Using the Clickup API to Get Tasks in PHP
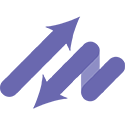
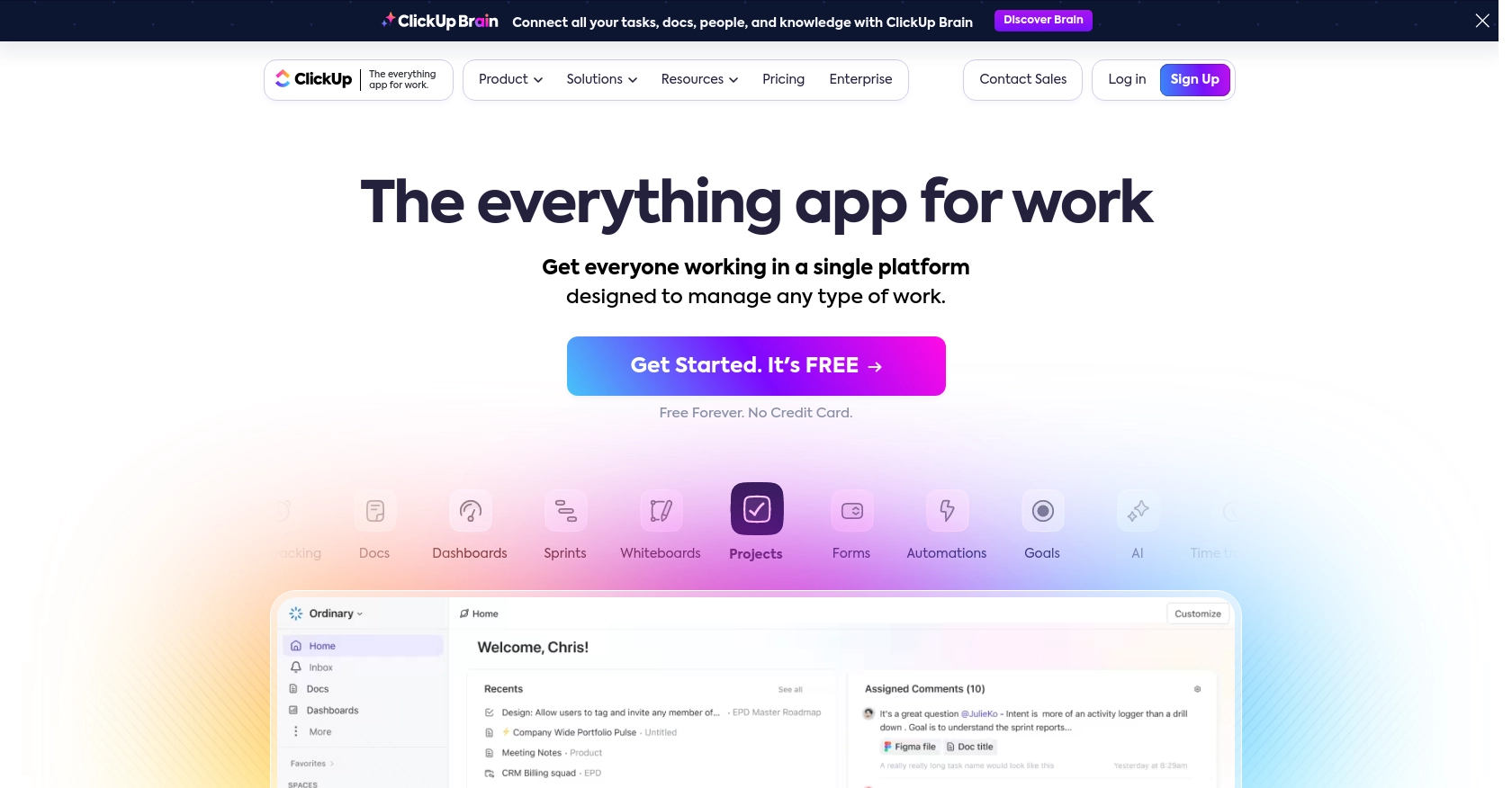
Introduction to ClickUp API Integration
ClickUp is a versatile project management tool that offers a wide range of features to help teams organize tasks, manage workflows, and enhance productivity. With its customizable interface and robust functionality, ClickUp is a popular choice for businesses looking to streamline their project management processes.
Developers may want to integrate with ClickUp's API to automate task management and enhance collaboration within their applications. For example, using the ClickUp API, a developer can retrieve tasks from a specific list to display them in a custom dashboard, enabling team members to track progress and deadlines efficiently.
Setting Up Your ClickUp Test Account for API Integration
Before diving into the ClickUp API, you need to set up a test account to experiment with API calls without affecting your live data. ClickUp offers a straightforward process to create a sandbox environment using either a personal API token or the OAuth flow for broader integrations.
Creating a ClickUp Account
If you don't already have a ClickUp account, start by signing up on the ClickUp website. You can choose a free plan to explore the API capabilities without any initial investment.
Generating a Personal API Token in ClickUp
For personal use or testing, you can generate a personal API token. Follow these steps:
- Log into your ClickUp account.
- In ClickUp 3.0, click your avatar in the upper-right corner and select Settings.
- Scroll down to the Apps section in the sidebar and click on it.
- Under API Token, click Generate to create your personal API token.
- Copy and securely store your personal API token, as you'll need it for API requests.
Setting Up OAuth for ClickUp API
If you're developing an application for other users, you'll need to use the OAuth flow:
- Log into ClickUp and click your avatar in the lower-left corner.
- Select Integrations and then click on ClickUp API.
- Click Create an App and provide a name and redirect URL for your app.
- Once created, you'll receive a
client_id
andclient_secret
. - Use these credentials to authenticate users and access their ClickUp data.
For more details on OAuth setup, refer to the ClickUp Authentication Documentation.
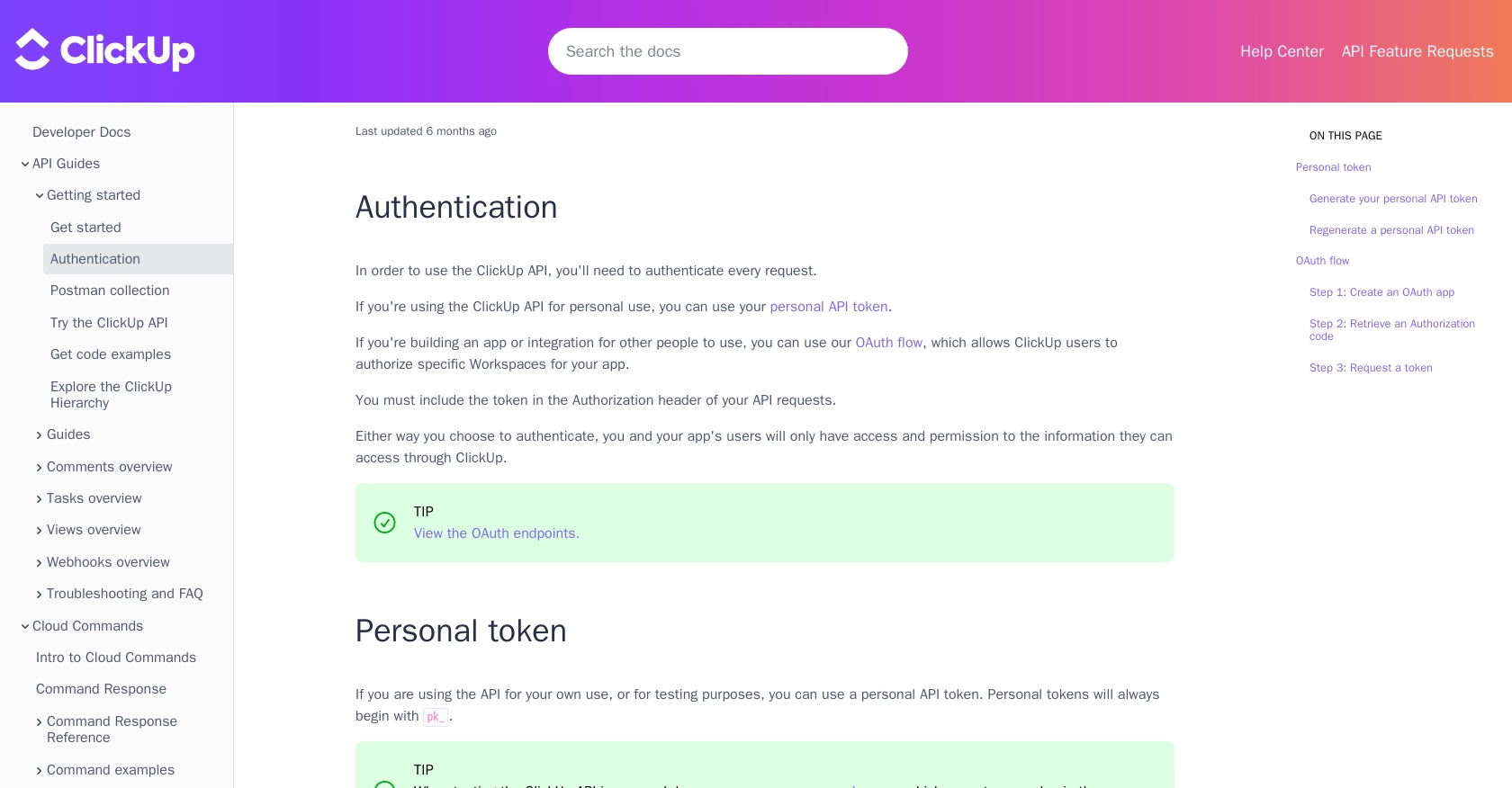
sbb-itb-96038d7
Making API Calls to Retrieve ClickUp Tasks Using PHP
To interact with the ClickUp API and retrieve tasks, you'll need to use PHP, a popular server-side scripting language. This section will guide you through the process of setting up your PHP environment, making the necessary API calls, and handling the responses effectively.
Setting Up Your PHP Environment for ClickUp API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, open your terminal and install the Guzzle HTTP client, which simplifies making HTTP requests in PHP:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Tasks from ClickUp
Create a new PHP file named get_clickup_tasks.php
and add the following code to it:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiToken = 'Your_API_Token';
$listId = 'Your_List_ID';
$response = $client->request('GET', "https://api.clickup.com/api/v2/list/{$listId}/task", [
'headers' => [
'Authorization' => $apiToken
],
'query' => [
'archived' => 'false',
'include_closed' => 'true',
'page' => 0
]
]);
$tasks = json_decode($response->getBody(), true);
foreach ($tasks['tasks'] as $task) {
echo "Task ID: " . $task['id'] . " - Name: " . $task['name'] . "\n";
}
Replace Your_API_Token
with your personal API token and Your_List_ID
with the ID of the list you want to retrieve tasks from.
Running the PHP Script and Verifying API Call Success
Run the script from your terminal using the following command:
php get_clickup_tasks.php
If successful, the script will output the task IDs and names from the specified ClickUp list. You can verify the retrieved tasks by comparing them with the tasks visible in your ClickUp account.
Handling Errors and Understanding ClickUp API Response Codes
When making API calls, it's crucial to handle potential errors. The ClickUp API may return various HTTP status codes indicating the success or failure of your request:
- 200 OK: The request was successful, and the tasks were retrieved.
- 401 Unauthorized: The API token is missing or invalid.
- 404 Not Found: The specified list ID does not exist.
Ensure you check the response status code and handle errors appropriately in your application.
For more details on making API calls, refer to the ClickUp API Documentation.
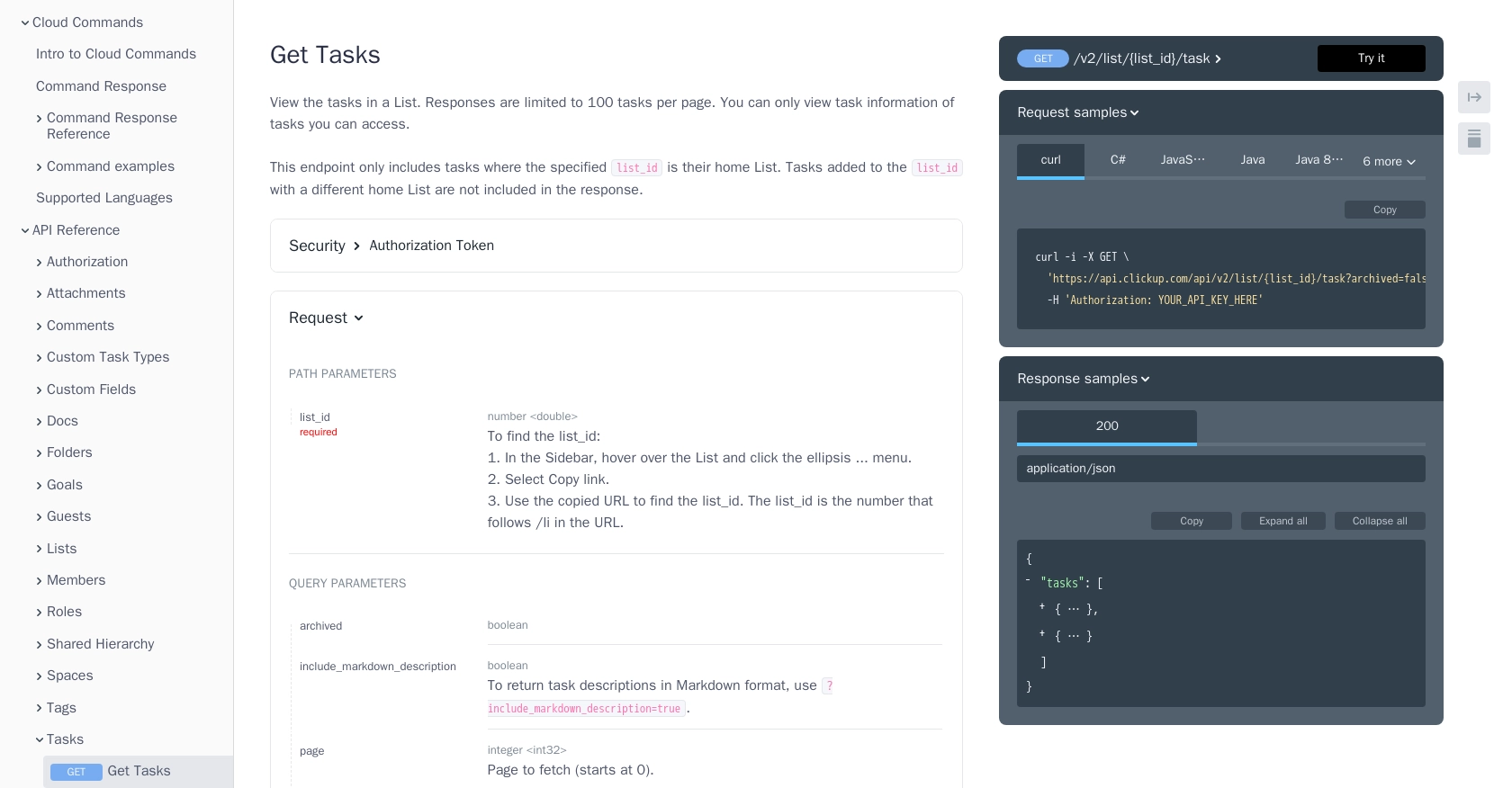
Conclusion and Best Practices for ClickUp API Integration in PHP
Integrating with the ClickUp API using PHP allows developers to automate task management and enhance productivity within their applications. By following the steps outlined in this guide, you can efficiently retrieve tasks from ClickUp and display them in a custom dashboard or application.
Best Practices for Secure and Efficient ClickUp API Usage
- Securely Store API Credentials: Always store your API tokens and OAuth credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of ClickUp's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Optimize API Calls: Use query parameters effectively to filter and paginate results, reducing the amount of data transferred and improving performance.
- Standardize Data Fields: Transform and standardize data fields to ensure consistency across your application, especially when integrating with multiple APIs.
Enhance Your Integration Strategy with Endgrate
While integrating with ClickUp's API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including ClickUp.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
Ready to get started?