How to Get Users with the Microsoft Dynamics 365 API in PHP
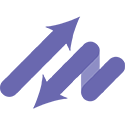
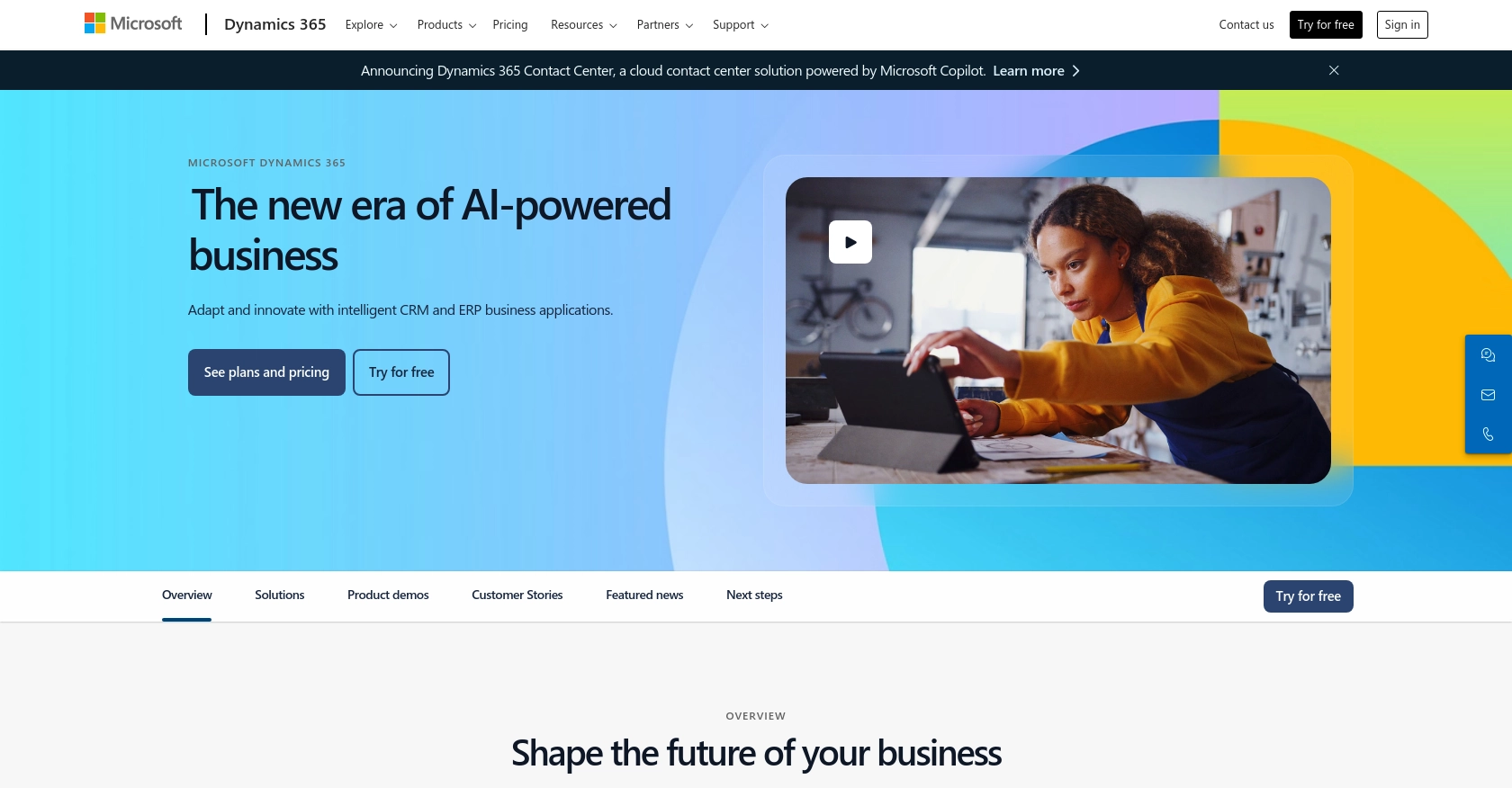
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications designed to streamline operations across various domains such as sales, customer service, and finance. It offers a unified platform that integrates CRM and ERP capabilities, enabling businesses to enhance productivity and drive growth.
For developers, integrating with the Microsoft Dynamics 365 API can unlock powerful capabilities to manage user data and automate business processes. By accessing user information through the API, developers can create custom solutions that enhance user management and improve operational efficiency.
An example use case is retrieving user data to synchronize with an external system, ensuring that user profiles are consistently updated across platforms. This integration can help businesses maintain accurate records and improve collaboration.
Setting Up a Microsoft Dynamics 365 Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you need to set up a sandbox account. This environment allows you to test your integration without affecting live data, ensuring a smooth development process.
Creating a Microsoft Dynamics 365 Free Trial Account
To begin, you need to create a free trial account with Microsoft Dynamics 365. Follow these steps:
- Visit the Microsoft Dynamics 365 Free Trial page.
- Click on the "Try for free" button and fill out the registration form with your details.
- Follow the instructions to complete the setup of your trial account.
Once your account is set up, you will have access to a sandbox environment where you can test your API integrations.
Registering an Application in Microsoft Entra ID
To use OAuth authentication with the Microsoft Dynamics 365 API, you must register an application in Microsoft Entra ID. This process involves creating an app that will allow you to obtain the necessary credentials for API access.
- Navigate to the Azure Portal and sign in with your Microsoft account.
- Go to "Azure Active Directory" and select "App registrations" from the sidebar.
- Click on "New registration" and fill in the required fields, such as the name of your app and the redirect URI.
- Choose the appropriate account type based on your application's needs.
- Click "Register" to create your application.
Configuring OAuth Credentials for Microsoft Dynamics 365
After registering your application, you need to configure OAuth credentials to authenticate API requests:
- In the app registration page, navigate to "Certificates & secrets" and create a new client secret. Make sure to copy the secret value as it will not be displayed again.
- Go to "API permissions" and click "Add a permission."
- Select "Dynamics 365" and choose the permissions your app requires, such as "Access Dynamics 365 as organization users."
- Grant admin consent for the permissions if necessary.
With these credentials, you can now authenticate your API requests using OAuth.
For more detailed information on setting up OAuth authentication, refer to the official documentation: Use OAuth authentication with Microsoft Dataverse.
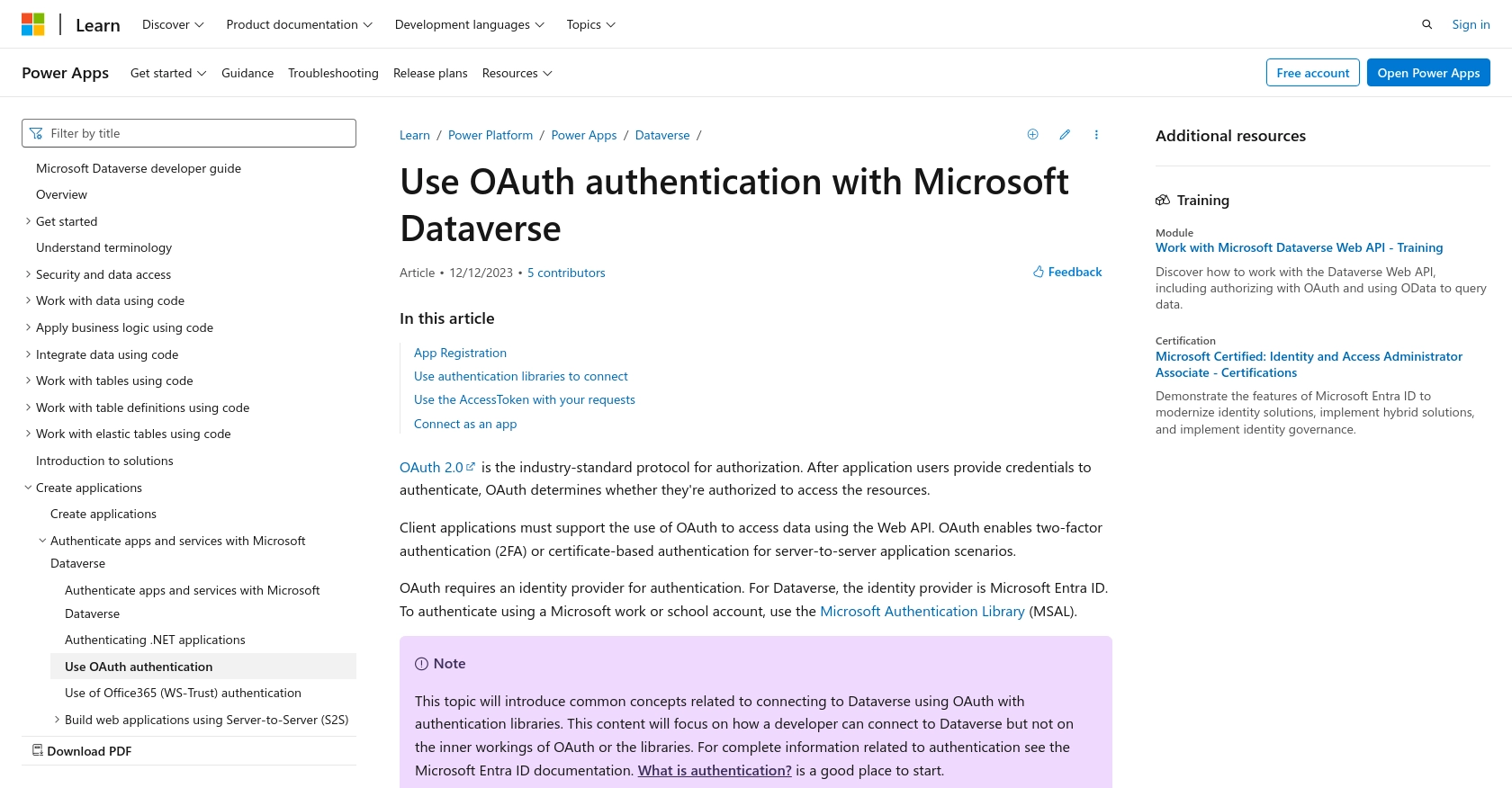
sbb-itb-96038d7
Making API Calls to Retrieve Users with Microsoft Dynamics 365 API in PHP
To interact with the Microsoft Dynamics 365 API using PHP, you need to ensure your development environment is properly set up. This includes having the correct version of PHP and necessary libraries installed. In this section, we will guide you through the process of making API calls to retrieve user data from Microsoft Dynamics 365.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- Composer for managing dependencies
To install the required libraries, use Composer to add the Guzzle HTTP client, which simplifies making HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Call Microsoft Dynamics 365 API
With your environment set up, you can now write PHP code to interact with the Microsoft Dynamics 365 API. Below is a sample script to retrieve users:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
use GuzzleHttp\Exception\RequestException;
// Set up the HTTP client
$client = new Client();
// Define the API endpoint and headers
$endpoint = 'https://yourorg.api.crm.dynamics.com/api/data/v9.2/systemusers';
$accessToken = 'Your_Access_Token'; // Replace with your access token
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'OData-MaxVersion' => '4.0',
'OData-Version' => '4.0',
'Accept' => 'application/json'
];
try {
// Make a GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Parse the JSON data from the response
$data = json_decode($response->getBody(), true);
// Loop through the users and print their information
foreach ($data['value'] as $user) {
echo 'User ID: ' . $user['systemuserid'] . ' - Name: ' . $user['fullname'] . "\n";
}
} catch (RequestException $e) {
// Handle errors
echo 'Request failed: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token obtained during OAuth authentication. This script uses Guzzle to send a GET request to the Microsoft Dynamics 365 API endpoint for users. It then parses the response and prints out user IDs and names.
Verifying API Call Success and Handling Errors
After running the script, verify the success of your API call by checking the output. The retrieved user data should match the users in your Microsoft Dynamics 365 sandbox environment.
In case of errors, the script will catch exceptions and print an error message. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 403 Forbidden: Verify that your app has the necessary permissions.
- 404 Not Found: Ensure the endpoint URL is correct.
For more detailed error handling, refer to the official documentation: systemuser EntityType (Microsoft.Dynamics.CRM).
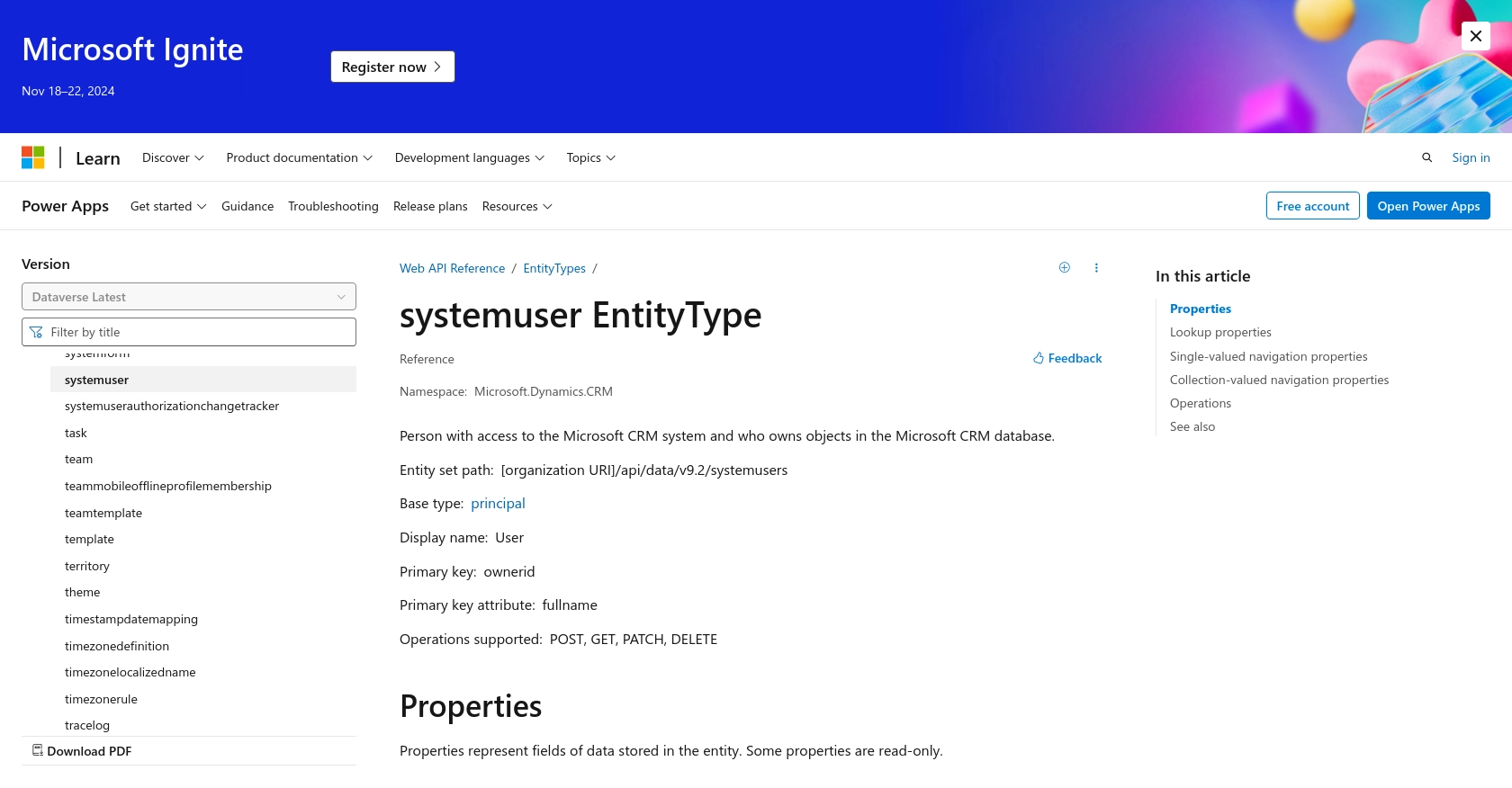
Conclusion and Best Practices for Using Microsoft Dynamics 365 API in PHP
Integrating with the Microsoft Dynamics 365 API using PHP provides developers with powerful tools to manage and automate user data processes. By following the steps outlined in this guide, you can efficiently retrieve user information and enhance your business operations.
Best Practices for Managing Microsoft Dynamics 365 API Integrations
- Securely Store Credentials: Always store OAuth credentials, such as client secrets and access tokens, securely in your environment. Avoid hardcoding them in your scripts.
- Handle Rate Limiting: Be aware of API rate limits to prevent throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that data retrieved from Microsoft Dynamics 365 is standardized and transformed as needed to maintain consistency across systems.
- Monitor API Usage: Regularly monitor API usage and performance to identify any issues or opportunities for optimization.
Streamline Your Integration Process with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Microsoft Dynamics 365. By leveraging Endgrate, you can focus on your core product while ensuring a seamless integration experience for your customers.
Visit Endgrate to learn more about how you can save time and resources by outsourcing your integration needs.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/systemuser?view=dataverse-latest
Ready to get started?