Using the Copper API to Get Leads in Python
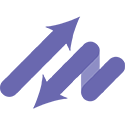
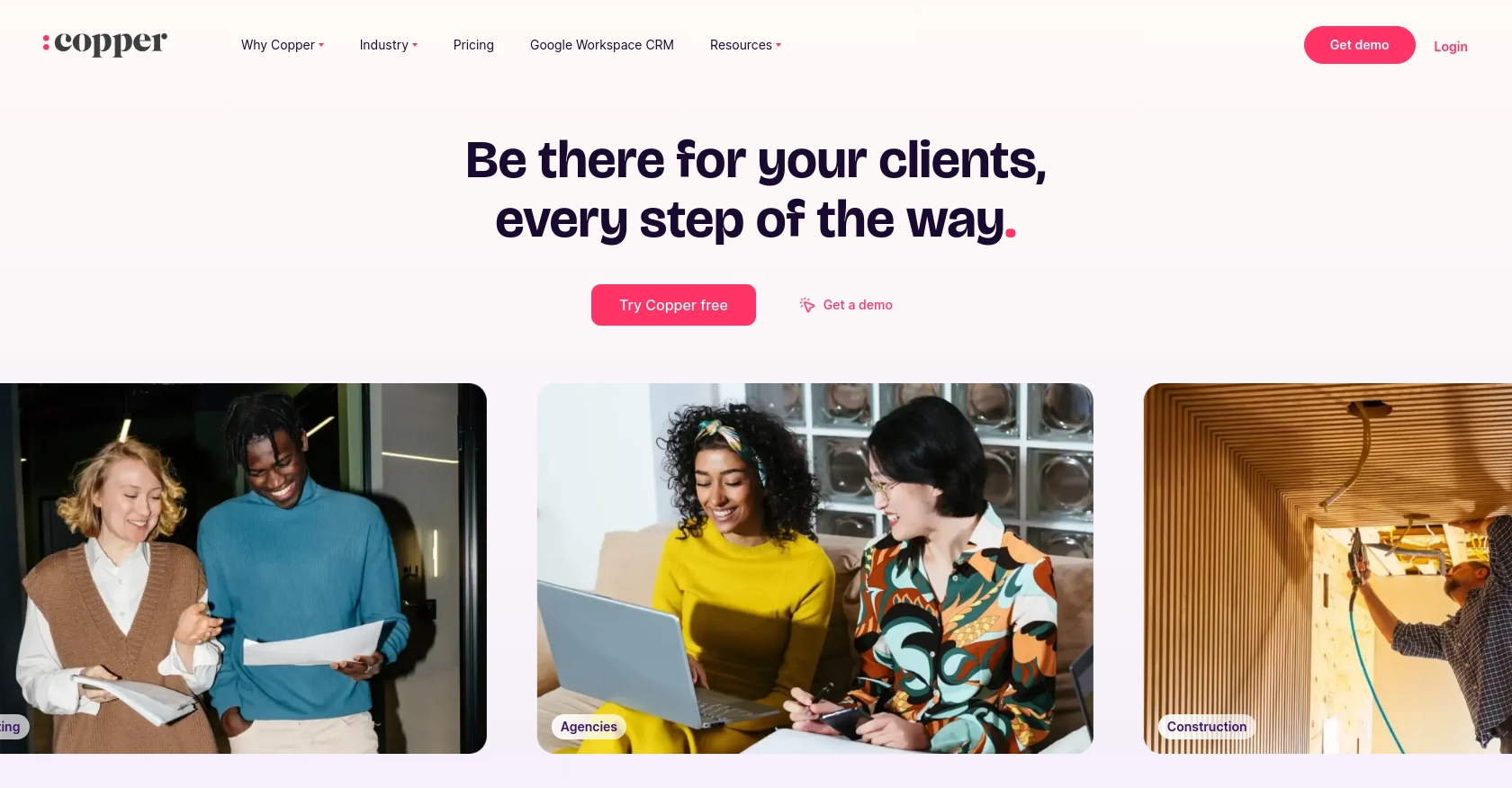
Introduction to Copper CRM API Integration
Copper is a powerful CRM platform designed to seamlessly integrate with Google Workspace, providing businesses with a streamlined way to manage customer relationships. Its user-friendly interface and robust features make it a popular choice for organizations looking to enhance their sales and marketing efforts.
Developers may want to connect with Copper's API to automate and optimize lead management processes. For example, by using the Copper API, developers can retrieve and manage leads directly within their applications, enabling more efficient tracking and follow-up of potential customers.
Setting Up Your Copper CRM Test Account
Before you can start integrating with the Copper API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Copper CRM Sandbox Account
To begin, you'll need to create a Copper CRM account. If you don't already have one, you can sign up for a free trial on the Copper website. This trial will give you access to all the features you need to test the API integration.
- Visit the Copper website and navigate to the sign-up page.
- Follow the instructions to create your account, providing necessary details such as your email and company information.
- Once your account is created, you'll be logged into the Copper dashboard.
Generating Copper API Keys for Authentication
Copper uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your Copper account and navigate to the settings page.
- Under the "Integrations" section, find the "API Keys" option.
- Click on "Create API Key" and provide a name for your key to identify it later.
- Copy the generated API key and API key secret. Store them securely, as you'll need them for authentication in your application.
Configuring Copper API Access
With your API key and secret in hand, you can now configure your application to interact with the Copper API. Ensure that your application includes the necessary headers for authentication:
import hashlib
import hmac
import requests
import time
ApiKey = 'your_api_key'
Secret = 'your_api_secret'
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/v1/leads"
body = ""
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
url = 'https://api.copper.co' + path
response = requests.get(url, headers={
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
})
print(response.json())
Replace your_api_key
and your_api_secret
with the values you obtained earlier. This code snippet demonstrates how to authenticate and make a simple GET request to retrieve leads from Copper.
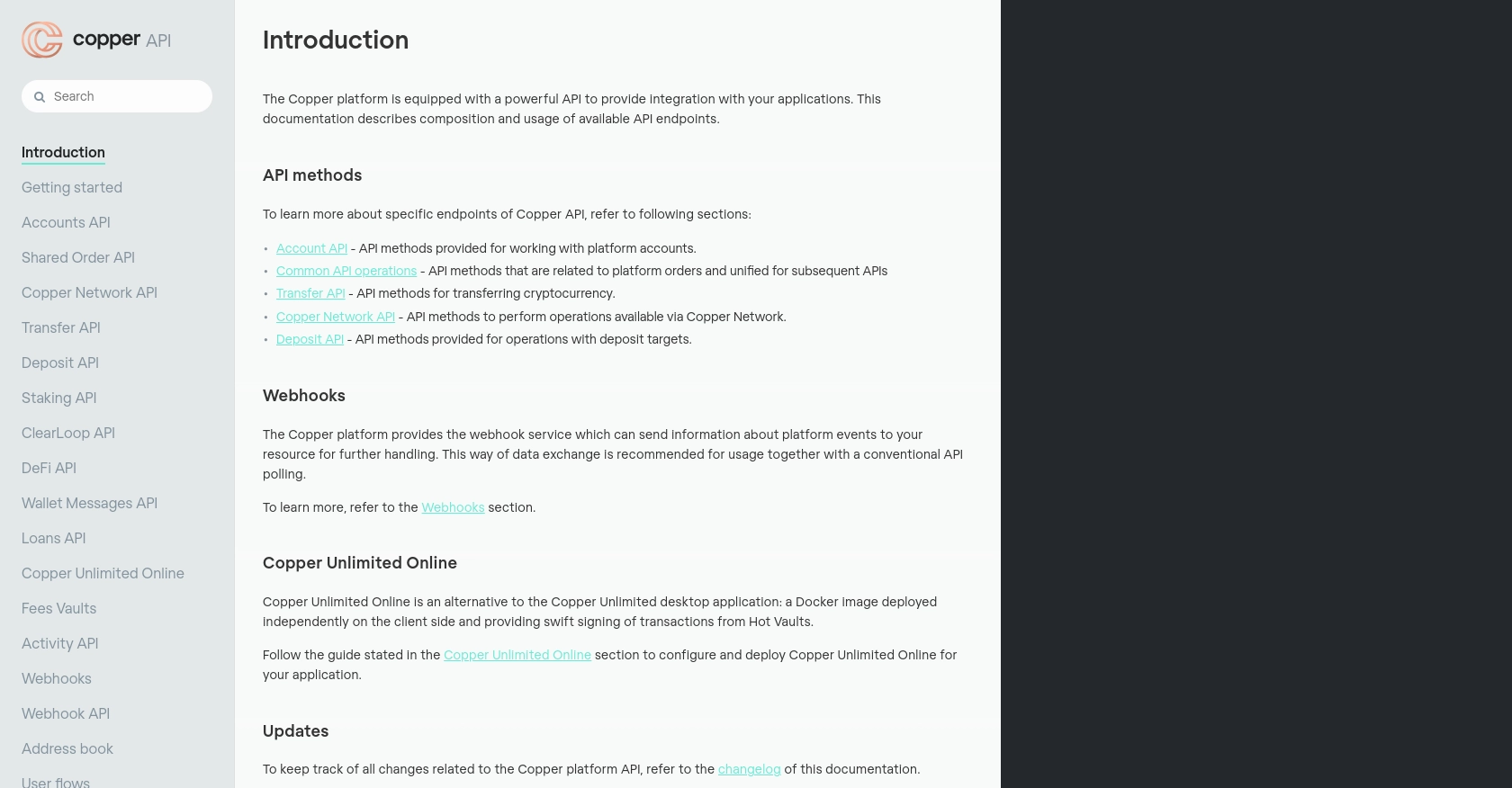
sbb-itb-96038d7
Making API Calls to Retrieve Leads Using Copper API in Python
To effectively interact with the Copper API and retrieve leads, you'll need to ensure your Python environment is correctly set up. This section will guide you through the necessary steps, including setting up your Python environment, writing the code to make API calls, and handling potential errors.
Setting Up Your Python Environment for Copper API Integration
Before making API calls, ensure you have the right version of Python and necessary libraries installed. For this tutorial, we recommend using Python 3.7 or later.
- Ensure Python is installed on your machine. You can download it from the official Python website.
- Install the
requests
library, which is essential for making HTTP requests. You can install it using pip:
pip install requests
Writing Python Code to Make Copper API Calls
With your environment set up, you can now write the Python code to interact with the Copper API. Below is an example of how to retrieve leads using the Copper API:
import hashlib
import hmac
import requests
import time
# Replace with your Copper API credentials
ApiKey = 'your_api_key'
Secret = 'your_api_secret'
# Set up the request details
timestamp = str(round(time.time() * 1000))
method = "GET"
path = "/v1/leads"
body = ""
# Generate the signature for authentication
signature = hmac.new(
key=bytes(Secret, 'utf-8'),
msg=bytes(timestamp + method + path + body, 'utf-8'),
digestmod=hashlib.sha256
).hexdigest()
# Make the API call
url = 'https://api.copper.co' + path
response = requests.get(url, headers={
'Authorization': 'ApiKey ' + ApiKey,
'X-Signature': signature,
'X-Timestamp': timestamp
})
# Output the response
print(response.json())
Replace your_api_key
and your_api_secret
with the credentials you generated earlier. This script authenticates with the Copper API and retrieves a list of leads.
Verifying API Call Success and Handling Errors
After executing the API call, it's crucial to verify the success of the request and handle any errors that may occur. Check the response status code to ensure the request was successful:
if response.status_code == 200:
print("Leads retrieved successfully:", response.json())
else:
print("Failed to retrieve leads. Status code:", response.status_code)
print("Error message:", response.json().get('error', 'No error message'))
By checking the status code, you can determine if the API call was successful (status code 200) or if there was an error. The error message can provide additional context for troubleshooting.
Testing and Debugging Your Copper API Integration
It's essential to test your integration thoroughly in the Copper sandbox environment before deploying it to production. This ensures that your application handles all possible scenarios and errors gracefully.
- Test various scenarios, such as retrieving leads with different filters or handling network errors.
- Use logging to capture detailed information about API requests and responses for debugging purposes.
By following these steps, you can successfully integrate with the Copper API and automate lead retrieval processes in your application.
Best Practices for Copper API Integration and Lead Management
Successfully integrating with the Copper API requires adherence to best practices to ensure secure and efficient data handling. Here are some recommendations:
- Securely Store API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Copper API has rate limits to prevent abuse. Ensure your application respects these limits to avoid being blocked. Implement exponential backoff strategies for retrying failed requests.
- Handle Errors Gracefully: Implement robust error handling to manage different HTTP status codes and error messages. This will help in diagnosing issues and maintaining a smooth user experience.
- Log API Interactions: Maintain logs of API requests and responses for auditing and debugging purposes. This can help in tracking down issues and understanding usage patterns.
- Regularly Update Your Integration: Keep your integration up-to-date with the latest Copper API changes and enhancements. Regular updates ensure compatibility and access to new features.
Streamline Your Integration Process with Endgrate
Integrating multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Copper. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of API integrations.
- Build Once, Deploy Everywhere: Create a single integration that works across multiple platforms, reducing development overhead.
- Enhance Customer Experience: Offer your customers a seamless integration experience with minimal effort.
Visit Endgrate to learn more about how you can streamline your integration processes and enhance your application's capabilities.
Read More
Ready to get started?