How to Get Invoices with the Zoho Books API in PHP
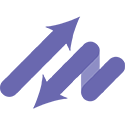
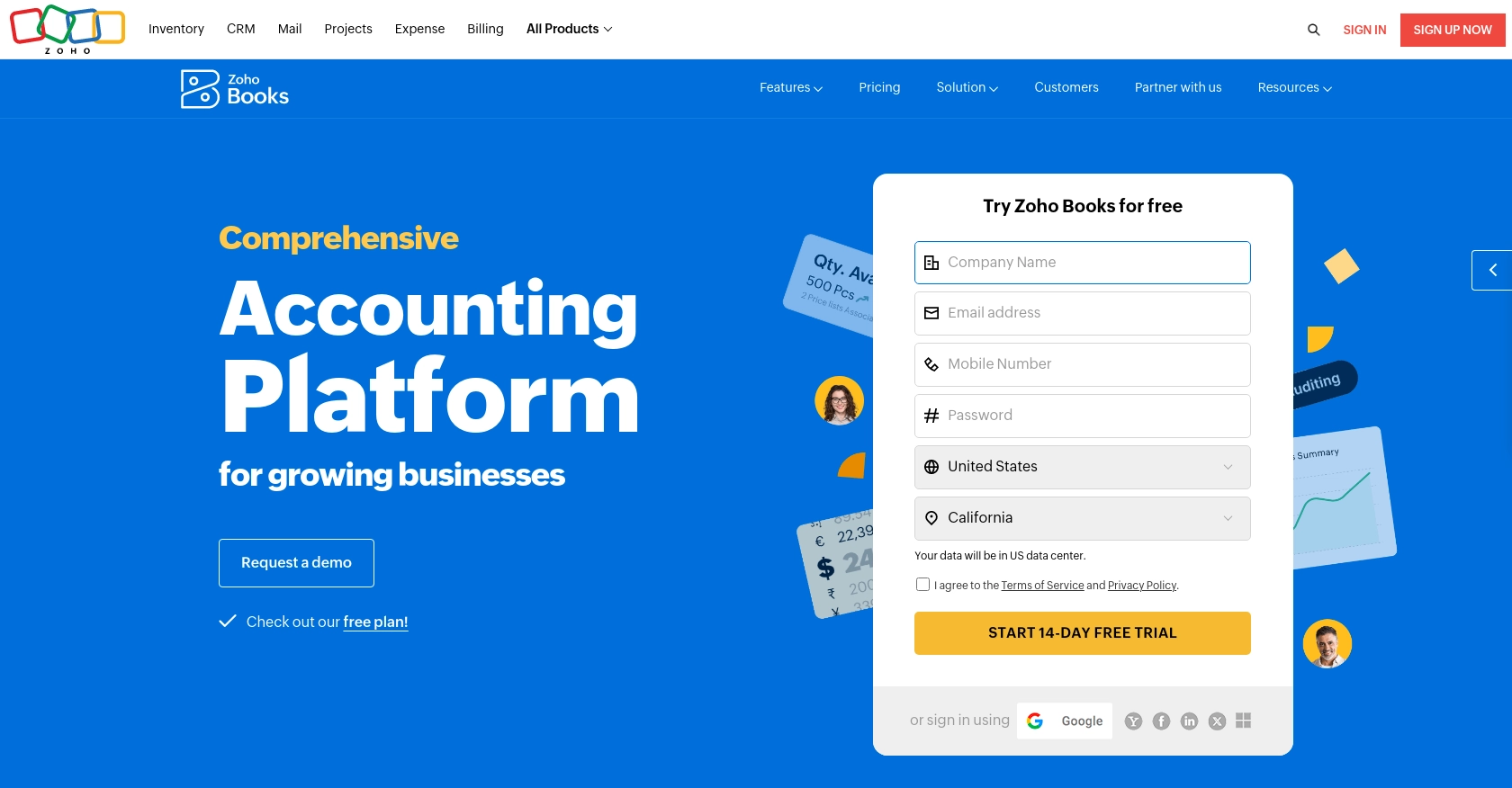
Introduction to Zoho Books
Zoho Books is a comprehensive online accounting software designed to streamline financial management for businesses. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it an ideal choice for small to medium-sized enterprises.
Integrating with the Zoho Books API allows developers to automate and enhance financial operations. For example, you can retrieve invoices programmatically to generate financial reports or integrate with other business systems. This capability is particularly useful for businesses looking to maintain accurate financial records and improve operational efficiency.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start interacting with the Zoho Books API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the instructions to create your account. Once your account is set up, you can access the Zoho Books dashboard.
Registering a New Client in Zoho's Developer Console
To use the Zoho Books API, you need to register your application in Zoho's Developer Console to obtain your Client ID and Client Secret.
- Visit the Zoho Developer Console.
- Click on Add Client ID and provide the necessary details to register your application.
- Upon successful registration, you will receive your OAuth 2.0 credentials, including the Client ID and Client Secret. Keep these credentials secure.
Generating OAuth Tokens for Zoho Books API
Zoho Books uses OAuth 2.0 for authentication. Follow these steps to generate the necessary tokens:
Step 1: Generate a Grant Token
Redirect users to the following URL to obtain a grant token:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.invoices.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
Replace YOUR_CLIENT_ID
and YOUR_REDIRECT_URI
with your actual client ID and redirect URI. After the user consents, Zoho will redirect to your specified URI with a code parameter.
Step 2: Exchange the Grant Token for Access and Refresh Tokens
Use the following POST request to exchange the grant token for access and refresh tokens:
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
In the response, you will receive both an access token and a refresh token. The access token is valid for a limited time, while the refresh token can be used to obtain new access tokens.
Step 3: Refresh the Access Token
When the access token expires, use the refresh token to obtain a new one:
https://accounts.zoho.com/oauth/v2/token?refresh_token=YOUR_REFRESH_TOKEN&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&grant_type=refresh_token
With these tokens, you are now ready to make authenticated API calls to Zoho Books.
For more detailed information, refer to the Zoho Books OAuth documentation.
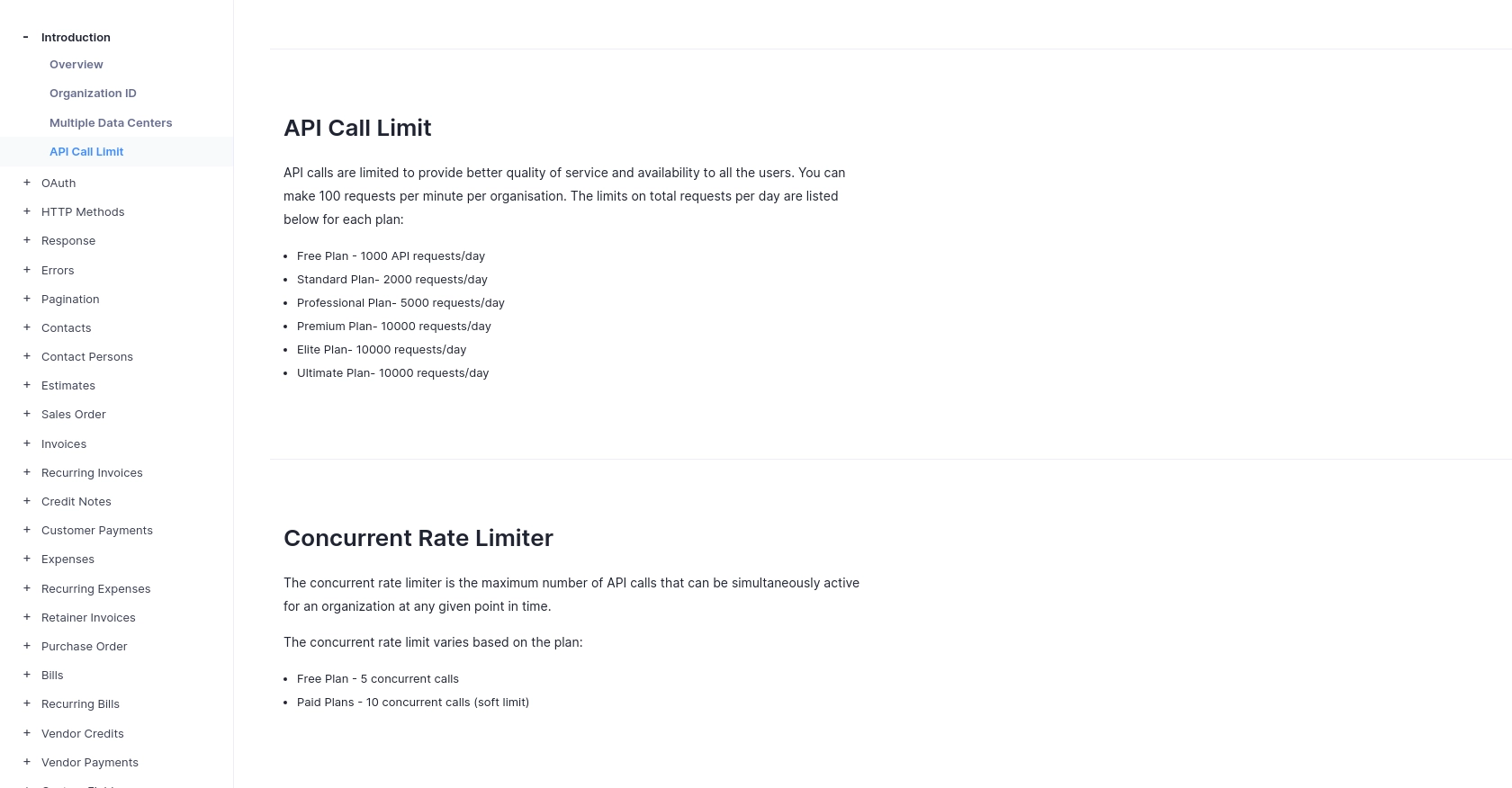
sbb-itb-96038d7
Making API Calls to Retrieve Invoices from Zoho Books Using PHP
In this section, we'll guide you through the process of making API calls to retrieve invoices from Zoho Books using PHP. This involves setting up your PHP environment, installing necessary dependencies, and executing the API request to fetch invoice data.
Setting Up Your PHP Environment for Zoho Books API
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or later
- Composer for dependency management
To install Composer, follow the instructions on the Composer website.
Installing Required PHP Libraries
To interact with the Zoho Books API, you'll need the Guzzle HTTP client. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Example Code to Fetch Invoices from Zoho Books
Now, let's write a PHP script to retrieve invoices from Zoho Books. Create a file named get_invoices.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$response = $client->request('GET', 'https://www.zohoapis.com/books/v3/invoices', [
'headers' => [
'Authorization' => 'Zoho-oauthtoken ' . $accessToken,
'Content-Type' => 'application/json'
],
'query' => [
'organization_id' => $organizationId
]
]);
$invoices = json_decode($response->getBody(), true);
foreach ($invoices['invoices'] as $invoice) {
echo 'Invoice Number: ' . $invoice['invoice_number'] . "\n";
echo 'Customer Name: ' . $invoice['customer_name'] . "\n";
echo 'Total: ' . $invoice['total'] . "\n\n";
}
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID.
Understanding the API Response
The API response will include a list of invoices. Each invoice contains details such as the invoice number, customer name, and total amount. You can modify the script to handle additional fields as needed.
Handling Errors and Verifying API Calls
To ensure your API calls are successful, check the HTTP status code of the response. A status code of 200 indicates success. Handle errors by catching exceptions and checking for error codes such as 400 (Bad Request) or 401 (Unauthorized).
For more details on error codes, refer to the Zoho Books API error documentation.
Verifying Data in Zoho Books Sandbox
After executing the script, verify the retrieved data by cross-referencing it with the invoices listed in your Zoho Books sandbox account. This ensures the API call is fetching the correct information.
By following these steps, you can efficiently retrieve invoices from Zoho Books using PHP, enabling seamless integration with your business processes.
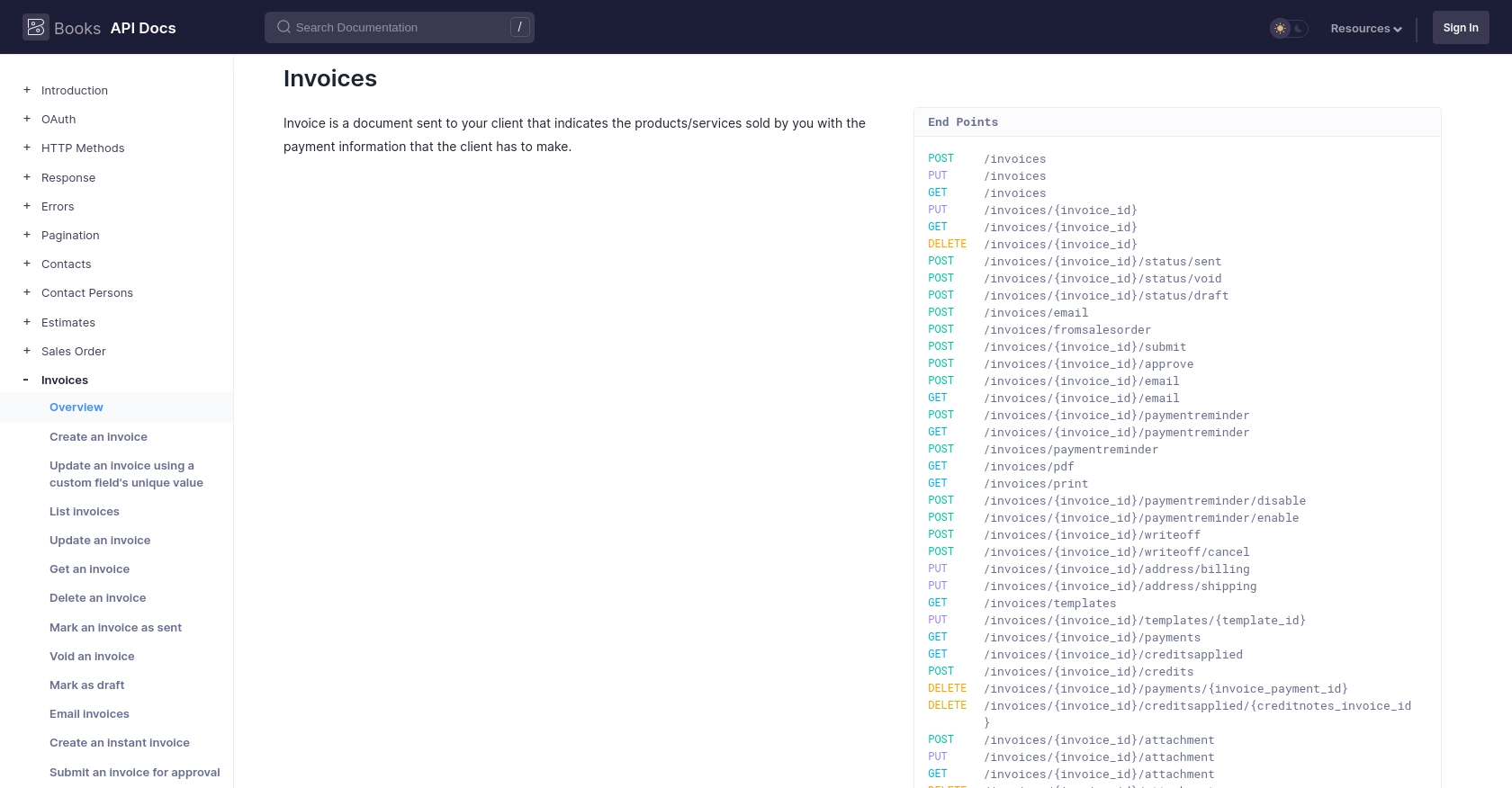
Best Practices for Using Zoho Books API in PHP
When integrating with the Zoho Books API, it's essential to follow best practices to ensure security and efficiency. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials, including the Client ID, Client Secret, and tokens, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Zoho Books API has rate limits of 100 requests per minute per organization. Implement logic to handle rate limit errors (HTTP status code 429) by retrying requests after a delay. For more details, refer to the Zoho Books API call limit documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across different systems you integrate with.
- Error Handling: Implement robust error handling by checking HTTP status codes and handling exceptions. Refer to the Zoho Books API error documentation for more information on error codes.
Enhance Your Integration with Endgrate
By leveraging Endgrate, you can simplify your integration processes with Zoho Books and other platforms. Endgrate offers a unified API endpoint that connects to multiple services, allowing you to build once and deploy across various integrations. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/invoices/#overview
Ready to get started?