How to Create or Update People with the PipelineCRM API in PHP
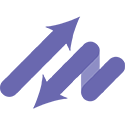
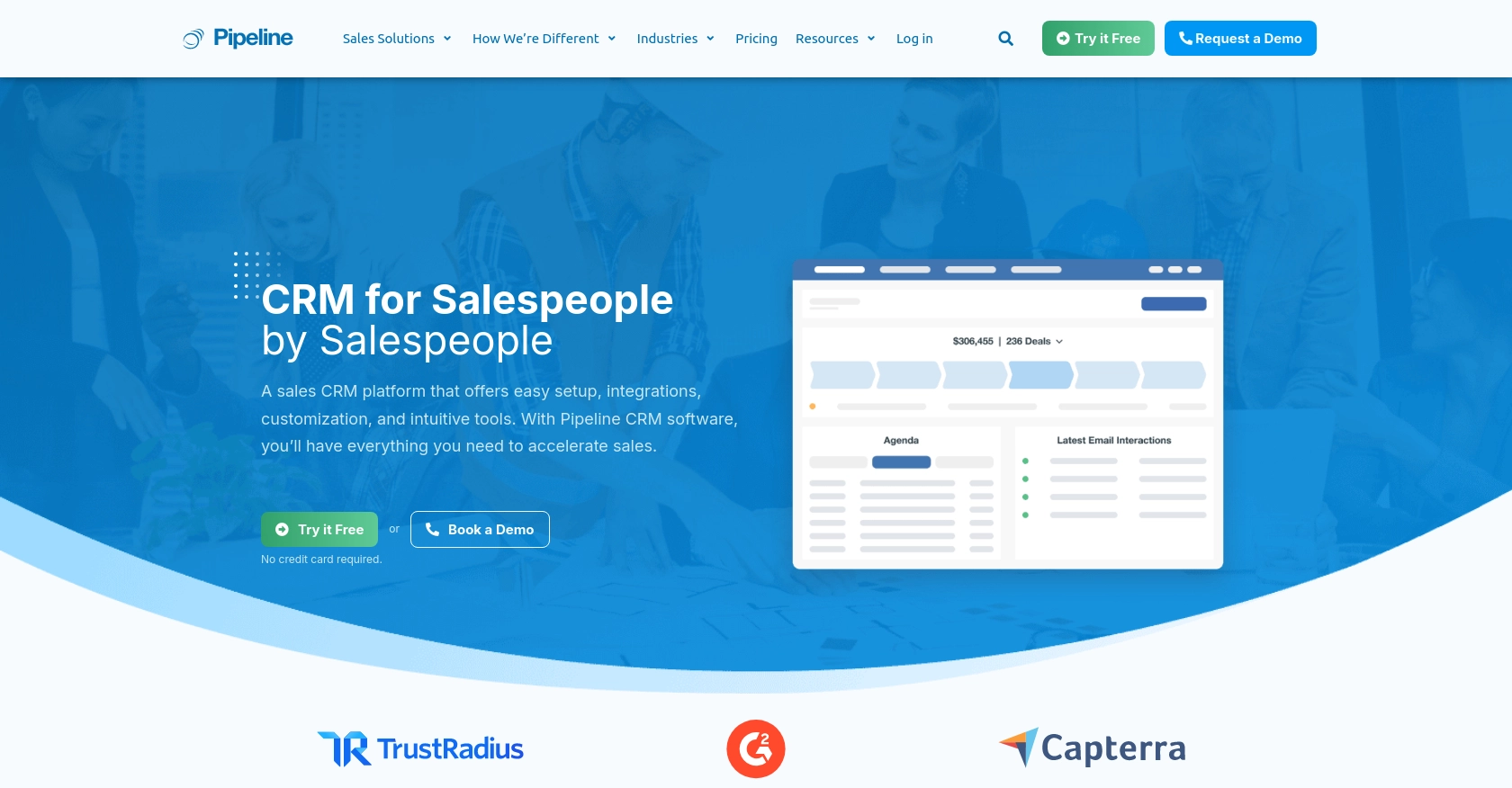
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. With features like contact management, sales tracking, and reporting, PipelineCRM is a valuable tool for sales teams looking to streamline their workflows and improve customer interactions.
Developers may want to integrate with PipelineCRM's API to automate and enhance their sales processes. For example, using the PipelineCRM API, a developer can create or update contact information directly from an external application, ensuring that sales teams have the most up-to-date data at their fingertips.
Setting Up Your PipelineCRM Test Account
Before you can start integrating with the PipelineCRM API, you'll need to set up a test account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a PipelineCRM Account
- Visit the PipelineCRM website and sign up for a free trial or demo account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the PipelineCRM dashboard.
Generate Your API Key for Authentication
PipelineCRM uses API key-based authentication to secure API requests. Here's how to generate your API key:
- Navigate to the account settings in your PipelineCRM dashboard.
- Locate the API section and click on "Generate API Key."
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
With your test account and API key ready, you can now proceed to make API calls to create or update people in PipelineCRM using PHP.
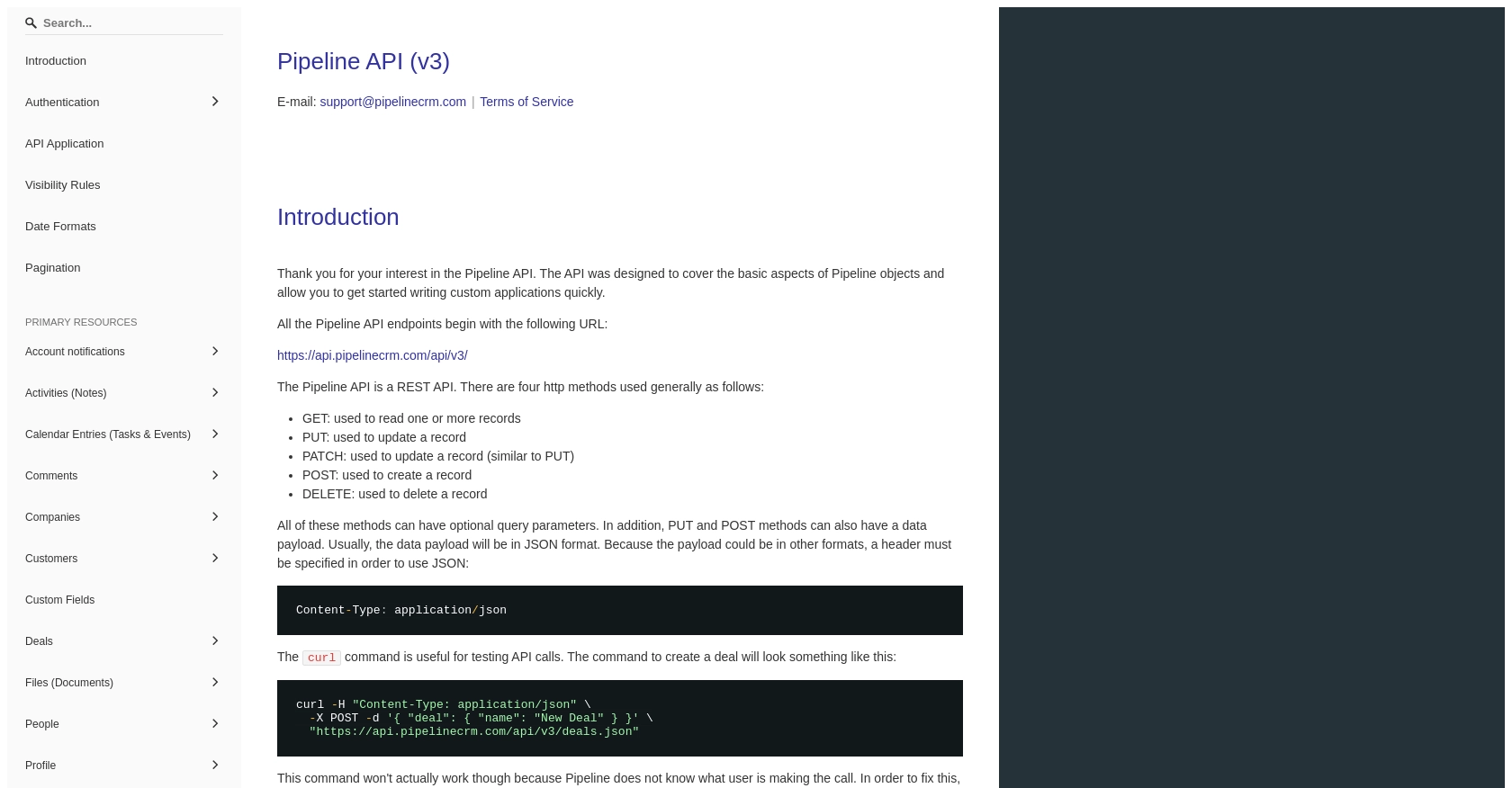
sbb-itb-96038d7
Making API Calls to Create or Update People in PipelineCRM Using PHP
To interact with the PipelineCRM API and manage people records, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, making API calls, and handling responses effectively.
Setting Up Your PHP Environment for PipelineCRM API
Before you begin, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for dependency management
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Creating or Updating People in PipelineCRM
With your environment set up, you can now write the PHP code to create or update people in PipelineCRM. Below is a sample script demonstrating how to perform these actions:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Set your API key
$apiKey = 'Your_API_Key';
// Define the API endpoint for creating or updating people
$endpoint = 'https://api.pipelinecrm.com/api/v3/people';
// Prepare the data for the person you want to create or update
$personData = [
'first_name' => 'John',
'last_name' => 'Doe',
'email' => 'john.doe@example.com',
'phone' => '123-456-7890'
];
try {
// Make the API request
$response = $client->request('POST', $endpoint, [
'headers' => [
'Authorization' => "Bearer $apiKey",
'Content-Type' => 'application/json'
],
'json' => $personData
]);
// Check if the request was successful
if ($response->getStatusCode() == 201) {
echo "Person created or updated successfully.";
} else {
echo "Failed to create or update person.";
}
} catch (Exception $e) {
// Handle errors
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This script initializes a Guzzle client, sets up the necessary headers, and sends a POST request to the PipelineCRM API to create or update a person.
Verifying API Call Success in PipelineCRM
After running the script, you can verify the success of the API call by checking your PipelineCRM dashboard. The new or updated person should appear in your people list. If you encounter any errors, ensure that your API key and endpoint URL are correct.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors gracefully. Common error codes you might encounter include:
- 400 Bad Request: The request was malformed. Check your data format.
- 401 Unauthorized: Authentication failed. Verify your API key.
- 404 Not Found: The endpoint URL is incorrect.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Refer to the PipelineCRM API documentation for more detailed information on error handling.
Conclusion and Best Practices for Using PipelineCRM API in PHP
Integrating with the PipelineCRM API using PHP allows developers to efficiently manage and update contact information, streamlining sales processes and ensuring data accuracy. By following the steps outlined in this guide, you can create or update people records in PipelineCRM with ease.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized before sending them to the API to maintain consistency and prevent errors.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users.
Streamlining Integrations with Endgrate
While integrating with individual APIs like PipelineCRM can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including PipelineCRM.
By leveraging Endgrate, you can focus on your core product development while outsourcing integration tasks. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?