How to Get Custom Objects with the Microsoft Dynamics 365 API in Python
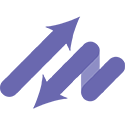
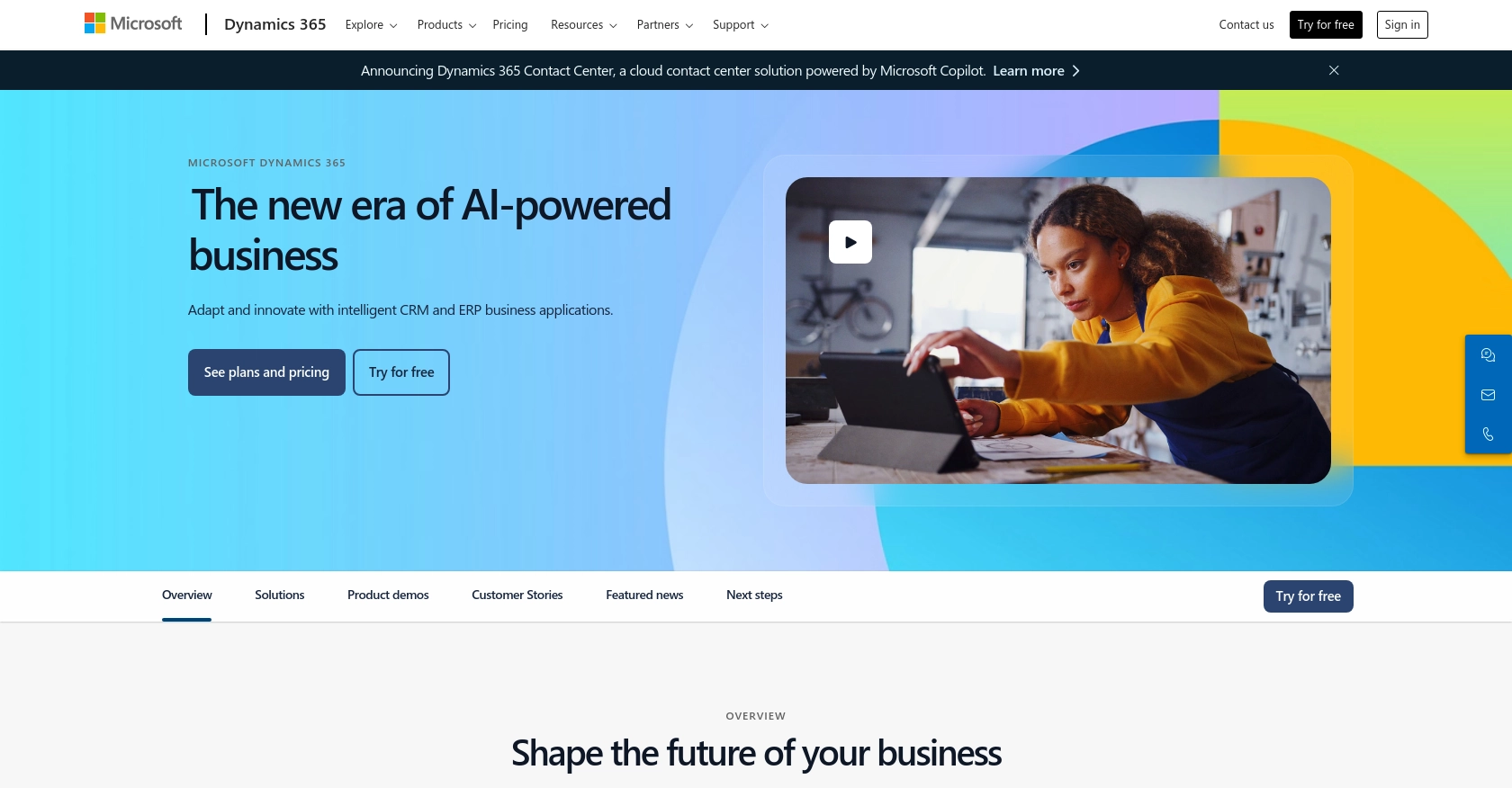
Introduction to Microsoft Dynamics 365 API Integration
Microsoft Dynamics 365 is a comprehensive suite of business applications that combines CRM and ERP capabilities to streamline business processes and improve customer engagement. It offers a flexible platform for managing sales, customer service, finance, operations, and more, making it a popular choice for enterprises looking to enhance their operational efficiency.
Integrating with the Microsoft Dynamics 365 API allows developers to access and manipulate data within the platform, enabling the creation of custom solutions tailored to specific business needs. For example, a developer might want to retrieve custom objects to analyze sales data or automate reporting processes, enhancing decision-making and operational workflows.
Setting Up a Microsoft Dynamics 365 Test or Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data, making it ideal for development and testing purposes.
Creating a Microsoft Dynamics 365 Sandbox Account
To begin, you need to create a Microsoft Dynamics 365 sandbox account. Follow these steps:
- Visit the Microsoft Dynamics 365 website and sign up for a free trial if you don't already have an account.
- Once logged in, navigate to the admin center and select the option to create a new environment.
- Choose the sandbox type for your environment. This will ensure that your testing does not interfere with production data.
- Complete the setup by following the on-screen instructions to configure your sandbox environment.
Registering an Application for OAuth Authentication
Microsoft Dynamics 365 uses OAuth 2.0 for authentication. To interact with the API, you must register an application in your Microsoft Entra ID tenant:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to the "Azure Active Directory" section and select "App registrations."
- Click on "New registration" and fill in the necessary details, such as the application name and redirect URI.
- After registering the app, note down the Application (client) ID and Directory (tenant) ID, as you will need these for authentication.
- Under "Certificates & secrets," create a new client secret and save it securely. This secret is crucial for obtaining access tokens.
Configuring API Permissions for Microsoft Dynamics 365
To access Microsoft Dynamics 365 data, you need to configure the appropriate API permissions for your registered application:
- In the Azure Portal, navigate to your registered app and select "API permissions."
- Click on "Add a permission" and choose "Dynamics CRM."
- Select the permissions required for your application, such as "user_impersonation" for delegated access.
- Grant admin consent for the permissions to ensure your application can access the necessary data.
With your sandbox account and application registration complete, you're now ready to start making API calls to Microsoft Dynamics 365. This setup ensures a secure and isolated environment for testing your integration solutions.
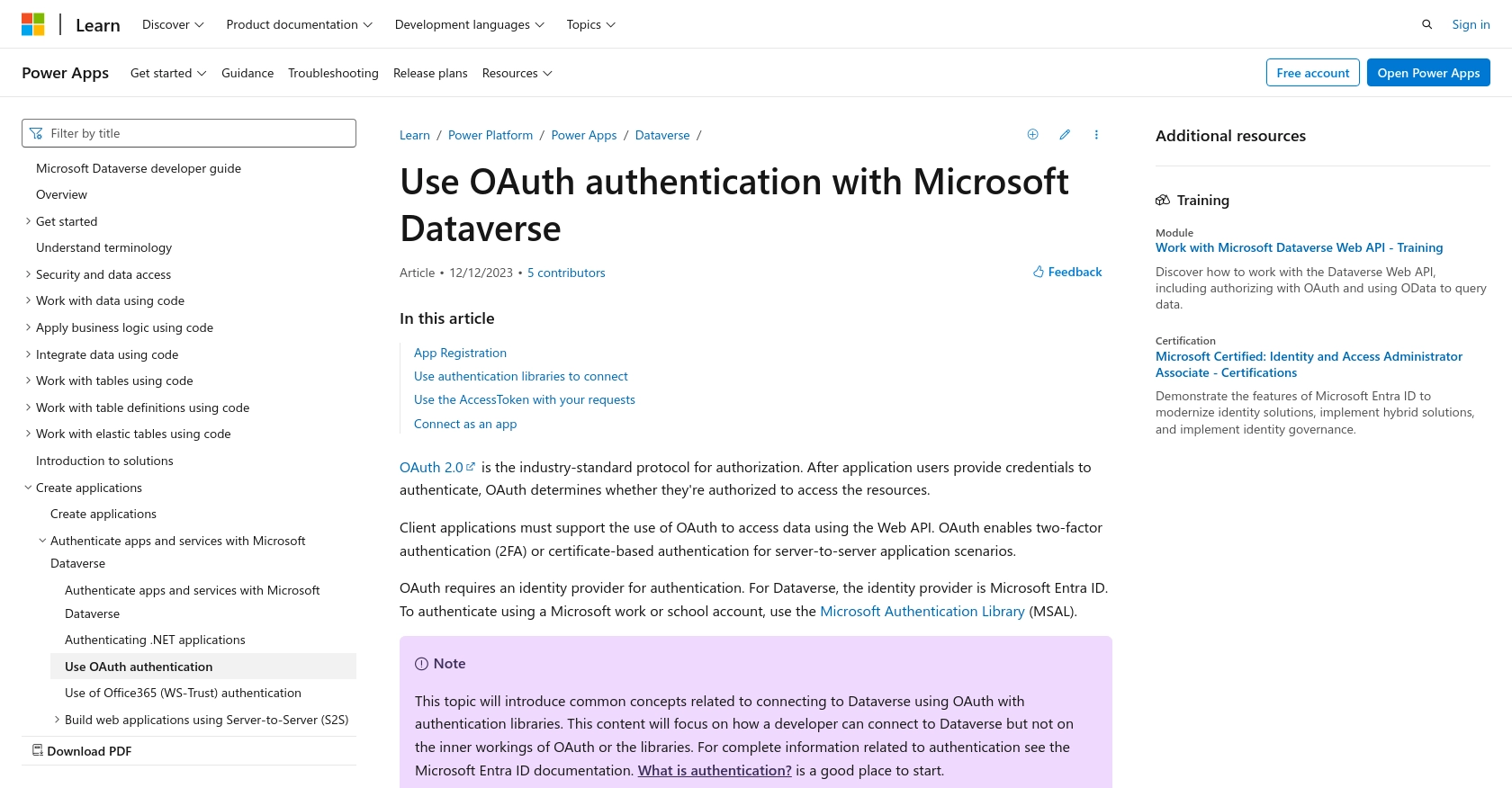
sbb-itb-96038d7
How to Make API Calls to Retrieve Custom Objects from Microsoft Dynamics 365 Using Python
In this section, we'll guide you through the process of making API calls to retrieve custom objects from Microsoft Dynamics 365 using Python. This involves setting up your Python environment, writing the code to interact with the API, and handling potential errors effectively.
Setting Up Your Python Environment for Microsoft Dynamics 365 API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.11.1 and the necessary libraries to handle HTTP requests and OAuth authentication.
- Ensure Python 3.11.1 is installed on your machine. You can download it from the official Python website.
- Install the required libraries using pip. Open your terminal and run the following command:
pip install requests msal
Writing Python Code to Retrieve Custom Objects from Microsoft Dynamics 365
With your environment set up, you can now write the Python code to retrieve custom objects from Microsoft Dynamics 365. Below is a sample script to get you started:
import requests
from msal import ConfidentialClientApplication
# Define your credentials and endpoint
client_id = 'Your_Client_ID'
client_secret = 'Your_Client_Secret'
tenant_id = 'Your_Tenant_ID'
authority = f'https://login.microsoftonline.com/{tenant_id}'
scope = ['https://yourorg.crm.dynamics.com/.default']
endpoint = 'https://yourorg.api.crm.dynamics.com/api/data/v9.2/entities'
# Acquire a token using MSAL
app = ConfidentialClientApplication(client_id, authority=authority, client_credential=client_secret)
token_response = app.acquire_token_for_client(scopes=scope)
# Check if token acquisition was successful
if 'access_token' in token_response:
headers = {
'Authorization': f"Bearer {token_response['access_token']}",
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json'
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for entity in data['value']:
print(entity)
else:
print(f"Failed to retrieve data: {response.status_code} - {response.text}")
else:
print("Failed to acquire token")
Replace Your_Client_ID
, Your_Client_Secret
, and Your_Tenant_ID
with your actual credentials. This script uses the MSAL library to handle OAuth authentication and the requests library to make HTTP calls to the Microsoft Dynamics 365 API.
Verifying API Call Success and Handling Errors
After running the script, verify the success of your API call by checking the output. The retrieved custom objects should be printed in the console. If the request fails, the script will output the error code and message, allowing you to troubleshoot the issue.
Common error codes include:
- 401 Unauthorized: Check your credentials and ensure your app has the necessary permissions.
- 403 Forbidden: Verify that your app has been granted admin consent for the required permissions.
- 404 Not Found: Ensure the endpoint URL is correct and that the resource exists.
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
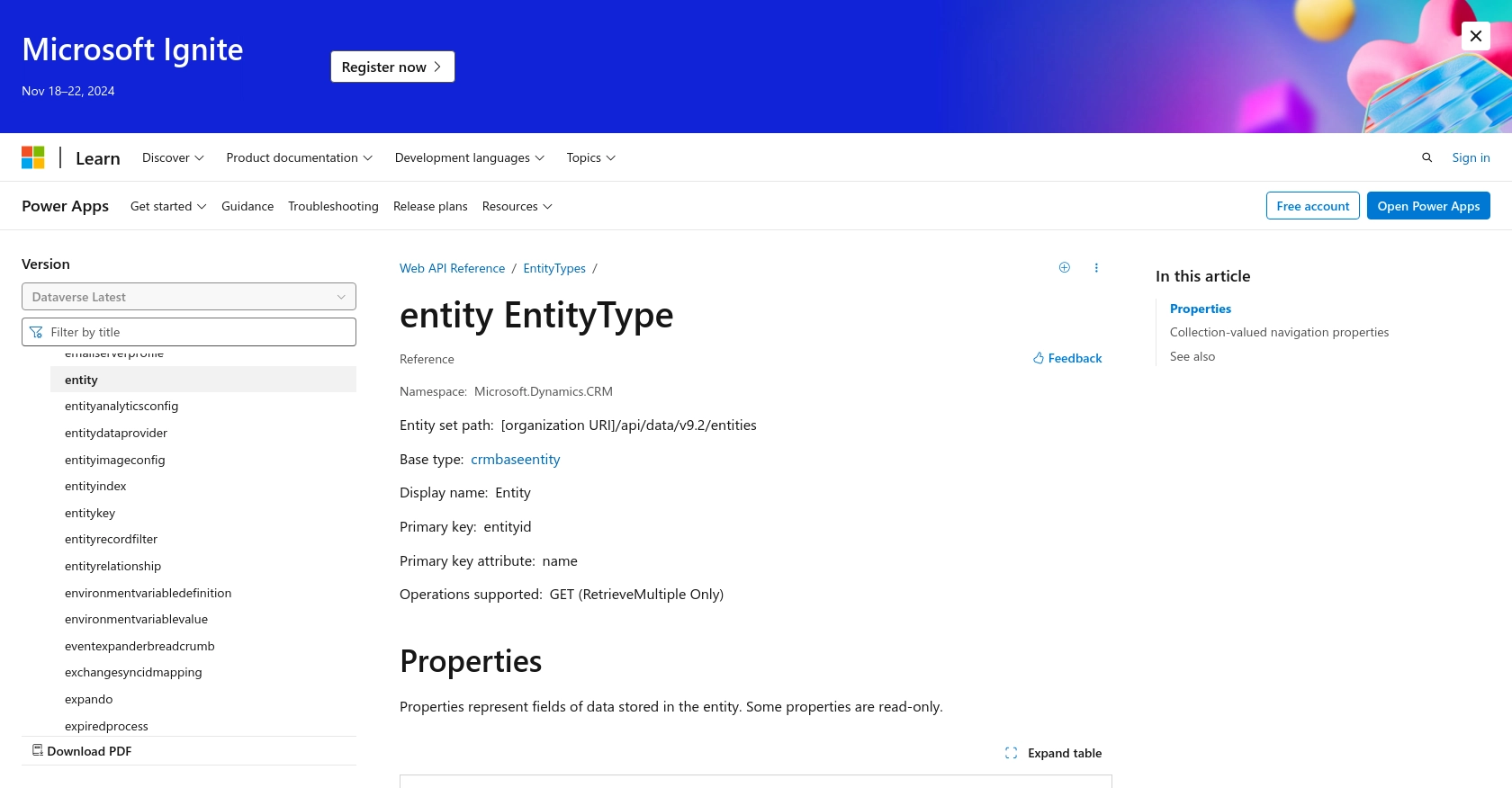
Conclusion and Best Practices for Microsoft Dynamics 365 API Integration
Integrating with the Microsoft Dynamics 365 API using Python provides a powerful way to access and manipulate data within the platform, enabling developers to create tailored solutions that enhance business processes. By following the steps outlined in this guide, you can efficiently retrieve custom objects and leverage the rich data capabilities of Microsoft Dynamics 365.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store sensitive information like client secrets and tokens securely. Consider using environment variables or secure vaults to protect these credentials.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Standardize Data Fields: Ensure consistent data formats when interacting with the API to maintain data integrity across different systems.
- Monitor API Usage: Regularly monitor your API calls and responses to identify any issues or opportunities for optimization.
Streamline Your Integration Process with Endgrate
While integrating with Microsoft Dynamics 365 can be rewarding, it can also be complex and time-consuming. Endgrate offers a solution to simplify this process by providing a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365. By using Endgrate, you can save time and resources, allowing you to focus on your core product development and deliver an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/entity?view=dataverse-latest
Ready to get started?