Using the Google Docs API to Create Document Texts in PHP
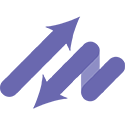
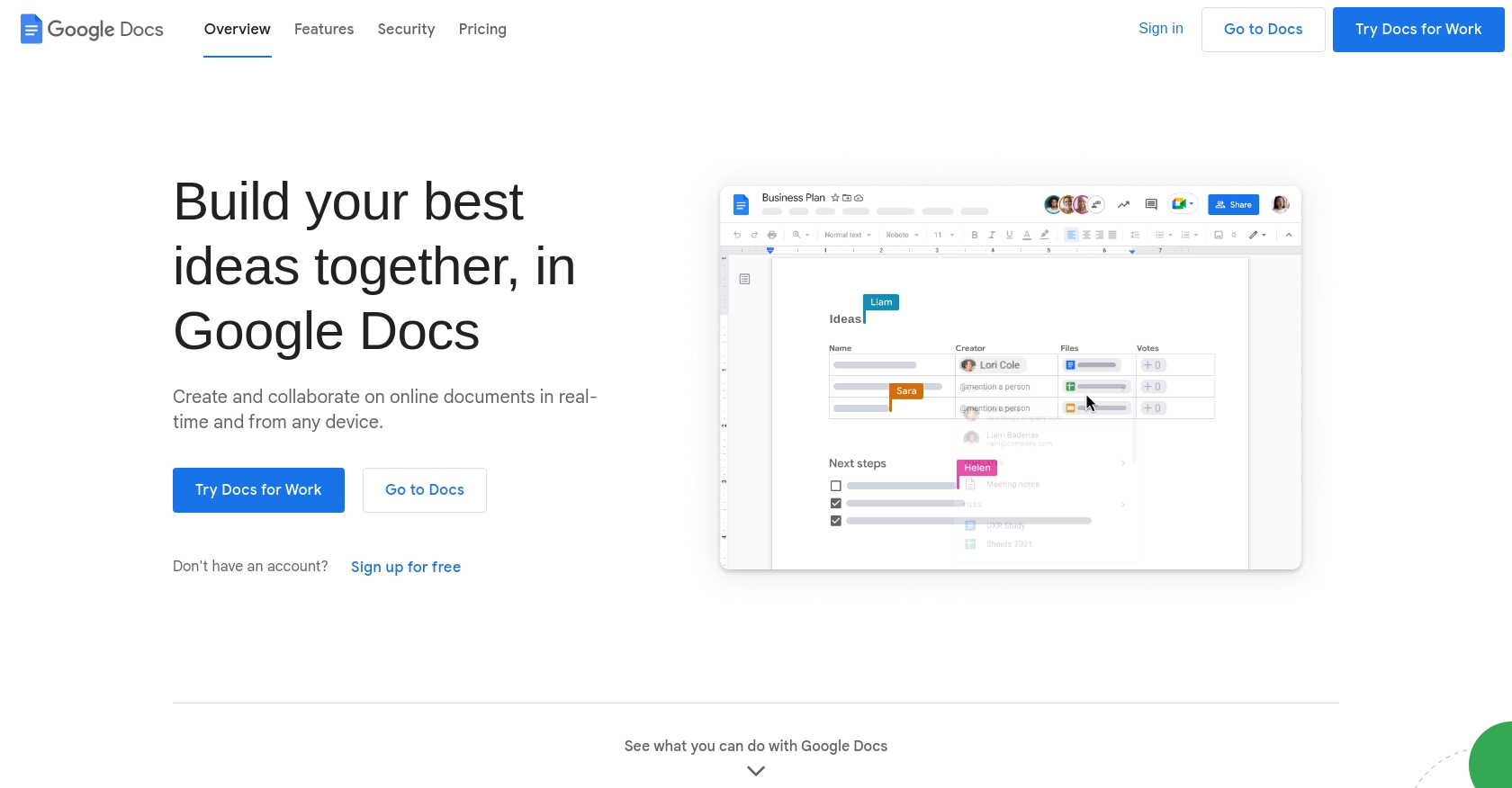
Introduction to Google Docs API
Google Docs is a widely used online word processor that allows users to create, edit, and collaborate on documents in real-time. It is part of the Google Workspace suite, offering seamless integration with other Google services like Google Drive, Sheets, and Slides. Its collaborative features and cloud-based accessibility make it a popular choice for businesses and individuals alike.
For developers, integrating with the Google Docs API opens up a world of possibilities for automating document creation and management. By using the API, developers can programmatically create, edit, and format documents, enabling them to build applications that enhance productivity and streamline workflows.
One practical use case for the Google Docs API is generating dynamic reports or documents based on data from other sources. For example, a developer could use the API to automatically generate a weekly report in Google Docs, pulling data from a database and formatting it into a structured document.
Setting Up Your Google Docs API Test Environment
Before you can start using the Google Docs API to create document texts in PHP, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup allows you to securely access the API and manage your documents programmatically.
Create a Google Cloud Project
To begin, you need a Google Cloud project. This project will serve as the foundation for enabling the Google Docs API and managing your credentials.
- Go to the Google Cloud Console.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable the Google Docs API
Once your project is created, you need to enable the Google Docs API to allow your application to interact with Google Docs.
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Docs API" and click on it.
- Click the Enable button.
Configure OAuth Consent Screen
Next, configure the OAuth consent screen to define what users will see when your application requests access to their Google Docs.
- In the Google Cloud Console, go to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields and click Save and Continue.
Create OAuth 2.0 Credentials
To authenticate your application, you need to create OAuth 2.0 credentials.
- In the Google Cloud Console, go to Menu > APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for the client ID and configure the authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
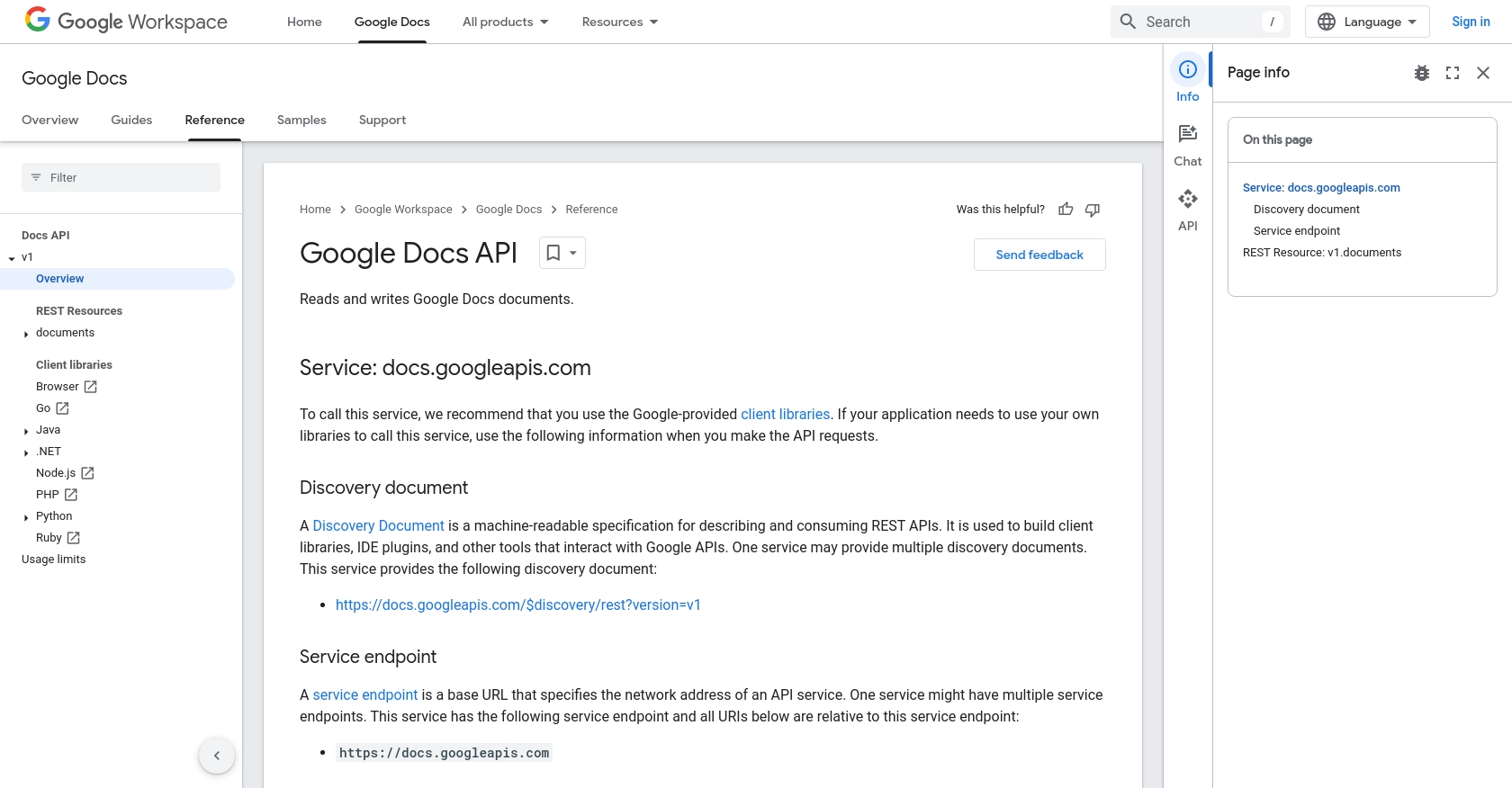
sbb-itb-96038d7
Making API Calls to Google Docs Using PHP
To interact with the Google Docs API using PHP, you'll need to set up your environment and write code that can send requests to the API. This section will guide you through the process of making API calls to create and manipulate document texts in Google Docs using PHP.
Setting Up Your PHP Environment
Before making API calls, ensure you have the necessary PHP version and dependencies installed. For this tutorial, you'll need:
- PHP 7.4 or later
- Composer for dependency management
- Google Client Library for PHP
Install the Google Client Library using Composer with the following command:
composer require google/apiclient:^2.0
Creating a Google Docs Document with PHP
To create a new Google Docs document, you'll use the documents.create
method. This method allows you to create a blank document with a specified title.
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\Docs;
// Initialize the Google Client
$client = new Client();
$client->setApplicationName('Google Docs API PHP Quickstart');
$client->setScopes(Google\Service\Docs::DOCUMENTS);
$client->setAuthConfig('credentials.json');
$client->setAccessType('offline');
// Initialize the Docs service
$service = new Docs($client);
// Create a new document
$document = new Google\Service\Docs\Document([
'title' => 'My New Document'
]);
$createdDocument = $service->documents->create($document);
echo 'Document ID: ' . $createdDocument->getDocumentId();
Replace 'credentials.json'
with the path to your OAuth 2.0 credentials file. This script initializes the Google Client and Docs service, then creates a new document titled "My New Document".
Inserting Text into a Google Docs Document
To insert text into a document, use the documents.batchUpdate
method with an InsertTextRequest
. This allows you to specify the text and location within the document.
$documentId = 'your-document-id';
$text = 'Hello, Google Docs!';
$requests = [
new Google\Service\Docs\Request([
'insertText' => [
'location' => ['index' => 1],
'text' => $text
]
])
];
$batchUpdateRequest = new Google\Service\Docs\BatchUpdateDocumentRequest([
'requests' => $requests
]);
$response = $service->documents->batchUpdate($documentId, $batchUpdateRequest);
echo 'Text inserted successfully.';
Replace 'your-document-id'
with the ID of the document you created. This code inserts the text "Hello, Google Docs!" at the beginning of the document.
Handling API Responses and Errors
After making an API call, it's important to handle responses and potential errors. Check the response status and handle any exceptions that may occur.
try {
$response = $service->documents->batchUpdate($documentId, $batchUpdateRequest);
echo 'Text inserted successfully.';
} catch (Exception $e) {
echo 'An error occurred: ' . $e->getMessage();
}
This code snippet demonstrates how to catch exceptions and display error messages, ensuring your application can handle issues gracefully.
For more detailed information on API calls, refer to the official documentation: Insert, delete, and move text.
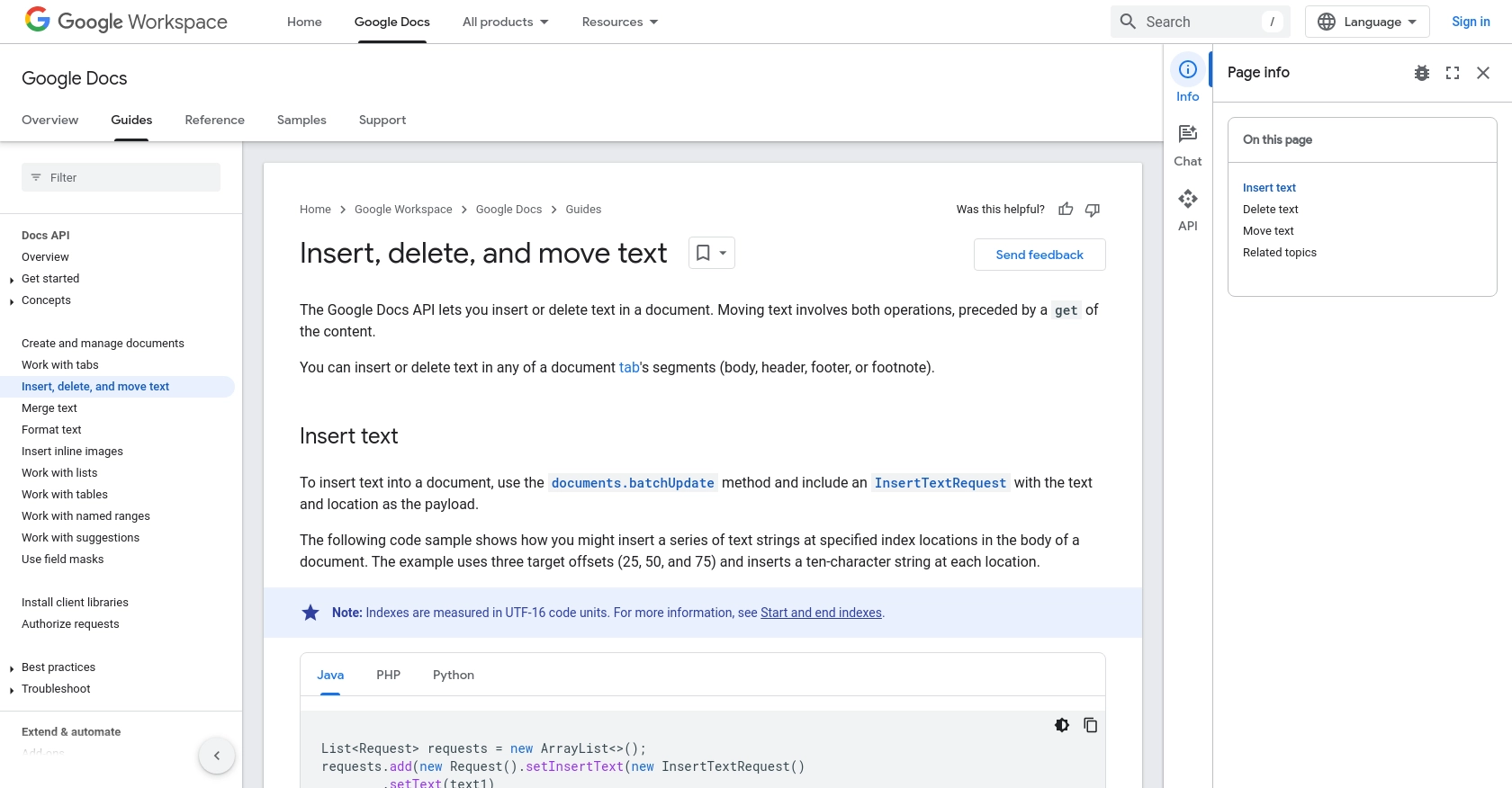
Conclusion and Best Practices for Using Google Docs API with PHP
Integrating the Google Docs API into your PHP applications can significantly enhance your ability to automate document creation and management. By following the steps outlined in this guide, you can efficiently create and manipulate Google Docs documents programmatically, streamlining workflows and boosting productivity.
Best Practices for Secure and Efficient Google Docs API Integration
- Securely Store Credentials: Always keep your OAuth 2.0 credentials secure. Avoid hardcoding them in your application and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the Google Docs API rate limits. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Optimize API Calls: Batch multiple requests into a single API call where possible to reduce the number of requests and improve performance.
- Validate API Responses: Always check the response status and handle errors appropriately to ensure your application remains robust and reliable.
Transforming and Standardizing Data for Google Docs
When inserting data into Google Docs, ensure that the data is properly formatted and standardized. This will help maintain consistency across documents and improve readability.
Leverage Endgrate for Seamless Integration
While integrating with the Google Docs API can be powerful, it can also be complex and time-consuming. Consider using Endgrate to simplify your integration processes. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration needs by visiting Endgrate.
Read More
- https://endgrate.com/provider/googledocs
- https://developers.google.com/docs/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/docs/api/how-tos/move-text
Ready to get started?