Using the ZoomInfo API to Get Contacts in PHP
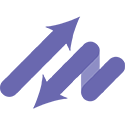
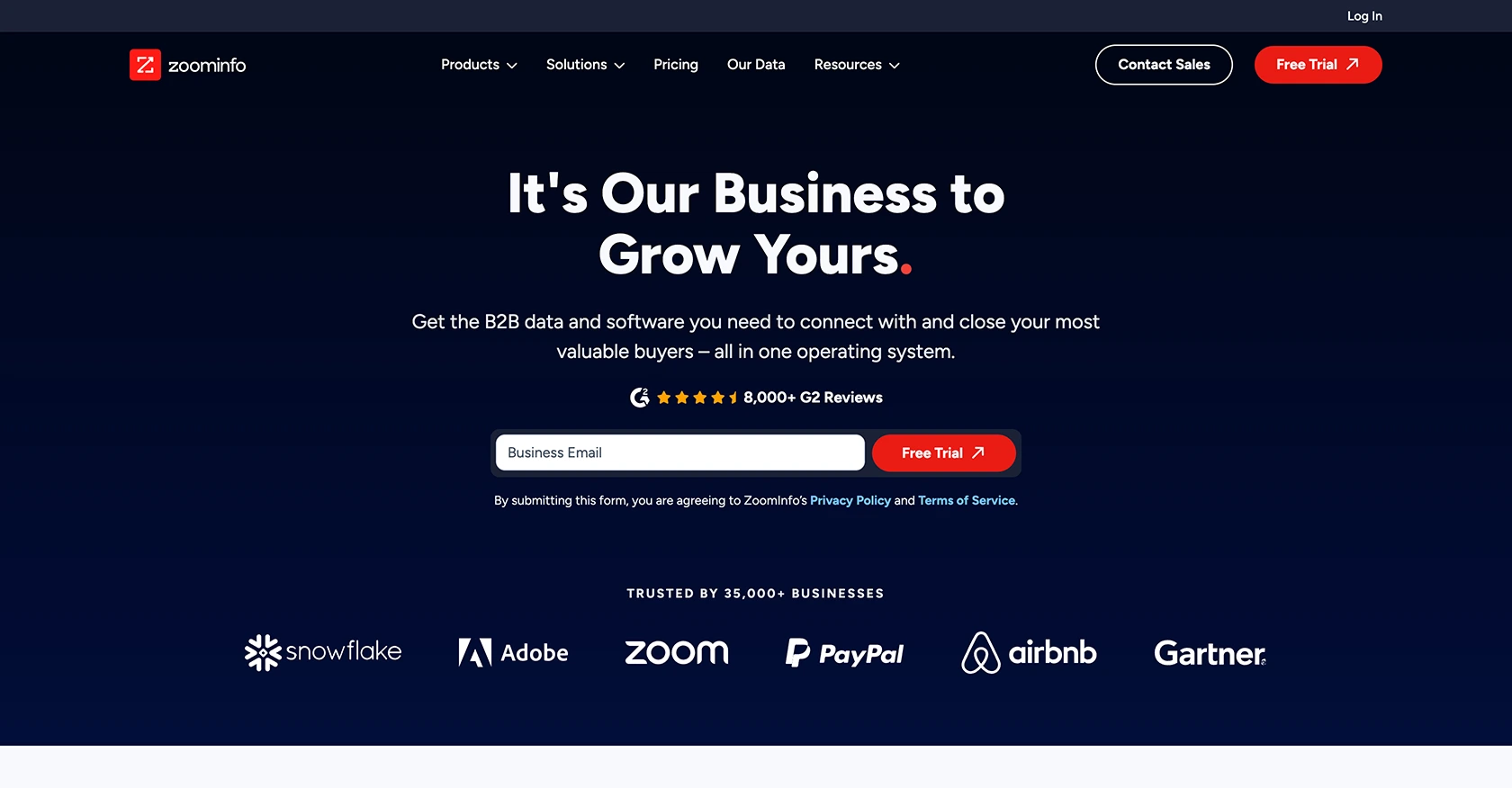
Introduction to ZoomInfo API
ZoomInfo is a powerful business intelligence platform that provides comprehensive data on companies and professionals. It offers a wealth of information that can be leveraged for sales, marketing, and recruitment efforts. With its extensive database, ZoomInfo helps businesses connect with the right contacts and make informed decisions.
Integrating with the ZoomInfo API allows developers to access and manage contact data programmatically. This can be particularly useful for automating lead generation processes or enriching CRM data with up-to-date contact information. For example, a developer might use the ZoomInfo API to retrieve contact details of potential leads and integrate them into a sales pipeline, enhancing the efficiency of outreach efforts.
Setting Up a ZoomInfo Test/Sandbox Account for API Integration
Before you can start using the ZoomInfo API to access contact data, you'll need to set up a test or sandbox account. This will allow you to experiment with the API without affecting live data. ZoomInfo provides a custom authentication method, which you will need to configure to access their API.
Creating a ZoomInfo Developer Account
To begin, you'll need to create a ZoomInfo developer account. Visit the ZoomInfo API documentation and follow the instructions to sign up for a developer account. This account will give you access to the necessary credentials for API authentication.
Generating API Credentials
Once your developer account is set up, you need to generate API credentials. These include a client ID and client secret, which are essential for authenticating your API requests.
- Log in to your ZoomInfo developer account.
- Navigate to the API credentials section.
- Click on "Generate New Credentials" to create your client ID and client secret.
- Store these credentials securely, as you'll need them to authenticate your API requests.
Configuring Custom Authentication
ZoomInfo uses a custom authentication method. To authenticate your API requests, you will need to include your client ID and client secret in the request headers. Refer to the ZoomInfo authentication documentation for detailed instructions on how to configure your requests.
Testing Your API Setup
With your credentials in place, you can now test your API setup. Use a tool like Postman or a simple PHP script to make a test API call. Ensure that your request includes the correct headers and credentials. If successful, you should receive a response with contact data from the ZoomInfo sandbox environment.
By setting up a test account and configuring authentication, you're now ready to explore the capabilities of the ZoomInfo API and integrate it into your applications.
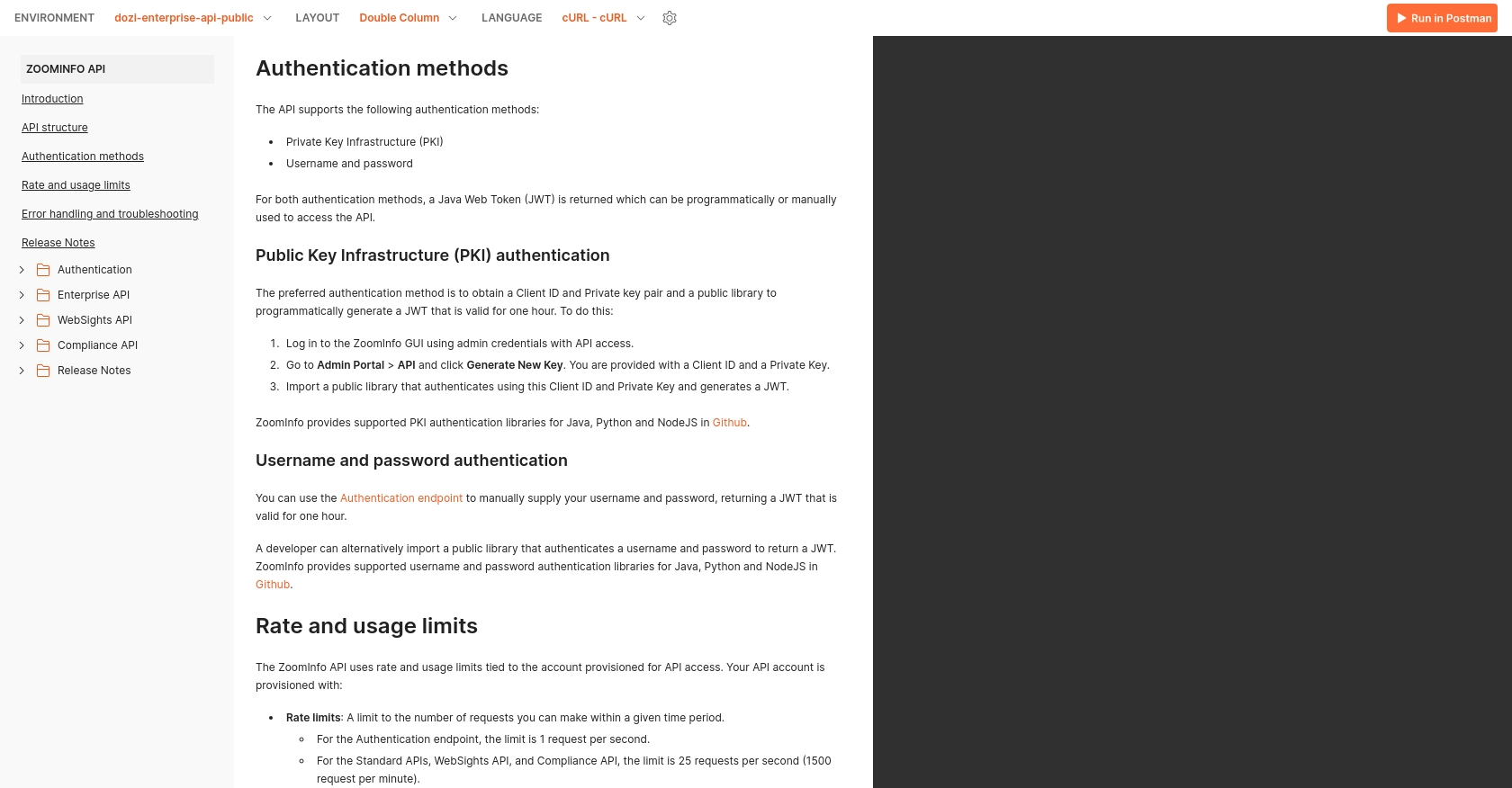
sbb-itb-96038d7
Making API Calls to ZoomInfo Using PHP
To interact with the ZoomInfo API and retrieve contact data, you'll need to set up your PHP environment and make authenticated API calls. This section will guide you through the necessary steps to achieve this.
Setting Up Your PHP Environment for ZoomInfo API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the cURL extension enabled to handle HTTP requests.
- Check your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m | grep curl
.
Installing Required PHP Dependencies
To make HTTP requests, you'll use the Guzzle HTTP client, a popular PHP library. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Contacts from ZoomInfo API
With your environment set up, you can now write PHP code to make an API call to ZoomInfo and retrieve contact data. Below is a sample script:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$clientId = 'Your_Client_ID';
$clientSecret = 'Your_Client_Secret';
$response = $client->request('GET', 'https://api.zoominfo.com/contacts', [
'headers' => [
'Authorization' => 'Basic ' . base64_encode("$clientId:$clientSecret"),
'Content-Type' => 'application/json'
]
]);
if ($response->getStatusCode() === 200) {
$contacts = json_decode($response->getBody(), true);
foreach ($contacts['data'] as $contact) {
echo 'Name: ' . $contact['firstName'] . ' ' . $contact['lastName'] . "\n";
echo 'Email: ' . $contact['email'] . "\n\n";
}
} else {
echo 'Failed to retrieve contacts. Status code: ' . $response->getStatusCode();
}
Replace Your_Client_ID
and Your_Client_Secret
with your actual ZoomInfo API credentials.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of contact names and emails if the request is successful. If the request fails, the script will output the status code, helping you diagnose the issue.
Common error codes include:
- 401 Unauthorized: Check your API credentials.
- 403 Forbidden: Ensure your account has the necessary permissions.
- 500 Internal Server Error: Retry the request or contact ZoomInfo support.
For more detailed error handling, refer to the ZoomInfo API documentation.
Best Practices for Using the ZoomInfo API in PHP
When integrating with the ZoomInfo API, it's crucial to follow best practices to ensure efficient and secure interactions. Here are some recommendations:
- Securely Store Credentials: Always store your ZoomInfo API credentials securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by ZoomInfo. Implement exponential backoff strategies to handle rate limit errors gracefully and avoid overwhelming the API.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your existing systems. This will help maintain data integrity across platforms.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Log errors for further analysis and troubleshooting.
Enhancing Integration Efficiency with Endgrate
While integrating with the ZoomInfo API can significantly enhance your business processes, managing multiple integrations can be time-consuming and complex. This is where Endgrate can make a difference.
Endgrate offers a unified API solution that simplifies the integration process. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of integration.
- Build Once, Use Multiple Times: Create a single integration for each use case and apply it across various platforms, reducing redundancy.
- Provide a Seamless Experience: Deliver an intuitive integration experience for your customers, enhancing satisfaction and retention.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?