How to Get Credit Notes with the Chargebee API in PHP
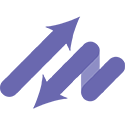
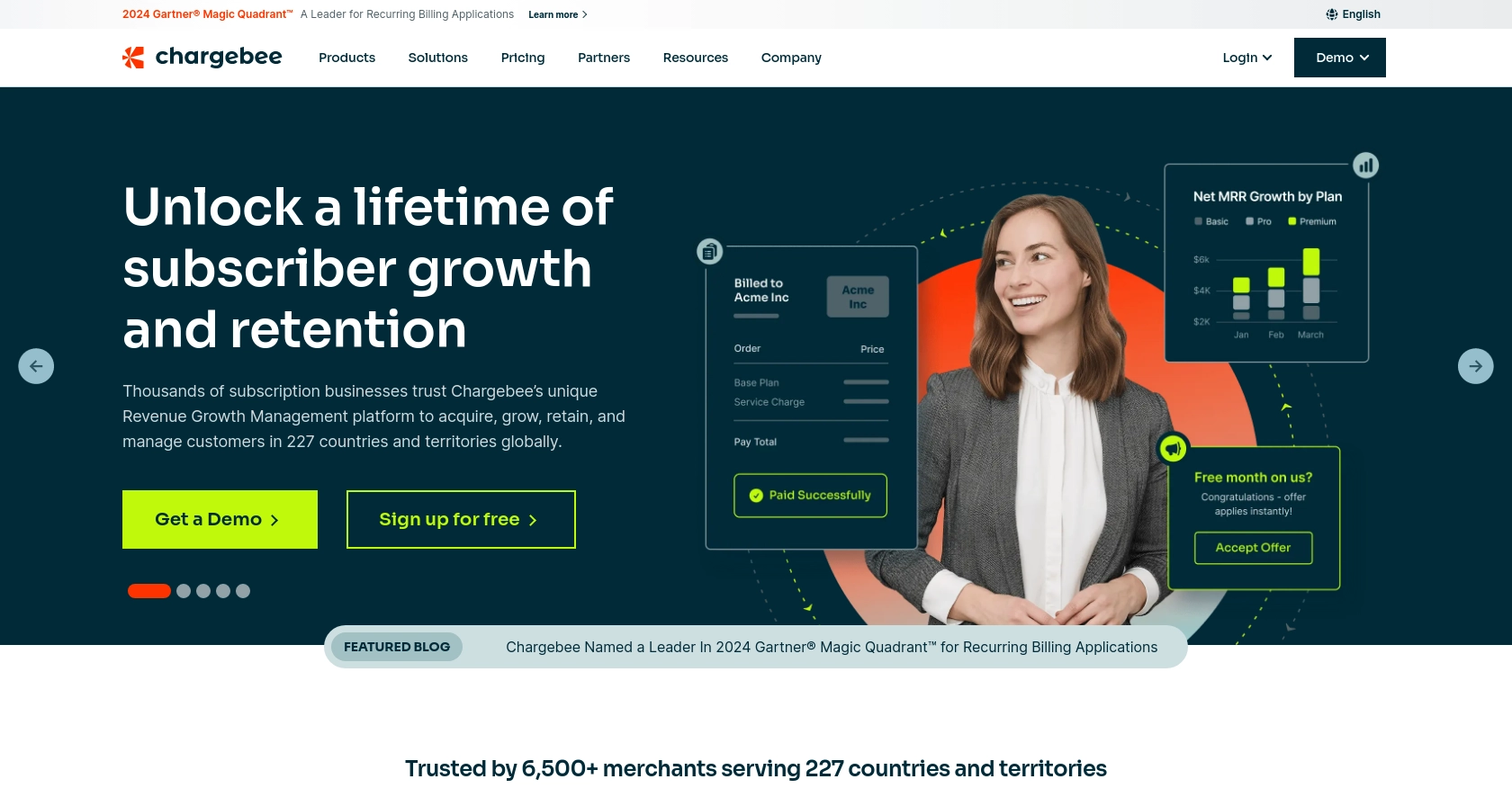
Introduction to Chargebee API for Credit Notes
Chargebee is a robust subscription management and billing platform that caters to businesses of all sizes. It offers a comprehensive suite of tools to manage subscriptions, invoicing, and revenue operations efficiently. Chargebee's flexibility and scalability make it a preferred choice for SaaS companies looking to streamline their billing processes.
Integrating with Chargebee's API allows developers to automate and manage various billing operations, such as handling credit notes. Credit notes are essential for adjusting invoices and managing refunds, providing businesses with the flexibility to handle customer credits effectively.
For example, a developer might want to retrieve credit notes to generate financial reports or automate refund processes. By using the Chargebee API, developers can seamlessly access and manage credit notes, enhancing the efficiency of their billing systems.
Setting Up Your Chargebee Test Account for API Integration
Before you can start interacting with the Chargebee API to manage credit notes, you'll need to set up a test account. This allows you to safely experiment with API calls without affecting live data. Chargebee provides a sandbox environment specifically for this purpose.
Step-by-Step Guide to Creating a Chargebee Sandbox Account
-
Sign Up for a Chargebee Account:
Visit the Chargebee website and sign up for a free trial. This will give you access to the sandbox environment where you can test API integrations.
-
Access the Sandbox Environment:
Once your account is created, log in to the Chargebee dashboard. Navigate to the 'Sites' section and select 'Create a Sandbox Site' to set up your testing environment.
-
Generate API Keys:
In the sandbox environment, go to 'Settings' > 'API Keys'. Click on 'Create API Key' to generate a new key. This key will be used for authenticating your API requests.
Authenticating API Requests with Chargebee
Chargebee uses HTTP Basic authentication for API calls. The API key acts as the username, and the password field should be left empty. This method ensures secure access to your Chargebee data.
// Example of setting up authentication in PHP
$apiKey = 'your_api_key_here';
$site = 'your_site_here';
$ch = curl_init("https://$site.chargebee.com/api/v2/credit_notes");
curl_setopt($ch, CURLOPT_USERPWD, $apiKey . ":");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
Replace your_api_key_here
and your_site_here
with your actual API key and site name. This setup will allow you to authenticate and make API calls to the Chargebee sandbox environment.
For more details on authentication, refer to the Chargebee API documentation.
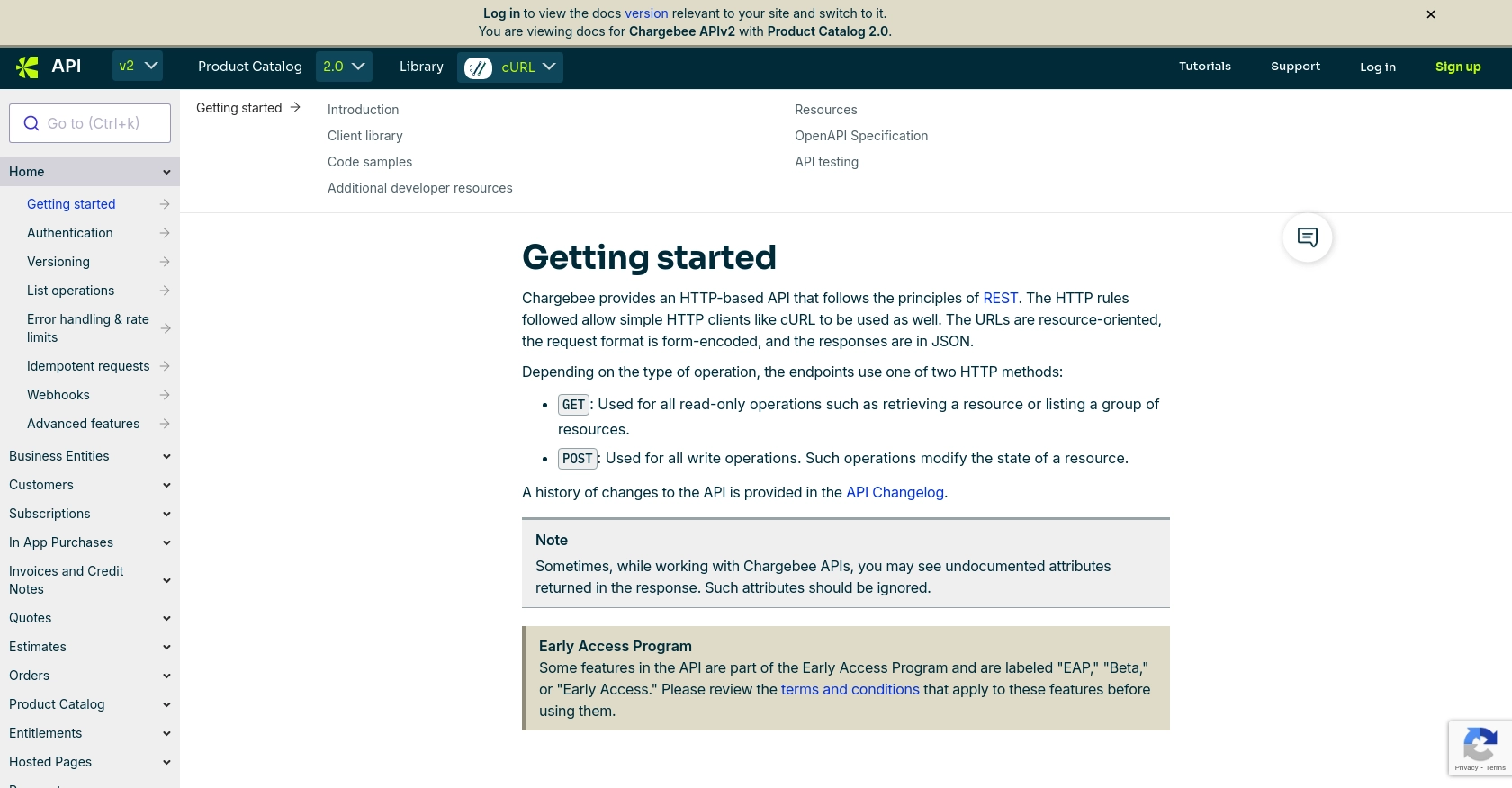
sbb-itb-96038d7
Making API Calls to Retrieve Credit Notes with Chargebee in PHP
To interact with the Chargebee API and retrieve credit notes using PHP, you'll need to set up your environment and write the necessary code to make the API call. This section will guide you through the process, ensuring you can efficiently access credit notes data from Chargebee.
Setting Up Your PHP Environment for Chargebee API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP version 7.0 or higher and the cURL extension enabled. If not already installed, you can add cURL using the following command:
sudo apt-get install php-curl
Writing PHP Code to Retrieve Credit Notes from Chargebee
Once your environment is set up, you can proceed to write the PHP code to make the API call. Below is a sample script to retrieve credit notes:
<?php
$apiKey = 'your_api_key_here';
$site = 'your_site_here';
// Initialize cURL session
$ch = curl_init("https://$site.chargebee.com/api/v2/credit_notes");
// Set cURL options
curl_setopt($ch, CURLOPT_USERPWD, $apiKey . ":");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPGET, true);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse and display the response
$creditNotes = json_decode($response, true);
foreach ($creditNotes['list'] as $creditNote) {
echo "Credit Note ID: " . $creditNote['credit_note']['id'] . "<br>";
echo "Amount: " . $creditNote['credit_note']['total'] . "<br>";
}
}
// Close cURL session
curl_close($ch);
?>
Replace your_api_key_here
and your_site_here
with your actual Chargebee API key and site name. This script initializes a cURL session, sets the necessary options for authentication, and makes a GET request to the Chargebee API to retrieve credit notes.
Verifying Successful API Requests and Handling Errors
After executing the API call, you should verify the response to ensure the request was successful. The response will contain the credit notes data, which you can parse and display as needed. If there are any errors, the script will output the error message.
For more information on error handling, refer to the Chargebee API error handling documentation.
By following these steps, you can efficiently retrieve credit notes from Chargebee using PHP, allowing you to automate and streamline your billing processes.
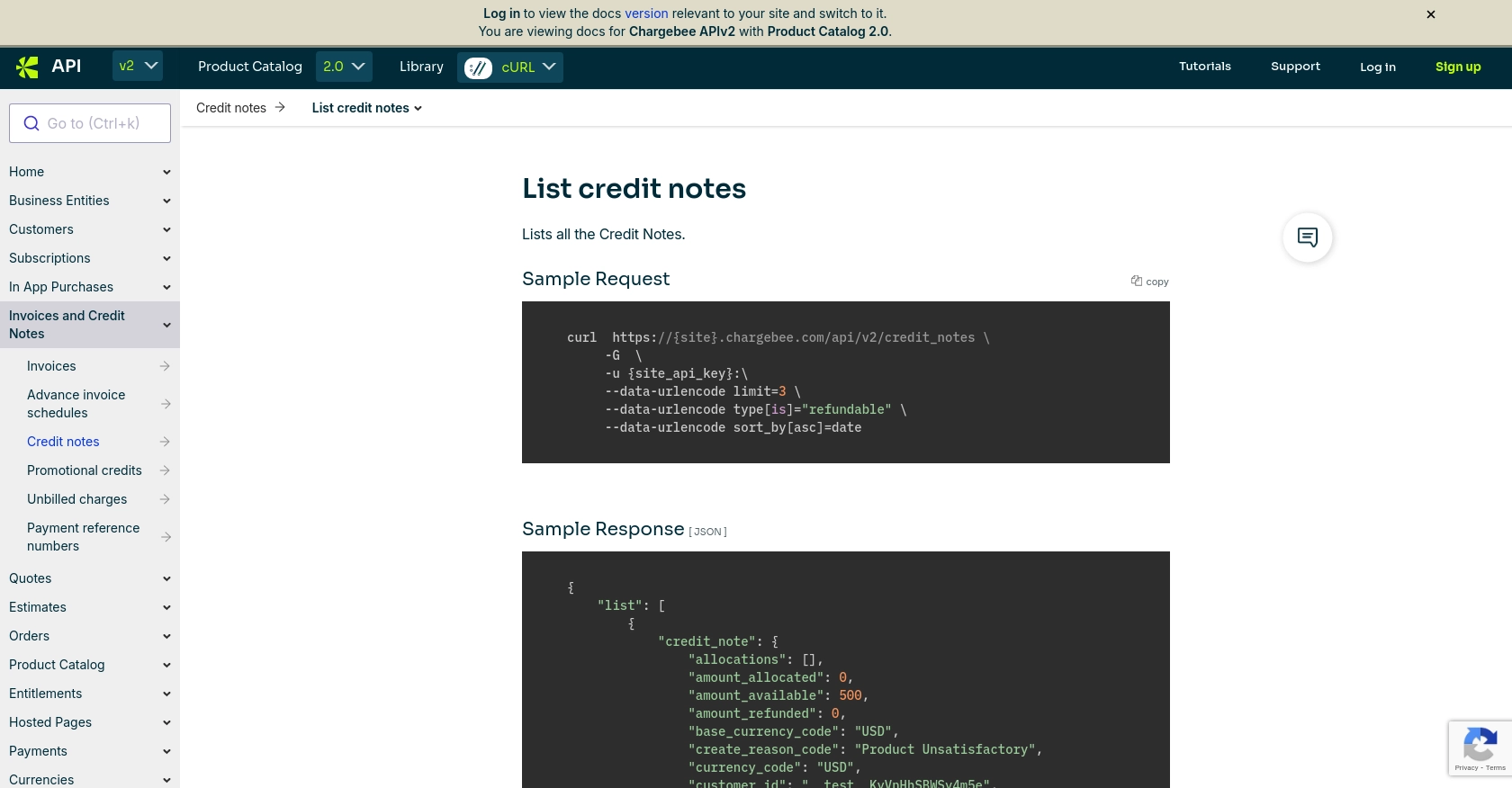
Conclusion and Best Practices for Using Chargebee API in PHP
Integrating with the Chargebee API to manage credit notes in PHP offers a powerful way to streamline billing operations and automate financial processes. By following the steps outlined in this guide, developers can efficiently retrieve and handle credit notes, enhancing their SaaS product's billing capabilities.
Best Practices for Secure and Efficient API Integration with Chargebee
- Secure API Key Management: Store your API keys securely and avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Chargebee imposes rate limits on API requests. Implement exponential backoff strategies to handle HTTP 429 errors gracefully and avoid exceeding request limits. For more details, refer to the Chargebee API rate limits documentation.
- Data Transformation and Standardization: Ensure that data retrieved from Chargebee is transformed and standardized to fit your application's requirements, maintaining consistency across different systems.
- Comprehensive Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and provide meaningful feedback to users when issues arise.
By adhering to these best practices, developers can ensure a secure, efficient, and reliable integration with Chargebee, allowing them to focus on building core product features while leveraging Chargebee's robust billing capabilities.
Streamline Your Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Chargebee, allowing you to build once and deploy across multiple services. This approach saves time and resources, enabling you to focus on your core product development.
Visit Endgrate to learn more about how you can enhance your integration experience and deliver a seamless solution to your customers.
Read More
Ready to get started?