Using the NetHunt API to Get Records in Javascript
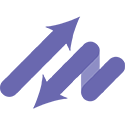
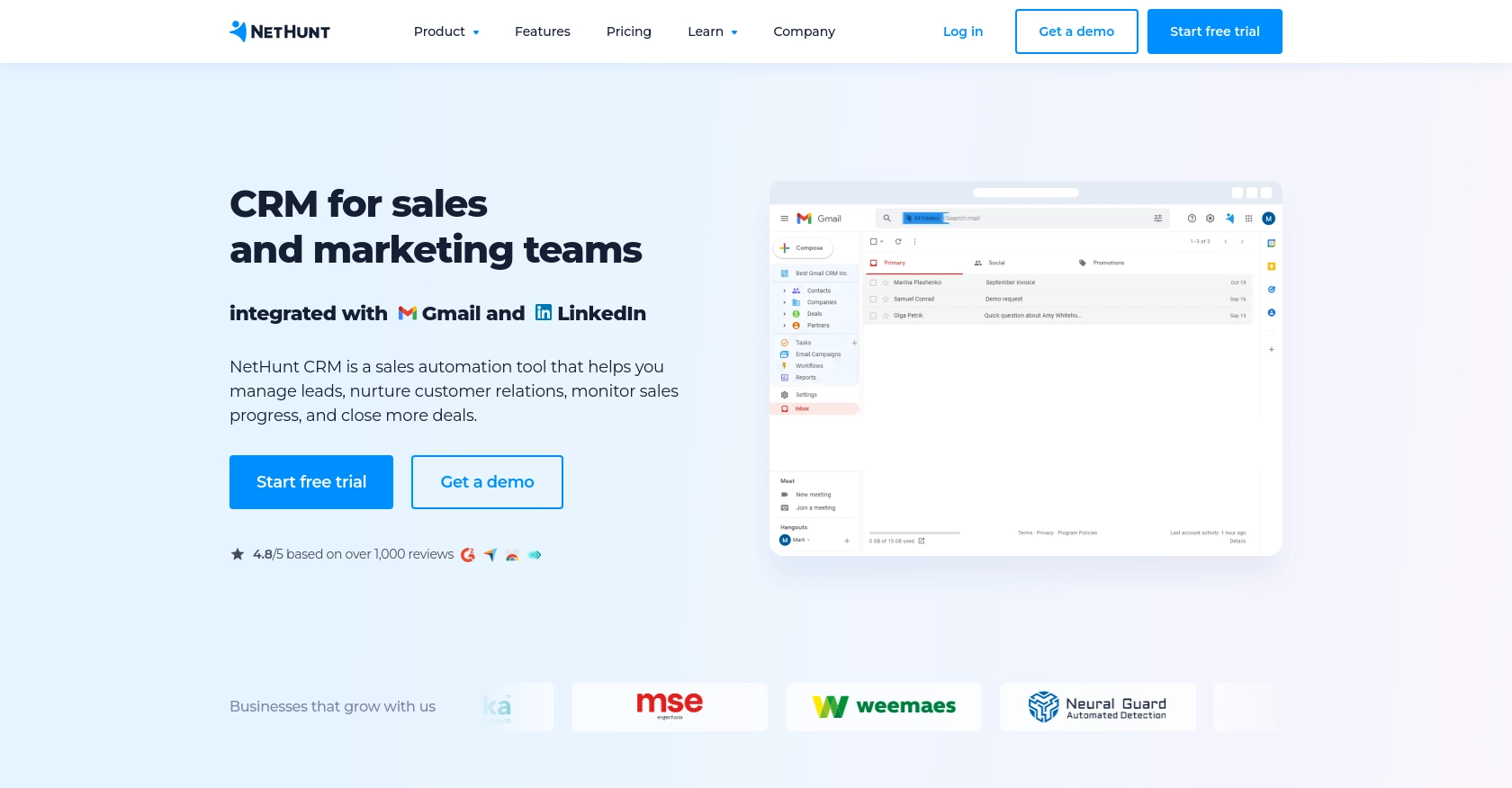
Introduction to NetHunt CRM
NetHunt CRM is a robust customer relationship management solution that seamlessly integrates with Gmail, providing businesses with a powerful tool to manage their customer interactions directly from their inbox. With features like lead management, sales automation, and email campaigns, NetHunt CRM is designed to enhance productivity and streamline workflows for businesses of all sizes.
Developers may want to integrate with NetHunt's API to automate and customize CRM functionalities, such as retrieving and managing records. For example, using the NetHunt API, a developer can fetch customer records and update them in real-time, allowing for a more dynamic and responsive CRM system.
Setting Up Your NetHunt CRM Test Account
Before you can start interacting with the NetHunt API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Creating a NetHunt CRM Account
If you don't already have a NetHunt CRM account, you can sign up for a free trial on their website. This will give you access to all the features you need to test the API integration.
- Visit the NetHunt CRM website.
- Click on "Start free trial" and fill out the registration form.
- Once registered, log in to your account to access the dashboard.
Generating Your NetHunt API Key
To authenticate your API requests, you'll need to generate an API key. This key will be used to verify your identity when making API calls.
- Navigate to the "Settings" section in your NetHunt CRM dashboard.
- Select "Integrations" from the menu.
- Follow the instructions to generate your API key.
- Keep your API key secure, as it provides access to your account's data.
For more detailed instructions, refer to the NetHunt API key documentation.
Understanding NetHunt API Authentication
NetHunt uses Basic authentication for API requests. This involves encoding your email and API key in base64 format. Here's how to set it up:
// Example of encoding email and API key
const email = 'your-email@example.com';
const apiKey = 'your-api-key';
const authString = btoa(`${email}:${apiKey}`);
const headers = {
'Authorization': `Basic ${authString}`,
'Content-Type': 'application/json'
};
Replace your-email@example.com
and your-api-key
with your actual email and API key.
For more information on authentication, visit the NetHunt API documentation.
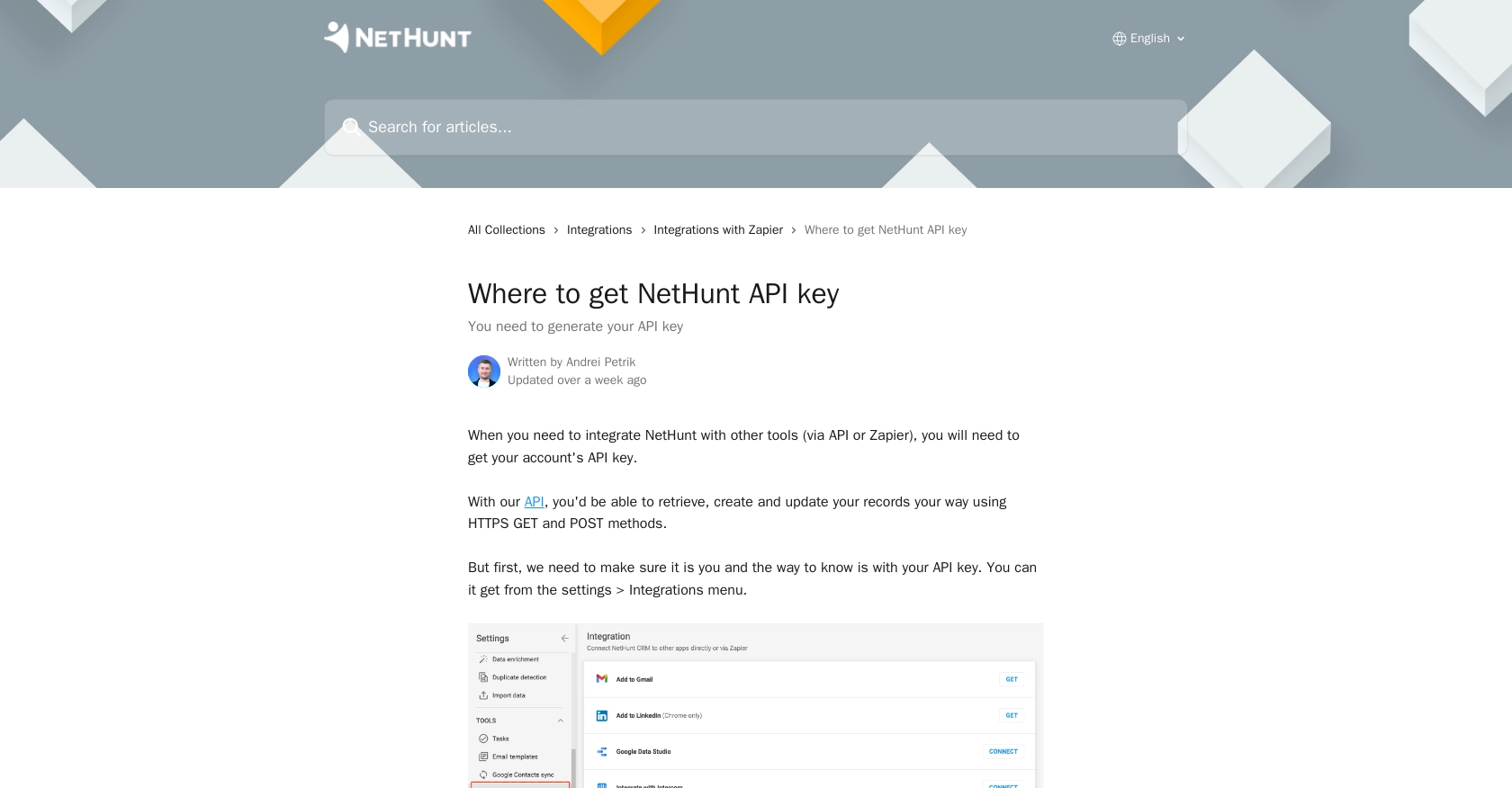
sbb-itb-96038d7
Making API Calls to Retrieve NetHunt Records Using JavaScript
To interact with the NetHunt API and retrieve records, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for NetHunt API Integration
Before you begin, ensure you have a modern JavaScript environment set up. You can use Node.js or a browser-based environment. For this tutorial, we'll use Node.js. Make sure you have Node.js installed on your machine.
- Download and install Node.js if you haven't already.
- Create a new project directory and navigate into it.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library for making HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Fetch Records from NetHunt CRM
Now that your environment is ready, let's write the code to fetch records from NetHunt CRM using the API.
const axios = require('axios');
// Your NetHunt API credentials
const email = 'your-email@example.com';
const apiKey = 'your-api-key';
// Encode credentials in base64
const authString = Buffer.from(`${email}:${apiKey}`).toString('base64');
// Set up the request headers
const headers = {
'Authorization': `Basic ${authString}`,
'Content-Type': 'application/json'
};
// Define the API endpoint to fetch records
const endpoint = 'https://nethunt.com/api/v1/zapier/searches/find-record/{folderId}?query=Name%3ADoe&limit=10';
// Function to fetch records
async function fetchRecords() {
try {
const response = await axios.get(endpoint, { headers });
const records = response.data;
console.log('Fetched Records:', records);
} catch (error) {
console.error('Error fetching records:', error.response ? error.response.data : error.message);
}
}
// Call the function to fetch records
fetchRecords();
Replace your-email@example.com
and your-api-key
with your actual NetHunt email and API key. Also, replace {folderId}
with the specific folder ID you wish to query.
Verifying Successful API Requests and Handling Errors
After running the code, you should see the fetched records printed in the console. To verify the request's success, check the response status code. A status code of 200
indicates a successful request.
If the request fails, the error handling in the code will log the error message. Common error codes include:
- 401 Unauthorized: Check your API key and email for correctness.
- 404 Not Found: Ensure the endpoint URL and folder ID are correct.
- 500 Internal Server Error: This may indicate an issue with the NetHunt server.
For more detailed error information, refer to the NetHunt API documentation.
Checking Results in Your NetHunt CRM Sandbox
To confirm that your API call is working as expected, you can log into your NetHunt CRM account and verify the records returned by the API match those in your sandbox environment. This ensures that your integration is correctly fetching data.
Conclusion and Best Practices for NetHunt API Integration in JavaScript
Integrating with the NetHunt API using JavaScript allows developers to enhance CRM functionalities by automating data retrieval and management. By following the steps outlined in this guide, you can efficiently interact with NetHunt CRM and customize your integration to suit specific business needs.
Best Practices for Secure and Efficient NetHunt API Usage
- Secure Storage of API Credentials: Always store your API key and email securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handling Rate Limits: Be mindful of any rate limits imposed by the API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from NetHunt is standardized and transformed as needed to integrate seamlessly with other systems.
Enhancing Integration Capabilities with Endgrate
While integrating with NetHunt API directly is powerful, using a tool like Endgrate can further streamline the process. Endgrate provides a unified API endpoint that connects to multiple platforms, allowing you to manage integrations more efficiently. By leveraging Endgrate, you can focus on your core product development while outsourcing complex integration tasks.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover how companies have scaled their integrations effortlessly.
Read More
Ready to get started?