Using the Klaviyo API to Create or Update Profiles (with Javascript examples)
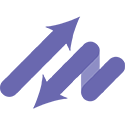
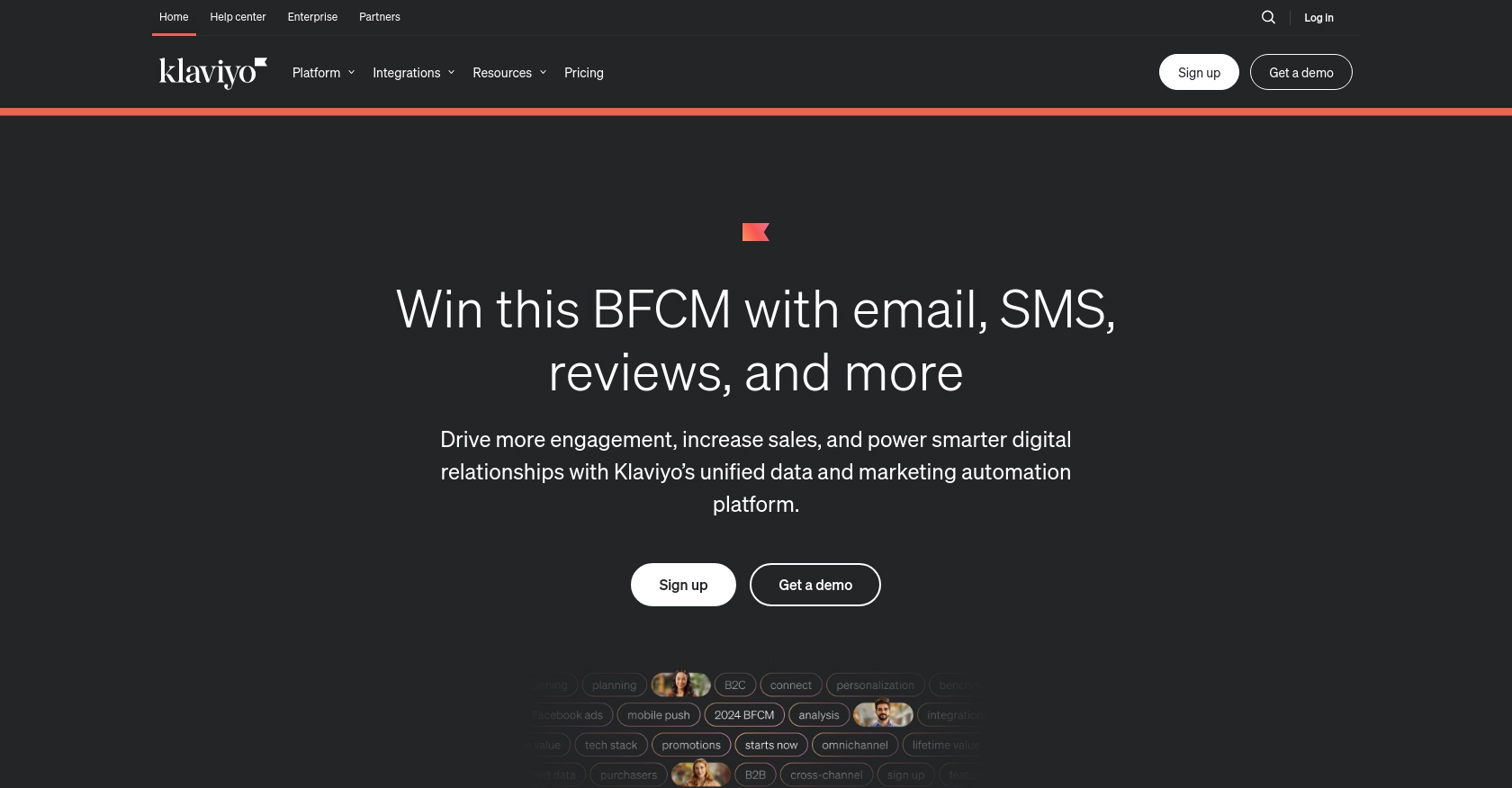
Introduction to Klaviyo's API for Profile Management
Klaviyo is a powerful email and SMS marketing platform that leverages data science to deliver personalized customer experiences at scale. With its robust API, developers can seamlessly integrate Klaviyo into their applications, enabling the creation and management of customer profiles with ease.
Connecting with Klaviyo's API allows developers to automate and enhance marketing strategies by efficiently managing customer data. For example, you can use the API to create or update customer profiles, ensuring that your marketing campaigns are always targeting the most up-to-date information.
This guide will walk you through using JavaScript to interact with Klaviyo's API, focusing on creating and updating profiles. By the end of this tutorial, you'll be equipped to integrate Klaviyo's powerful features into your own applications, streamlining your marketing efforts and improving customer engagement.
Setting Up a Klaviyo Test Account and Obtaining API Credentials
Before you can start integrating with Klaviyo's API, you'll need to set up a test account and obtain the necessary API credentials. This will allow you to authenticate your requests and interact with Klaviyo's platform securely.
Creating a Klaviyo Test Account
If you don't already have a Klaviyo account, you can easily create one by visiting the Klaviyo signup page. Follow the instructions to set up your account. Once your account is created, you'll be able to access the Klaviyo dashboard.
Generating API Credentials in Klaviyo
To interact with Klaviyo's API, you'll need to generate API credentials. Here's how you can do it:
- Log in to your Klaviyo account and navigate to the Account section.
- Under Settings, click on API Keys.
- Click on Create API Key to generate a new private API key. Make sure to copy this key and store it securely, as it will not be displayed again.
It's important to note that Klaviyo uses both public and private API keys. For secure operations such as creating or updating profiles, you'll need to use the private API key, which should never be exposed in client-side code.
For more detailed information on obtaining API credentials, refer to the Klaviyo documentation.
Understanding API Key Scopes
When creating your API key, you can define specific scopes to control access. For profile management, ensure that your API key has the profiles:write
scope enabled. This allows you to create and update profiles using the API.
Testing Your API Setup
Once you have your API credentials, it's a good practice to make a test API request to ensure everything is set up correctly. You can use tools like Postman or cURL to send a request to Klaviyo's API and verify your credentials are working.
For more information on making test API requests, visit the Klaviyo API documentation.
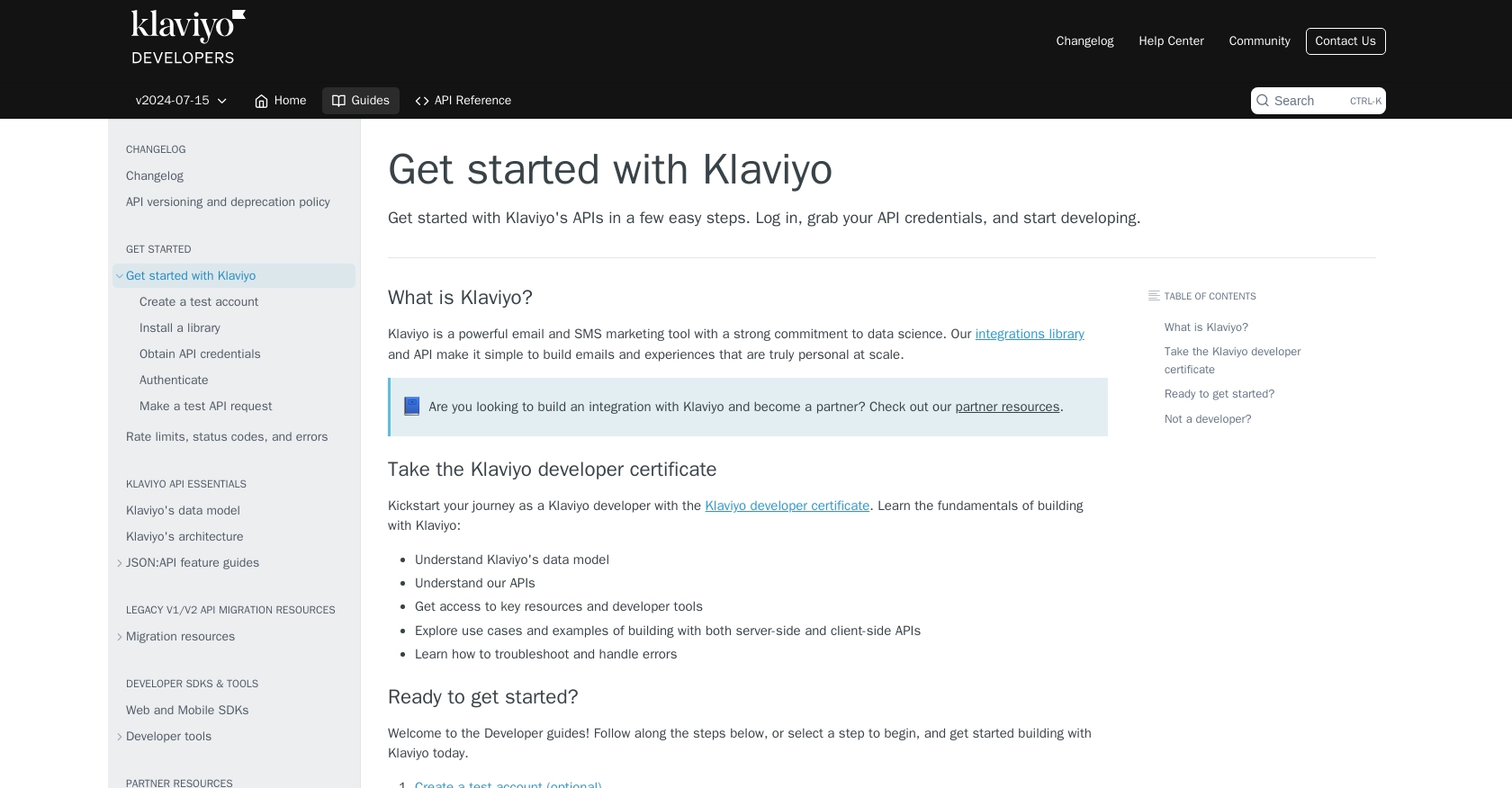
sbb-itb-96038d7
Making API Calls to Create or Update Profiles in Klaviyo Using JavaScript
To interact with Klaviyo's API for creating or updating profiles, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Klaviyo API Integration
Before you start coding, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript outside of a browser, which is essential for server-side operations like API calls.
You'll also need the axios
library, a popular HTTP client for making requests. Install it using npm:
npm install axios
Writing JavaScript Code to Create a Profile in Klaviyo
To create a new profile, you'll send a POST request to Klaviyo's API endpoint. Here's a sample code snippet:
const axios = require('axios');
const createProfile = async () => {
const url = 'https://a.klaviyo.com/api/profiles/';
const apiKey = 'your-private-api-key';
const data = {
data: {
type: 'profile',
attributes: {
email: 'example@example.com',
first_name: 'John',
last_name: 'Doe'
}
}
};
try {
const response = await axios.post(url, data, {
headers: {
'Authorization': `Klaviyo-API-Key ${apiKey}`,
'Content-Type': 'application/json',
'revision': '2024-07-15'
}
});
console.log('Profile Created:', response.data);
} catch (error) {
console.error('Error creating profile:', error.response.data);
}
};
createProfile();
Replace your-private-api-key
with your actual API key. This code sends a request to create a profile with basic attributes like email and name.
Updating an Existing Profile in Klaviyo Using JavaScript
To update an existing profile, use a PATCH request. Here's how you can do it:
const updateProfile = async (profileId) => {
const url = `https://a.klaviyo.com/api/profiles/${profileId}/`;
const apiKey = 'your-private-api-key';
const data = {
data: {
type: 'profile',
id: profileId,
attributes: {
first_name: 'Jane',
last_name: 'Smith'
}
}
};
try {
const response = await axios.patch(url, data, {
headers: {
'Authorization': `Klaviyo-API-Key ${apiKey}`,
'Content-Type': 'application/json',
'revision': '2024-07-15'
}
});
console.log('Profile Updated:', response.data);
} catch (error) {
console.error('Error updating profile:', error.response.data);
}
};
updateProfile('profile-id-here');
Replace profile-id-here
with the ID of the profile you wish to update. This code updates the profile's first and last name.
Handling API Responses and Errors in Klaviyo
When making API calls, it's crucial to handle responses and errors properly. Klaviyo's API provides status codes to indicate success or failure:
- 2xx: Success
- 4xx: Client error (e.g., invalid request)
- 5xx: Server error
For detailed error handling, refer to the Klaviyo documentation. Implement retry mechanisms for 429 and 503 errors, using exponential backoff to manage request rates effectively.
Verifying API Requests in Klaviyo's Dashboard
After making API calls, verify the changes in your Klaviyo dashboard. Newly created or updated profiles should reflect the changes made through your API requests.
By following these steps, you can efficiently manage customer profiles in Klaviyo using JavaScript, enhancing your marketing automation and customer engagement strategies.
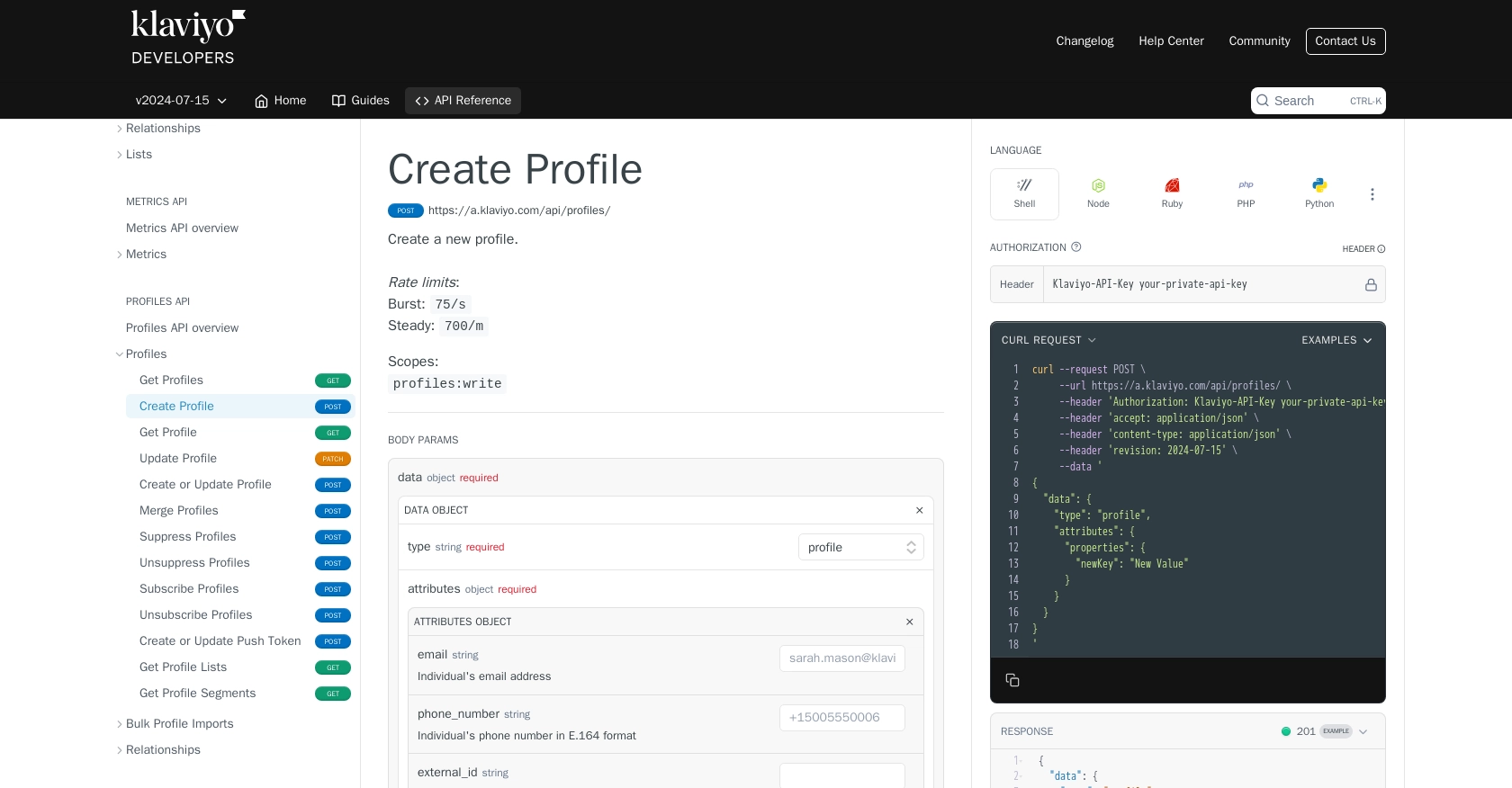
Conclusion and Best Practices for Integrating Klaviyo API with JavaScript
Integrating Klaviyo's API into your applications using JavaScript can significantly enhance your marketing automation and customer engagement strategies. By following the steps outlined in this guide, you can efficiently create and update customer profiles, ensuring your marketing campaigns are always based on the most current data.
Best Practices for Secure and Efficient API Integration with Klaviyo
- Secure API Key Management: Always store your private API keys securely and never expose them in client-side code. Consider using environment variables or secure vaults for storing sensitive information.
- Handle Rate Limits: Be mindful of Klaviyo's rate limits to avoid hitting the maximum request thresholds. Implement retry mechanisms with exponential backoff for handling 429 (Rate Limit) and 503 (Service Unavailable) errors. For more details, refer to the Klaviyo documentation.
- Data Standardization: Ensure consistency in data fields when creating or updating profiles. This helps maintain data integrity across your marketing platforms.
- Monitor API Usage: Regularly monitor your API usage and performance through Klaviyo's dashboard to optimize your integration and identify any potential issues early.
Enhancing Your Integration Strategy with Endgrate
While integrating with Klaviyo's API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Klaviyo. This allows you to build once for each use case instead of multiple times for different integrations, saving you time and resources.
With Endgrate, you can focus on your core product while outsourcing the complexities of integration management. Explore how Endgrate can simplify your integration strategy by visiting Endgrate's website.
By leveraging these best practices and tools, you can ensure a robust and efficient integration with Klaviyo, enhancing your marketing efforts and delivering personalized experiences to your customers.
Read More
- https://endgrate.com/provider/klaviyo
- https://developers.klaviyo.com/en/docs/get_started
- https://developers.klaviyo.com/en/docs/retrieve_api_credentials
- https://developers.klaviyo.com/en/docs/rate_limits_and_error_handling
- https://developers.klaviyo.com/en/reference/create_profile
- https://developers.klaviyo.com/en/reference/update_profile
Ready to get started?