Using the Younium API to Get Accounts (with PHP examples)
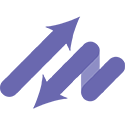
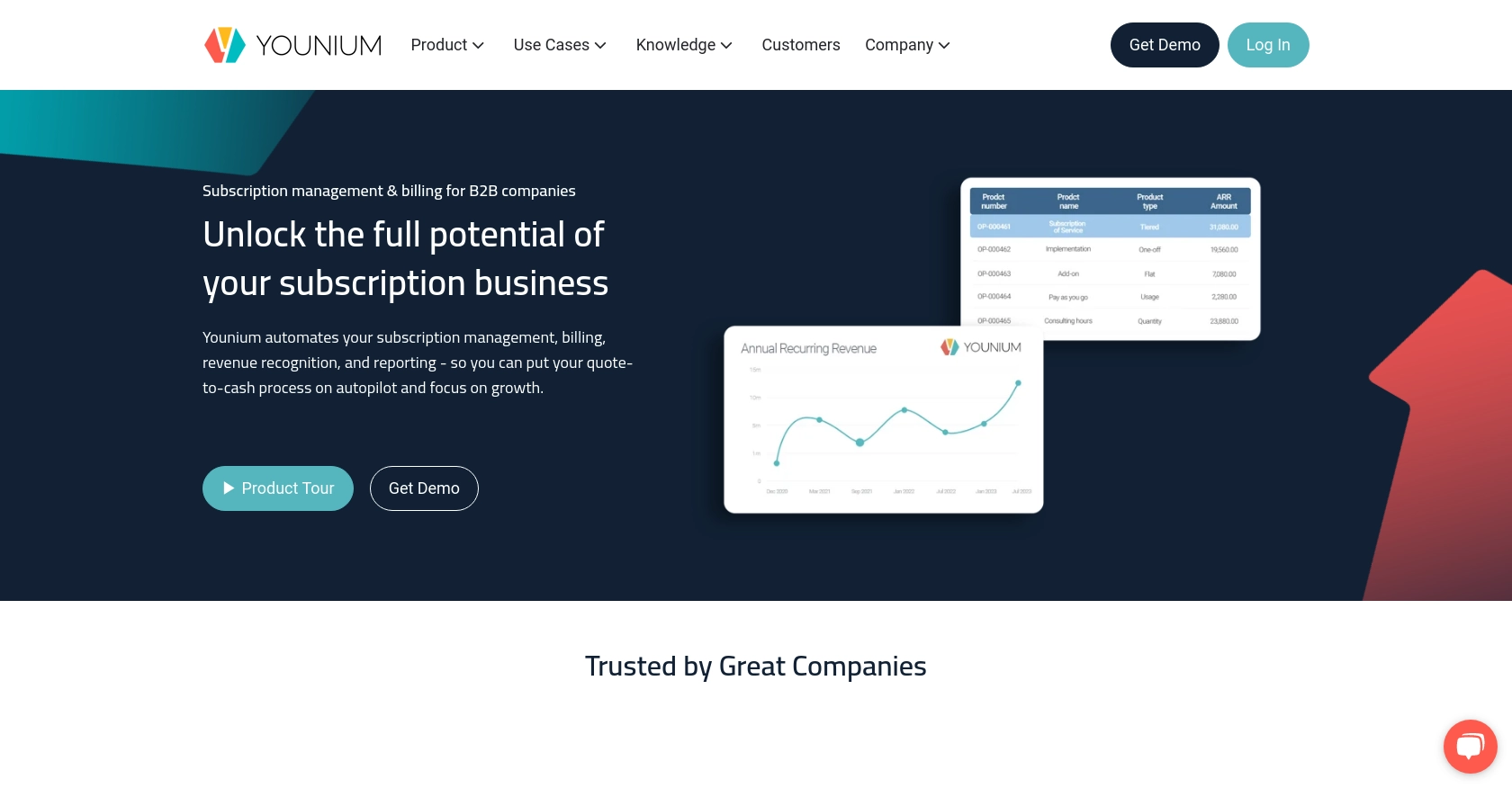
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing, invoicing, and revenue recognition for B2B SaaS companies. It offers a robust API that allows developers to integrate seamlessly with its features, enabling automation and enhanced data management.
Connecting with the Younium API can empower developers to efficiently manage account data, automate billing processes, and synchronize customer information across platforms. For example, a developer might use the Younium API to retrieve account details and integrate them into a custom dashboard, providing real-time insights into customer subscriptions and financial metrics.
Setting Up Your Younium Test/Sandbox Account
Before you can start integrating with the Younium API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data, making it an essential step for development and testing.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. Follow these steps to get started:
- Visit the Younium Developer Portal and sign up for a developer account if you haven't already.
- Once registered, log in to your account and navigate to the sandbox environment setup section.
- Follow the on-screen instructions to create your sandbox account, ensuring you have access to all necessary features for testing API interactions.
Generating API Token and Client Credentials
To authenticate your API requests, you'll need to generate an API token and client credentials. Here's how:
- Open the user profile menu by clicking your name in the top right corner, then select “Privacy & Security”.
- Choose “Personal Tokens” from the left panel and click “Generate Token”.
- Provide a relevant description for your token and click “Create”.
- Copy the generated Client ID and Secret Key, as they will not be visible again. These credentials are crucial for generating your JWT access token.
Acquiring a JWT Access Token
With your client credentials ready, you can now acquire a JWT access token to authenticate your API requests:
// Define the endpoint and headers
$url = 'https://api.sandbox.younium.com/auth/token';
$headers = [
'Content-Type: application/json'
];
// Set the request body with client credentials
$body = json_encode([
'clientId' => 'Your_Client_ID',
'secret' => 'Your_Secret_Key'
]);
// Initialize a cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $body);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the response to get the access token
$data = json_decode($response, true);
$accessToken = $data['accessToken'];
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This script will return a JWT access token, valid for 24 hours, which you'll use to authenticate your API calls.
For more detailed information on authentication, refer to the Younium Authentication Documentation.
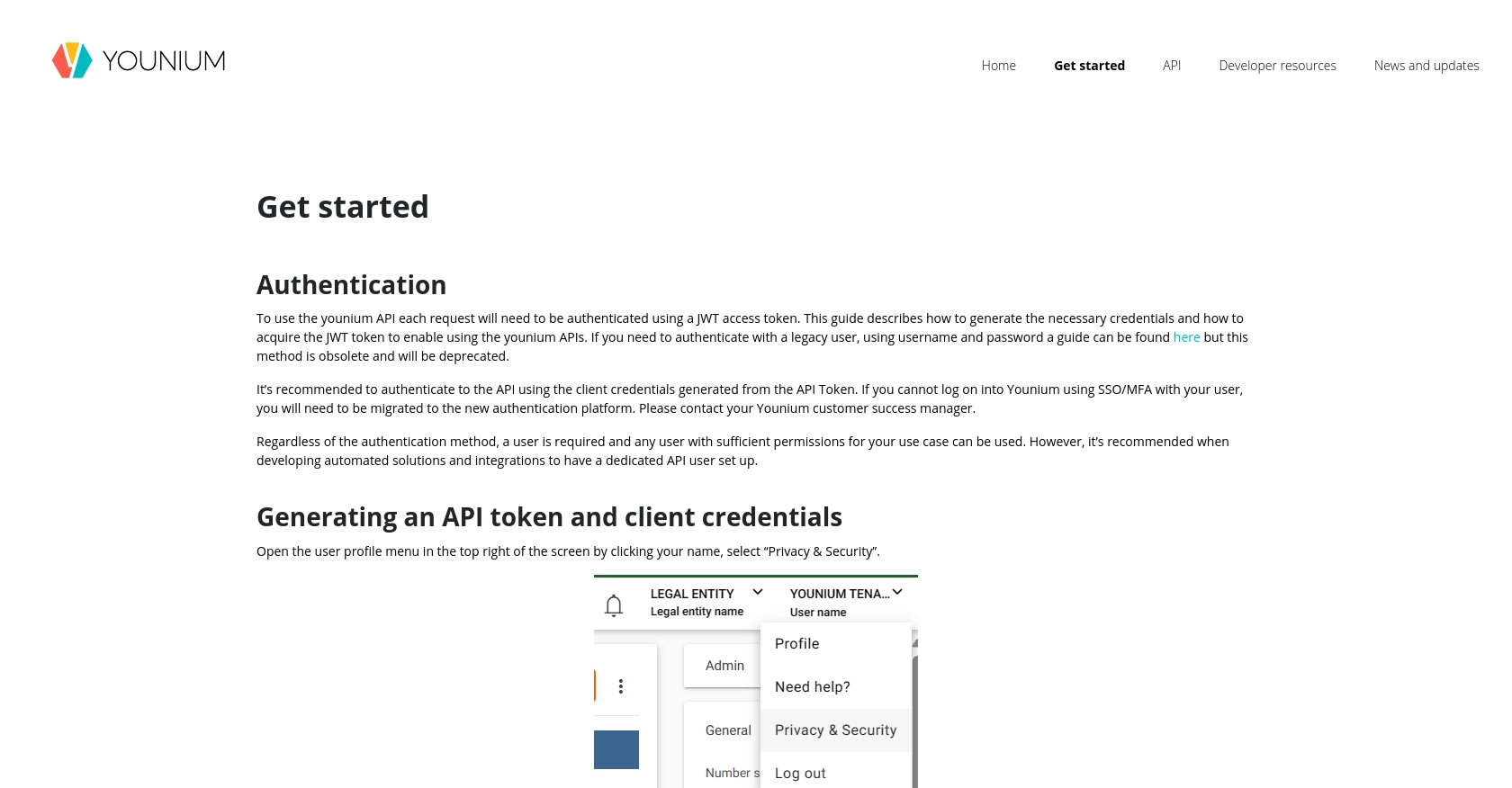
sbb-itb-96038d7
Making API Calls to Retrieve Accounts Using Younium API with PHP
With your JWT access token in hand, you can now proceed to make authenticated API calls to the Younium platform. In this section, we'll guide you through the process of retrieving account data using PHP, ensuring you have the necessary setup to interact with the Younium API effectively.
Setting Up Your PHP Environment for Younium API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP 7.4 or later
- cURL extension enabled
These prerequisites will allow you to execute HTTP requests and handle JSON responses efficiently.
Retrieving Accounts from Younium API
To retrieve account information, you'll need to make a GET request to the Younium API's accounts endpoint. Here's a step-by-step guide:
// Define the API endpoint and headers
$url = 'https://api.sandbox.younium.com/accounts';
$headers = [
'Authorization: Bearer Your_Access_Token',
'Content-Type: application/json',
'api-version: 2.1', // Optional but recommended
'legal-entity: Your_Legal_Entity_ID' // Required if multiple legal entities exist
];
// Initialize a cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the JSON response
$data = json_decode($response, true);
// Output the account data
foreach ($data['accounts'] as $account) {
echo 'Account ID: ' . $account['id'] . "\n";
echo 'Account Name: ' . $account['name'] . "\n";
echo '------------------------' . "\n";
}
Replace Your_Access_Token
and Your_Legal_Entity_ID
with the appropriate values. This script will fetch and display account details from your Younium sandbox environment.
Verifying API Call Success and Handling Errors
After executing the API call, it's crucial to verify the success of your request. Check the response status code and ensure the data returned matches the expected output. If the request fails, handle errors gracefully:
- 401 Unauthorized: Indicates an expired or invalid access token. Ensure your token is valid and correctly included in the headers.
- 403 Forbidden: Occurs if the legal entity is incorrect or the user lacks permissions. Verify the legal entity ID and user permissions.
For more detailed error handling, refer to the Younium API Documentation.
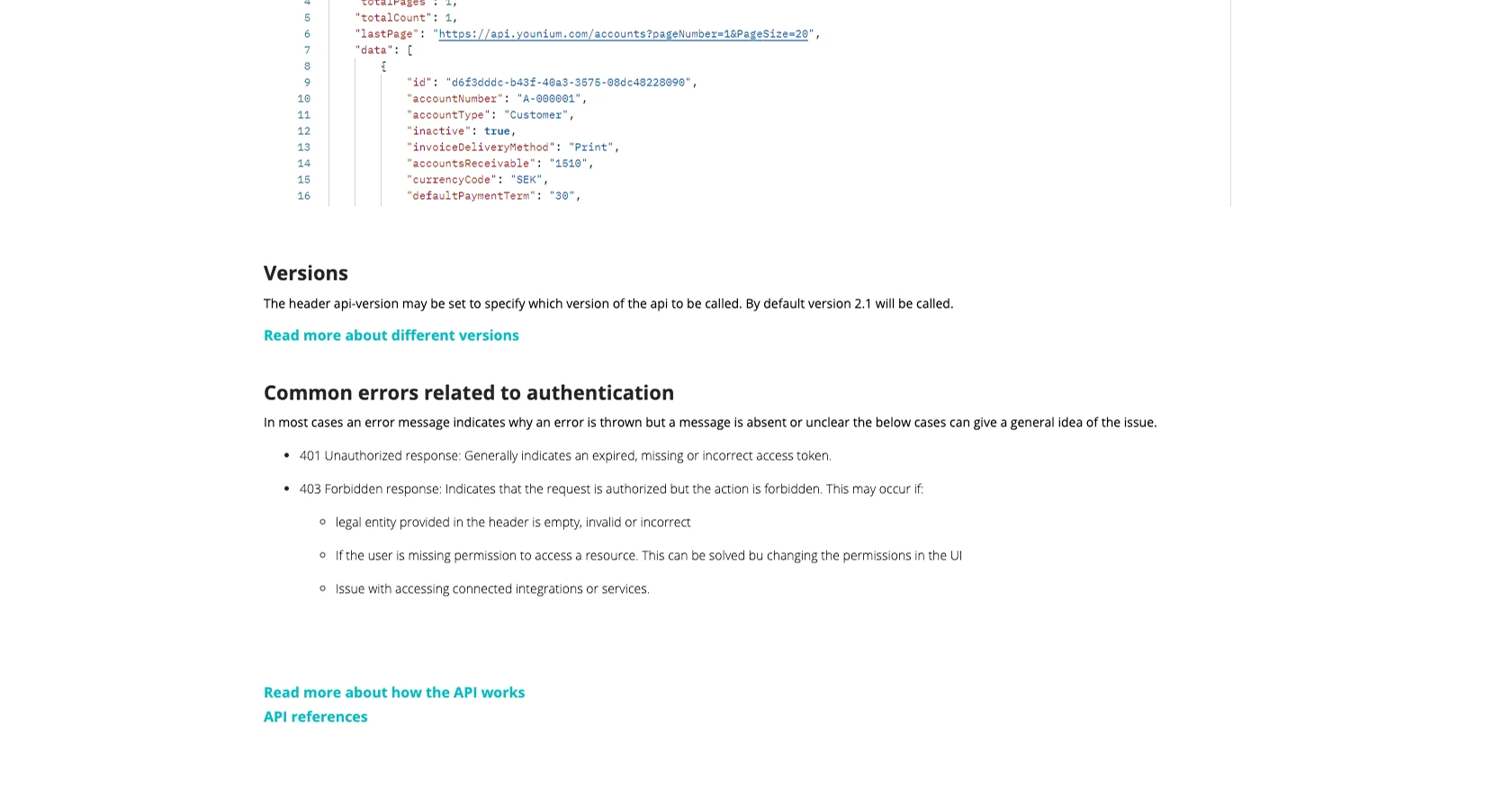
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API provides a powerful way to manage subscription data and automate billing processes for B2B SaaS companies. By following the steps outlined in this guide, you can effectively retrieve account information using PHP, enhancing your data management capabilities.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your client credentials and JWT access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Regular Token Renewal: Since JWT tokens are valid for 24 hours, implement a mechanism to renew tokens automatically to maintain seamless API access.
Enhance Your Integration Strategy with Endgrate
While integrating with Younium is a significant step, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Younium.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate's website today.
Read More
Ready to get started?