Using the Salesforce API to Get Records in Python
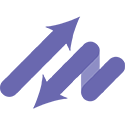
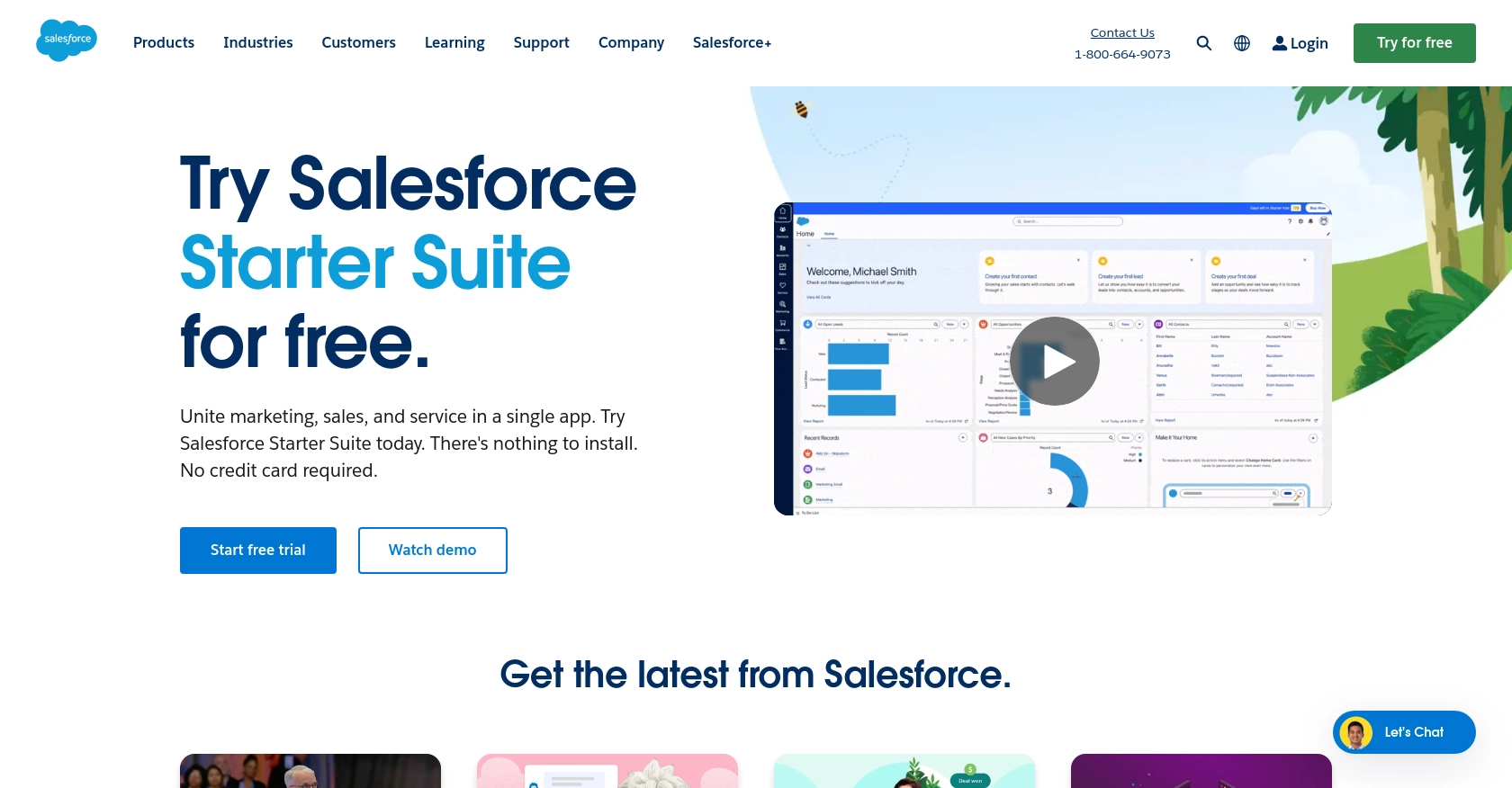
Introduction to Salesforce API Integration
Salesforce is a leading cloud-based customer relationship management (CRM) platform that empowers businesses to manage their sales, service, marketing, and more. With its robust suite of tools, Salesforce helps organizations streamline their operations and enhance customer engagement.
Developers often integrate with Salesforce's API to access and manipulate data within the platform, enabling custom solutions and automations. For example, a developer might use the Salesforce API to retrieve records such as leads or opportunities, allowing for seamless data synchronization between Salesforce and other business applications.
Setting Up Your Salesforce Developer Account and Sandbox Environment
Before you can start interacting with the Salesforce API, you need to set up a Salesforce Developer account and create a sandbox environment. This allows you to test your API integrations without affecting your live data.
Step-by-Step Guide to Creating a Salesforce Developer Account
- Visit the Salesforce Developer Signup Page and fill out the registration form with your details.
- Once registered, you will receive a confirmation email. Click the link in the email to verify your account.
- Log in to your Salesforce Developer account using the credentials you created.
Creating a Salesforce Sandbox for Testing
Salesforce provides a sandbox environment that allows developers to test their applications in a safe setting. Follow these steps to set up your sandbox:
- Navigate to the Setup area in your Salesforce Developer account.
- In the Quick Find box, type "Sandboxes" and select the Sandboxes option.
- Click on "New Sandbox" and choose the type of sandbox that suits your needs. For most API testing, a Developer Sandbox is sufficient.
- Enter a name for your sandbox and click "Create." The sandbox creation process may take some time.
Configuring OAuth for Salesforce API Access
Salesforce uses OAuth 2.0 for authentication, which requires setting up a connected app. Here's how to create one:
- In the Setup area, enter "App Manager" in the Quick Find box and select it.
- Click "New Connected App" in the top right corner.
- Fill in the required fields, such as the Connected App Name and Contact Email.
- Under "API (Enable OAuth Settings)," check the box for "Enable OAuth Settings."
- Enter a Callback URL. This can be a placeholder URL like
https://localhost/callback
for testing purposes. - Select the OAuth Scopes you need, such as "Full access (full)" and "Perform requests on your behalf at any time (refresh_token, offline_access)."
- Click "Save" to create your connected app. Note down the Consumer Key and Consumer Secret, as you'll need these for authentication.
For more detailed information, refer to the Salesforce OAuth Documentation.
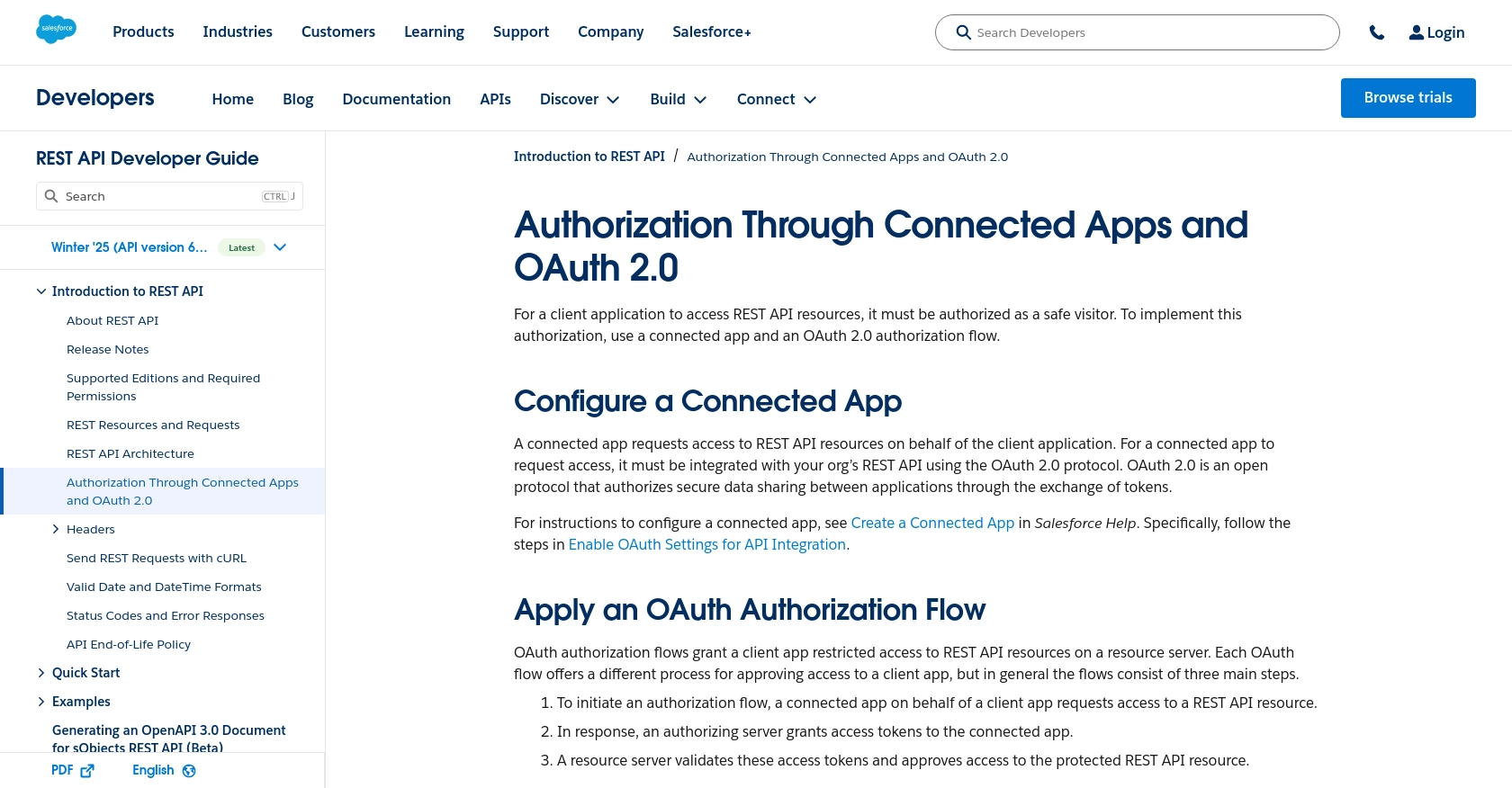
sbb-itb-96038d7
Making API Calls to Salesforce Using Python
Once your Salesforce Developer account and OAuth settings are configured, you can start making API calls to interact with Salesforce data. In this section, we'll guide you through the process of retrieving records using Python.
Prerequisites for Salesforce API Integration with Python
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
You'll also need the requests
library to make HTTP requests. Install it using the following command:
pip install requests
Authenticating with Salesforce API Using OAuth 2.0
To authenticate with the Salesforce API, you'll need to obtain an access token using the OAuth 2.0 protocol. Here's how to do it:
- Set your Salesforce credentials and OAuth details in your Python script:
- Make a POST request to the OAuth token endpoint to obtain an access token:
import requests
# Salesforce credentials
client_id = 'Your_Consumer_Key'
client_secret = 'Your_Consumer_Secret'
username = 'Your_Salesforce_Username'
password = 'Your_Salesforce_Password'
security_token = 'Your_Security_Token'
# OAuth endpoint
token_url = 'https://login.salesforce.com/services/oauth2/token'
# Prepare the payload
payload = {
'grant_type': 'password',
'client_id': client_id,
'client_secret': client_secret,
'username': username,
'password': password + security_token
}
# Request the access token
response = requests.post(token_url, data=payload)
access_token = response.json().get('access_token')
instance_url = response.json().get('instance_url')
Replace the placeholders with your actual Salesforce credentials and security token. The response will contain the access_token
and instance_url
, which you'll use for subsequent API calls.
Retrieving Salesforce Records Using Python
With the access token in hand, you can now retrieve records from Salesforce. Here's an example of how to get a list of accounts:
# Define the API endpoint for retrieving accounts
api_endpoint = f'{instance_url}/services/data/v52.0/sobjects/Account'
# Set the headers with the access token
headers = {
'Authorization': f'Bearer {access_token}',
'Content-Type': 'application/json'
}
# Make a GET request to the Salesforce API
response = requests.get(api_endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
accounts = response.json()
print("Retrieved Accounts:", accounts)
else:
print("Failed to retrieve accounts:", response.status_code, response.text)
This code snippet makes a GET request to the Salesforce API to retrieve account records. If successful, it prints the retrieved accounts; otherwise, it displays an error message.
Handling Errors and Verifying API Requests
It's crucial to handle potential errors when making API calls. Salesforce may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Always check the response status code and handle errors appropriately.
To verify that your API requests are successful, you can log into your Salesforce sandbox and check the data. Ensure that the records retrieved or manipulated match your expectations.
Conclusion and Best Practices for Salesforce API Integration
Integrating with the Salesforce API using Python offers a powerful way to access and manipulate CRM data, enabling developers to create custom solutions and automate workflows. By following the steps outlined in this guide, you can efficiently set up your Salesforce Developer account, configure OAuth authentication, and make API calls to retrieve records.
Best Practices for Secure and Efficient Salesforce API Usage
- Securely Store Credentials: Always store your Salesforce credentials, such as the client ID, client secret, and access tokens, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Salesforce imposes rate limits on API requests. Monitor your API usage and implement retry logic or backoff strategies to handle rate limit errors gracefully. For more details, refer to the Salesforce API documentation.
- Standardize Data Fields: Ensure that data fields retrieved from Salesforce are transformed and standardized to match your application's data model, facilitating seamless integration.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with Salesforce directly can be rewarding, it may also be time-consuming and complex, especially when dealing with multiple integrations. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs.
Read More
- https://endgrate.com/provider/salesforce
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?