How to Get Records with the Google Sheets API in Javascript
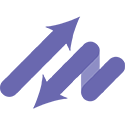
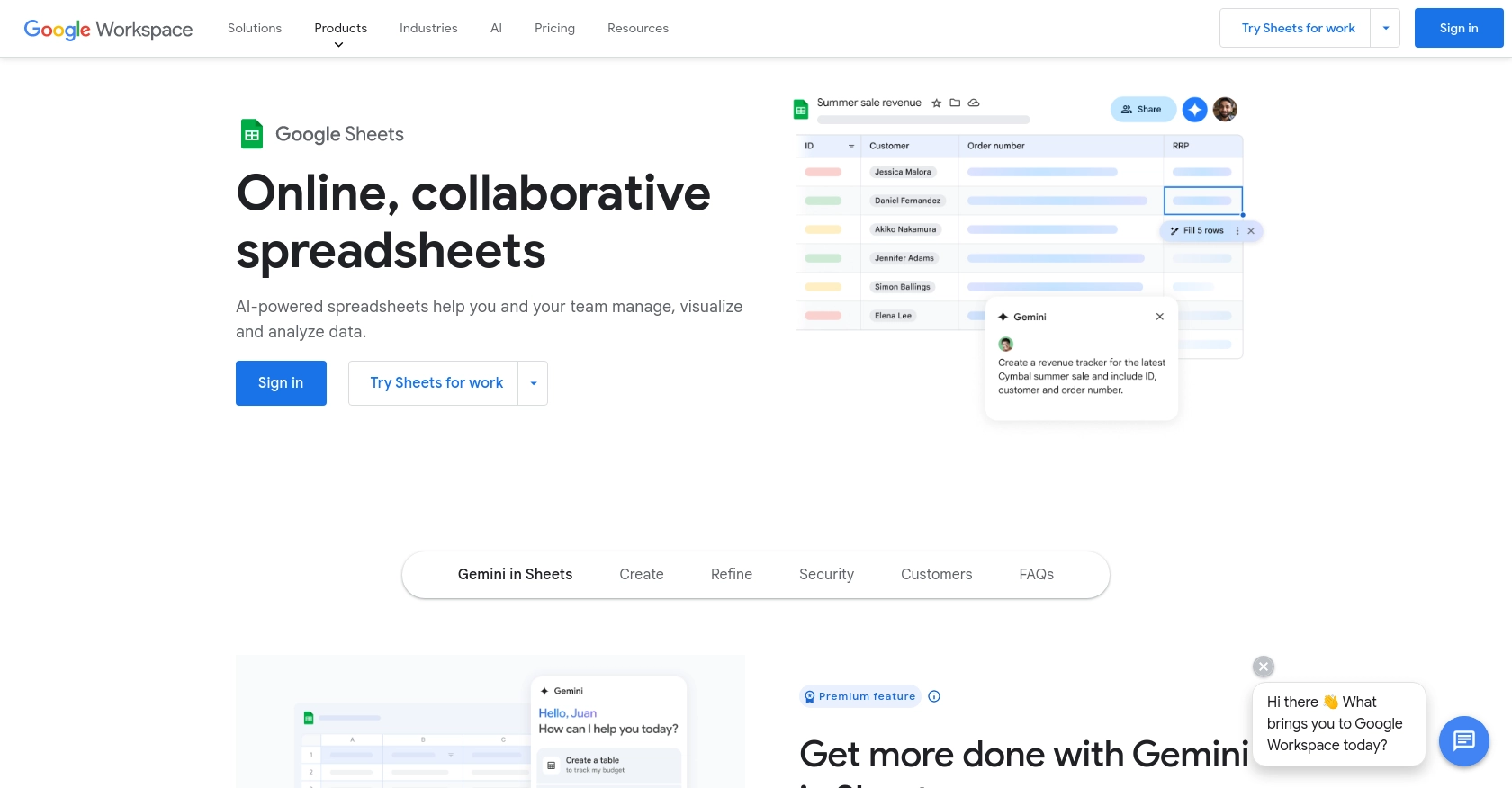
Introduction to Google Sheets API
Google Sheets is a powerful, cloud-based spreadsheet application that allows users to create, edit, and collaborate on spreadsheets in real-time. It is part of the Google Workspace suite of productivity tools and is widely used by businesses and individuals for data management and analysis.
Integrating with the Google Sheets API enables developers to programmatically access and manipulate spreadsheet data, facilitating automation and enhancing productivity. For example, a developer might use the Google Sheets API to retrieve data from a spreadsheet and display it on a web application, or to update spreadsheet records based on external data sources.
This article will guide you through the process of using JavaScript to interact with the Google Sheets API, focusing on retrieving records from a spreadsheet. By the end of this tutorial, you will be able to efficiently access and manage spreadsheet data using JavaScript.
Setting Up Your Google Sheets API Test Environment
Before you can start interacting with the Google Sheets API using JavaScript, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup will allow you to securely access and manage your Google Sheets data programmatically.
Create a Google Cloud Project
- Go to the Google Cloud Console.
- Click on the menu icon and navigate to IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Sheets API
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Sheets API" and click on it.
- Click the Enable button to activate the API for your project.
Configure OAuth Consent Screen
- In the Google Cloud Console, go to APIs & Services > OAuth consent screen.
- Select the user type and fill out the required information, such as application name and support email.
- Click Save and Continue.
Create OAuth 2.0 Credentials
- Navigate to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Under Authorized redirect URIs, add the URI where your application will handle the OAuth callback.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you'll need them to authenticate your API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Access Credentials.
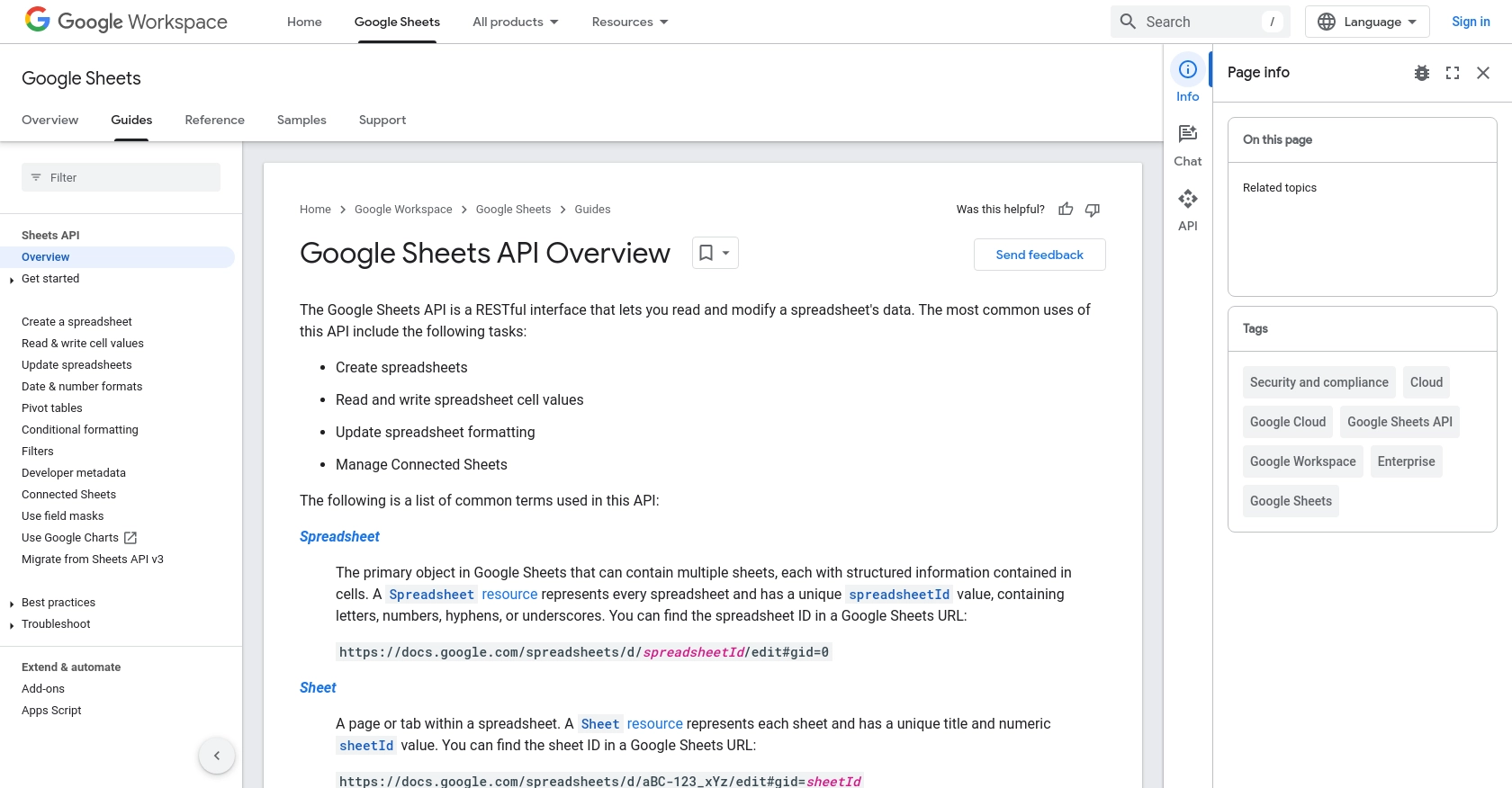
sbb-itb-96038d7
Making API Calls to Retrieve Google Sheets Records Using JavaScript
To interact with the Google Sheets API using JavaScript, you'll need to set up your environment and write code to make API requests. This section will guide you through the necessary steps to retrieve records from a Google Sheet using JavaScript.
Prerequisites for Google Sheets API Integration with JavaScript
Before you begin, ensure you have the following installed on your machine:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Once you have these installed, you can proceed to set up the necessary dependencies for your project.
Install Required Node.js Packages
Open your terminal and navigate to your project directory. Run the following command to initialize a new Node.js project:
npm init -y
Next, install the Google APIs client library for Node.js:
npm install googleapis
Writing JavaScript Code to Retrieve Google Sheets Data
Create a new file named getGoogleSheetsData.js
and add the following code to it:
const { google } = require('googleapis');
const sheets = google.sheets('v4');
const { OAuth2 } = google.auth;
// Replace with your client ID, client secret, and redirect URI
const oauth2Client = new OAuth2(
'YOUR_CLIENT_ID',
'YOUR_CLIENT_SECRET',
'YOUR_REDIRECT_URI'
);
// Set the access token
oauth2Client.setCredentials({
access_token: 'YOUR_ACCESS_TOKEN',
});
// Define the spreadsheet ID and range
const spreadsheetId = 'YOUR_SPREADSHEET_ID';
const range = 'Sheet1!A1:D10';
// Function to get data from Google Sheets
async function getSheetData() {
try {
const response = await sheets.spreadsheets.values.get({
auth: oauth2Client,
spreadsheetId,
range,
});
const rows = response.data.values;
if (rows.length) {
console.log('Data retrieved from Google Sheets:');
rows.forEach((row) => {
console.log(row);
});
} else {
console.log('No data found.');
}
} catch (error) {
console.error('Error retrieving data:', error);
}
}
getSheetData();
Replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_REDIRECT_URI
, YOUR_ACCESS_TOKEN
, and YOUR_SPREADSHEET_ID
with your actual credentials and spreadsheet details.
Executing the JavaScript Code
Run the following command in your terminal to execute the script and retrieve data from your Google Sheet:
node getGoogleSheetsData.js
If successful, the script will output the data from the specified range in your Google Sheet.
Handling Errors and Verifying API Requests
Ensure that your API requests are successful by checking the response status and handling any errors that may occur. Common error codes include 400 (Bad Request), 401 (Unauthorized), and 403 (Forbidden). For more information on error handling, refer to the Google Sheets API documentation.
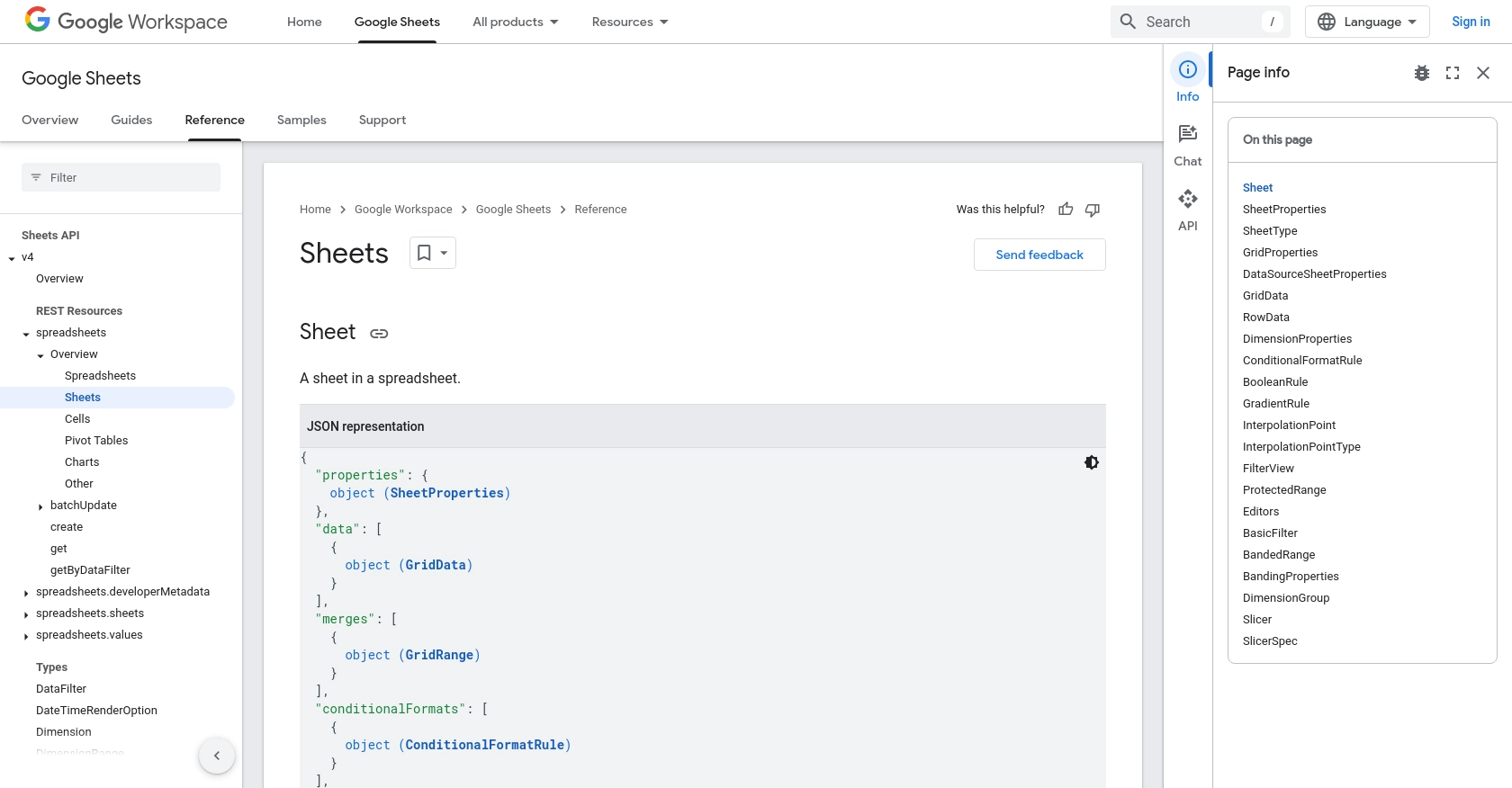
Conclusion and Best Practices for Using Google Sheets API with JavaScript
Integrating with the Google Sheets API using JavaScript can significantly enhance your ability to manage and automate spreadsheet data. By following the steps outlined in this tutorial, you can efficiently retrieve and manipulate records from Google Sheets, streamlining your data workflows.
Best Practices for Secure and Efficient Google Sheets API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the Google Sheets API rate limits. Implement exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the Google Sheets API documentation.
- Optimize Data Requests: Limit the range of data you request to only what is necessary. This reduces the load on the API and improves the performance of your application.
- Implement Error Handling: Ensure robust error handling in your application to manage API errors effectively. Log errors for debugging and provide user-friendly messages when issues occur.
Call to Action: Simplify Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to streamline your processes. Endgrate provides a unified API endpoint that connects to various platforms, including Google Sheets, allowing you to focus on your core product while outsourcing integration complexities. Visit Endgrate to learn more about how you can save time and resources by simplifying your integration needs.
Read More
- https://endgrate.com/provider/googlesheets
- https://developers.google.com/sheets/api/guides/concepts
- https://developers.google.com/sheets/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/sheets
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/cells
Ready to get started?