How to Send Messages with the Outlook API in PHP
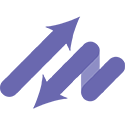
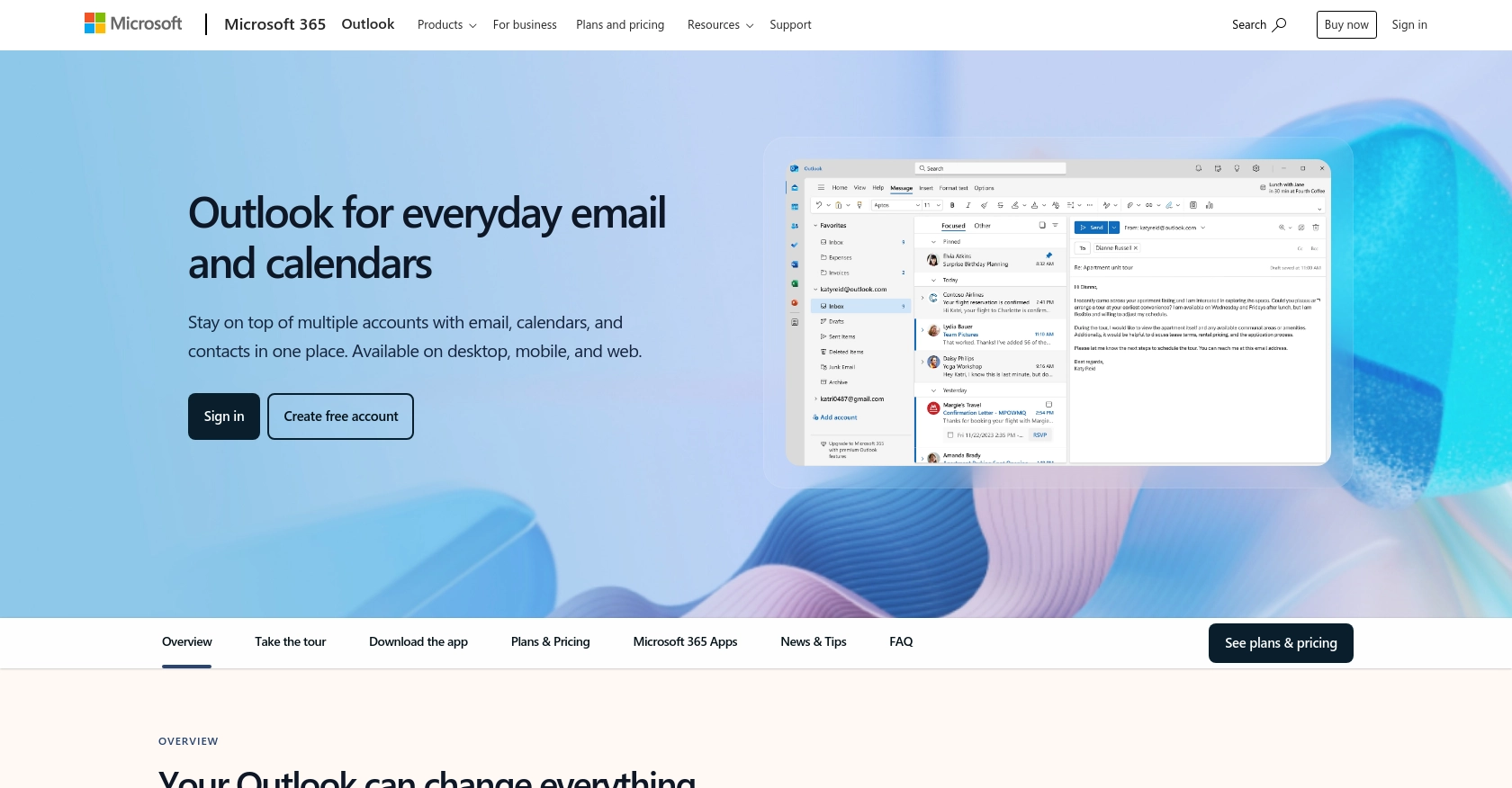
Introduction to Outlook API for Sending Emails
The Outlook API, part of the Microsoft Graph, provides developers with the ability to access and interact with Outlook mail data. This powerful API allows for seamless integration with Microsoft 365 services, enabling developers to send, receive, and manage emails programmatically.
Integrating with the Outlook API can significantly enhance the functionality of your applications by automating email processes. For example, a developer might want to send automated notifications or newsletters to users directly from their application, streamlining communication and improving user engagement.
In this article, we will explore how to send messages using the Outlook API with PHP, providing you with the necessary steps and code examples to get started.
Setting Up Your Outlook API Test/Sandbox Account
Before you can start sending messages with the Outlook API in PHP, you need to set up a test or sandbox account. This involves creating an application in the Microsoft Entra admin center, which will allow you to authenticate and interact with the Outlook API securely.
Step-by-Step Guide to Creating a Microsoft Entra Account
If you don't already have a Microsoft Entra account, follow these steps to create one:
- Visit the Microsoft Azure Portal and sign up for a free account if you don't have one.
- Once your account is set up, navigate to the Microsoft Entra admin center.
Registering Your Application in Microsoft Entra
To interact with the Outlook API, you need to register your application:
- Sign in to the Microsoft Entra admin center.
- Go to Identity > Applications > App registrations.
- Click on New registration.
- Enter a name for your application and select the appropriate account type.
- Click Register to complete the registration.
Configuring Authentication for Your Outlook API Application
After registering your application, you need to configure authentication settings:
- In the app registration page, go to Authentication under Manage.
- Click on Add a platform and select Web.
- Enter a redirect URI, which is where the Microsoft identity platform will send responses after authentication.
- Configure any additional settings as needed and click Configure.
Generating Client ID and Client Secret for OAuth Authentication
To authenticate with the Outlook API, you'll need a client ID and client secret:
- Navigate to Certificates & secrets under Manage.
- Click on New client secret and provide a description.
- Select an expiration period and click Add.
- Copy the client secret value immediately, as it will not be displayed again.
With your application registered and authentication configured, you are now ready to use the Outlook API to send messages programmatically using PHP. In the next section, we will cover how to make the actual API call.
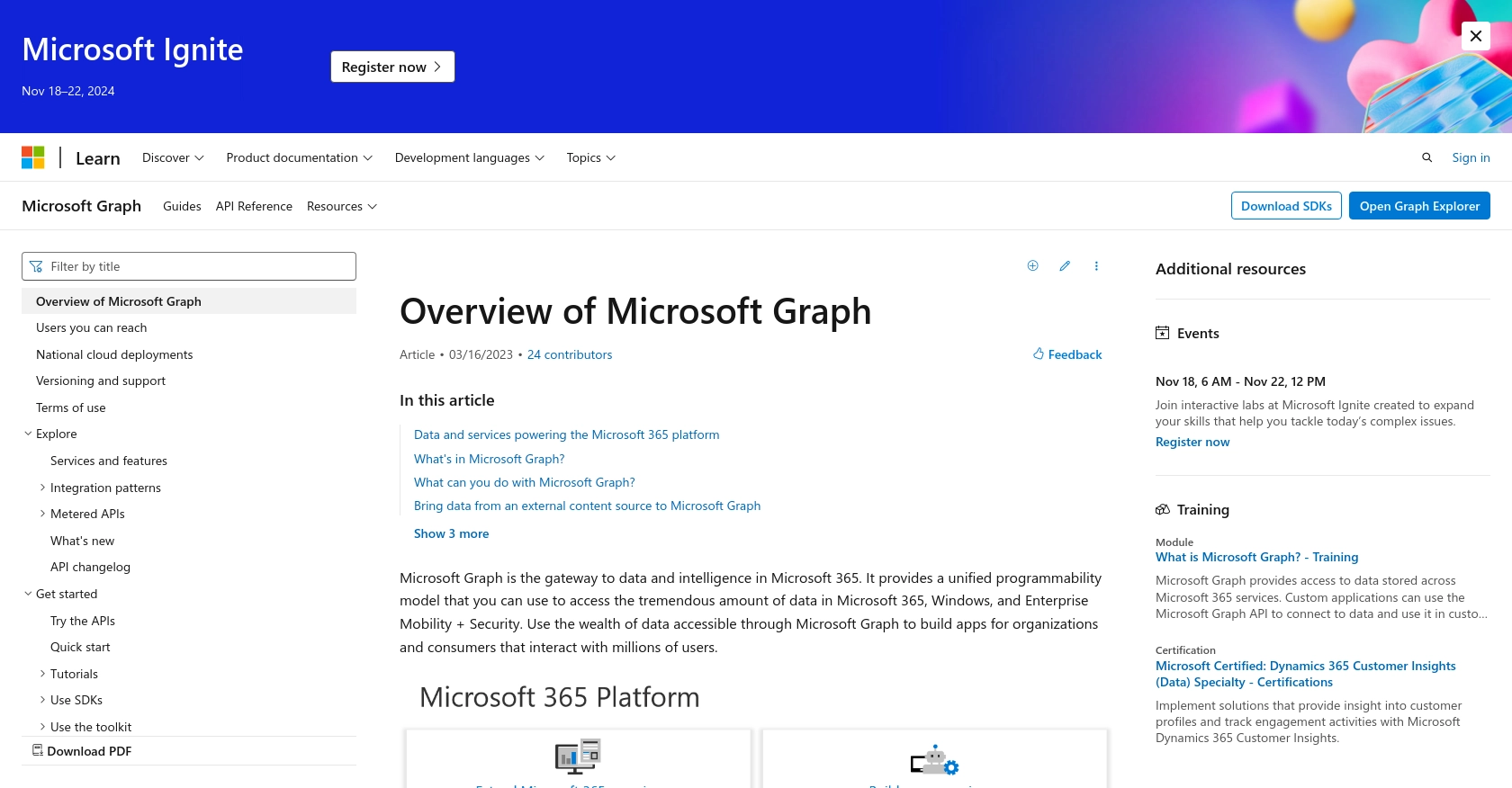
sbb-itb-96038d7
Making the Actual API Call to Send Emails with Outlook API in PHP
Now that you have set up your application and configured authentication, it's time to make the actual API call to send emails using the Outlook API in PHP. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling potential errors.
Setting Up Your PHP Environment for Outlook API Integration
Before you start coding, ensure your PHP environment is ready:
- Ensure you have PHP 7.4 or later installed on your machine.
- Install the required dependencies using Composer. You will need the Microsoft Graph SDK for PHP:
composer require microsoft/microsoft-graph
Writing the PHP Code to Send Emails Using Outlook API
With your environment set up, you can now write the PHP code to send an email:
use Microsoft\Graph\Graph; use Microsoft\Graph\Model\Message; use Microsoft\Graph\Model\ItemBody; use Microsoft\Graph\Model\BodyType; use Microsoft\Graph\Model\Recipient; use Microsoft\Graph\Model\EmailAddress; // Initialize the Graph client $graph = new Graph(); $graph->setAccessToken('YOUR_ACCESS_TOKEN'); // Create the email message $message = new Message(); $message->setSubject('Hello from PHP'); $body = new ItemBody(); $body->setContentType(BodyType::TEXT); $body->setContent('This is a test email sent using the Outlook API.'); $message->setBody($body); // Set the recipient $recipient = new Recipient(); $emailAddress = new EmailAddress(); $emailAddress->setAddress('recipient@example.com'); $recipient->setEmailAddress($emailAddress); $message->setToRecipients([$recipient]); // Send the email $graph->createRequest("POST", "/me/sendMail") ->attachBody(['message' => $message, 'saveToSentItems' => true]) ->execute();
Replace 'YOUR_ACCESS_TOKEN'
with the access token you obtained during authentication. This code initializes the Graph client, creates a message, sets the recipient, and sends the email using the Outlook API.
Verifying the Email Sending Process and Handling Errors
After running the code, verify that the email was sent successfully:
- Check the Sent Items folder in your Outlook account to confirm the email was sent.
- If the email does not appear, review the code for any errors or missing information.
Handle potential errors by catching exceptions in your code:
try { // Your API call here } catch (Exception $e) { echo 'Error: ', $e->getMessage(), "\n"; }
By wrapping your API call in a try-catch block, you can capture and display any errors that occur during the process, allowing you to debug and resolve issues efficiently.
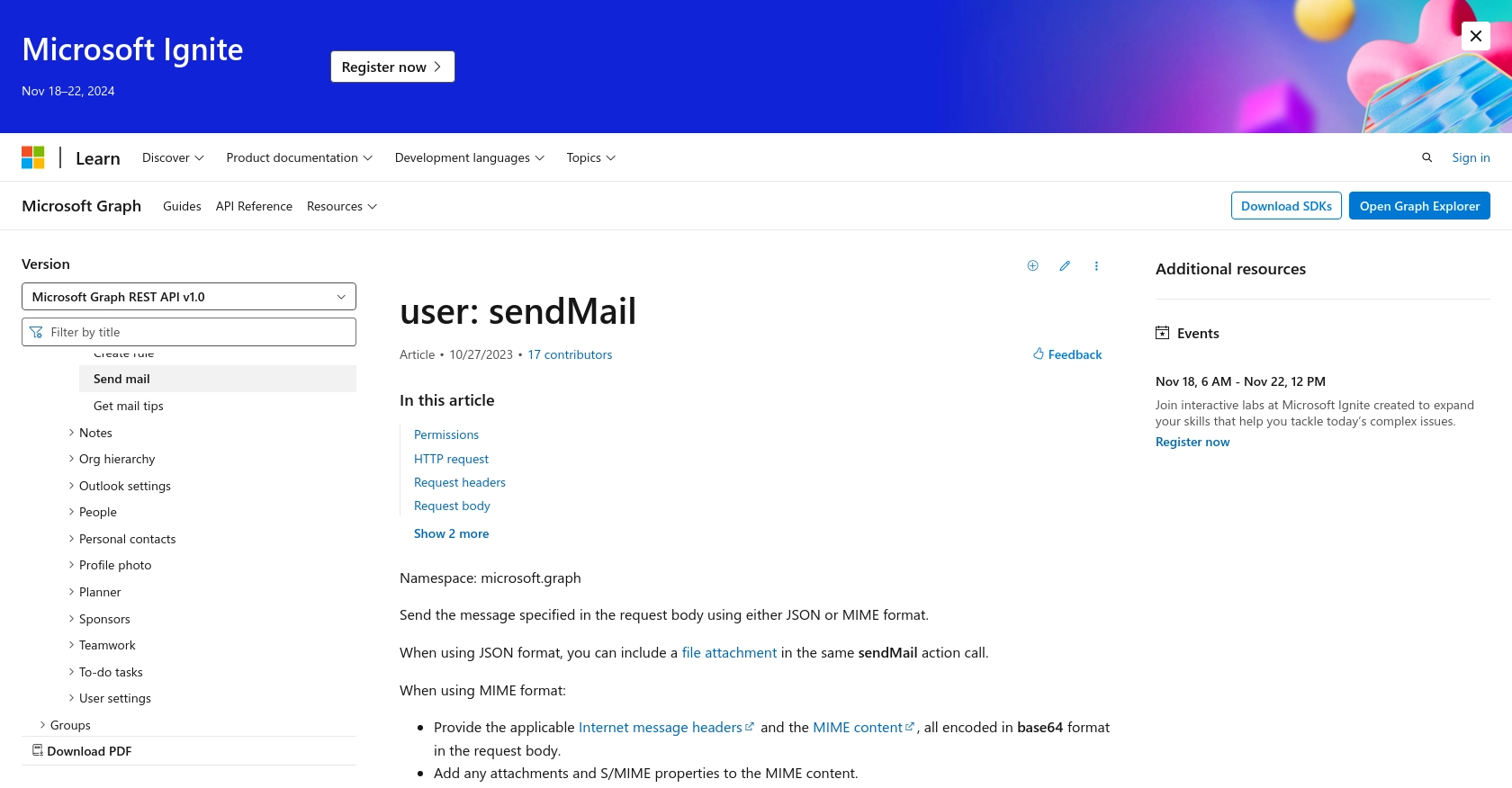
Conclusion and Best Practices for Using Outlook API in PHP
Integrating the Outlook API into your PHP applications can greatly enhance your ability to manage and automate email communications. By following the steps outlined in this guide, you can efficiently send emails programmatically, improving user engagement and streamlining processes.
Best Practices for Secure and Efficient Outlook API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Optimize Data Handling: Ensure that your application efficiently processes and stores email data. Consider transforming and standardizing data fields to maintain consistency across your application.
Leverage Endgrate for Seamless Integration Solutions
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Outlook, offering an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover how companies like yours have successfully scaled their integrations.
Read More
- https://endgrate.com/provider/outlook
- https://docs.microsoft.com/en-us/graph/overview
- https://learn.microsoft.com/en-us/graph/auth/auth-concepts
- https://learn.microsoft.com/en-us/graph/auth-register-app-v2
- https://learn.microsoft.com/en-us/graph/auth-v2-user?tabs=http
- https://learn.microsoft.com/en-us/graph/api/user-sendmail?view=graph-rest-1.0&tabs=http
Ready to get started?