How to Get Users with the Outreach API in PHP
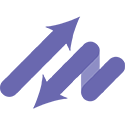
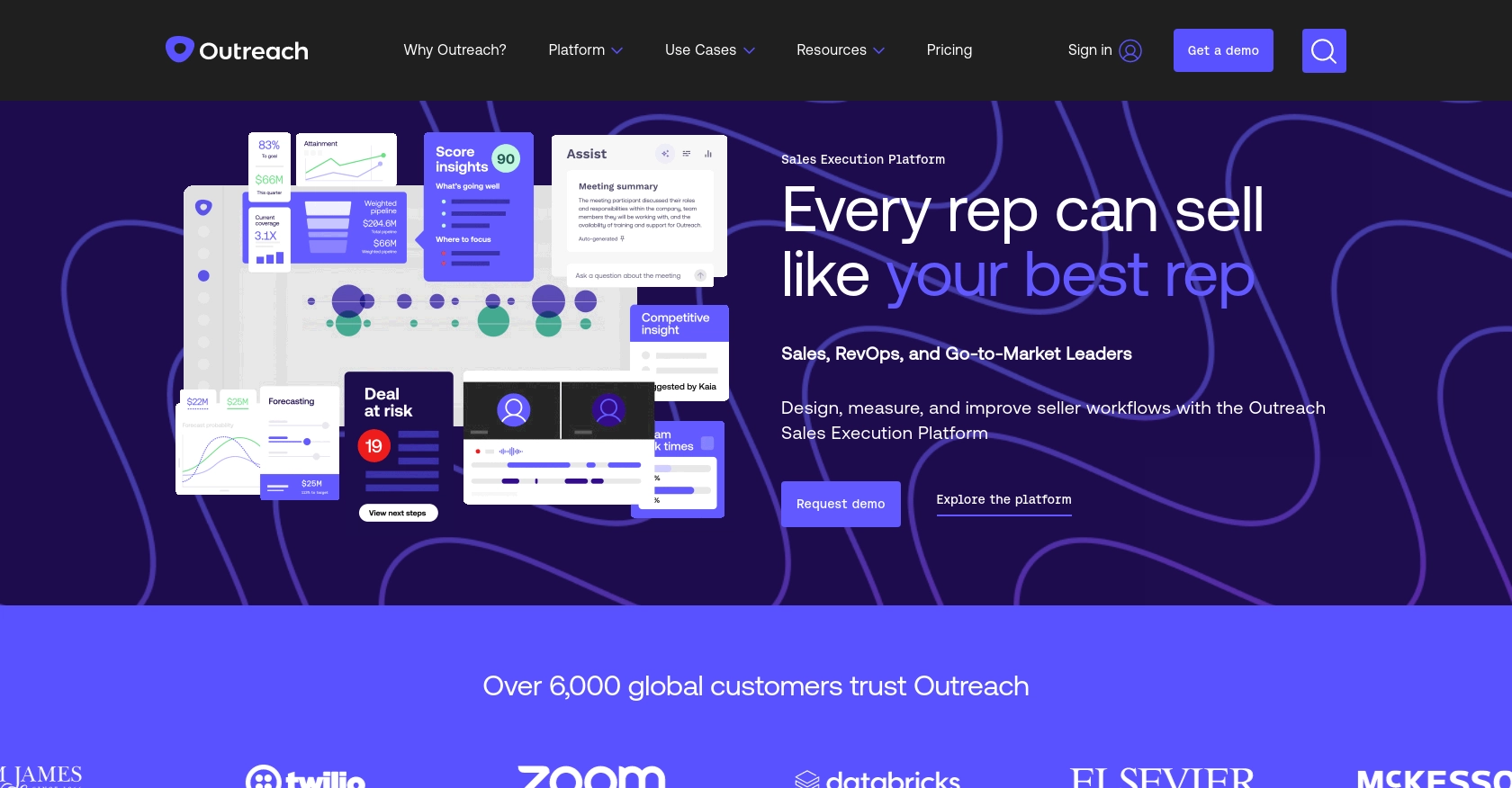
Introduction to Outreach API Integration
Outreach is a powerful sales engagement platform that helps businesses streamline their sales processes and improve productivity. By providing tools for managing customer interactions, tracking sales activities, and automating workflows, Outreach enables sales teams to focus on what matters most—closing deals.
Integrating with the Outreach API allows developers to access and manage user data efficiently. For example, you might want to retrieve user information to customize sales strategies or synchronize user data with other systems. This integration can enhance your application's functionality by providing seamless access to Outreach's robust features.
Setting Up Your Outreach Test/Sandbox Account
Before you can start integrating with the Outreach API, you'll need to set up a test or sandbox account. This allows you to safely develop and test your application without affecting live data.
Create an Outreach Developer Account
If you don't already have an Outreach account, you can sign up for a developer account on the Outreach Developer Portal. This account will provide you with access to the necessary tools and resources for API integration.
Configure OAuth for Outreach API Access
The Outreach API uses OAuth 2.0 for authentication. Follow these steps to set up OAuth:
- Log in to your Outreach developer account.
- Navigate to the "My Apps" section and click on "Create New App."
- Fill in the required details for your app, such as the name and description.
- Under the "API Access" tab, configure your OAuth settings:
- Specify one or more redirect URIs.
- Select the OAuth data scopes your application will use.
- Save your app settings to generate your OAuth credentials, including the client ID and client secret.
Remember to securely store your client secret, as it will only be displayed once. If needed, you can regenerate it later.
Authorize Your Application
To authorize your application, redirect users to the Outreach authorization URL:
https://api.outreach.io/oauth/authorize?client_id=<your_client_id>&redirect_uri=<your_redirect_uri>&response_type=code&scope=users.read
After the user consents, Outreach will redirect them to your specified redirect URI with an authorization code. Use this code to request an access token:
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<your_client_id> \
-d client_secret=<your_client_secret> \
-d redirect_uri=<your_redirect_uri> \
-d grant_type=authorization_code \
-d code=<authorization_code>
This will return an access token, which you can use to authenticate API requests on behalf of the user.
Testing with Development Credentials
Development credentials are intended for testing and should not be used in production. They allow up to 10 users from your organization to authorize the app. Be aware that tokens may expire, requiring re-authorization periodically.
For more details on setting up OAuth, refer to the Outreach OAuth Documentation.
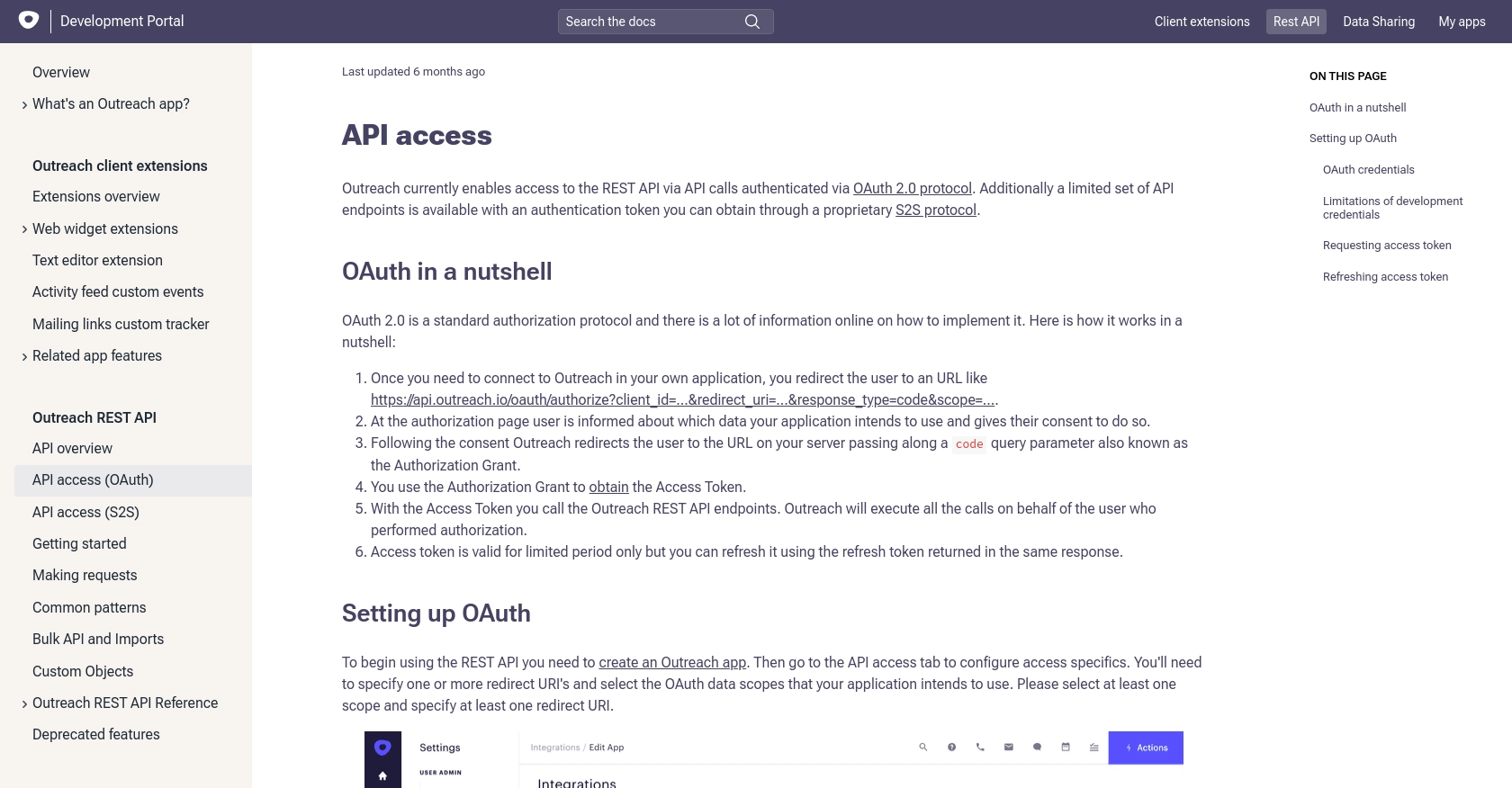
sbb-itb-96038d7
Making API Calls to Retrieve Users with Outreach API in PHP
To interact with the Outreach API and retrieve user data, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your PHP Environment for Outreach API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for dependency management
Next, install the Guzzle HTTP client, which simplifies making HTTP requests in PHP:
composer require guzzlehttp/guzzle
Writing PHP Code to Get Users from Outreach API
Create a new PHP file named get_outreach_users.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your access token
try {
$response = $client->request('GET', 'https://api.outreach.io/api/v2/users', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/vnd.api+json',
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['data'] as $user) {
echo 'User ID: ' . $user['id'] . ' - Name: ' . $user['attributes']['name'] . "\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token obtained from the OAuth process. This script uses Guzzle to send a GET request to the Outreach API, retrieves user data, and prints each user's ID and name.
Verifying API Call Success and Handling Errors
After running the script, verify the output to ensure it matches the users in your Outreach test account. If the request is successful, you should see a list of user IDs and names.
In case of errors, the script will catch exceptions and display an error message. Common issues include:
- Invalid access token: Ensure your token is correct and hasn't expired.
- Network issues: Check your internet connection and API endpoint URL.
- Rate limits: Outreach API allows up to 10,000 requests per hour. Exceeding this limit results in a 429 error.
For more details on error codes, refer to the Outreach API User Documentation.
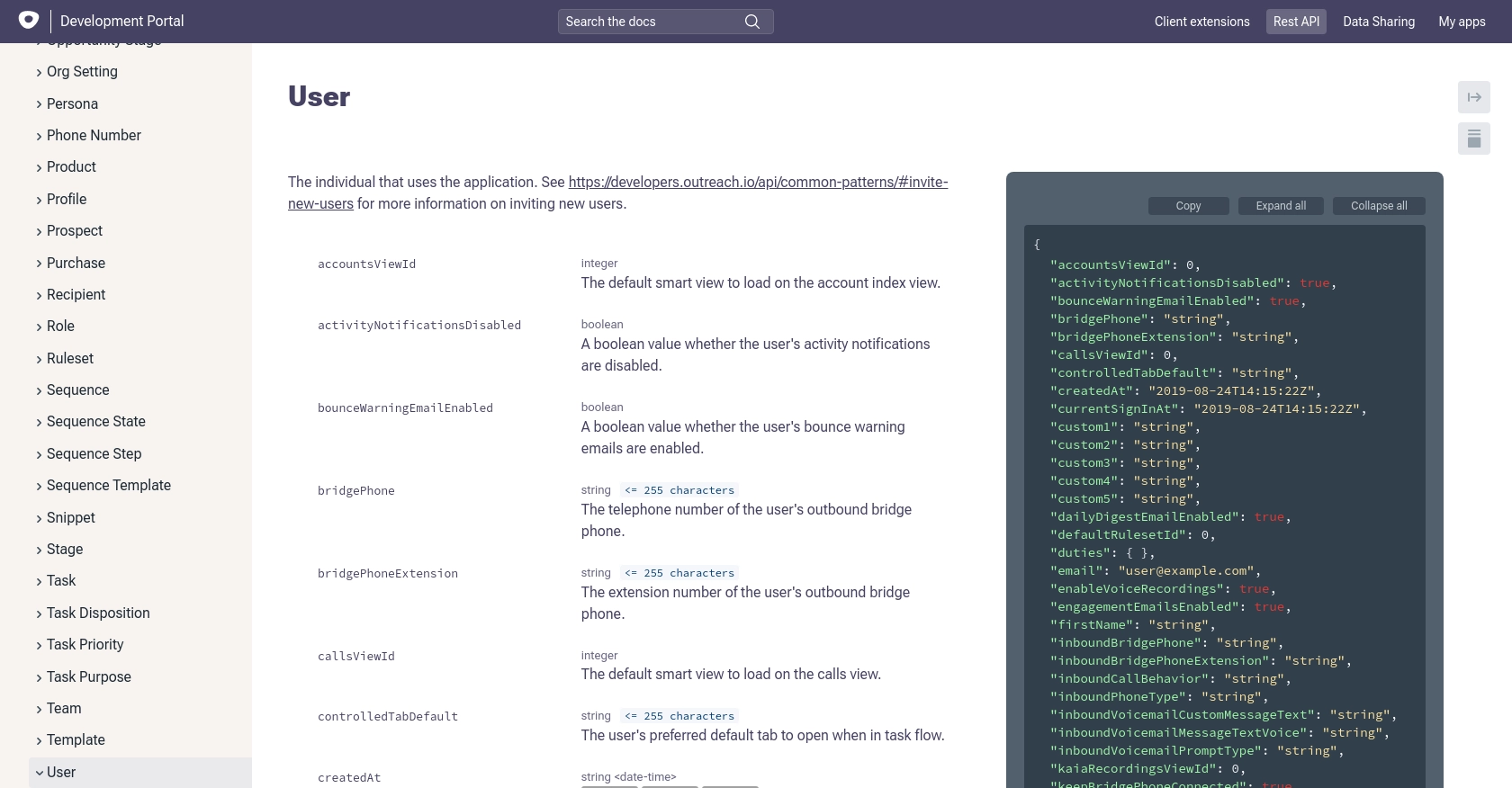
Conclusion and Best Practices for Integrating Outreach API with PHP
Integrating the Outreach API into your PHP application can significantly enhance your ability to manage user data and streamline sales processes. By following the steps outlined in this guide, you can efficiently retrieve user information and leverage Outreach's powerful features.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: The Outreach API allows up to 10,000 requests per hour. Implement logic to handle 429 errors gracefully and retry requests after the rate limit resets.
- Refresh Tokens Appropriately: Access tokens are short-lived. Use refresh tokens to obtain new access tokens before they expire, ensuring uninterrupted API access.
- Standardize Data Fields: When synchronizing data with other systems, ensure consistent data formats to avoid discrepancies and maintain data integrity.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a unified API solution that simplifies the process. By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can enhance your integration experience by visiting Endgrate.
By following these best practices and leveraging tools like Endgrate, you can create robust and efficient integrations with the Outreach API, enhancing your application's capabilities and delivering a seamless experience to your users.
Read More
Ready to get started?