Using the Hubspot API to Create Products (Professional/Enterprise Only) in PHP
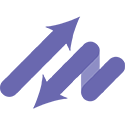
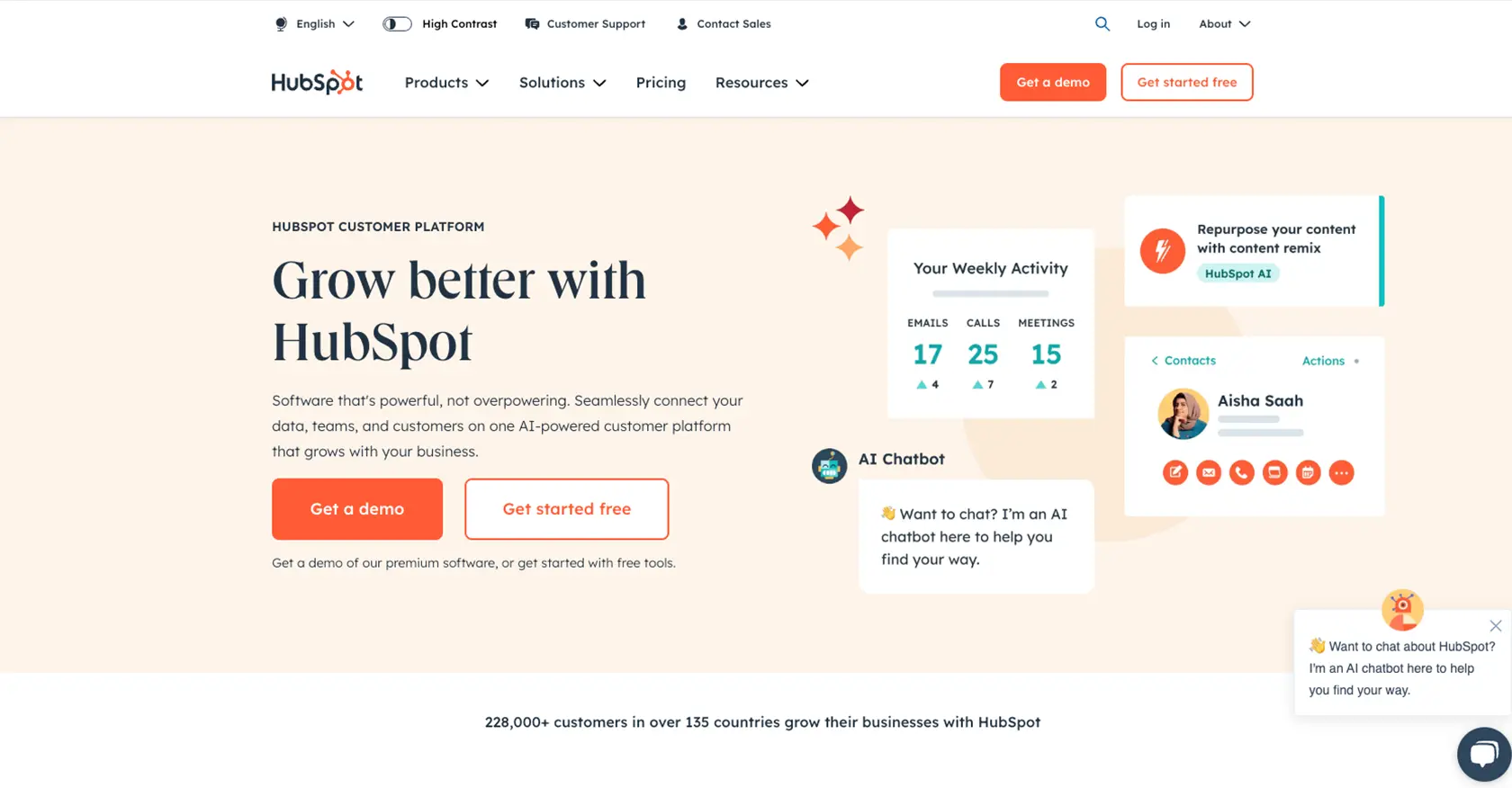
Introduction to HubSpot API for Product Management
HubSpot is a powerful CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Its robust API allows developers to integrate HubSpot's functionalities into their own applications, enabling seamless data exchange and automation.
For developers working with B2B SaaS products, integrating with HubSpot's API can significantly enhance product management capabilities. By leveraging the API, developers can create, update, and manage product information directly within HubSpot, streamlining the process of maintaining accurate product data across platforms.
In this article, we will explore how to use the HubSpot API to create products using PHP. This integration is particularly beneficial for businesses operating on HubSpot's Professional or Enterprise tiers, as it allows for efficient product data management and synchronization.
Imagine a scenario where a developer needs to automate the addition of new products from an external inventory system into HubSpot. By using the HubSpot API, this process can be automated, ensuring that product information is always up-to-date and reducing the risk of manual errors.
Setting Up Your HubSpot Developer Account for API Access
Before you can start creating products using the HubSpot API, you'll need to set up a developer account and create a private app. This will allow you to authenticate your API requests using OAuth, ensuring secure access to HubSpot's resources.
Step-by-Step Guide to Creating a HubSpot Developer Account
-
Sign Up for a Developer Account:
Visit the HubSpot Developer Portal and sign up for a developer account. This account will give you access to HubSpot's API documentation and tools.
-
Create a Test Account:
Once your developer account is set up, create a test account. This sandbox environment will allow you to safely test your API integrations without affecting live data.
Creating a HubSpot Private App for OAuth Authentication
-
Navigate to Private Apps:
In your HubSpot account, go to the settings icon in the main navigation bar. From the left sidebar menu, select Integrations > Private Apps.
-
Create a New Private App:
Click on Create a private app. Fill in the necessary details such as the app name and description.
-
Set Scopes for Product Management:
Under the Scopes section, ensure you select the appropriate scopes that allow access to product management features. This is crucial for enabling your app to interact with the products API.
-
Generate an Access Token:
After setting the scopes, click on Create app. You will receive an access token, which you should store securely. This token will be used to authenticate your API requests.
With your developer account and private app set up, you're now ready to start making API calls to create products in HubSpot using PHP. For more details on authentication, refer to the HubSpot Authentication Documentation.
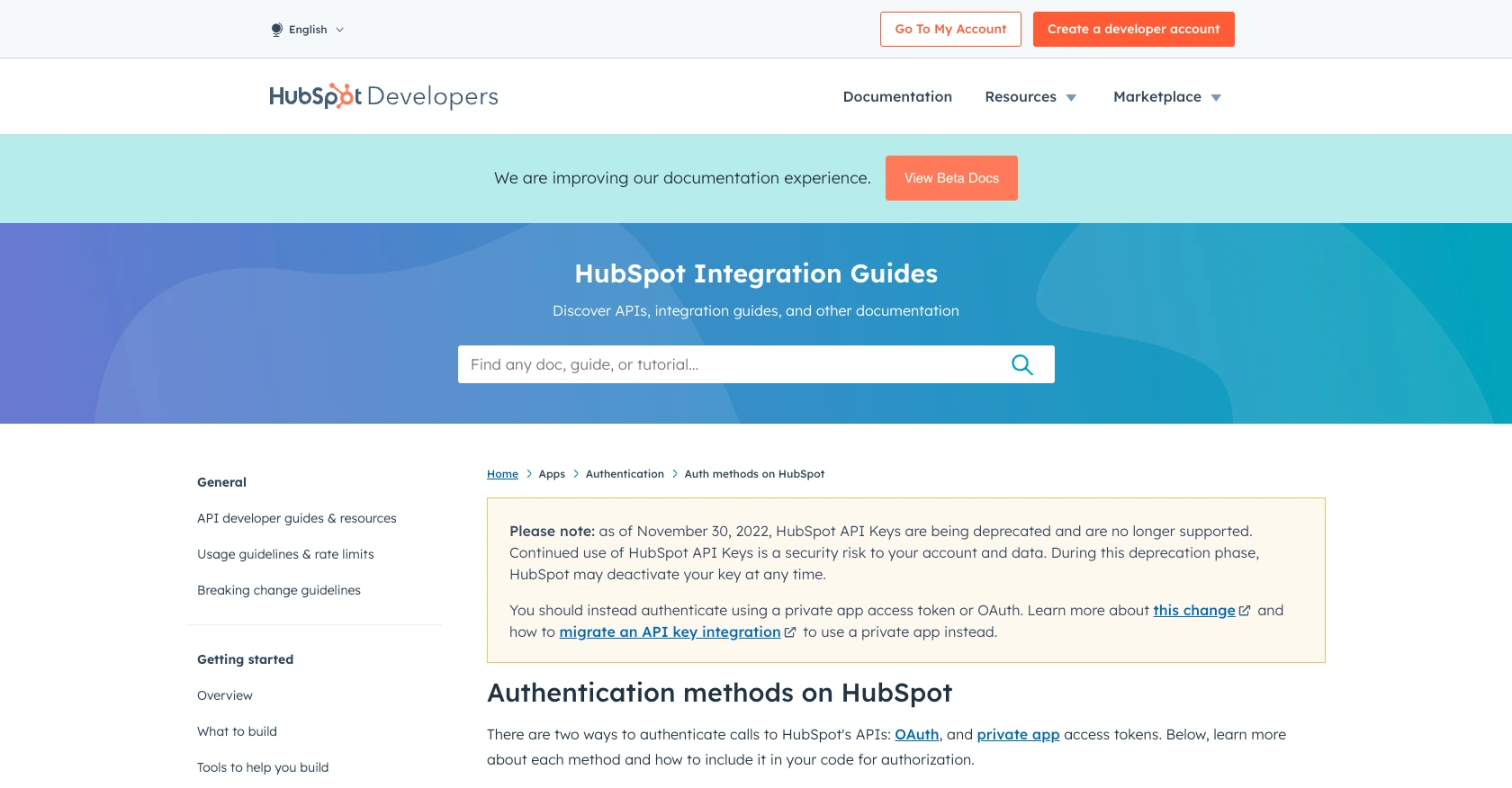
sbb-itb-96038d7
How to Make the HubSpot API Call to Create Products Using PHP
To create products in HubSpot using PHP, you'll need to set up your environment and write the necessary code to interact with the HubSpot API. This section will guide you through the process, including setting up PHP, installing dependencies, and writing the code to make the API call.
Setting Up Your PHP Environment for HubSpot API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, use Composer to install the HubSpot API client library by running the following command in your terminal:
composer require hubspot/api-client
Writing the PHP Code to Create Products in HubSpot
With your environment set up, you can now write the PHP code to create a product in HubSpot. Create a file named create_hubspot_product.php
and add the following code:
<?php
require 'vendor/autoload.php';
use HubSpot\Factory;
use HubSpot\Client\Crm\Products\ApiException;
// Initialize the HubSpot client
$client = Factory::createWithAccessToken('Your_Access_Token');
// Define the product properties
$productProperties = [
'name' => 'Example Product',
'description' => 'This is a sample product created via the HubSpot API.',
'price' => 100.00
];
try {
// Create the product
$product = $client->crm()->products()->basicApi()->create(['properties' => $productProperties]);
echo "Product created successfully with ID: " . $product->getId();
} catch (ApiException $e) {
echo "Exception when calling HubSpot API: ", $e->getMessage(), PHP_EOL;
}
Replace Your_Access_Token
with the access token you generated earlier. This code initializes the HubSpot client, defines the product properties, and makes an API call to create the product.
Verifying the API Call and Handling Errors
After running the script, you should see a message indicating the successful creation of the product, along with its ID. To verify, log in to your HubSpot account and check the products section to see the newly created product.
If the API call fails, the script will catch the exception and display an error message. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and has the necessary scopes.
- 429 Too Many Requests: You have hit the rate limit. Refer to the HubSpot API Usage Guidelines for more information on rate limits.
For more details on error handling, consult the HubSpot Authentication Documentation.
.webp)
Conclusion and Best Practices for Using HubSpot API with PHP
Integrating with the HubSpot API to manage products using PHP offers a powerful way to streamline product data management within your business processes. By automating the creation and synchronization of product information, you can ensure data accuracy and reduce manual errors.
When working with the HubSpot API, it's crucial to follow best practices to maintain security and efficiency:
- Securely Store Access Tokens: Always store your access tokens securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of HubSpot's rate limits, which are 150 requests per 10 seconds for Professional and Enterprise accounts. Implement request throttling to avoid hitting these limits. For more details, refer to the HubSpot API Usage Guidelines.
- Implement Error Handling: Properly handle API errors by catching exceptions and implementing retry logic where appropriate. This ensures your application can gracefully recover from temporary issues.
- Use Granular Scopes: When setting up your private app, choose the most specific scopes necessary for your integration to minimize access and enhance security.
By adhering to these practices, you can build robust integrations that enhance your product management capabilities within HubSpot.
For developers looking to simplify and scale their integration efforts, consider leveraging Endgrate. Endgrate allows you to build once for each use case and connect to multiple platforms with ease, saving time and resources. Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/products
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?