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the token you obtained during the authentication setup.
Verifying the API Call and Handling Errors
After running the script, you should see the list of invoices printed in your terminal. If the request fails, the script will output an error message with the status code or exception details.
To verify that the request succeeded, you can cross-check the retrieved invoice data with the records in your SAP Business One sandbox account.
Handling Common Errors and Error Codes
When interacting with the SAP Business One API, you may encounter various error codes. Here are some common ones and how to handle them:
- 401 Unauthorized: Ensure that your access token is valid and has not expired.
- 403 Forbidden: Check your API permissions and scopes to ensure you have access to the invoice data.
- 500 Internal Server Error: This may indicate an issue with the SAP Business One server. Try again later or contact support if the problem persists.
For more detailed error handling, refer to the SAP Business One Service Layer documentation.
Conclusion and Best Practices for Using SAP Business One API in PHP
Integrating with the SAP Business One API to retrieve invoices using PHP can significantly enhance your business's financial operations by automating data retrieval and improving efficiency. However, to ensure a smooth integration process, it's essential to follow best practices.
Best Practices for Secure and Efficient SAP Business One API Integration
- Securely Store Credentials: Always store your API keys and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Streamline Your Integration Process with Endgrate
While integrating with SAP Business One API can be rewarding, it can also be complex and time-consuming. Endgrate offers a solution to simplify this process by providing a unified API endpoint that connects to multiple platforms, including SAP Business One. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy and intuitive integration experience for your customers.
Explore how Endgrate can help you streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?