Using the Xero API to Get Sale Orders in PHP
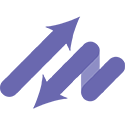
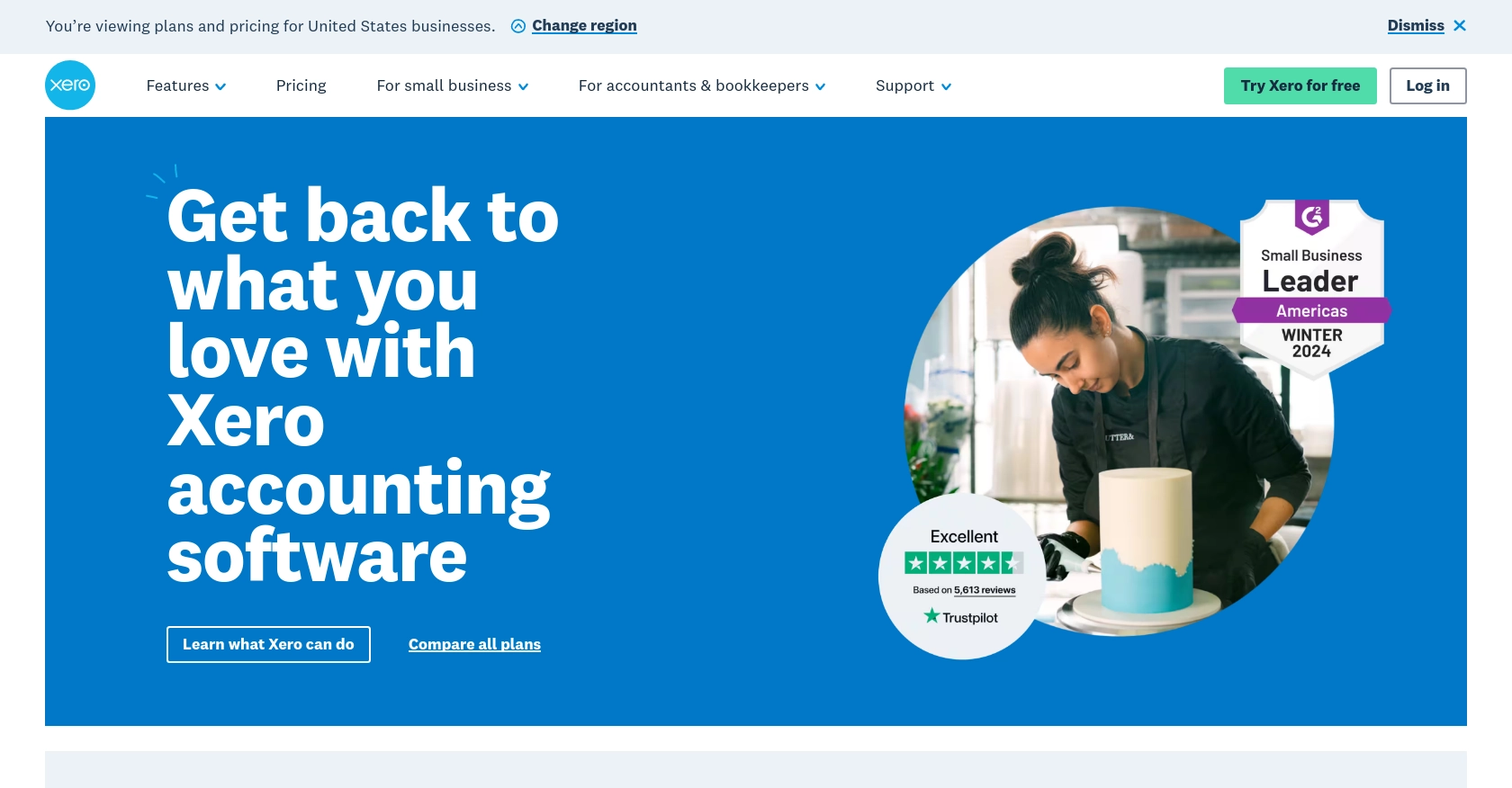
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform that caters to small and medium-sized businesses. It offers a wide range of features, including invoicing, payroll, expense management, and financial reporting, making it an essential tool for businesses looking to streamline their financial operations.
For developers, integrating with Xero's API can unlock numerous possibilities for automating and enhancing accounting processes. One common use case is retrieving sales orders to synchronize them with other business systems, such as inventory management or CRM platforms. This integration can help businesses maintain accurate records and improve operational efficiency.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Xero provides a demo company that you can use for this purpose.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, follow these steps:
- Visit the Xero Developer Portal and sign up for a free account.
- Once registered, log in to your account.
Accessing the Xero Demo Company
After logging in, you can access the demo company provided by Xero:
- Navigate to the "My Apps" section in the Xero Developer Portal.
- Select "Try the Demo Company" to access a pre-configured sandbox environment.
Creating a Xero App for OAuth Authentication
Since the Xero API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials:
- In the "My Apps" section, click on "New App."
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI to a valid endpoint where you can handle OAuth callbacks.
- Once created, note down the client ID and client secret, as you'll need these for authentication.
Configuring OAuth Scopes
To interact with sales orders, you'll need to configure the appropriate scopes:
- In your app settings, navigate to the "Scopes" section.
- Select the scopes relevant to sales orders, such as
accounting.transactions
andaccounting.settings
.
With your sandbox account and app set up, you're ready to start making API calls to Xero. This setup ensures that you can safely test your integration without impacting real data.
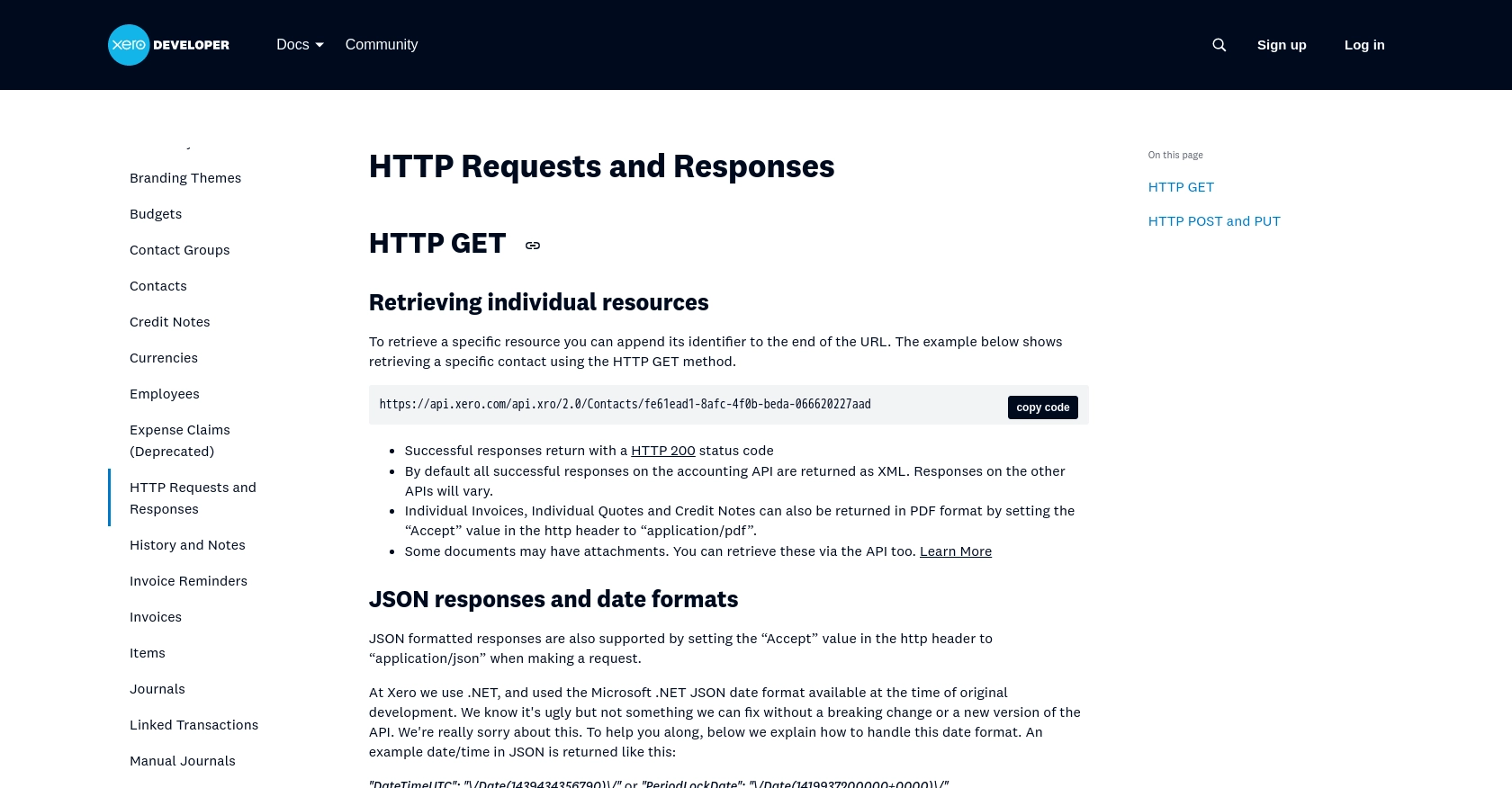
sbb-itb-96038d7
Making API Calls to Retrieve Xero Sales Orders Using PHP
To interact with the Xero API and retrieve sales orders, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your PHP Environment for Xero API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
You'll also need to install the guzzlehttp/guzzle
package, which simplifies making HTTP requests in PHP. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing PHP Code to Authenticate and Fetch Sales Orders from Xero
With your environment set up, you can now write the PHP code to authenticate with Xero and retrieve sales orders. Create a file named get_xero_sales_orders.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$token = 'Your_Access_Token'; // Replace with your actual access token
try {
$response = $client->request('GET', 'https://api.xero.com/api.xro/2.0/Orders', [
'headers' => [
'Authorization' => 'Bearer ' . $token,
'Accept' => 'application/json',
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['Orders'] as $order) {
echo 'Order ID: ' . $order['OrderID'] . '<br>';
echo 'Order Number: ' . $order['OrderNumber'] . '<br>';
echo 'Order Status: ' . $order['Status'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Replace Your_Access_Token
with the access token obtained during the OAuth authentication process. This code uses Guzzle to make a GET request to the Xero API endpoint for sales orders. It then parses the JSON response and prints out the details of each order.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the sales orders from your Xero demo company displayed. If the request is successful, the orders will match those in your sandbox account.
In case of errors, the script will catch exceptions and print an error message. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and has not expired.
- 403 Forbidden: Ensure you have the correct scopes configured for your app.
- 429 Too Many Requests: Xero's API rate limit is 60 requests per minute. Implement retry logic if you encounter this error.
For more details on error handling, refer to the Xero API documentation.
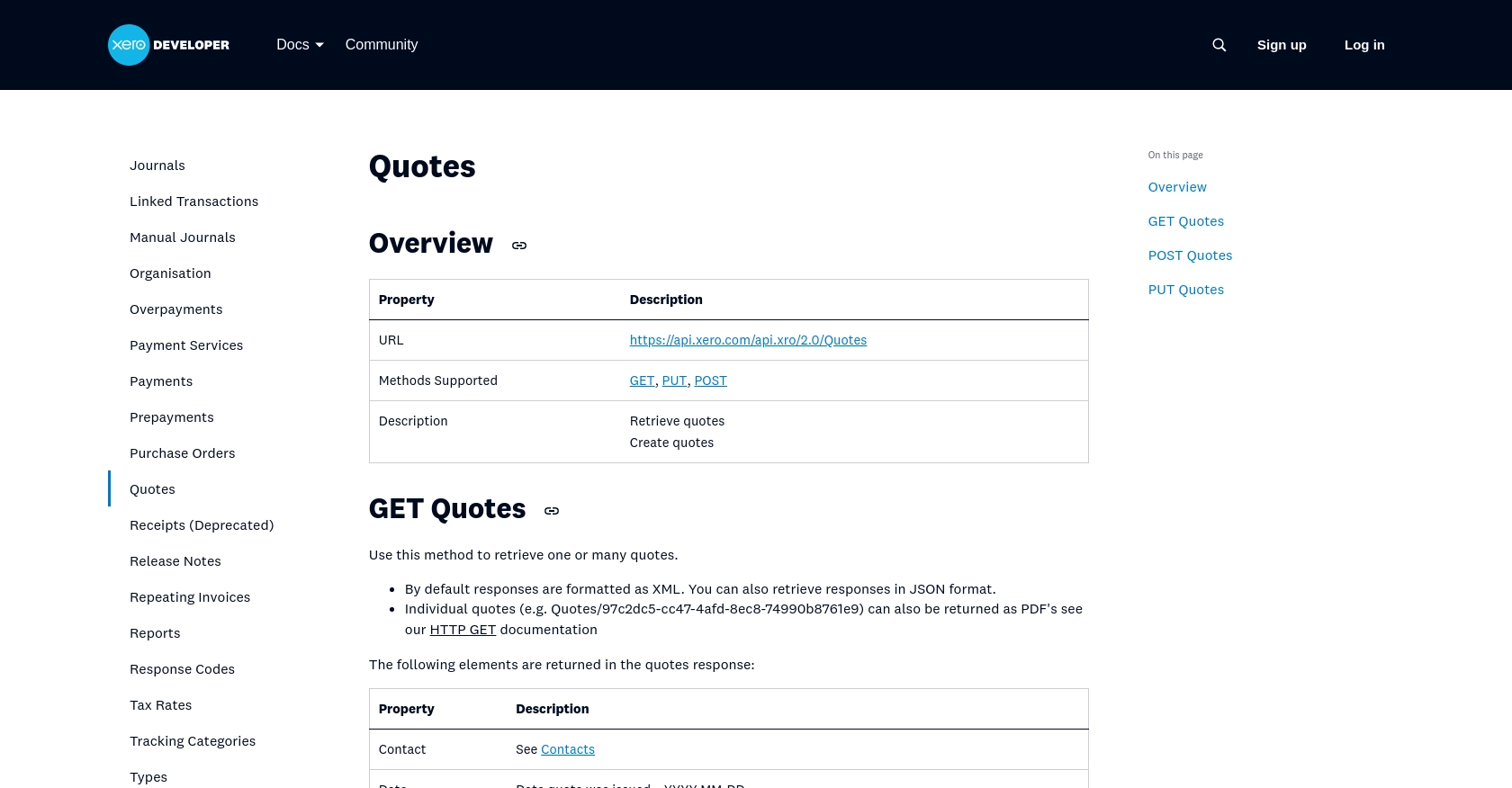
Best Practices for Xero API Integration and Error Handling
Successfully integrating with the Xero API requires attention to best practices, especially when handling sensitive data and potential errors. Here are some recommendations to ensure a smooth integration process:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Use environment variables or a secure vault to prevent unauthorized access.
- Implement Rate Limiting: Xero's API has a rate limit of 60 requests per minute. Implement retry logic with exponential backoff to handle
429 Too Many Requests
errors gracefully. For more details, refer to the Xero API rate limits documentation. - Handle Expired Tokens: OAuth tokens can expire. Implement logic to refresh tokens automatically to maintain seamless API access.
- Data Transformation and Standardization: Ensure that data retrieved from Xero is transformed and standardized to match your application's requirements. This will help maintain consistency across different systems.
Streamlining Xero API Integrations with Endgrate
Building and maintaining integrations with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Xero.
With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy and intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/quotes
Ready to get started?