Using the FreshService API to Create or Update Departments (with Python examples)
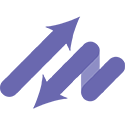
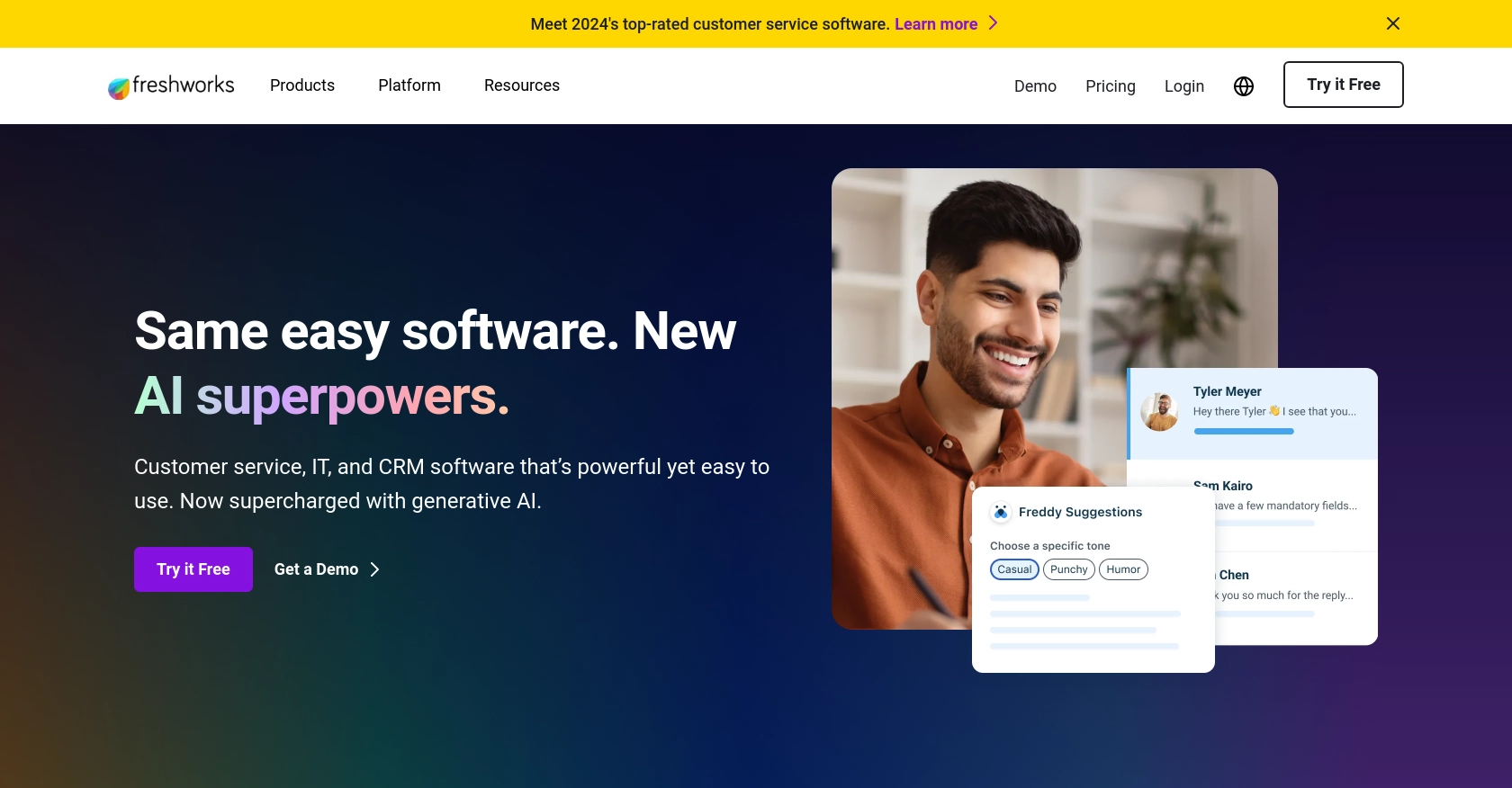
Introduction to FreshService API Integration
FreshService is a robust IT service management (ITSM) platform designed to streamline IT operations for businesses of all sizes. It offers a comprehensive suite of tools for incident management, asset management, change management, and more, making it a popular choice among IT professionals.
Integrating with the FreshService API allows developers to automate and enhance IT service processes. For example, you can create or update department information programmatically, ensuring that your IT service records are always up-to-date and accurate. This can be particularly useful for organizations that frequently restructure or onboard new departments, allowing seamless integration with existing IT workflows.
Setting Up Your FreshService Test/Sandbox Account
Before you can start integrating with the FreshService API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. FreshService provides a free trial that you can use to explore its features and test your integrations.
Creating a FreshService Account
To get started, visit the FreshService signup page and register for a free trial account. Follow the on-screen instructions to complete the registration process. Once your account is created, you will have access to the FreshService dashboard.
Generating API Key for FreshService
FreshService uses a custom authentication method that involves an API key. Follow these steps to generate your API key:
- Log in to your FreshService account.
- Navigate to your profile icon in the top right corner and select Profile Settings.
- In the API Key section, you will find your unique API key. Copy this key and store it securely, as you will need it to authenticate your API requests.
Setting Up a FreshService Sandbox Environment
While FreshService does not provide a separate sandbox environment, you can use your trial account as a testing ground. Ensure that any data you input is for testing purposes only. You can create sample departments and other entities to test your API interactions.
With your FreshService account and API key ready, you are now set to begin integrating with the FreshService API to create or update departments using Python.
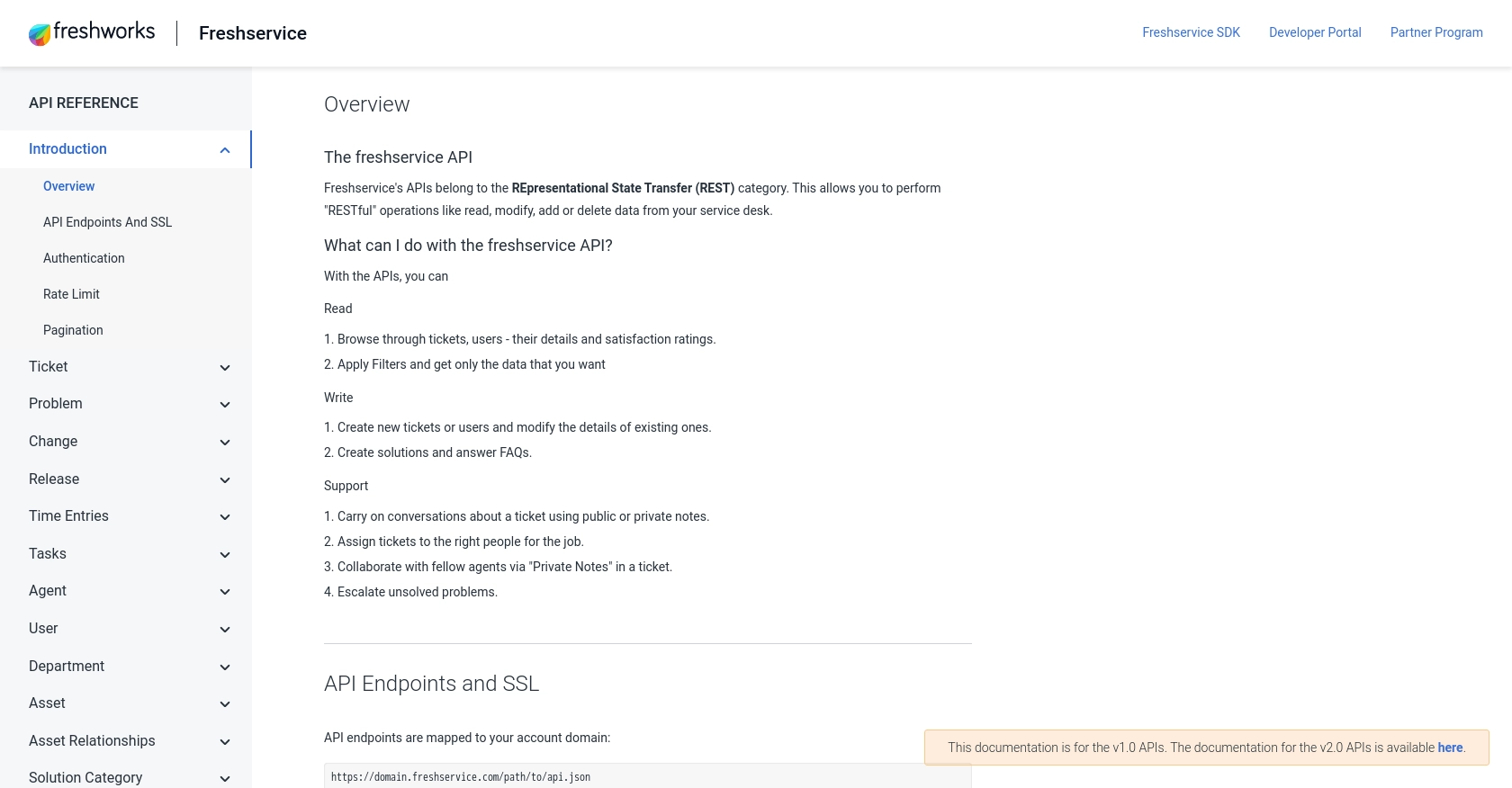
sbb-itb-96038d7
Making API Calls to FreshService for Department Management Using Python
To interact with the FreshService API and manage department information, you'll need to use Python to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your Python Environment for FreshService API Integration
Before you begin, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Creating or Updating Departments in FreshService Using Python
With your environment ready, you can now write the Python code to create or update departments in FreshService. Below is an example of how to make a POST request to create a department:
import requests
# Define the API endpoint
url = "https://yourdomain.freshservice.com/api/v2/departments"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Basic Your_API_Key"
}
# Define the department data
department_data = {
"department": {
"name": "New Department",
"description": "Description of the new department"
}
}
# Make the POST request to create a department
response = requests.post(url, json=department_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Department created successfully.")
else:
print(f"Failed to create department. Status code: {response.status_code}")
print(response.json())
Replace Your_API_Key
with the API key you obtained from your FreshService account. The above code sends a POST request to create a new department with the specified name and description.
Verifying API Call Success in FreshService
After running the script, you can verify the success of your API call by checking the FreshService dashboard. Navigate to the departments section to see if the new department has been added. If the request was successful, it should appear there.
Handling Errors and Troubleshooting FreshService API Calls
When making API calls, it's essential to handle potential errors. The FreshService API will return various status codes to indicate the result of your request:
- 201 Created: The department was successfully created.
- 400 Bad Request: The request was invalid. Check your input data.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 403 Forbidden: You do not have permission to perform this action.
- 404 Not Found: The requested resource could not be found.
Always check the response code and message to understand what went wrong and adjust your request accordingly.
Conclusion and Best Practices for FreshService API Integration
Integrating with the FreshService API to manage departments can significantly enhance your IT service management processes. By automating the creation and updating of department information, you ensure that your IT records are always current and accurate, which is crucial for efficient operations.
Best Practices for Secure and Efficient FreshService API Usage
- Secure Storage of API Keys: Always store your API keys securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of FreshService's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure that department data is standardized before making API calls. This helps maintain consistency across your IT service management system.
- Error Handling: Implement robust error handling to manage different response codes effectively. This will help you troubleshoot issues quickly and maintain a smooth integration process.
Streamline Your Integrations with Endgrate
While integrating with FreshService can be highly beneficial, managing multiple integrations can become complex and time-consuming. This is where Endgrate can make a difference. By using Endgrate, you can simplify your integration processes, allowing you to focus on your core product development.
Endgrate provides a unified API endpoint that connects to various platforms, including FreshService, enabling you to build once for each use case instead of multiple times for different integrations. This not only saves time but also capital, providing an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?